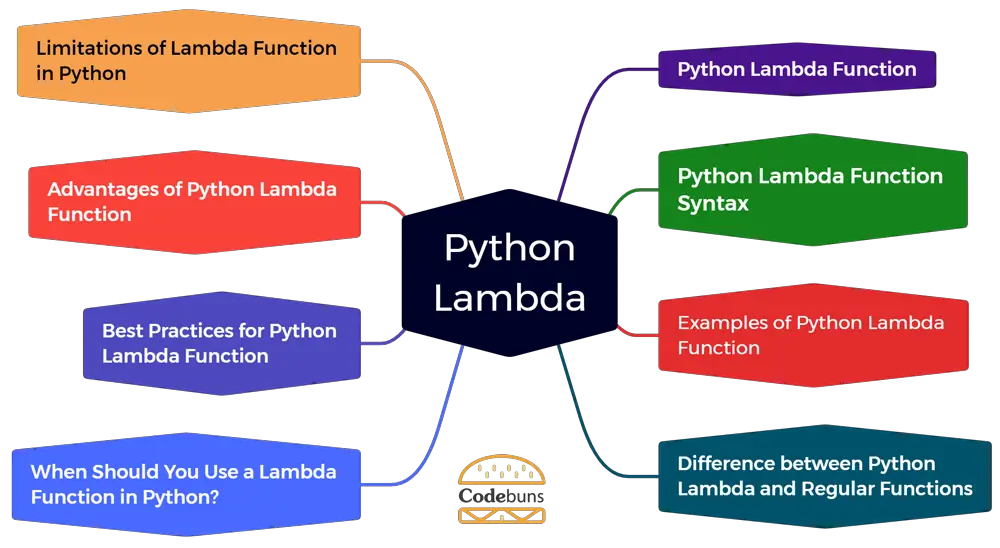
Table of Contents | |
Python Lambda Function
Python Lambda function is defined with the keyword lambda, followed by a set of parameters, a colon (:), and an expression that the function will return. These functions are anonymous, meaning they don’t have a name (nameless function) like regular functions defined with the def keyword. Lambda functions are also known as inline functions or function literals. They are commonly used for creating short functions that can be passed as arguments to other functions.
Example of Python Lambda Function
Let’s create a simple Python lambda function that calculates the square of a given number:
square = lambda x: x * x
print(square(5))
Explanation
square
: This is the name of the lambda function.lambda
: This keyword indicates that we are creating a lambda function.x
: This is the argument for our lambda function, which represents the number we want to square.:
(colon): This separates the list of arguments from the expression.x * x
: This is the expression that calculates the square of x.print(square(5))
: We call the square function with an argument of 5. The function calculates 5 * 5 and returns 25, which is then printed.
Python Lambda Function Syntax
Python lambda function syntax starts with the lambda keyword, which is unique to these anonymous functions. Following the lambda keyword are the arguments similar to the parameters declared in a regular function. After the arguments, a colon (:) separates the parameter list from the expression. This expression, which comes after the colon, is the function’s return value when executed. Unlike regular Python functions, lambda functions are limited to a single expression and cannot contain multiple statements or commands.
Syntax of Python Lambda Function
lambda arguments: expression
Explanation
- The keyword lambda indicates that we are creating an anonymous function.
- The arguments refer to the input parameters of the function, which can be zero or more.
- The expression is the single statement that is executed when the function is called.
Example of Lambda Function in Python
Here is a simple example of a lambda function:
add = lambda x, y: x + y
Explanation
add
: This is the name assigned to the lambda function.lambda
: This is the keyword used to define a lambda function in Python.x, y
: These are the parameters of the lambda function.- (
:
) colon: This symbol separates the parameters from the expression or code block of the lambda function. x + y
: This is the expression or code block the lambda function will execute. In this case, it adds the two parameters x and y.
Now, let’s see how this lambda function can be used:
result = add(5, 3)
print(result) # Output: 8
Explanation
result
: This is the name assigned to the value the lambda function will return.add(5, 3)
: This statement calls the lambda function with the parameters 5 and 3, which will result in the expression being evaluated as 5 + 3.print(result)
: This statement prints the value of result.# Output: 8
: This comment shows the expected output of the code, which is 8.
Examples of Python Lambda Function
Simple Arithmetic
One simple example of a Python lambda function is for performing addition. Using the lambda keyword, we can define a function that takes two arguments and returns their sum. This allows us to perform quick calculations without defining a full-fledged function.
Syntax
add = lambda a, b: a + b
Explanation
- add is the name of the lambda function.
- lambda a, b: Declares a lambda function with two parameters a and b.
- : separates the parameters from the return statement.
- a + b is the expression or code executed, and the result is returned.
Example
add = lambda a, b: a + b
print(add(5, 3))
Explanation
add(5, 3)
: Calls the lambda function with 5 and 3 as arguments, returning 8.- This is because the function takes 5 as the value for a, and 3 as the value for b, returning the sum of both values.
print(...)
: Outputs the result of the addition.
Using Python Lambda as an Argument
One interesting use case for lambda functions is using them as arguments when calling other functions. Since lambda functions are just small snippets of code, they can be passed in as an argument without defining them separately.
When using a Python lambda function as an argument, the function call is made directly within the parentheses of the higher-order function. This allows for more concise and cleaner code as there is no need for extra lines to define and call a regular function.
Example Part 1
def apply_operation(x, operation):
return operation(x)
Explanation
def apply_operation(x, operation):
: Defines a function that takes two arguments:x
: A value to apply an operation to.operation
: A function that performs the operation.
return operation(x)
: Calls the provided operation function with x and returns the result.
Example Part 2
result = apply_operation(4, lambda x: x * x) # Square a number
print(result)
Explanation
apply_operation(4, lambda x: x * x)
:- Calls apply_operation with:
x = 4
: The value to operate on.operation = lambda x: x * x
: A lambda function squaring its argument.
- Calls apply_operation with:
print(result)
: Prints the result (16, the square of 4).
Using Python Lambda Function with sorted()
The sorted() function in Python is used for sorting any iterable object, such as lists, tuples, dictionaries, etc. It takes an optional parameter called key, which specifies a function used to extract the comparison key from each element in the iterable. This is where Python Lambda functions come in handy.
A Python Lambda function can be defined on-the-fly and passed as an argument to the sorted() function, allowing for quick and efficient data sorting without having to define a separate named function. For example, we can sort a list of strings based on their lengths using a lambda function like lambda x: len(x).
Syntax
sorted(iterable, key=lambda x: [sorting_criteria])
Explanation
- sorted(): Built-in function for sorting iterable objects.
- iterable: The sequence (list, tuple, etc.) to be sorted.
- key=lambda x: [sorting_criteria]: Custom sorting criteria using a lambda function:
- lambda x:: Defines the lambda function, taking each element x from the iterable.
- [sorting_criteria]: Specifies the basis for sorting using properties of x.
Example 1
pairs = [(1, 'one'), (3, 'three'), (2, 'two')]
sorted_pairs = sorted(pairs, key=lambda x: x[0])
print(sorted_pairs)
Explanation
pairs = [(1, 'one'), (3, 'three'), (2, 'two')]
:- Creates a list of tuples, each containing a number and a corresponding string.
sorted_pairs = sorted(pairs, key=lambda x: x[0])
:sorted(pairs)
: Sorts the pairs list using the sorted function.key=lambda x: x[0]
: Specifies a custom sorting key using a lambda function:lambda x:
: Defines an anonymous function.x[0]
: Accesses the first element (the number) of each tuple.- This means that the sorting is based on the first element of each tuple, which are the numbers 1, 3, and 2 in the pairs list.
- The sorted list is stored in
sorted_pairs
.
print(sorted_pairs)
: This line prints the sorted_pairs list.- Since the pairs list was sorted based on the first element of each tuple, the expected output will be the list of tuples sorted in ascending order of the numbers.
- So, the output will be [(1, ‘one’), (2, ‘two’), (3, ‘three’)].
Example 2
words = ['apple', 'banana', 'cherry']
sorted_words = sorted(words, key=lambda x: x[1])
print(sorted_words)
Explanation
words
list: Contains the words to be sorted.sorted(words, key=lambda x: x[1])
:sorted()
: Initiates sorting.words
: The list to be sorted.key=lambda x: x[1]
: The key parameter specifies a function to be called on each element before making comparisons for sorting:lambda x:
: Defines the function, taking each word x from words.x[1]
: Specifies sorting based on the second character of each word.
sorted_words
: Stores the sorted list.print(sorted_words)
: Print the sorted list based on the second letter of each word in the words list. The output will be the words ordered by their second letter.
The result of this code will be a list of words sorted in an order determined by the second letter of each word. For example, if the second letters are p, a, and h for “apple“, “banana“, and “cherry” respectively, the sorted order will be [“banana”, “cherry”, “apple”].
Using Python Lambda Function with List Comprehension
List comprehension is a method of creating lists in Python by combining loops and conditional statements. It allows for more compact and efficient code compared to traditional methods of creating lists.
Combining Python Lambda functions with list comprehension can greatly reduce the amount of code needed while still producing the desired result. For example, we can use a lambda function with a list comprehension to filter out even numbers from a list or square each element of a list. This combination allows us to write clean and compact code that is easy to read and understand.
Syntax
[lambda_function(item) for item in iterable]
Explanation
- [ ]: Encloses the list comprehension syntax.
- lambda_function(item): Lambda function applied to each item:
- lambda_function: Anonymous function defined inline.
- item: Represents each element from the iterable.
- for item in iterable: Iterates through the elements of the iterable.
Example
numbers = [1, 2, 3, 4, 5]
squared_numbers = [(lambda x: x**2)(x) for x in numbers]
print(squared_numbers)
Explanation
numbers
list: Contains the numbers to be squared.squared_numbers = [(lambda x: x**2)(x) for x in numbers]
:- List comprehension:
[ ... for x in numbers]
creates a new list. - Lambda function:
lambda x: x**2
squares each element x. - Immediate call:
(lambda x: x**2)(x)
applies the lambda function directly within the comprehension.
- List comprehension:
print(squared_numbers)
: Outputs the result: [1, 4, 9, 16, 25]
Using Python Lambda Function with if-else
Using the lambda function, we can also include an if-else statement within the function definition. This allows us to perform different operations based on a certain condition.
The if-else statement within the lambda function follows the same structure as a regular if-else statement in Python, with a conditional expression followed by a colon and an indented code block for each condition. However, it must be written on one line to fit within the lambda function syntax.
Syntax
lambda [parameters]: [expression1] if [condition] else [expression2]
Explanation
- lambda [parameters]:: Defines the lambda function with optional parameters.
- [expression1] if [condition] else [expression2]: Conditional expression:
- [expression1]: Evaluated and returned if the condition is true.
- [expression2]: Evaluated and returned if the condition is false.
Example
numbers = [1, 2, 3, 4, 5]
classify = lambda x: 'Even' if x % 2 == 0 else 'Odd'
classified_numbers = [classify(num) for num in numbers]
print(classified_numbers)
Explanation
numbers
list: Contains the numbers to be classified.classify = lambda x: 'Even' if x % 2 == 0 else 'Odd'
:- Lambda function: Defines a function to classify numbers based on parity.
- Conditional expression: Returns ‘Even’ if divisible by 2; otherwise, ‘Odd’.
classified_numbers = [classify(num) for num in numbers]
:- List comprehension: Creates a new list based on the classification.
- Applies classify function: Calls the lambda function for each number in numbers.
print(classified_numbers)
: Outputs the result: [‘Odd’, ‘Even’, ‘Odd’, ‘Even’, ‘Odd’]
Using Python Lambda Function with Multiple Statements
While lambda functions are typically limited to a single expression, we can simulate multiple operations by combining expressions. However, this is generally not recommended as it goes against the purpose of lambda functions being concise and simple.
Syntax
lambda [parameters]: [single_expression]
Explanation
- Parameters: Optional comma-separated list of function arguments.
- Single Expression: The core of the lambda function, evaluated and returned as the result.
Example
num = 4
operation = (lambda x: (x+2, x*3))(num)
print(operation)
Explanation
num = 4
: Assigns the value 4 to the variable num.operation = (lambda x: (x+2, x*3))(num)
:Lambda
function: Defined inline and immediately called with num as input.x+2, x*3
: Expression within the lambda:- Calculates x+2 (resulting in 6).
- Calculates x*3 (resulting in 12).
- Packs both results into a tuple (6, 12).
- Assigns tuple: The tuple is assigned to the operation variable.
print(operation)
: Outputs the tuple: (6, 12)
Using Python Lambda Function with filter()
One common use of lambda functions in Python is with the filter() method. This built-in function filters out the elements from an iterable based on a given condition and returns an iterator object.
The filter() method takes two arguments: lambda function and iterable. The lambda function is applied to each element in the iterable, and only those elements for which the condition evaluates to True are returned.
Syntax
filter(lambda [parameter]: [condition], iterable)
Explanation
- filter() function: Built-in function for filtering elements from an iterable.
- lambda [parameter]: [condition]: Lambda function defining the filtering criteria:
- [parameter]: Optional argument representing each element from the iterable.
- [condition]: Expression that evaluates to True or False for each element.
- iterable: The sequence (list, tuple, etc.) to be filtered.
Example 1: Filtering Even Numbers from a List
Suppose we have a list of integers and want to filter out only the even numbers.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) # Filter even numbers
print(even_numbers)
Explanation
numbers = [1, 2, 3, ..., 10]
: Creates a list of numbers.even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
:filter()
function: Applies a filtering process to the numbers list.lambda x: x % 2 == 0
: Lambda function defining the filtering criteria:x
: Represents each number from the list.x % 2 == 0
: Checks if the number is even (divisible by 2).
list()
: Converts the filter object to a list for easier use.even_numbers
: Stores the resulting list of even numbers.
print(even_numbers)
: Outputs the filtered list: [2, 4, 6, 8, 10]
Example 2: Filtering by Age
We can use a lambda function to filter a list of people by age, such as filtering out all people older than 18.
people = [{'name': 'Alice', 'age': 17}, {'name': 'Bob', 'age': 20}]
adults = list(filter(lambda person: person['age'] > 18, people))
print(adults)
Explanation
people = [{'name': 'Alice', 'age': 17}, {'name': 'Bob', 'age': 20}]
:- Creates a list of dictionaries, each representing a person with their name and age.
adults = list(filter(lambda person: person['age'] > 18, people))
:filter()
function: Applies a filtering process to the people list.lambda person: person['age'] > 18
: Lambda function defining the filtering criteria:person
: Represents each person’s dictionary from the list.person['age'] > 18
: Accesses the ‘age’ value and checks if it’s greater than 18.
list()
: Converts the filter object to a list for easier use.adults
: Stores the resulting list of adults.
print(adults)
: Outputs the filtered list: [{‘name’: ‘Bob’, ‘age’: 20}]
Using Python Lambda Function with map()
The map() function in Python is used to apply a given function to each element in an iterable, such as a list. It takes two arguments – the function and the iterable – and returns a new iterator with the results of applying the function on each element.
Using lambda functions with map() can be particularly useful when working with large datasets or performing repetitive tasks. It allows for compact and efficient code without the need to define a separate function for each task
Syntax
map(lambda [parameter]: [operation], iterable)
Explanation
- map() function: Built-in function for applying a function to each element of an iterable.
- lambda [parameter]: [operation]: Lambda function defining the operation to be applied:
- [parameter]: Optional argument representing each element from the iterable.
- [operation]: Expression or calculation to be performed on each element.
- iterable: The sequence (list, tuple, etc.) to be processed.
Example 1: Squaring Numbers in a List
numbers = [1, 2, 3, 4]
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(squared_numbers)
Explanation
numbers = [1, 2, 3, 4]
: Creates a list of numbers.squared_numbers = list(map(lambda x: x ** 2, numbers))
:map()
function: Applies a function to each element of the numbers list.lambda x: x ** 2
: Lambda function defining the squaring operation:x
: Represents each number from the list.x ** 2
: Calculates the square of each number.
list()
: Converts the map object to a list for easier use.squared_numbers
: Stores the resulting list of squared numbers.
print(squared_numbers)
: Outputs the squared list: [1, 4, 9, 16]
Example 2: Transforming to Upper Case
Transforming all elements in a list to upper case is another example where map() and lambda can be used effectively.
words = ['hello', 'world']
upper_words = list(map(lambda x: x.upper(), words))
print(upper_words)
Explanation
words = ['hello', 'world']
: Creates a list of words.upper_words = list(map(lambda x: x.upper(), words))
:map()
function: Applies a function to each element of the words list.lambda x: x.upper()
: Lambda function defining the capitalization operation:x
: Represents each word from the list.x.upper()
: Calls the upper() method to capitalize each word.
list()
: Converts the map object to a list for easier use.upper_words
: Stores the resulting list of capitalized words.
print(upper_words)
: Outputs the capitalized list: [‘HELLO’, ‘WORLD’]
Using Python Lambda Function with reduce()
The reduce() function in Python is used to apply a particular function passed in its argument to all of the list elements mentioned in the sequence passed along. reduce() function is a part of the functools module.
Combining Python lambda functions and reduce() can be very useful when we want to perform an operation on a list of elements, such as finding the maximum or minimum value, summing up all the values, etc. These operations can be done easily in a single line of code, making the code more efficient and readable.
Syntax
reduce(lambda accumulator, item: [operation], iterable, [initializer])
Explanation
- reduce() function: Built-in function for applying a function cumulatively to items in an iterable.
- lambda accumulator, item: [operation]: Lambda function defining the reduction operation:
- accumulator: Stores the accumulated result across iterations.
- item: Represents each element from the iterable.
- [operation]: Expression combining accumulator and item to produce the new accumulated value.
- iterable: The sequence (list, tuple, etc.) to be processed.
- [initializer]: Optional initial value for the accumulator (defaults to the first item).
Example 1: Calculating the Sum of a List
Suppose we want to calculate the sum of all numbers in a list.
numbers = [1, 2, 3, 4, 5]
from functools import reduce
sum_of_numbers = reduce(lambda acc, x: acc + x, numbers)
print(sum_of_numbers)
Explanation
numbers = [1, 2, 3, 4, 5]
: Creates a list of numbers.from functools import reduce
: Imports the reduce() function from the functools module, as it’s not a built-in function in Python 3.sum_of_numbers = reduce(lambda acc, x: acc + x, numbers)
:reduce()
function: Applies a function cumulatively to the elements of the numbers list.lambda acc, x: acc + x
: Lambda function defining the addition operation:acc
: Represents the accumulated sum across iterations.x
: Represents each number from the list.acc + x
: Adds the current number x to the accumulated sum acc, creating the new accumulated value.
numbers
: The list of numbers to be summed.
print(sum_of_numbers)
: Outputs the sum of all numbers in the list, which will be 15.
Example 2: Finding Maximum Element
Finding the maximum element in a list using reduce() and lambda is straightforward.
numbers = [1, 2, 3, 4]
max_number = reduce(lambda x, y: x if x > y else y, numbers)
print(max_number)
Explanation
numbers = [1, 2, 3, 4]
: Creates a list of numbers.max_number = reduce(lambda x, y: x if x > y else y, numbers)
:reduce()
function: Applies a function cumulatively to the elements of the numbers list.lambda x, y: x if x > y else y
: Lambda function defining the comparison logic:x
: Represents the current maximum value found so far.y
: Represents each element from the list.x if x > y else y
: Returns x if it’s greater than y, otherwise returns y, ensuring the maximum value is always retained.
numbers
: The list to be processed.max_number
: Stores the final maximum value.
print(max_number)
: Outputs the maximum value, which will be 4 in this case.
Difference between Python Lambda and Regular Functions
Python offers two ways to define functions: the standard def keyword for regular functions and the lambda keyword for lambda (or anonymous) functions. While both serve the purpose of creating functions, there are notable differences in their syntax, usage, and capabilities.
One of the fundamental differences between lambda functions and regular functions is that lambda functions can only contain expressions, not statements. Expressions are pieces of code that return a value, such as arithmetic operations, function calls, or logical operations. In contrast, statements like return, pass, assert, or raise are used to perform actions and don’t return a value. Regular functions can include expressions and statements, making them more versatile for complex logic. However, this versatility can sometimes be overkill for simple operations, where a lambda function would be more efficient.
Python Lambda Function Syntax
lambda arguments: expression
Explanation
- lambda: Keyword to start a lambda function.
- arguments: Parameters that the function takes.
- expression: A single expression that gets evaluated and returned.
Python Regular Function Syntax
Regular functions are defined using the def keyword:
def function_name(parameters):
# function body
return result
Explanation
- def: The keyword to start a function definition.
- function_name: The name of the function.
- parameters: The parameters that the function takes. Can be none, one, or many.
- Function Body: Contains one or more statements that define what the function does.
- return: Sends back a value from the function (optional).
Python Lambda Function Example
add = lambda a, b: a + b
result = add(5, 3)
Explanation
add = lambda a, b: a + b:
lambda a, b:
: Defines a lambda function with two parameters, a and b.a + b
: Expression calculating the sum of a and b.=
: Assigns the lambda function to the variable add.
result = add(5, 3):
add(5, 3)
: Calls the add function, passing arguments 5 and 3.=
: Assigns the returned value (8) to the variable result.
Python Regular Function Example
def add(a, b):
return a + b
result = add(5, 3)
Explanation
def keyword
: Identifies a function definition.add
: The function’s name.(a, b)
: Parameters the function accepts.return a + b
: Returns the sum of a and b.
Key Points
- Regular functions (defined with def):
- Have a name and can be called multiple times.
- Can contain multiple statements and have a more complex structure.
- Use the return statement to return a value explicitly.
- Lambda functions (defined with lambda keyword):
- Anonymous functions, often used inline for simple operations.
- Limited to a single expression and can’t contain multiple statements.
- Implicitly return the value of the expression.
Key Differences
- Syntax: Regular functions use def, lambdas use lambda.
- Name: Regular functions have a name, lambdas are anonymous.
- Body: Regular functions can have multiple statements; lambdas are limited to a single expression.
- Return: Regular functions use return, lambdas return the expression’s value implicitly.
Conclusion
Python Lambda functions, first introduced in Lisp in the 1950s, have become widely adopted in programming languages like Python, Java, and C++. With minimal code, Lambda functions provide a concise and powerful way to write functions. They are especially useful when a small, one-time function is needed without defining a separate function. Incorporating Lambda functions into your Python programming can enhance code readability and efficiency while maintaining elegant solutions.
Python Reference
FAQ
What is a Lambda Function in Python?
Python Lambda function is a small, anonymous (nameless) function that can be defined without a name. It provides a concise way to create functions on the fly without needing a formal definition. Lambda functions are commonly used when we only need to define a simple, one-line function that won’t be reused elsewhere in our code. They are particularly useful for tasks like filtering or mapping elements in lists and performing quick calculations.
How do you use a Lambda Function with map() and filter()?
The map() function allows you to apply the Python Lambda Function to each element in an iterable, returning a new iterable with the results. This is particularly useful when you want to perform the same operation on multiple elements at once. On the other hand, the filter() function enables you to selectively include or exclude elements from an iterable based on a given condition specified by the Python Lambda Function.
Can Lambda Functions have multiple arguments in Python?
Yes, the Lambda function in Python can have multiple arguments. Lambda functions are anonymous functions that can take any number of arguments but only have a single expression. These arguments are separated by commas and defined within the lambda function itself.
What are the limitations of using Lambda Functions in Python?
Firstly, Python Lambda Functions are restricted to a single expression, making it unsuitable for complex logic or multiple statements. Secondly, they cannot include documentation or annotations, hindering code readability and maintainability. Thirdly, debugging lambda functions can be challenging as they provide limited traceback information.
Additionally, lambda functions cannot contain statements such as print or assert, further limiting their functionality.
Are Lambda Functions in Python more efficient than regular functions?
While Python regular functions have advantages, lambda functions excel in certain scenarios. They are particularly useful when writing short, one-time-use functions or when passing a function as an argument to another function. However, it’s important to note that lambda functions can be less readable and unsuitable for complex logic or large-scale projects.