Table of Contents | |
What Is a List in Python?
A list in Python is a mutable, ordered sequence of elements. It is one of the four built-in data types used to store collections of data, the other three being Tuple, Set, and Dictionary. Lists are mutable, meaning their elements can be changed or removed after being created. Lists can hold any type of data, including integers, floats, strings, and even complex types like objects or other lists.
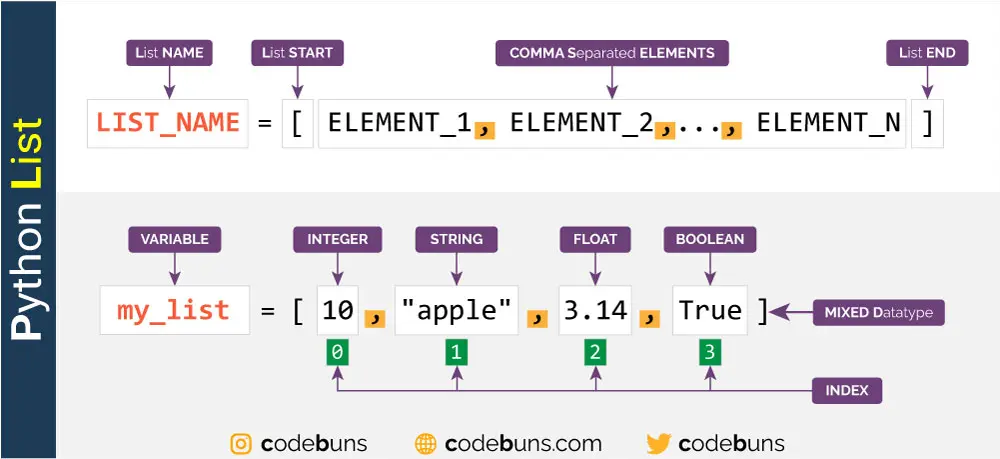
Example of Python List
my_list = []
Empty square brackets ([ ]) create an empty list.
my_list = [10, "apple", 3.14, True]
Explanation
- Initialization: The variable
my_list
is assigned a list value. - Mixed Data Types: Lists can store multiple data types. In this case,
my_list
contains an integer (1), a string (“apple“), a float (3.14), and a boolean value (True).
Python List Syntax
The syntax for a list in Python is straightforward. Lists are defined by enclosing a comma-separated sequence of elements within square brackets [ ].
LIST_NAME = [ELEMENT_1, ELEMENT_2, …, ELEMENT_N]
Explanation
- List Declaration: In the list syntax, LIST_NAME represents the variable name that holds the list.
- Square Brackets: Lists are enclosed in square brackets [ ].
- Elements: Inside the square brackets, individual elements (ELEMENT_1, ELEMENT_2, etc.) are separated by commas.
- Access Pattern: To retrieve an element from the list, we’d use an index, like LIST_NAME[INDEX]. Remember, Python indexing starts at 0.
Characteristics of a Python List
When diving into Python programming, understanding the core characteristics of a Python list is pivotal to harnessing its full potential. Here are some of the core characteristics of Python lists:
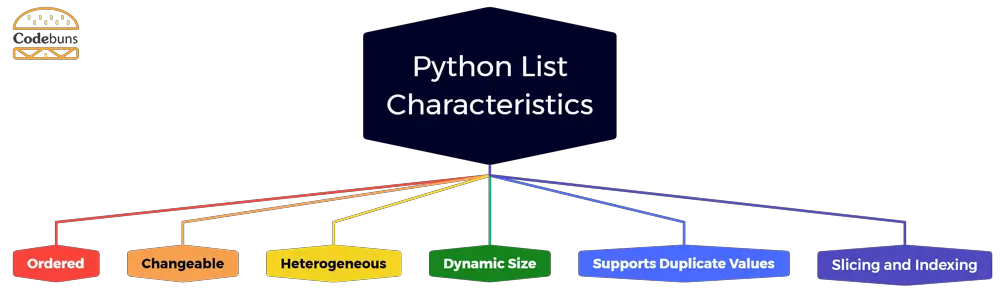
Ordered
- Explanation: Python list maintains the order of elements. When elements are inserted into a list, they can be accessed in the same order they were added.
- Example:
[3, 1, 4, 2]
- The first element is 3, followed by 1, 4, and 2.
- When we access the list, the order remains consistent unless changed.
Changeable
- Explanation: Python List can be altered. Elements can be modified, added, or removed after the list has been created.
- Example:
fruits = ['apple', 'banana', 'cherry']
fruits[1] = 'blueberry'
- Starts with
['apple', 'banana', 'cherry']
- Changes the second item to ‘blueberry‘, resulting in
['apple', 'blueberry', 'cherry']
.
- Starts with
Heterogeneous
- Explanation: A single list can contain elements of different data types, including strings, integers, and objects.
- Example:
mixed_list = [1, 'hello', 3.14, True]
- mixed_list contains an integer, string, float, and boolean as elements.
- This feature facilitates the storage of varied data types in a single collection.
Dynamic Size
- Explanation: Python list can dynamically adjust size, meaning elements can be added or removed, and the list will resize accordingly.
- Example:
colors = ['red'];
colors.append('blue')
- Initially, the colors list contains one element: ‘red‘.
- With the use of
.append('blue')
, a second element, ‘blue‘, is added without redefining the list.
Supports Duplicate Values
- Explanation: A Python list can have multiple elements with the same value.
- Example:
[1, 2, 2, 3, 3, 3]
- The number 2 appears twice, and the number 3 appears thrice.
Slicing and Indexing
- Explanation: Python list supports slicing, which lets us get a subset of the list. Each item in a list has an index, starting from 0.
- Example:
animals = ['cat', 'dog', 'bird'];
slice = animals[1:3]
- The animals list consists of three strings.
- Through slicing
animals[1:3]
, a new list slice is created with elements ‘dog‘ and ‘bird‘ from indices 1 to 2.
Creating a List in Python
There are a few different ways to create a Python list. Here are two of the most common methods:
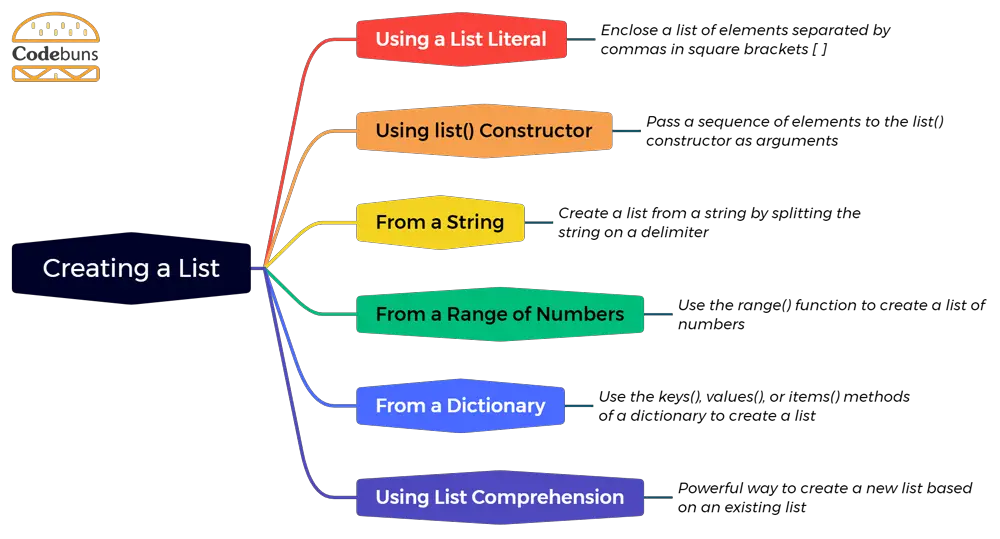
Using a List Literal
This is the most common way to create a list. To do this, we enclose a list of elements separated by commas in square brackets ([ ]). The elements can be any object.
Example
animals = ["cat", "dog", "bird"]
Explanation
animals
is the name of the list.[ ]
: These square brackets indicate that we’re defining a list.["cat", "dog", "bird"]
is the list literal.- The list contains three string elements separated by a comma: “cat“, “dog“, and “bird“.
Using list()
Constructor
The list() constructor can also be used to create a list. To do this, we pass a sequence of elements to the list() constructor as arguments. The elements can be any type of object.
Syntax
list_name = list(iterable)
Explanation
- list_name is the name of the list.
- iterable is an iterable object, such as a tuple, string, or another list.
Example
animals = list(("cat", "dog", "bird")) # converts tuple to list
This creates a list with the same three elements as the previous example.
In addition to the two main ways to create Python list, there are many other ways to create lists, such as:
From a String
We can create a list from a string by splitting the string on a delimiter.
Example
my_list = list("This is a string")
The above example creates a list from the string “This is a string“.
From a Range of Numbers
We can use the range() function to create a list of numbers.
Example
numbers = list(range(10))
This creates a list with the numbers 0 through 9.
From a Dictionary
We can use the keys(), values(), or items() methods of a dictionary to create a list from the keys, values, or key-value pairs of the dictionary.
Example
my_dict = {"name": "Mark", "age": 2}
# Create a list from the keys of the dictionary
keys = list(my_dict.keys())
# Create a list from the values of the dictionary
values = list(my_dict.values())
# Create a list from the key-value pairs of the dictionary
items = list(my_dict.items())
This creates three lists:
Explanation
- keys contains the strings “name” and “age“.
- values contains the strings “Mark” and 2.
- items include a list of tuples, each containing a key-value pair from the dictionary.
Using List Comprehension
List comprehension is a powerful way to create a new list in Python. It allows us to create a new list based on an existing list, filtering and transforming the elements as needed.
Syntax
new_list = [expression for element in iterable]
Explanation
- new_list is the name of the new list.
- expression is the expression that will be evaluated for each element in the iterable.
- element is a temporary variable used to iterate over the iterable.
- iterable is an iterable object, such as a list, tuple, or string.
List comprehensions can also incorporate if conditions and multiple loops, but the example provided is a simple and straightforward usage of the concept.
Example
my_list = [x for x in range(10)]
This creates a list with the numbers from 0 to 9, inclusive.
Explanation
my_list
is the name of the new list.x for x in range(10)
is the list comprehension.range(10)
is an iterable object that generates the numbers 0 to 9.
Adding Elements in a Python List
There are four different ways to add elements in a Python list:
- Using += Operator
- Using append() Method
- Using extend() Method
- Using insert() Method
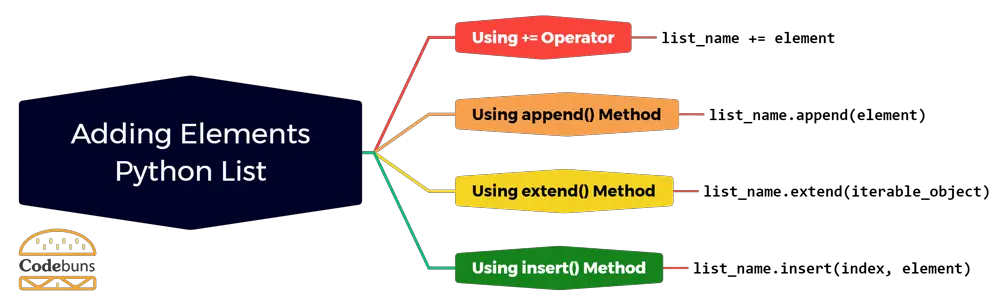
Using +=
Operator
To add elements to a Python list using the assignment operator, we use the += operator. The += operator adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand.
Syntax
list_name += element
Explanation
- list_name is the name of the list.
- element is the element to be added to the list.
Example
my_list = ["apple", "banana"]
# Add the element "cherry" to the end of the list
my_list += ["cherry"]
# Print the list
print(my_list)
#Output: ['apple', 'banana', 'cherry']
Explanation
- The += operator adds the element
["cherry"]
to the list my_list. - The result is assigned to the list my_list.
- The list my_list now contains the elements “apple“, “banana“, and “cherry“.
We can also use the += operator to add a list of elements to a list. For example, the following code adds the list ["orange", "mango"]
to the list my_list:
my_list += ["orange", "mango"]
#Output: ['apple', 'banana', 'cherry', 'orange', 'mango']
The assignment operator can also be used to add elements to a list in a specific position. For example, the following code adds the element “orange” to the list my_list at index 1:
my_list[1] = "kiwi"
#Output: ['apple', 'kiwi', 'cherry', 'orange', 'mango']
Note: The assignment operator cannot be used to add elements to a tuple, as tuples are immutable.
Using append()
Method
To add elements to a Python list using the append() method, we call the append() method on the list and pass the element(s) to be added as arguments. The append() method adds the element(s) to the end of the list.
Syntax
list_name.append(element)
Explanation
- list_name is the name of the list.
- element is the element(s) to be added to the list.
Example
my_list = ["apple", "banana"]
# Add the element "cherry" to the list
my_list.append("cherry")
# Print the list
print(my_list)
#Output: ['apple', 'banana', 'cherry']
Explanation
- The
append()
method adds the element “cherry” to the end of the list my_list. - The list my_list now contains the elements “apple“, “banana“, and “cherry“.
We can also use the append() method to add a list of elements to a list. For example, the following code adds the list ["orange", "mango"]
to the list my_list:
my_list = ["apple", "banana"]
# Add the elements "orange" and "mango" to the list
my_list.append(["orange", "mango"])
# Print the list
print(my_list)
#Output: ['apple', 'banana', ['orange', 'mango']]
Using extend()
Method
To add elements to a Python list using the extend() method, we pass an iterable object to the extend() method as an argument. The extend() method adds each element of the iterable object to the end of the list.
Syntax
list_name.extend(iterable_object)
Explanation
- list_name is the name of the list.
- iterable_object is an iterable object, such as a list, tuple, or string.
Example
my_list = ["apple", "banana"]
# Add the elements "cherry" and "orange" to the list
my_list.extend(["cherry", "orange"])
# Print the list
print(my_list)
#Output: ['apple', 'banana', 'cherry', 'orange']
Explanation
- The
extend()
method adds each element of the iterable object["cherry", "orange"]
to the end of the list my_list. - The list my_list now contains the elements “apple“, “banana“, “cherry“, and “orange“.
The extend() method can also be used to add elements to a list in reverse order. For example, the following code adds the elements of the iterable object ["cherry", "orange"]
to the list my_list in reverse order:
my_list = ["apple", "banana"]
# Add the elements "cherry" and "orange" to the list in reverse order
my_list.extend(["cherry", "orange"][::-1])
# Print the list
print(my_list)
#Output: ['apple', 'banana', 'orange', 'cherry']
Using insert()
Method
To add elements to a Python list using the insert() method, we pass the index at which to insert the element and the element to be inserted as arguments to the insert() method. The insert() method inserts the element at the specified index and shifts all of the elements to the right of the specified index down one position.
Syntax
list_name.insert(index, element)
Explanation
- list_name is the name of the list.
- index is the index at which to insert the element.
- element is the element to be inserted.
Example
my_list = ["apple", "banana"]
# Insert the element "cherry" at the beginning of the list
my_list.insert(0, "cherry")
# Print the list
print(my_list)
#Output: ['cherry', 'apple', 'banana']
Explanation
- The
insert()
method inserts the element “cherry” at index 0 of the list my_list. - All of the elements in the list to the right of index 0 are shifted down one position.
- The list my_list now contains the elements “cherry“, “apple“, and “banana“.
The insert() method can also be used to insert a list of elements into a list. For example, the following code inserts a list ["cherry", "orange"]
into a list my_list:
my_list = ["apple", "banana"]
# Insert the elements "cherry" and "orange" at index 1 of the list
my_list.insert(1, ["cherry", "orange"])
# Print the list
print(my_list)
#Output: ['apple', ['cherry', 'orange'], 'banana']
Updating Elements in a Python List
To update an element in a Python list, we use the assignment operator (=) and the index of the element to be updated.
Syntax
list_name[index] = new_element
Explanation
- list_name is the name of the list.
- index is the index of the element to be updated.
- new_element is the new value to be assigned to the element at the specified index.
Example
my_list = ["apple", "banana", "cherry"]
# Update the element at index 1 to "orange"
my_list[1] = "orange"
# Print the list
print(my_list)
#Output: ['apple', 'orange', 'cherry']
Explanation
- The assignment operator (=) assigns the new value “orange” to the element at index 1 in the list my_list.
- The list my_list now contains the elements “apple“, “orange“, and “cherry“.
We can also use the assignment operator (=) to update a list of elements in a list.
my_list = ["apple", "banana", "cherry"]
# Update the elements at indices 1 and 2 to "orange" and "peach"
my_list[1:3] = ["orange", "peach"]
# Print the list
print(my_list)
#Output: ['apple', 'orange', 'peach']
Explanation
- The assignment operator (=) assigns the new values “orange” and “peach” to the elements at indices 1 and 2 in the list my_list.
- The list my_list now contains the elements “apple“, “orange“, and “peach“.
Accessing Elements in Python List
There are a few different ways to access elements in a Python list. The most common way is to use the index operator.
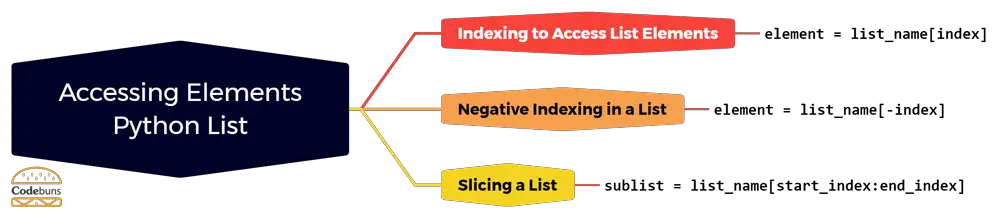
Indexing to Access List Elements
The index operator ([ ]) is the most common way to access elements in a Python list. To access an element in a list using the index operator, we specify the index of the element in square brackets. The index of an element is its position in the list, starting at 0.
Syntax
element = list_name[index]
Explanation
- element is the variable that will store the element at the specified index.
- list_name is the name of the list.
- index is the index of the element to be accessed.
Example
my_list = ["apple", "banana", "cherry"]
# Access the first element in the list
first_element = my_list[0]
# Print the first element
print(first_element)
# Output: apple
Explanation
my_list
is a list of three elements: “apple“, “banana“, and “cherry“.my_list[0]
is the first element in the list.- The
first_element
variable is assigned the value of the first element in the list. - The
print()
function is used to print the value of the first_element variable to the console.
Negative Indexing in a List
We can also use negative indices to access elements in a list. Negative indices start at -1 and refer to the elements from the end of the list.
Syntax
element = list_name[-index]
Explanation
- element is the variable that will store the element at the specified index.
- list_name is the name of the list.
- index is the negative index of the element to be accessed.
Example
my_list = ["apple", "banana", "cherry"]
# Access the last element in the list
last_element = my_list[-1]
# Print the last element
print(last_element)
# Output: cherry
Explanation
last_element = my_list[-1]
accesses the last element in the list, which is “cherry“. This is because the index of the last element in a list is always -1.print(last_element)
prints the last element in the list to the console.
Slicing a List
Slicing a list in Python is a way to extract a sublist from the original list. This can be done using the square bracket ([ ]) operator with a colon (:). The slice expression is a colon-separated pair of indices, which specifies the start and end indices of the sublist.
Syntax
sublist = list_name[start_index:end_index]
Explanation
- sublist is the variable that will store the sublist.
- list_name is the name of the list.
- start_index is the start index of the slice.
- end_index is the end index of the slice.
- [start_index:end_index] slice expression.
Example
my_list = ["apple", "banana", "cherry"]
# Get a sublist of the first two elements in the list
sublist = my_list[0:2]
# Print the sublist
print(sublist)
#Output: ['apple', 'banana']
Explanation
sublist = my_list[0:2]
gets a sublist of the first two elements in the list my_list. The slice operator (:) allows us to access a subset of elements in a list. The start index is 0 (inclusive) and the end index is 2 (exclusive), so the sublist will contain the elements at indices 0 and 1.print(sublist)
prints the sublist to the console.
Finding an Element in a Python List
There are several ways to find an element in a Python list. Here are the most common methods:
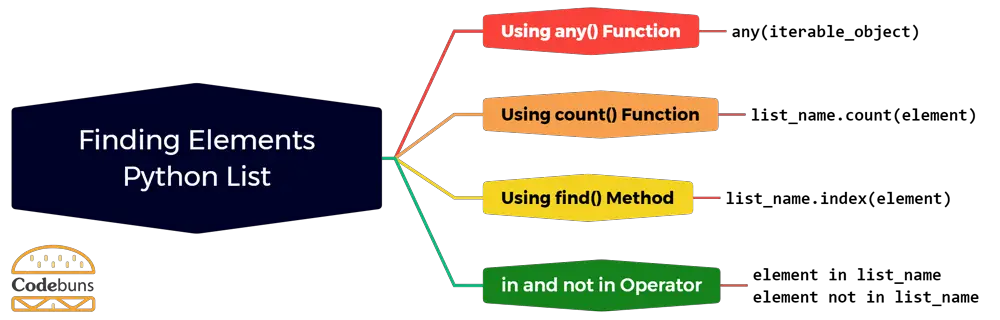
Using any()
Function
The any() function takes an iterable object as an argument and returns True if any element of the iterable is True, False otherwise. This can be used to find an element in a Python list by checking if any of the elements in the list match the specified element.
Syntax
any(iterable_object)
Explanation
- iterable_object is an iterable object, such as a list, tuple, or string.
Example
my_list = ["apple", "banana", "cherry"]
# Check if the element "cherry" is in the list
is_in_list = any(element == "cherry" for element in my_list)
# Print the result
print(is_in_list)
#Output: True
Explanation
- The
any()
function iterates over the list my_list and checks if any of the elements in the list match the element “cherry“. - The
element == "cherry"
expression returns True if the element matches “cherry“, and False otherwise. - The
any()
function returns True if any of the elements in the list match “cherry“, and False otherwise. - In this example, the element “cherry” is in the list my_list, so the
any()
function returns True.
The any() function can also be used to find multiple elements in a list. To do this, we can use a generator expression to create an iterable object that contains the elements to search for.
my_list = ["apple", "banana", "cherry"]
# Check if the elements "cherry" and "orange" are in the list
are_in_list = any(element in ["cherry", "orange"] for element in my_list)
# Print the result
print(are_in_list)
#Output: True
Explanation
- The generator expression element in
["cherry", "orange"]
creates an iterable object that contains the elements “cherry” and “orange“. - The
any()
function iterates over the iterable object and checks if any of the elements in the list my_list match the elements in the iterable object. - The
any()
function returns True if any of the elements in the list my_list match the elements in the iterable object, and False otherwise. - In this example, the elements “cherry” and “orange” are in the list my_list, so the
any()
function returns True.
Using count()
Function
The count() function returns the number of times the specified element appears in the list.
Syntax
list_name.count(element)
Explanation
- list_name is the name of the list.
- element is the element to be counted in the list.
Example
my_list = ["apple", "banana", "cherry", "banana"]
count = my_list.count("banana")
print(count)
#Output: 2
Explanation
- The my_list variable is assigned a list containing the elements “apple“, “banana“, “cherry“, and “banana“.
- The
count()
function is called on the list my_list and passed the element “banana” as an argument. - The
count()
function returns the value 2, which is the number of times the element “banana” appears in the list my_list. - The
print()
function is used to print the value 2 to the console.
Note: The count() function is short-circuiting, meaning that it stops iterating over the list as soon as it finds the specified element. This can make the count() function more efficient than other methods of finding an element in a list, such as using a for loop.
Using find()
Method
The index() method returns the index of the first occurrence of the specified element in the list. If the element is not in the list, the index() method raises a ValueError exception.
Syntax
list_name.index(element)
Explanation
- list_name is the name of the list.
- element is the element to be found in the list.
Example
my_list = ["apple", "banana", "cherry"]
index = my_list.index("banana")
if index >= 0:
print("The element is in the list at index {}.".format(index))
else:
print("The element is not in the list.")
#Output: The element is in the list at index 1.
The index() method returns the index of the first occurrence of the element “banana” in the list my_list. The index of the element “banana” is 1, so the value 1 is printed to the console.
Note: The index() method is short-circuiting, meaning that it stops iterating over the list as soon as it finds the element being searched for.
Checking if an Element Exists in a Python List (in
and not in
Operator)
The in and not in operators can be used to check if an element exists in a list. The in operator is a binary operator that returns True if the left operand (the element) is found in the right operand (the list), and False otherwise.
Syntax
element in list_name
element not in list_name
Explanation
- element is the element that is being checked for in the list.
- list_name is the name of the list.
Example
my_list = ["apple", "banana", "cherry"]
# Check if the element "apple" exists in the list
if "apple" in my_list:
print("Yes, the element 'apple' exists in the list.")
else:
print("No, the element 'apple' does not exist in the list.")
#Output: Yes, the element 'apple' exists in the list.
The in operator returns True because the element “apple” is present in the list my_list. Therefore, the if statement is evaluated to True and the message “Yes, the element ‘apple’ exists in the list.” is printed to the console.
Deleting Elements from a Python List
There are several ways to delete elements from a Python list. Here are the most common methods:
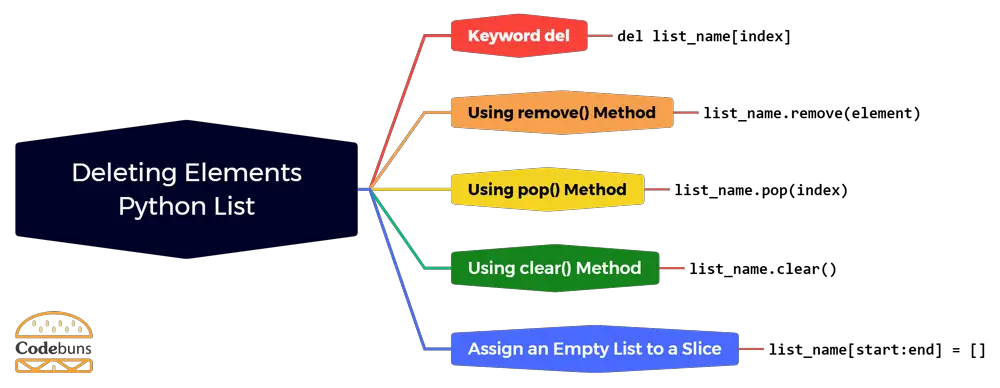
Keyword del
To delete an element from a Python list using the del keyword, we specify the index of the element to be deleted. The del keyword deletes the element at the specified index from the list, shifting the existing elements to the left.
Syntax
del list_name[index]
Deletes the element at the specified index from the list.
Explanation
- list_name is the name of the list.
- index is the index of the element to be deleted.
Example
my_list = ["apple", "banana", "cherry"]
# Delete the element at index 0
del my_list[0]
# Print the list
print(my_list)
#Output: ['banana', 'cherry']
The del
keyword deletes the element at index 0 from the list my_list
. The element at index 0 is the element “apple“, so the element “apple” is deleted from the list. After the deletion, the list my_list
contains the elements “banana” and “cherry“.
The del
keyword can also be used to delete a slice of the list. To do this, we specify the start and end indices of the slice, separated by a colon. The del
keyword deletes all elements from the start index to the end index, excluding the end index.
Syntax
del list_name[start:end]
Explanation
- list_name is the name of the list.
- start is the start index of the slice to be deleted.
- end is the end index of the slice to be deleted.
For example, the following code deletes the elements at indices 0 and 1 from the list my_list:
my_list = ["apple", "banana", "cherry"]
# Delete the elements at indices 0 and 1 from the list
del my_list[0:2]
# Print the list
print(my_list)
#Output: ['cherry']
Using remove()
Method
To delete an element from a Python list using the remove() method, we simply specify the element to be deleted as the argument to the remove() method. The remove() method will then remove the first occurrence of the specified element from the list, shifting the existing elements to the left.
The remove() method raises a ValueError exception if the element to be removed is not in the list.
Syntax
list_name.remove(element)
Explanation
- list_name is the name of the list.
- element is the element to be deleted from the list.
Example
my_list = ["apple", "banana", "cherry"]
# Delete the element "banana" from the list
my_list.remove("banana")
# Print the list
print(my_list)
#Output: ['apple', 'cherry']
The remove()
method removes the first occurrence of the element “banana” from the list my_list
. The list my_list
now contains the elements “apple” and “cherry“.
Note: The remove() method is short-circuiting, meaning that it stops iterating over the list as soon as it finds the element being searched for.
Using pop()
Method
To delete an element from a Python list using the pop() method, we pass the index of the element to be removed as an argument to the pop() method. The pop() method then removes and returns the element at the specified index, shifting the remaining elements to the left.
If the index is not specified, the pop() method removes and returns the last element from the list.
Syntax
list_name.pop(index)
Explanation
- list_name is the name of the list.
- index is the index of the element to be removed from the list. If the index is not specified, the last element in the list is removed and returned.
Example
my_list = ["apple", "banana", "cherry"]
# Remove the first element from the list
element = my_list.pop(0)
# Print the element that was removed
print(element)
#Output: apple
The pop()
method removes and returns the first element from the list my_list
. The element that was removed is stored in the variable element. The list my_list
now contains the elements “banana” and “cherry“.
Note: The pop() method raises an IndexError exception if the index is out of range.
Using clear()
Method
The clear() method in Python is used to remove all elements from a list. This leaves the list empty, but the list object itself still exists.
To delete all elements from a Python list using the clear() method, we simply call the clear() method on the list object.
Syntax
list_name.clear()
Example
my_list = ["apple", "banana", "cherry"]
# Remove all elements from the list
my_list.clear()
# Print the list
print(my_list)
#Output: []
The clear()
method removes all elements from the list my_list
. The list my_list
is still present, but it is empty. The print()
function is then called to print the list my_list
to the console.
Note: The clear() method does not return anything.
Assign an Empty List to a Slice
Assigning an empty list to a slice is a powerful technique in Python that can be used to achieve a variety of tasks, such as deleting multiple elements from a list at once, clearing a slice of a list, or inserting a new slice into a list.
Syntax
list_name[start:end] = []
Explanation
- list_name is the name of the list.
- start is the index of the first element to be deleted.
- end is the index of the first element to be retained.
For example, the following code deletes the first two elements from the list my_list:
Example
my_list = ["apple", "banana", "cherry"]
# Delete the first two elements from the list
my_list[0:2] = []
# Print the list
print(my_list)
#Output: ['cherry']
The following code deletes all elements from the list my_list:
my_list = ["apple", "banana", "cherry"]
# Delete all elements from the list
my_list[:] = []
# Print the list
print(my_list)
#Output: []
Note:The start and end indices can be negative to refer to elements from the end of the list. If the end index is not specified, the remaining elements in the list are deleted.
Iterating Over a Python List
Iterating over a Python list means going through each element of the list individually. This can be done using a for loop. The for loop iterates over the list and assigns each element to a variable. The code inside the for loop is then executed for each element in the list.
Syntax
for element in list_name:
# Code to be executed for each element in the list
Explanation
- list_name is the name of the list.
- element is the variable that will hold the current element in the list.
The for loop iterates over the list, assigning each element to the variable element. The code block within the for loop is executed for each element in the list.
Example
my_list = ["apple", "banana", "cherry"]
# Iterate over the list and print each element
for element in my_list:
print(element)
#Output:
# apple
# banana
# cherry
Explanation
- The my_list variable is assigned a list containing the elements “apple“, “banana“, and “cherry“.
- The for loop iterates over the list my_list and assigns each element to the variable fruit.
- The code inside the for loop prints the current element to the console.
Operations on Python Lists
Python lists are a powerful and versatile data structure that can be used to solve a wide variety of problems. Some of the most common operations that can be performed on Python lists include:
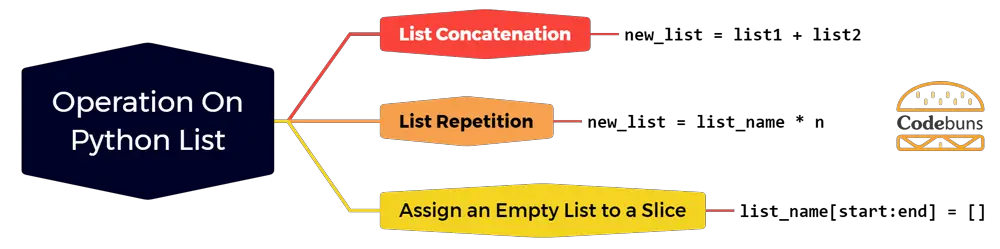
List Concatenation
To concatenate two lists using the + operator, simply add the two lists together. For example, the following code concatenates the lists list1 and list2 to form a new list called new_list:
Syntax
new_list = list1 + list2
Example
list1 = ["apple", "banana", "cherry"]
list2 = ["orange", "kiwi", "strawberry"]
new_list = list1 + list2
print(new_list)
#Output: ['apple', 'banana', 'cherry', 'orange', 'kiwi', 'strawberry']
The + operator is used to concatenate the lists list1 and list2. The result is a new list, new_list, which contains the elements of both list1 and list2.
List Repetition
The * operator can be used to create a new list that contains the elements of an existing list repeated a specified number of times. The syntax for repeating Python lists is as follows:
Syntax
new_list = list_name * n
Explanation
- new_list is the new list that will contain the elements of list_name repeated n times.
- list_name is the list to be repeated.
- n is the number of times to repeat the list.
For example, the following code creates a new list called new_list that contains the elements of the list my_list repeated 3 times:
Example
my_list = ["apple", "banana"]
# Repeat the list 3 times and assign the result to the list new_list
new_list = my_list * 3
# Print the new list
print(new_list)
#Output: ['apple', 'banana', 'apple', 'banana', 'apple', 'banana']
Explanation
- The my_list variable is assigned a list containing the elements “apple” and “banana“.
- The * operator is used to repeat the list my_list 3 times. The result of this operation is assigned to the list new_list.
- The
print()
function is used to print the list new_list to the console.
List Membership (using in
operator)
List membership in Python is the ability to check if a value is present in a list. This can be done using the in operator. The in operator returns a True value if the value is present in the list, and a False value if the value is not present in the list. The syntax for the in operator is as follows:
Syntax
element in list_name
Returns a boolean value, True if the element is present in the list, and False if it is not.
Explanation
- element is the value to check for membership in the list.
- list_name is the list to check.
For example, the following code checks if the value “apple” is present in the list my_list:
Example
my_list = ["apple", "banana", "cherry"]
# Check if the value "apple" is present in the list
is_member = "apple" in my_list
# Print the result
print(is_member)
#Output: True
Use Built-in Functions with Python List
Python lists come with a number of built-in functions that can be used to perform common operations on lists. Some of the most useful built-in functions for lists include:
Using len()
Function
The len() function in Python is used to get the length of an object. For lists, this means the number of elements in the list. The syntax for the len() function is as follows:
Syntax
length = len(list_name)
Explanation
- length is the variable that will store the length of the list.
- list_name is the list whose length is to be determined.
For example, the following code gets the length of the list my_list and assigns it to the variable length:
Example
my_list = ["apple", "banana", "cherry"]
# Get the length of the list
length = len(my_list)
# Print the length of the list
print(length)
#Output: 3
Explanation
- The my_list variable is assigned a list containing the elements “apple“, “banana“, and “cherry“.
- The
len()
function is used to get the length of the list my_list. The result is assigned to the variable length. - The
print()
function is used to print the length of the list my_list to the console.
Using max()
Function
The max() function in Python is used to get the largest element in a list. If the list contains strings, the max() function will return the element with the highest alphabetical order. The syntax for the max() function is as follows:
Syntax
max(list_name)
The max() function returns the largest element in the list list_name. If the list is empty, the max() function raises a ValueError exception.
For example, the following code gets the largest element in the list my_list and assigns it to the variable largest_element:
Example
my_list = [1, 2, 3, 4, 5]
# Get the largest element in the list and assign it to the variable largest_element
largest_element = max(my_list)
# Print the largest element in the list
print(largest_element)
#Output: 5
The max() function can also be used to compare elements of different types. For example, the following code gets the largest element in the list my_list, which contains both strings and numbers:
my_list = ["apple", "banana", "cherry", 1, 2, 3]
# Get the largest element in the list and assign it to the variable largest_element
largest_element = max(my_list)
# Print the largest element in the list
print(largest_element)
#Output: 3
Using min()
Function
The min() function in Python is used to find the smallest element in a list. The syntax for the min() function is as follows:
Syntax
min(list_name)
The min() function returns the smallest element in the list list_name. If the list is empty, the min() function raises a ValueError exception.
For example, the following code finds the smallest element in the list my_list:
Example
my_list = [1, 2, 3, 4, 5]
# Find the smallest element in the list and assign it to the variable smallest_element
smallest_element = min(my_list)
# Print the smallest element
print(smallest_element)
#Output: 1
Python List Methods
Python list methods are functions that can be used to perform common operations on lists. These methods can be used to make your code more efficient and readable.
Using copy()
Method
The copy() method in Python is used to create a shallow copy of a list. This means that the new list will contain references to the same elements as the original list, but the new list will be a separate object.
The syntax for the copy() method is as follows:
Syntax
new_list = list_name.copy()
Explanation
- list_name is the name of the list to be copied.
- new_list is the name of the new list that will contain the shallow copy of the original list.
For example, the following code creates a shallow copy of the list my_list and assigns it to the list new_list:
Example
my_list = [1, 2, 3, 4, 5]
# Create a shallow copy of the list and assign it to the list new_list
new_list = my_list.copy()
# Print the new list
print(new_list)
#Output: [1, 2, 3, 4, 5]
Comparing Python Lists
Comparing Python lists can be done in a number of ways, depending on what we want to compare.
If we want to compare the elements of two lists in the same order, we can use the == operator. The == operator returns True if the lists have the same elements in the same order, and False otherwise.
For example, the following code compares the two lists my_list1 and my_list2:
Example
my_list1 = [1, 2, 3, 4, 5]
my_list2 = [1, 2, 3, 4, 5]
# Compare the two lists using the == operator
if my_list1 == my_list2:
print("The two lists are equal.")
else:
print("The two lists are not equal.")
#Output: The two lists are equal.
To check if one list is a subset of another list, we can use the <= or => operator. This will return True if the first list contains all of the elements of the second list, and False otherwise.
For example, the following code will print True to the console:
Example
my_list1 = [1, 2, 3, 4, 5]
my_list2 = [1, 2, 3]
if my_list2 <= my_list1:
print(True)
else:
print(False)
#Output: True
Python List Comprehension
List comprehension is a concise and powerful way to create lists in Python. It allows us to create a new list from an existing list, applying a filter and/or transformation to each element of the existing list.
The syntax for list comprehension is as follows:
Syntax
new_list = [expression for element in iterable if condition]
Explanation
- new_list is the new list that will be created.
- expression is the expression to be evaluated for each element in the iterable.
- element is the element in the iterable.
- iterable is the iterable to be iterated over.
- condition is an optional condition that must evaluate to True for the element to be included in the new list.
For example, the following list comprehension creates a new list containing the squares of all the numbers from 1 to 10:
Example
squares = [x * x for x in range(1, 11)]
# Print the new list
print(squares)
#Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
List comprehensions can also be used to filter lists. For example, the following list comprehension creates a new list containing only the even numbers from 1 to 10:
even_numbers = [x for x in range(1, 11) if x % 2 == 0]
# Print the new list
print(even_numbers)
#Output: [2, 4, 6, 8, 10]
Reversing a Python List
Lists in Python can be reversed using different methods. Here are the most common ones:
Using reversed()
Method
The reversed() method in Python is used to reverse a list. It returns an iterator that yields the elements of the list in reverse order. The reversed() method does not modify the original list.
For example, the following code reverses the list my_list and assigns the result to the iterator reversed_list:
Example
my_list = [1, 2, 3, 4, 5]
# Reverse the list my_list
reversed_list = reversed(my_list)
# Print the reversed list
print(reversed_list)
#Output: [5, 4, 3, 2, 1]
Using .reverse()
Method
The .reverse() method in Python is used to reverse a list in place. This means that it modifies the original list to contain the elements of the list in reverse order.
For example, the following code reverses the list my_list:
Example
my_list = [1, 2, 3, 4, 5]
# Reverse the list my_list
my_list.reverse()
# Print the reversed list
print(my_list)
#Output: [5, 4, 3, 2, 1]
Sorting a Python List
Python lists have a couple of methods to sort elements:
Using sorted()
Method
The sorted() function in Python is used to sort a list. It returns a new list containing the elements of the original list in sorted order. The original list is not modified.
For example, the following code sorts the list my_list in ascending order:
Example
my_list = [1, 5, 3, 4, 2]
# Sort the list my_list in ascending order and assign the sorted list to the new list sorted_list
sorted_list = sorted(my_list)
# Print the sorted list
print(sorted_list)
#Output: [1, 2, 3, 4, 5]
Using .sort()
Method
The sort() method in Python is used to sort a list in ascending order by default. It can also be used to sort a list in descending order by passing the reverse=True argument. The sort() method modifies the original list in place.
For example, the following code sorts the list my_list in ascending order:
Example
my_list = [1, 5, 3, 4, 2]
# Sort the list my_list in ascending order
my_list.sort()
# Print the sorted list
print(my_list)
#Output: [1, 2, 3, 4, 5]
Nested Python Lists
A nested list in Python is a list that contains other lists. This can be useful for representing data that has multiple dimensions, such as a 2D grid or a 3D matrix.
To create a nested list in Python, we can simply create a list and put one or more lists inside of that list.
Syntax
list_name = [ [item1, item2, …], [item1, item2, …], … ]
Explanation
- list_name is the name of the nested list.
- [] are square brackets, which are used to create a list in Python.
[[item1, item2, ...]]
is a sublist. It contains a list of elements, separated by commas.- , is a comma, which is used to separate elements in a list.
- ... indicates that there can be multiple sublists in the nested list.
Example
my_list = [[], []]
Explanation
my_list
is the name of the nested list.[ ]
are square brackets, which are used to create a list in Python.[[ ], [ ]]
are two empty sublists.
For example, the following code creates a 2D nested list:
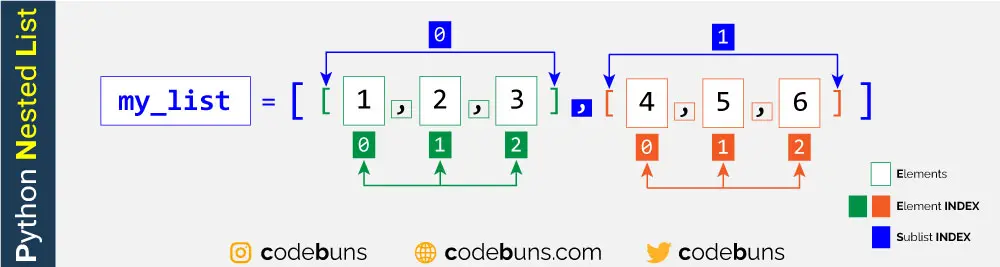
my_list = [[1, 2, 3], [4, 5, 6]]
OR
my_list
[
[1, 2, 3],
[4, 5, 6]
]
Explanation
- This code creates a nested list called my_list.
- The first sublist of my_list contains the elements 1, 2, and 3.
- The second sublist of my_list contains the elements 4, 5, and 6.
Access Elements of a Nested List
To access elements of a Python nested list, we can use nested index expressions. A nested index expression is an expression that contains multiple index operators.
Syntax
list_name[sublist_index][element_index]
For example, to access the element at row 1, column 2 of the 2D nested list above, we would use the following code:
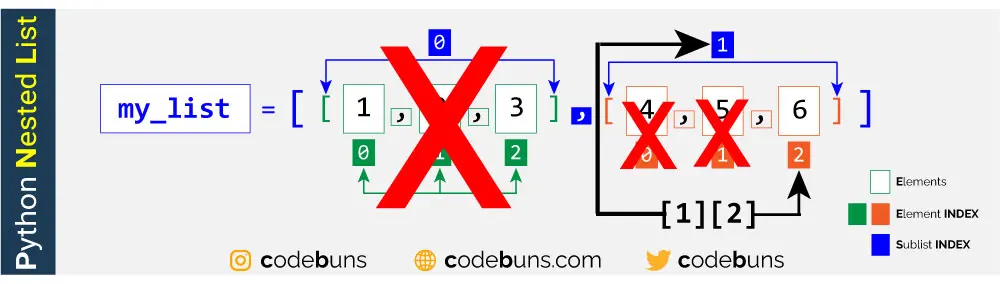
my_list = [[1, 2, 3], [4, 5, 6]]
element = my_list[1][2]
print(element)
#Output: 6
Add Elements to a Nested List
To add elements to a Python nested list, we can use the append() or insert() method.
- The append() method adds an element to the end of a list.
- The insert() method inserts an element at a specific index in a list, shifting elements to the right.
Syntax of insert()
Method
my_list[sublist_index].insert(index, element)
The following code inserts the element 7 at index 2 in the first sublist of the nested list my_list:
my_list = [[1, 2, 3], [4, 5, 6]]
my_list[0].insert(2, 7)
print(my_list)
#Output: [[1, 2, 7, 3], [4, 5, 6]]
Syntax of append()
Method
list_name[sublist_index].append(element)
For example, the following code adds the element 7 to the end of the first sublist of the nested list my_list:
my_list = [[1, 2, 3], [4, 5, 6]]
my_list[0].append(7)
print(my_list)
#Output: [[1, 2, 3, 7], [4, 5, 6]]
Repeat append() method for each element we want to add to the nested list.
my_list[0].append(2)
my_list[1].append(3)
my_list[1].append(4)
print(my_list)
#Output: [[1, 2, 3, 7, 2], [4, 5, 6, 3, 4]]
This adds the elements 2, 3, and 4 to the nested list my_list.
Here is a complete example of adding elements to a nested list in Python:
# Create a nested list
my_list = [[], []]
# Add elements to the nested list
my_list[0].append(1)
my_list[0].append(2)
my_list[1].append(3)
my_list[1].append(4)
# Print the nested list
print(my_list)
#Output: [[1, 2], [3, 4]]
Note: We can also use the insert() method to add elements to a nested list. However, the append() method is more commonly used.
Add Elements to a Nested List Using List Comprehension
We can also use list comprehensions to add elements to a nested list in a more concise way. For example, the following code adds the elements 7, 8, and 9 to the end of each sublist in the nested list my_list:
my_list = [[1, 2, 3], [4, 5, 6]]
my_list = [[element + 7 for element in sublist] for sublist in my_list]
print(my_list)
#Output: [[8, 9, 10], [11, 12, 13]]
Update Elements of a Nested List
To update the elements of a Python nested list, we can use the following steps:
- Access the element to be updated using nested index expressions.
- Assign a new value to the element.
Syntax
Access the element to be updated using nested index expressions
list_name[sublist_index][element_index]
Assign a new value to the element
list_name[sublist_index][element_index] = new_value
Explanation
- sublist_index: This is the index number of the outer list we want to access.
- list_name[sublist_index][element_index]: After accessing the desired outer list, use the inner index to access the specific element within that list.
- new_value: This is the value we want to assign to the specific position in the nested list.
Example
# Create a nested list
my_list = [[1, 2, 3], [4, 5, 6]]
# Update the third element of the first sublist to 7
my_list[0][2] = 7
# Update the third element of the second sublist to 13.
my_list[1][2] = 13
# Print the nested list
print(my_list)
#Output: [[1, 7, 3], [4, 5, 13]]
Looping Through Nested List
Looping through nested lists is a common operation in Python, especially when dealing with data structures like matrices or data from JSON-like structures. Python offers several methods to traverse nested lists, each with its benefits.
Nested For-Loops
To loop through a nested list, we can use two nested for loops. The outer for loop will iterate over the sublists in the nested list, and the inner for loop will iterate over the elements in each sublist.
Syntax
for sublist in list_name:
for element in sublist:
# Do something with the element.
Explanation
- for is a loop statement that iterates over a sequence of elements.
- sublist is a variable that will be assigned to each sublist in the nested list.
- list_name is the name of the nested list to be iterated over.
- element is a variable that will be assigned to each element in the sublist.
- The code block within the inner loop will be executed for each element in each sublist.
For example, the following code loops through the nested list my_list and prints each element to the console:
Example
my_list = [[1, 2, 3], [4, 5, 6]]
# Loop through the nested list and print each element.
for sublist in my_list:
for element in sublist:
print(element)
Output
1
2
3
4
5
6
Using Enumerate for Index and Value
The enumerate() function can be used to get both the index and value of items in a list, which can be handy when traversing nested lists.
Syntax
for i, innerList in enumerate(nestedList):
for j, item in enumerate(innerList):
#do something with index and value(i, j, item)
Explanation
for i, innerList in enumerate(nestedList):
- i: The index of the sublist.
- innerList: The sublist itself.
- enumerate(): A function that returns a sequence of tuples, where each tuple contains the index and element from the original sequence.
- nestedList: The nested list to be iterated over.
for j, item in enumerate(innerList):
- j: The index of the element in the sublist.
- item: The element itself.
Example
my_list = [[1, 2, 3], [4, 5, 6]]
for i, inner_list in enumerate(my_list):
for j, element in enumerate(inner_list):
print(f"Element at position {i},{j} is {element}")
Output
Element at position 0,0 is 1
Element at position 0,1 is 2
Element at position 0,2 is 3
Element at position 1,0 is 4
Element at position 1,1 is 5
Element at position 1,2 is 6
Python List vs Tuple
Conclusion
Python lists are like the Swiss Army knife of data structures. They are simple and easy to use, but they are also incredibly powerful and versatile. Lists can be used to represent a wide variety of data structures, including matrices, trees, and graphs. They can also be used to implement a variety of algorithms, such as sorting, searching, and traversing data structures.
Python Reference
FAQ
What is a Python list and how is it different from other data structures?
A Python list is a built-in data type that can hold a collection of items, which can be of any type, including other lists. Lists are ordered, mutable, and can contain duplicate values. This differentiates them from sets (unordered and without duplicates), tuples (ordered but immutable), and dictionaries (key-value pairs).
How do you add or remove items from a Python list?
To add items to a list, you can use the append() method for single items or extend() for multiple items from another list. To insert an item at a specific index, use insert(index, item). To remove an item, remove(item) deletes the first occurrence, while pop(index) removes and returns the item at a specific index.
How do you sort or reverse a list in Python?
To sort a list in ascending order, use the sort() method. For descending order, use sort(reverse=True). If you want to return a sorted list without modifying the original, use sorted(list). To reverse the order of items in a list, you can use the reverse() method or the slicing technique list[::-1].
Are Python lists thread-safe?
Python lists are not inherently thread-safe. If multiple threads modify a list simultaneously without proper synchronization, unpredictable results may occur. To ensure thread safety when working with lists, use synchronization mechanisms like locks or utilize data structures from the collections module designed for concurrent access.
How can you create a deep copy of a list in Python?
To create a deep copy of a list, meaning a new list with entirely independent copies of the nested items, you can use the copy module's deepcopy() function. This ensures that even nested lists or objects inside the original list are replicated without keeping references to the originals.