What Is Tuple in Python?
A tuple in Python is a collection of ordered, immutable elements. Unlike lists, tuples are immutable, which means their elements cannot be modified after they have been created. Tuples can hold any type of data, including numbers, strings, and other objects.
Tuples are particularly useful when we want to group specific values and ensure they aren’t accidentally modified elsewhere in the code. They can also be used as keys in dictionaries, whereas lists cannot due to their mutability.
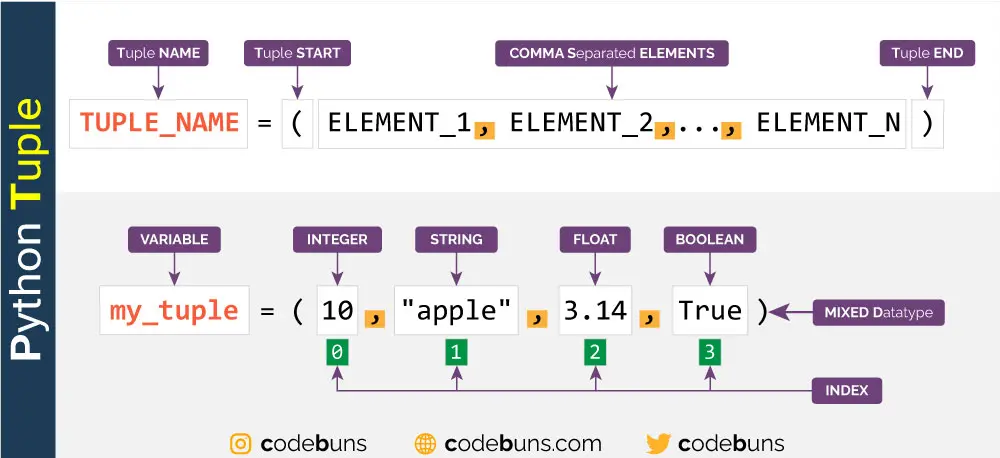
Python Tuple Example
The example demonstrates how to define a tuple in Python.
my_tuple = (10, "apple", 3.14, True)
Explanation
- Initialization: The variable
my_tuple
is assigned a tuple value. - Mixed Data Types: Tuples can store multiple data types. In this case,
my_tuple
contains an integer (1), a string (“apple“), a float (3.14), and a boolean (True). - Accessing Elements: To access elements in a tuple, indexing is used. For instance,
my_tuple[1]
would return “apple“.
Python Tuple Syntax
The general syntax of a tuple is:
TUPLE_NAME = (ELEMENT_1, ELEMENT_2, …, ELEMENT_N)
Explanation
- Tuple Declaration: In the tuple syntax, TUPLE_NAME represents the variable name that holds the tuple.
- Parentheses: Tuples are enclosed in parentheses ( ).
- Elements: Inside the parentheses, individual elements (ELEMENT_1, ELEMENT_2, etc.) are separated by commas.
The Core Characteristics of Tuple in Python
Tuple in Python provides a reliable and efficient way to handle grouped data. As with any data type, understanding their characteristics ensures their optimal use.
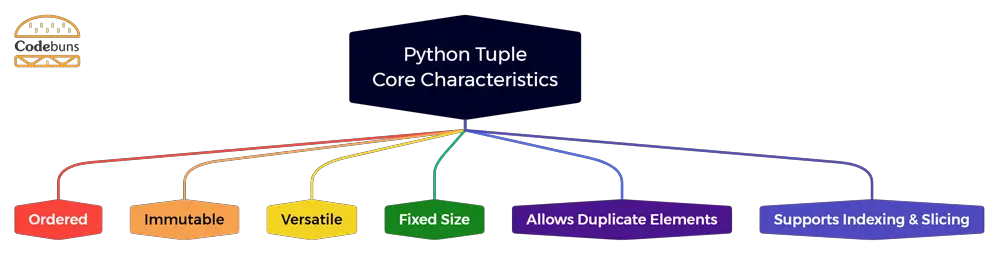
Ordered
- Example:
- Consider a tuple
fruits = ("apple", "banana", "cherry")
. The order of the elements will always be maintained as “apple” first, “banana” second, and “cherry” third.
- Consider a tuple
- Explanation:
- Elements maintain a consistent sequence.
- The first item is always at index 0, the second at index 1, and so on.
- The order is only rearranged if a new tuple is created.
Immutable
- Example:
- If we take the previous tuple fruits, attempting to change “apple” to “grape” like this:
fruits[0] = "grape"
would result in an error.
- If we take the previous tuple fruits, attempting to change “apple” to “grape” like this:
- Explanation:
- After creation, the elements of the tuple can’t be altered.
- Provides safety against accidental data modification.
- Any attempt at modification throws an error, ensuring data integrity.
Versatile
- Example:
- A tuple can encapsulate various data types. For instance,
mix = (3, "hello", [1, 2, 3], {"key": "value"})
comprises an integer, string, list, and dictionary, all in a single tuple.
- A tuple can encapsulate various data types. For instance,
- Explanation:
- Mix and match data types as needed.
- Offers flexibility in storing diverse data.
- Useful for complex data structures like database records.
Fixed Size
- Example:
- If you define a tuple with 3 elements, e.g.,
tpl = (14, 15, 16)
, it will always have a size of 3.
- If you define a tuple with 3 elements, e.g.,
- Explanation:
- Length is determined at the time of tuple creation.
- Unlike lists, size can’t be dynamically altered.
- Provides stability for tasks that require fixed sizes.
Allows Duplicate Elements
- Example:
- A tuple can have repeated elements, e.g.,
tpl = (7, 7, 8)
.
- A tuple can have repeated elements, e.g.,
- Explanation:
- It’s possible to have multiple identical items.
- Useful in scenarios where repetition of data is valid.
- No restrictions on the count of duplicates.
Supports Indexing and Slicing
- Example:
- With a tuple
tpl = (10, 11, 12, 13)
, we can accesstpl[1]
to get 11 or slicetpl[1:3]
to get (11, 12).
- With a tuple
- Explanation:
- Elements are accessible via their position (index).
- Slicing allows getting a subrange of the tuple.
- Indexing starts from 0 for the first element.
Creating a Tuple in Python
In Python, creating a tuple can be done in several ways: by enclosing a comma-separated list of items in parentheses, using tuple() constructor, or even without parentheses when assigning multiple values. It’s also possible to create a tuple with a single item by placing a trailing comma after it. These methods ensure flexibility while working with tuples in Python programming.
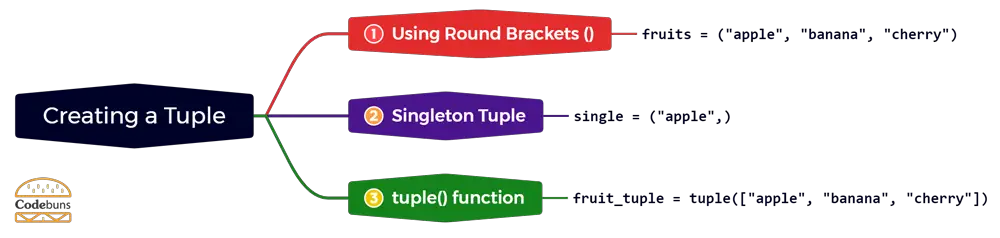
Create Python Tuple Using Round Brackets ()
To create a tuple in Python using round brackets, we simply list the elements of the tuple inside a pair of round brackets, separated by commas. The use of round brackets distinguishes tuples from other data types in Python, and they are especially useful for representing fixed collections of items or records where each position has a specific meaning or significance.
The following code creates a tuple of fruits:
Example
fruits = ("apple", "banana", "cherry")
Explanation
fruits
is the name of the tuple.()
are the round brackets used to create the tuple.- “apple”, “banana”, and “cherry” are the elements of the tuple.
,
are the commas that separate the elements of the tuple.
Once a tuple is created, it cannot be modified. This means that we cannot add, remove, or change the elements of the tuple.
Here is an example of how to use the fruits tuple:
print(fruits[0]) # Prints "apple"
print(fruits[1]) # Prints "banana"
print(fruits[2]) # Prints "cherry"
# We cannot modify the tuple
# fruits[0] = "orange" # This will generate an error
Create a Python Tuple with One Element (Singleton Tuple)
To create a tuple with one element (singleton tuple) in Python, we simply follow the same steps as creating a regular tuple, but with the addition of a comma at the end of the single element. The comma is necessary to distinguish the singleton tuple from a parenthetical expression.
Here is an example of how to create a singleton tuple:
Singleton Tuple Example
single = ("apple",)
Explanation
single
is the name of the singleton tuple.()
are the round brackets used to create the tuple.- “apple” is the element of the tuple.
,
is the comma that indicates that the tuple has one element.
Once a singleton tuple is created, it cannot be modified, just like any other tuple.
Here is an example of how to use a single tuple:
print(single[0]) # Prints "apple"
# We cannot modify the tuple
# single[0] = "orange" # This will generate an error
Singleton tuples are useful in various situations, such as when we need to pass a single element to a function that expects a tuple.
Python tuple()
function
The Python tuple() function creates a tuple from an iterable. The iterable can be a list, a string, or another tuple.
To use the tuple() function, we simply pass the iterable to the function as an argument. For example, the following code creates a tuple of fruits:
Example
fruit_tuple = tuple(["apple", "banana", "cherry"])
print(fruit_tuple) # Prints ('apple', 'banana', 'cherry')
Explanation
fruit_tuple
is the name of the tuple variable.tuple(...)
is the function that creates the tuple.["apple", "banana", "cherry"]
is the iterable that the tuple is created from.
Adding Elements in a Python Tuple
Since tuples are immutable, elements cannot be added directly. However, two tuples can be concatenated to form a new tuple.
To add elements in a Python tuple, we can use the + operator. The + operator will create a new tuple containing all the elements of the two original tuples. For example, the following code creates a new tuple called new_tuple that contains the elements of the tuples tuple1 and tuple2:
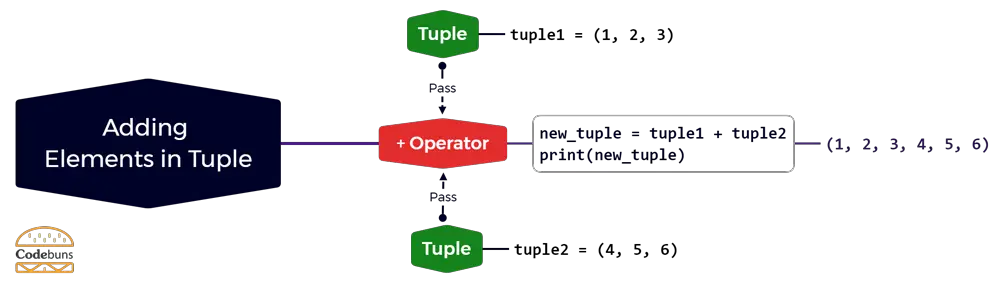
Example
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
new_tuple = tuple1 + tuple2
Explanation
tuple1
andtuple2
are the two original tuples.- + is the operator, which is used to add the two tuples together.
new_tuple
is the new tuple that contains the elements of the two original tuples.
Here is an example of how to use the new_tuple tuple:
print(new_tuple) # Prints (1, 2, 3, 4, 5, 6)
Adding elements to tuples can be useful in various situations, such as when we need to combine two tuples into one or when we need to add a new element to a tuple.
Updating Elements in a Python Tuple
Directly updating an element is not feasible due to immutability. But you can concatenate parts of tuples to achieve a similar outcome.
To update elements in a Python tuple, we can use a combination of slicing and concatenation. Slicing allows us to extract a subset of elements from a tuple, while concatenation allows us to combine two tuples. For example, the following code updates the second element of the tuple tuple1 to 9:
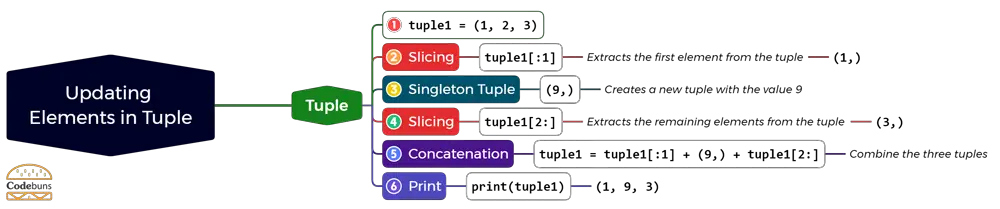
Example
tuple1 = (1, 2, 3)
tuple1 = tuple1[:1] + (9,) + tuple1[2:]
Explanation
tuple1
is the tuple that we want to update.tuple1[:1]
extracts the first element from the tuple.(9,)
creates a new tuple with the value 9.tuple1[2:]
extracts the remaining elements from the tuple.- + is the concatenation operator, which is used to combine the three tuples.
Here is an example of how to use the updated tuple tuple1:
print(tuple1) # Prints (1, 9, 3)
Updating elements in tuple can be useful in various situations, such as when we need to correct a mistake in a tuple or when we need to change the value of an element in a tuple.
Accessing Elements in Python Tuple
There are two main ways to access elements of a Python tuple:
- Indexing: Use square brackets [] and the element’s index to access tuple elements.
- Slicing: Use the slice operator : to access a range of elements in a tuple.
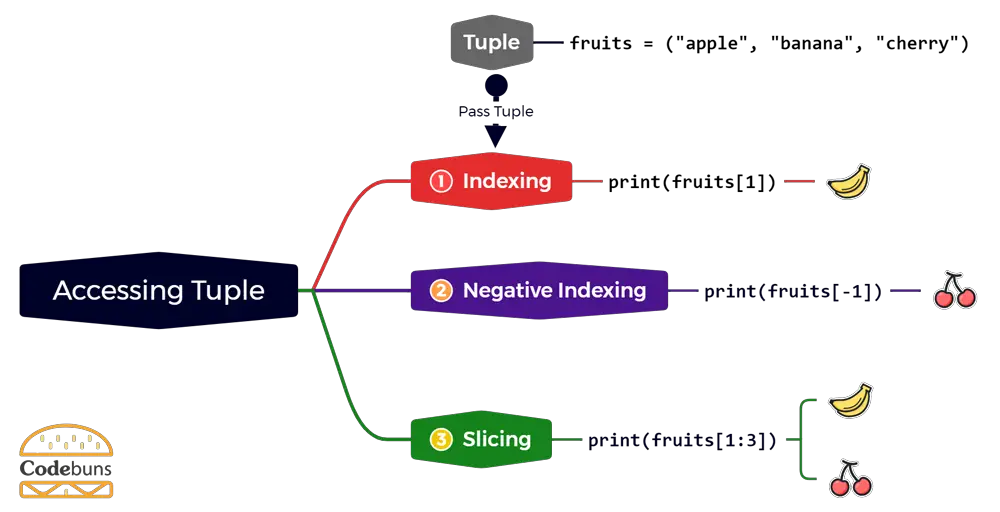
Indexing to Access Tuple Elements
Indexing is a way to access the elements of a tuple by their position.
To index a Python tuple element, we use the square bracket ([]) operator. The index of the first element in a tuple is 0, and the index of the last element is the length of the tuple minus 1. For example, the following code prints the second element of the tuple fruits:
Example
fruits = ("apple", "banana", "cherry")
print(fruits[1])
# Output: banana
Explanation
fruits = ("apple", "banana", "cherry")
creates a tuple called fruits with the elements apple, banana, and cherry.print(fruits[1])
prints the element at index 1 of the tuple fruits, which is banana.
Negative Indexing in Tuple
Negative indexing is a way to access the elements of a tuple from the end.
To use negative indexing in Python tuples, we simply use negative integers as indices. The index of the first element in a tuple is 0, and the index of the last element is the length of the tuple minus 1. So, the index of the second-to-last element is -1, the index of the third-to-last element is -2, and so on. For example, the following code prints the last element of the tuple fruits using negative indexing:
Example
fruits = ("apple", "banana", "cherry")
print(fruits[-1])
# Output: cherry
Explanation
fruits = ("apple", "banana", "cherry")
creates a tuple called fruits with the elements apple, banana, and cherry.print(fruits[-1])
prints the element at index -1 of the tuple fruits, which is cherry.
Negative indexing can be a useful way to access and manipulate tuples, especially when we need to work with the elements of the tuple in reverse order.
Slicing a Tuple
Slicing a tuple is a way to create a new tuple from a subset of the elements of the original tuple.
To slice a tuple in Python, we use the square bracket ([]) operator with a colon (:). The colon separates the start and end indices of the slice. The start index is inclusive, and the end index is exclusive. For example, the following code prints a subtuple containing the second and third elements of the tuple fruits:
Example
fruits = ("apple", "banana", "cherry")
print(fruits[1:3])
# Output: ('banana', 'cherry')
Explanation
fruits = ("apple", "banana", "cherry")
creates a tuple called fruits with the elements apple, banana, and cherry.print(fruits[1:3])
prints the elements of the tuple fruits from index 1 to index 3, excluding index 3.
Finding an Element in a Python Tuple
We can find an element in a Python tuple using several methods, some of which are outlined below.
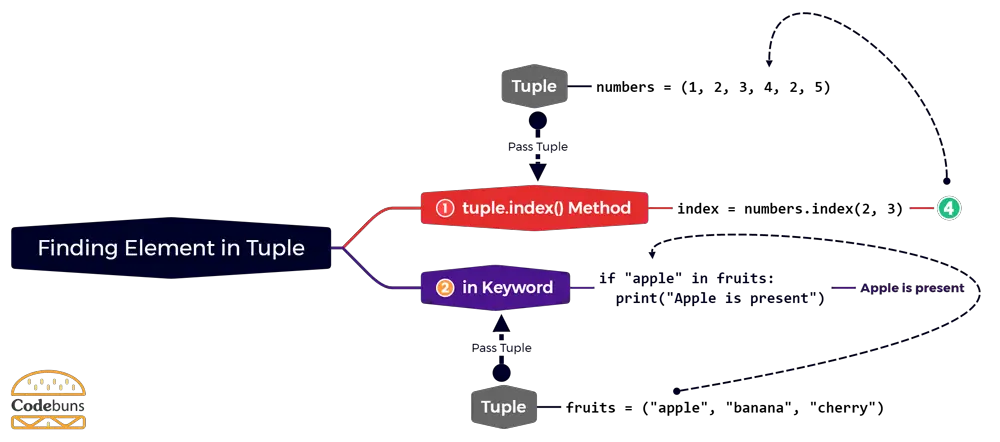
Find an Element Within a Range (using tuple.index()
Method)
The index() method in Python tuples can take an optional third argument, start, which specifies the index at which to start the search for the element. If start is not specified, the search starts at the beginning of the tuple.
To find an element within a range in a Python tuple, we can simply pass the start and end indices of the range to the index() method as the second and third arguments. For example, the following code finds the index of the first occurrence of the element 2 in the tuple numbers, starting at index 3:
Example
numbers = (1, 2, 3, 4, 2, 5)
# Find the index of the first occurrence of the element 2 in the tuple, starting from index 3:
index = numbers.index(2, 3)
# Print the index:
print(index)
# Output: 4
This code will return index 4, because that is the first occurrence of element 2 in the tuple, starting at index 3. If the element is not found within the specified range, the index() method will raise a ValueError exception.
Explanation
numbers = (1, 2, 3, 4, 2, 5)
creates a tuple called numbers with the elements 1, 2, 3, 4, 2, and 5.index = numbers.index(2, 3)
finds the index of the first occurrence of the element 2 in the tuple numbers, starting from index 3.print(index)
prints the index to the console.
Checking if an Element Exists in a Tuple (using in
Keyword)
To check if an element exists in a Python tuple, we can use the in operator. The in operator returns True if the element is found in the tuple, and False otherwise. For example, the following code checks if the element “apple” is present in the tuple fruits:
Example1
fruits = ("apple", "banana", "cherry")
# Check if the element "apple" is present in the tuple:
if "apple" in fruits:
print("Apple is present")
# Output: Apple is present
Explanation
fruits = ("apple", "banana", "cherry")
creates a tuple called fruits with the elements apple, banana, and cherry.if "apple" in fruits:
checks if the element “apple” is present in the tuple fruits.print("Apple is present")
prints the message “Apple is present” to the console if the element “apple” is present in the tuple fruits.
The following code checks if subtuple (3, 4) is present in the tuple main_tuple:
Example2
# Define a tuple with several subtuples
main_tuple = (1, 2, (3, 4), (5, 6), 7, 8)
# Check if a subtuple exists within the main tuple
subtuple_to_search = (3, 4)
if subtuple_to_search in main_tuple:
print(f"The subtuple {subtuple_to_search} exists within the main tuple.")
Deleting Elements from a Python Tuple
While you can’t directly delete an element from a tuple due to its immutable nature, you can delete an entire tuple using the del keyword. To “delete” individual elements, you’d typically convert the tuple to a mutable type (like a list), perform the deletion, and then convert it back to a tuple if needed.
Using del
Keyword
The del keyword in Python is used to delete objects, variables, functions, classes, and even modules. To delete a tuple using the del keyword, we simply pass the tuple name to the del keyword. For example, the following code deletes the tuple fruits:
Example
fruits = ("apple", "banana", "cherry")
# Delete the entire tuple:
del fruits
# Try to access the tuple:
print(fruits)
Output
Traceback (most recent call last):
File “<stdin>”, line 4, in <module>
NameError: name ‘fruits’ is not defined
Explanation
fruits = ("apple", "banana", "cherry")
creates a tuple called fruits with the elements apple, banana, and cherry.del fruits
deletes the entire tuple fruits.print(fruits)
tries to access the tuple fruits, but since it has been deleted, a NameError exception is raised.
You can’t remove individual elements from a tuple, but you can delete the tuple entirely using the del keyword. Once the tuple is deleted, it cannot be recovered.
Packing and Unpacking Tuple in Python
Tuple packing and unpacking are two related operations in Python. Tuple packing is the process of creating a tuple from a sequence of elements. Tuple unpacking is the process of extracting the elements of a tuple into a sequence of variables.
These operations allow efficient assignment and extraction of multiple variables without intermediary steps. Understanding this feature can simplify code and improve readability.
Tuple Packing
When we create a tuple without using parentheses (), enclosing multiple elements separated by commas (,), it’s known as tuple packing.
Syntax
tuple_name = value1, value2, …, valueN
For example, the following code creates a tuple of fruits:
Example of Tuple Packing
fruits = "apple", "banana", "cherry"
print(fruits)
Explanation
- We define three string values: “apple”, “banana”, and “cherry”.
- Without enclosing them in parentheses, we separate them using commas. This is tuple packing.
- The variable fruits now holds a tuple with three elements.
- The
print
statement outputs: (‘apple’, ‘banana’, ‘cherry’)
Tuple Unpacking
Tuple unpacking is a way to assign multiple values from a tuple to multiple variables in a single line of code.
Syntax
variable1, variable2, …, variableN = tuple_name
For example, the following code unpacks the fruits tuple into three variables:
Example of Tuple Unpacking
fruits = ("apple", "banana", "cherry")
fruit1, fruit2, fruit3 = fruits
print(fruit1, fruit2, fruit3)
Explanation
- We initially define a tuple fruits containing three elements.
- We then declare three new variables (fruit1, fruit2, and fruit3).
- The tuple unpacking process assigns each value from the fruits tuple to the corresponding variable in order. fruit1 gets “apple”, fruit2 gets “banana”, and fruit3 gets “cherry”.
- The print statement outputs: apple banana cherry
Tuple Packing and Unpacking Example
The following code shows how to use tuple packing and unpacking:
fruits = "apple", "banana", "cherry"
# Pack the elements of the fruits sequence into a tuple.
fruit_tuple = fruits
# Unpack the fruit_tuple tuple into three variables.
fruit1, fruit2, fruit3 = fruit_tuple
# Print the fruits.
print(fruit1) # Prints "apple"
print(fruit2) # Prints "banana"
print(fruit3) # Prints "cherry"
Output
apple
banana
Cherry
Iterating a Python Tuple
To iterate over a tuple in Python, we can use a for loop. The for loop will iterate over tuple’s elements and execute the code block inside the loop for each element.
Example
t1 = (1, 2, 3)
for item in t1:
print(item)
Output
1
2
3
Explanation
t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.for item in t1:
iterates over the elements of the tuple t1 and assigns each element to the variable item.print(item)
prints the value of the variable item to the console.
Using the enumerate()
Function
The enumerate() function in Python is a built-in function that takes an iterable object as an argument and returns an iterator of tuples, where the first element of each tuple is the index of the corresponding element in the iterable object, and the second element is the element itself.
Syntax
for idx, item in enumerate(iterable_object):
# Code to be executed
Explanation
- idx is the index of the current element in the iterable object.
- item is the current element in the iterable object.
Example
t1 = (1, 2, 3)
for idx, item in enumerate(t1):
print(f"Index {idx} has value {item}")
Output
Index 0 has value 1
Index 1 has value 2
Index 2 has value 3
Explanation
for idx, item in enumerate(t1):
This line of code starts a for loop that will iterate over the elements of the tuple t1. The idx variable will be assigned the index of the current element in the tuple, and the item variable will be assigned the current element.print(f"Index {idx} has value {item}"):
This line of code prints the index and value of the current element in the tuple.
Copying Python Tuple
To copy a tuple in Python, we can use the slice operator [:]. The slice operator creates a new tuple that contains all of the elements of the original tuple.
Example
original = (1, 2, 3, 4)
copy = original[:]
print(original)
print(copy)
Output
(1, 2, 3, 4)
(1, 2, 3, 4)
As you can see, the copy tuple is a copy of the original tuple.
Explanation
original = (1, 2, 3, 4)
creates a tuple called original with the elements 1, 2, 3, and 4.copy = original[:]
creates a new tuple called copy from the tuple original. The [:] slice operator is used to copy all of the elements from the tuple original to the tuple copy.print(original)
prints the tuple original to the console.print(copy)
prints the tuple copy to the console.
Note
- The [:] slice operator copies the elements of a tuple by value. This means that the new tuple is a completely separate object from the original tuple.
- We cannot change the elements of the original tuple by changing the elements of the copy tuple.
Converting Python Tuple into a List
Tuples are immutable, meaning their elements cannot be modified directly. However, we can still remove elements from a tuple by converting it to a list. This is because lists are mutable, meaning their elements can be changed once created.
To delete an element from a tuple in Python, we can convert it into a list, remove the element from the list, and then convert the list back to a tuple.
For example, the following code removes the element “banana” from the tuple fruits:
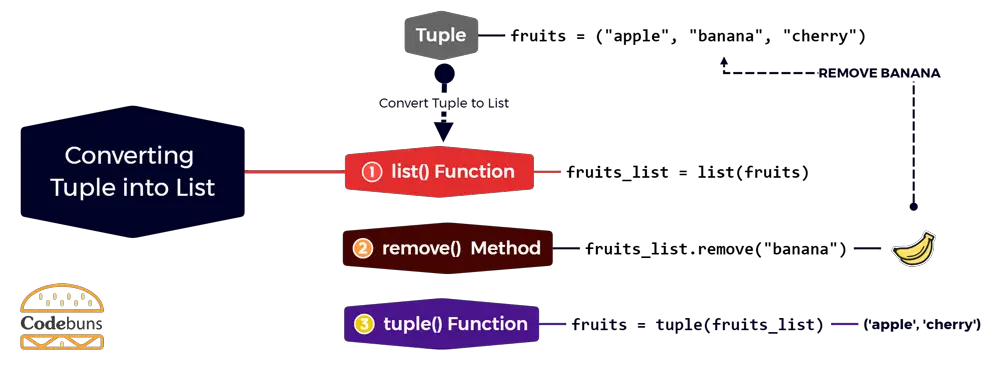
Example
fruits = ("apple", "banana", "cherry")
# Convert the tuple to a list.
fruits_list = list(fruits)
# Remove the element "banana" from the list.
fruits_list.remove("banana")
# Convert the list back to a tuple.
fruits = tuple(fruits_list)
print(fruits)
# Output: ('apple', 'cherry')
Explanation
fruits = ("apple", "banana", "cherry")
creates a tuple called fruits with the elements apple, banana, and cherry.fruits_list = list(fruits)
converts the tuple fruits to a list.fruits_list.remove("banana")
removes the element “banana” from the list fruits_list.fruits = tuple(fruits_list)
converts the list fruits_list back to a tuple.print(fruits)
prints the tuple fruits to the console.
Note: This method requires converting the tuple to a list and back to a tuple, which can be inefficient if the tuple is large.
Converting a List to a Python Tuple
To convert a list to a tuple in Python, we can use the tuple() function. The tuple() function takes an iterable object, such as a list, as an argument and returns a tuple containing the elements of the iterable object. For example, the following code converts the list list_data to a tuple:
Example
list_data = [1, 2, 3]
tuple_data = tuple(list_data)
print(tuple_data)
# Output: (1, 2, 3)
Explanation
list_data = [1, 2, 3]
creates a list called list_data with the elements 1, 2, and 3.tuple_data = tuple(list_data)
converts the list list_data to a tuple and assigns the result to the variable tuple_data. The tuple() function in Python takes an iterable as input and returns a tuple containing the elements of the iterable.print(tuple_data)
prints the tuple tuple_data to the console.
Operations on Python Tuple
Operations on tuples often revolve around accessing their elements, iterating through them, or performing standard manipulations like concatenation or repetition.
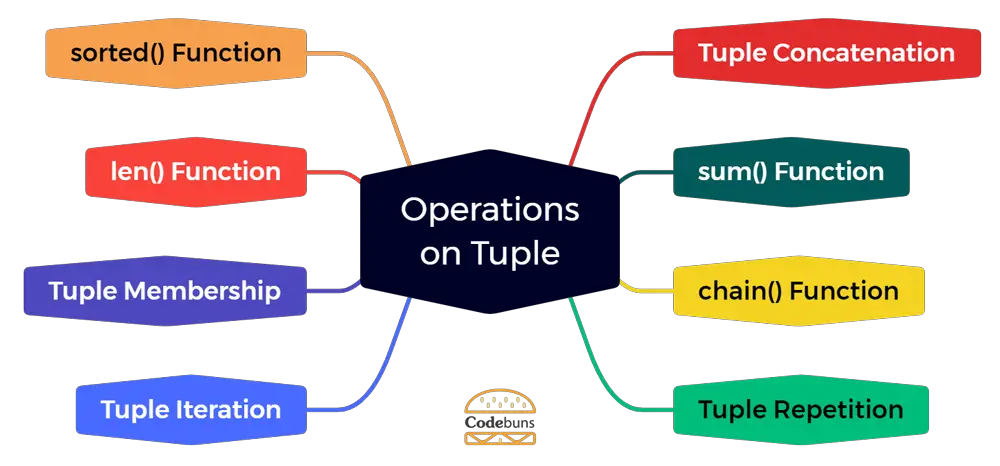
Tuple Concatenation
Tuple concatenation in Python is the process of combining two or more tuples into a single tuple. This can be done using the + operator.
To concatenate tuples, we simply place the + operator between the tuples that we want to concatenate. For example, the following code concatenates the tuples t1 and t2 into a new tuple called result:
Example
t1 = (1, 2, 3)
t2 = (4, 5, 6)
result = t1 + t2
print(result)
# Output: (1, 2, 3, 4, 5, 6)
Explanation
t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.t2 = (4, 5, 6)
creates a new tuple called t2 with the elements 4, 5, and 6.result = t1 + t2
concatenates the two tuples t1 and t2 and assigns the result to the variable result.print(result)
prints the tuple result to the console.
As you can see, the result tuple contains all of the elements of the original tuples in the order in which they were concatenated.
Note: Tuple concatenation does not modify the original tuples.
Using the sum()
Function
The sum() function in Python can be used to sum the elements of a sequence. The sequence can be a list, a tuple, or a string. The sum() function takes two arguments:
- iterable: The sequence whose elements are to be summed.
- start: The value to start the summing process with. This is an optional argument. If start is not specified, the summing process starts with 0.
The following Python code shows how to use the sum() function to add the elements of a list of tuples:
Example
tuples_list = [(1, 2), (3, 4), (5, 6)]
result = sum(tuples_list, ())
print(result)
# Output: (1, 2, 3, 4, 5, 6)
Explanation
tuples_list = [(1, 2), (3, 4), (5, 6)]
creates a list of tuples called tuples_list.result = sum(tuples_list, ())
sums the elements of the tuples in the list tuples_list and assigns the result to the variable result. The empty tuple()
is passed as the second argument to the sum() function to start the summing process with an empty tuple.print(result)
prints the value of the variable result to the console.
The result variable will contain a tuple containing all of the elements of the original tuples, in the order in which they were summed.
Note: The sum() function does not modify the original tuples.
Using the chain()
Function
To use the itertools.chain() function to concatenate tuples, we simply pass the tuples that we want to concatenate to the chain() function as arguments. The chain() function will return an iterator that yields the elements of the input tuples in sequence.
To convert the iterator to a tuple, we can use the tuple() function.
For example, the following code concatenates the tuples t1 and t2 into a new tuple called result using the chain() function:
Example
import itertools
t1 = (1, 2, 3)
t2 = (4, 5, 6)
result = tuple(itertools.chain(t1, t2))
print(result)
# Output: (1, 2, 3, 4, 5, 6)
Explanation
import itertools
imports the itertools module, which contains the chain() function.t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.t2 = (4, 5, 6)
creates a new tuple called t2 with the elements 4, 5, and 6.result = tuple(itertools.chain(t1, t2))
creates a new tuple from the elements of the tuples t1 and t2, using the itertools.chain() function. The tuple() function converts the iterator returned by the itertools.chain() function to a tuple.print(result)
prints the tuple result to the console.
Note: The itertools.chain() function can be used to concatenate any iterable of iterables, not just tuples.
Tuple Repetition
The * operator can be used to repeat tuples in Python. When we multiply a tuple by a number, the tuple is repeated the specified number of times. The resulting tuple is a new one completely separates from the original one. For example, the following code creates a repetition of the tuple t1 three times:
Example
t1 = (1, 2, 3)
result = t1 * 3
print(result)
# Output: (1, 2, 3, 1, 2, 3, 1, 2, 3)
As you can see, the result tuple contains the elements of the t1 tuple repeated three times.
Explanation
t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.result = t1 * 3
repeats the tuple t1 three times and assigns the result to the variable result.print(result)
prints the tuple result to the console.
Note: Tuple repetition does not modify the original tuple.
Tuple Iteration
To iterate over a tuple in Python, we can use a for loop. The for loop will iterate over the elements of the tuple and execute the code block inside the loop for each element. For example, the following code iterates over the tuple t1 and prints each element to the console:
Example
t1 = (1, 2, 3)
for item in t1:
print(item)
Output
1
2
3
Explanation
t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.for item in t1:
iterates over the elements of the tuple t1 and assigns each element to the variable item.print(item)
prints the value of the variable item to the console.
Note: Tuple repetition does not modify the original tuple.
Tuple Membership (using the in
operator)
To check if an element exists in a tuple in Python, we can use the in operator. The in operator returns True if the element is found in the tuple and False otherwise. For example, the following code checks if the element 2 exists in the tuple t1:
Example
t1 = (1, 2, 3)
if 2 in t1:
print("Element exists!")
# Output: Element exists!
Explanation
t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.if 2 in t1:
checks if the element 2 is present in the tuple t1 using if statement.print("Element exists!")
prints the message “Element exists!” to the console if element 2 is present in the tuple t1.
Tuple Length (using the len()
function)
To get the length of a tuple in Python, we can use the len() function. The len() function returns the number of elements in the tuple. For example, the following code gets the length of the tuple t1:
Example
t1 = (1, 2, 3)
length = len(t1)
print(length)
# Output: 3
As you can see, the length variable contains the value 3, which is the length of the tuple t1.
Explanation
t1 = (1, 2, 3)
creates a tuple called t1 with the elements 1, 2, and 3.length = len(t1)
calculates the length of the tuple t1 and assigns the result to the variable length. The len() function in Python returns the length of a sequence, which is the number of elements in the sequence.print(length)
prints the value of the variable length to the console.
Tuple Sorting (using the sorted()
function)
To sort a tuple in Python, we simply pass the tuple to the sorted() function as an argument. The sorted() function will return a sorted list of the elements of the tuple.
To convert the sorted list back to a tuple, we can use the tuple() function. For example, the following code sorts the tuple t1 and converts the sorted list to a tuple:
Example
t1 = (3, 2, 1)
sorted_tuple = tuple(sorted(t1))
print(sorted_tuple)
# Output: (1, 2, 3)
As you can see, the sorted_tuple variable contains the sorted tuple.
Explanation
t1 = (3, 2, 1)
creates a tuple called t1 with the elements 3, 2, and 1.sorted_tuple = tuple(sorted(t1))
sorts the elements of the tuple t1 and creates a new tuple from the sorted elements. The sorted() function in Python returns a sorted list of the elements of a sequence. The tuple() function is used to convert the sorted list to a tuple.print(sorted_tuple)
prints the tuple sorted_tuple to the console.
Using the count()
And index()
Methods in Python Tuple
The count() and index() methods are two useful methods that can be used on Python tuples.
count()
Method
The count() method returns the number of times a specified element appears in the tuple.
To use the count() method, we simply call the count() method on the tuple and pass the element we want to count as an argument. For example, the following code counts the number of times the element 2 appears in the tuple numbers:
Example
numbers = (1, 2, 2, 3, 4)
# Count the number of times the element 2 appears in the tuple:
count = numbers.count(2)
# Print the count:
print(count)
# Output: 2
Explanation
numbers = (1, 2, 2, 3, 4)
creates a tuple called numbers with the elements 1, 2, 2, 3, and 4.count = numbers.count(2)
counts the number of times the element 2 appears in the tuple numbers.print(count)
prints the count to the console.
This count() method can be useful for a variety of tasks, such as finding the most common element in a tuple or checking if a tuple contains a specific element.
index()
Method
The index() method returns the index of the first occurrence of a specified element in a tuple. If the element is not found in the tuple, the index() method raises a ValueError exception.
To use the index() method, we simply call the index() method on the tuple and pass the element we want to find as an argument. The following code finds the index of the first occurrence of the element 2 in the tuple numbers:
Example
numbers = (1, 2, 2, 3, 4)
# Get the index of the first occurrence of the element 2 in the tuple:
index = numbers.index(2)
# Print the index:
print(index)
# Output: 1
Explanation
numbers = (1, 2, 2, 3, 4)
creates a tuple called numbers with the elements 1, 2, 2, 3, and 4.index = numbers.index(2)
gets the index of the first occurrence of the element 2 in the tuple numbers.print(index)
prints the index to the console.
The index() method can be useful for a variety of tasks, such as finding the location of an element in a tuple or checking if a tuple contains a specific element.
Use Built-in Functions with Python Tuple
Python offers a suite of built-in functions to efficiently interact with and derive information from them. These functions provide a means to easily navigate, manipulate, and analyze tuple data without changing its core structure.
min()
and max()
Functions
To use the min() and max() functions, we simply pass the tuple to the function as an argument. The function will return the minimum or maximum value in the tuple, depending on which function is used.
- min() function returns the minimum value in a tuple.
- max() function returns the maximum value in a tuple.
For example, the following code finds the minimum and maximum values in the tuple t1:
Example
t1 = (1, 2, 3, 4, 5)
min_val = min(t1)
max_val = max(t1)
print("Minimum value:", min_val)
print("Maximum value:", max_val)
Output
Minimum value: 1
Maximum value: 5
Explanation
t1 = (1, 2, 3, 4, 5)
creates a tuple called t1 with the elements 1, 2, 3, 4, and 5.min_val = min(t1)
finds the smallest element in the tuple t1 and assigns it to the variable min_val.max_val = max(t1)
finds the largest element in the tuple t1 and assigns it to the variable max_val.print("Minimum value:", min_val)
prints the minimum value to the console.print("Maximum value:", max_val)
prints the maximum value to the console.
all()
Function
To use the all() function, we simply pass the tuple to the function as an argument. The function will return True if all elements in the tuple are True, and False otherwise. The all() function can be used on any sequence, including tuples, lists, strings, and sets. For example, the following code checks if all elements in the tuple t1 are True:
Example
t1 = (True, True, True)
result = all(t1)
print(result)
# Output: True
Explanation
t1 = (True, True, True)
creates a tuple called t1 with the elements True, True, and True.result = all(t1)
checks if all the elements in the tuple t1 are True and assigns the result to the variable result.print(result)
prints the value of the variable result to the console.
Note: The all() function returns True if the sequence is empty.
any()
Function
To use the any() function, we simply pass the tuple to the function as an argument. The function will return True if any element in the tuple is True and False otherwise. For example, the following code checks if any element in the tuple t1 is True:
Example
t1 = (False, False, True)
result = any(t1)
print(result)
# Output: True
Explanation
t1 = (False, False, True)
creates a tuple called t1 with the elements False, False, and True.result = any(t1)
checks if any of the elements in the tuplet1
are True and assigns the result to the variable result.print(result)
prints the value of the variable result to the console.
Note: The any() function returns False if the sequence is empty.
Nested Python Tuples
Nested tuples are tuples that contain other tuples. This means that the elements of a nested tuple can be either individual values or other tuples.
To create a nested tuple, we simply list the elements of the tuple inside parentheses, including the other tuples. For example, the following code creates a nested tuple with the elements 1, 2, and a tuple containing the elements 3 and 4:
Example
nested = (1, 2, (3, 4))
print(nested)
# Output: (1, 2, (3, 4))
Explanation
nested = (1, 2, (3, 4))
creates a tuple called nested with three elements: 1, 2, and a nested tuple (3, 4).print(nested)
prints the tuple nested to the console.
Ways to Access Nested Tuples
Accessing nested tuples in Python involves using multiple indices, one for each level of nesting. Here are different ways to access nested tuples:
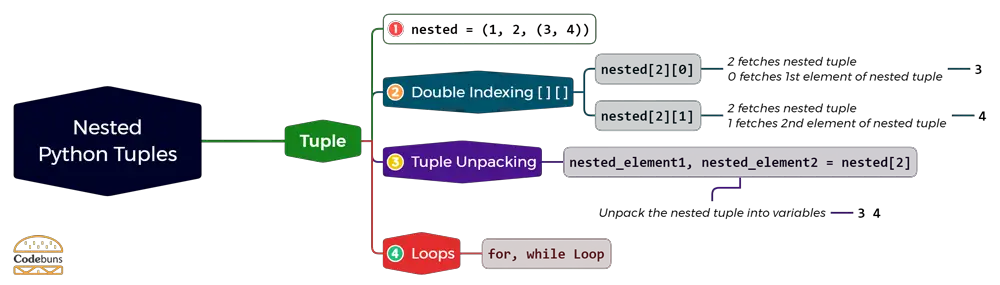
Direct Indexing: When dealing with nested data structures like nested tuples, “double indexing” is a technique used to access the inner elements. A nested tuple is essentially a tuple within another tuple.
Syntax
tuple_name[index_1][index_2]…
Explanation
- tuple_name: The name of the tuple.
- index_1: The index of the element to access in the outermost tuple.
- index_2: The index of the element to access in the next level of nesting.
- …: This indicates that there may be additional levels of nesting, and that the syntax can be extended to access elements at any level.
Example
nested = (1, 2, (3, 4))
print(nested[2][0]) # Outputs: 3
print(nested[2][1]) # Outputs: 4
Explanation
nested[2]
points to the second inner tuple, i.e., (3, 4).[0]
then fetches the first element from this inner tuple, i.e., 3.[1]
fetches the second element from the inner tuple, i.e., 4.
Understanding and utilizing double indexing is essential when working with nested collections in Python, as it allows for more precise and flexible data access.
This concept can be extended to deeper levels of nesting by adding more indices. For instance, if we have a tuple inside a tuple inside another tuple, we might end up using triple indexing, and so on.
Example
deep_nested = (1, 2, (3, 4, (5, 6)))
print(deep_nested[2][2][0]) # Outputs: 5
To access the element 5
, we can use the following indexing syntax: deep_nested[2][2][0]
. This means that we start at the outermost tuple, deep_nested, and then access the third element, which is another tuple. Then, we access the third element of that tuple, which is yet another tuple. Finally, we access the first element of that tuple, which is 5
.
Tuple Unpacking: Another way to access nested tuples is to use tuple unpacking. To do this, we use the assignment operator = and enclose the variables in parentheses (). We can use tuple unpacking to assign multiple values from a nested tuple to variables in a single expression.
Example
nested = (1, 2, (3, 4))
# Unpack the nested tuple into variables
nested_element1, nested_element2 = nested[2]
# Print the nested elements
print(nested_element1, nested_element2)
# Output: 3 4
Nested for Loops: We can also use nested for loops to iterate over nested tuples.
nested = (1, 2, (3, 4))
for element in nested:
if isinstance(element, tuple):
for inner_element in element:
print(inner_element)
else:
print(element)
Output
1
2
3
4
When we don’t know how deep the nesting goes, we can use a while loop to traverse through the tuple until we find a non-tuple element.
Syntax
while element is tuple:
element = element[index]
Example
nested_tuple = (1, 2, (3, 4, (5, 6)))
element = nested_tuple
while isinstance(element, tuple):
element = element[0]
print(element) # Outputs: 1
Explanation
- The variable element is assigned the value of nested_tuple.
- The
isinstance()
function checks if element is an instance of the tuple class. - Since nested_tuple is a tuple, the
isinstance()
function will return True. - The while loop will execute.
- Inside the while loop, the variable element is assigned the value of
element[0]
. - Since nested_tuple is a nested tuple, the first element of nested_tuple is another tuple.
- Therefore, the variable element is now assigned the value of the first element of the nested tuple.
- The
isinstance()
function checks if element is an instance of the tuple class. - Since element now contains a nested tuple, the
isinstance()
function will return True. - The while loop will execute again.
- Steps 5-10 will repeat until element is no longer a tuple.
- Once element is no longer a tuple, the while loop will exit.
- The
print()
function prints the value of element.
Each method has its own use cases. Direct indexing is straightforward and most commonly used. Unpacking is useful when the structure of the tuple is known, and you want to extract specific elements. Loops are useful for more complex tasks or when the structure might not be well-defined.
Python Tuple vs List
Python tuples and lists are two of the most common data structures in the language. While they are both useful for storing and organizing data, there are some key differences between the two.
Python Tuple
Tuple in Python is an ordered collection of elements, similar to a list, but with a key distinction: tuples are immutable. This means that once a tuple is created, its contents cannot be modified, added to, or removed. Tuples are often used to group related data, represent collections of values that shouldn’t be altered, or to ensure data integrity.
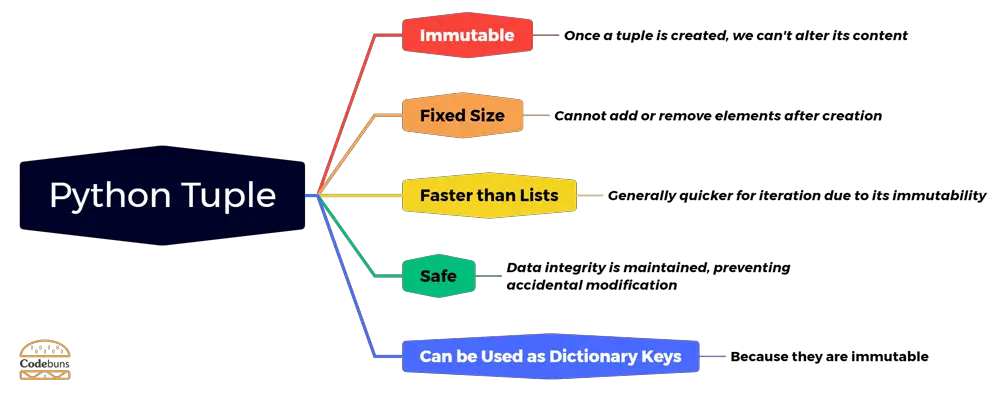
Key Points
- To create a tuple, use parentheses () and separate the elements with commas.
- To access an element of a tuple, use the [] operator and specify the index of the element. The first element in a tuple has index 0, and so on.
- To iterate over a tuple, use a for loop.
Syntax
# Create a tuple
tuple_name = (element1, element2, ..., elementN)
# Access elements of a tuple
tuple_name[index]
# Iterate over a tuple
for element in tuple_name:
# Do something with the element
Syntax Explanation
- Creating a Tuple
- tuple_name is the name of the tuple.
- element1, element2, …, elementN are the elements of the tuple.
- Accessing Elements of a Tuple
- tuple_name is the name of the tuple.
- []: Square brackets are used for indexing.
- index is the index of the element you want to access. Remember, indexing in Python starts from 0 for the first element.
- Iterating Over a Tuple
- for: This keyword indicates that the following code is a for loop.
- element: This is the variable that will be assigned each element in the tuple.
- in: This keyword indicates that the for loop will iterate over the elements of the tuple.
- tuple_name: This is the name of the tuple you want to iterate over.
Example
# Create a tuple
my_tuple = (1, 2, 3, "hello", [1, 2, 3])
# Access elements of a tuple
print(my_tuple[0]) # Outputs: 1
print(my_tuple[1]) # Outputs: 2
print(my_tuple[2]) # Outputs: 3
print(my_tuple[3]) # Outputs: hello
print(my_tuple[4]) # Outputs: [1, 2, 3]
# my_tuple[1] = 4 # This will raise a TypeError since tuples are immutable
# Iterate over the tuple
for element in my_tuple:
print(element)
Output
1
2
3
hello
[1, 2, 3]
Note: If you try to access an element of a tuple that does not exist, you will get an IndexError.
When to use Tuple in Python
- When we want a collection of items that shouldn’t be modified.
- For faster iteration over elements.
- When working with data that should remain constant throughout its usage.
Python List
A Python list is a dynamic and ordered collection of items that can be of any type. Lists are mutable, which means that the elements in a list can be changed, added, or removed after its creation. They are one of the most versatile and commonly used data structures in Python.
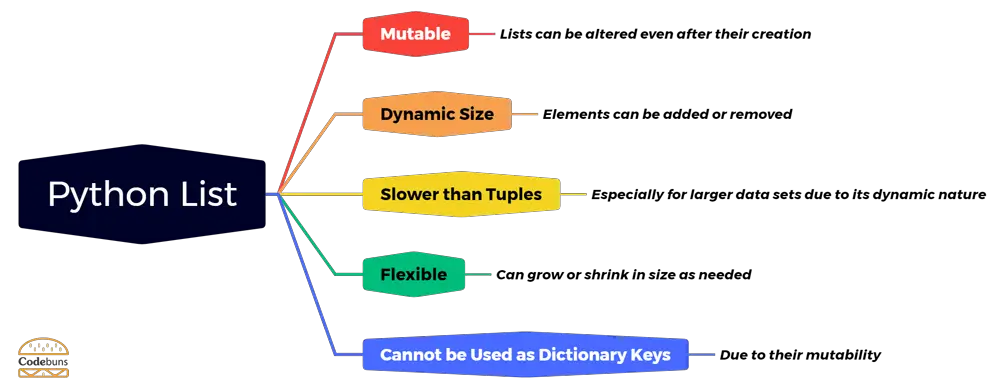
Key Points
- To create a list, use square brackets [] and separate the elements with commas.
- To access an element of a list, use the [] operator and specify the index of the element. The first element in a list has index 0, and so on.
- To add an element to a list, use the append() method.
- To remove an element from a list, use the remove() method.
- To iterate over a list, use a for loop.
Syntax
# Create a list
list_name = [element1, element2, ..., elementN]
# Access elements of a list
list_name[index]
# Add an element to a list
list_name.append(element)
# Remove an element from a list
list_name.remove(element)
# Iterate over a list
for element in list_name:
# Do something with the element
Syntax Explanation
- Creating a List:
- list_name is the name of the list.
- element1, element2, …, elementN are the elements of the list.
- Accessing Elements of a List:
- list_name is the name of the list.
- []: Square brackets are used for indexing.
- index is the index of the element you want to access. Python indexing starts from 0 for the first element.
- Adding an Element to a List:
- list_name is the name of the list.
- element is the element you want to add to the list.
- .append(element): The append() method is used to add element to the end of the list.
- Removing an Element from a List:
- list_name is the name of the list.
- element is the element you want to add to the list.
- .remove(element): The remove() method removes the first occurrence of element from the list. If the element doesn’t exist, it raises a ValueError.
- Iterating Over a List:
- for: This initiates the loop to iterate through each element of the list.
- element: This is a temporary variable assigned to each item in the list as the loop progresses.
- in: This keyword indicates that the for loop will iterate over the elements of the list.
- list_name: Name of the list to iterate over.
Example
# Create a list
my_list = [1, 2, 3]
# Access elements of the list
print(my_list[0]) # Prints 1
print(my_list[1]) # Prints 2
print(my_list[2]) # Prints 3
# my_list[5] # This will raise an IndexError because index does not exist
# Add an element to the list
my_list.append(4)
# Remove an element from a list
my_list.remove(2)
# Print the list
print(my_list) # Prints [1, 3, 4]
# Iterate over the tuple
for element in my_list:
print(element)
Output
1
3
4
Note: If you try to access an element of a list that does not exist, you will get an IndexError.
When to use List in Python
- When we require a collection that might need altering (add, remove, or modify items).
- When working with a collection that will frequently change in size.
- For operations that have built-in support in lists, like append, remove, and insert.
Conclusion
Tuple in Python is a powerful data structure that can be used to store and manipulate data. They are immutable, meaning that their elements cannot be changed once they are created. This makes them ideal for storing data that needs to be protected from accidental changes. Tuples are also faster and more efficient than lists, making them a good choice for applications where performance is essential.
Python Reference
FAQ
What is a tuple in Python?
A tuple is an immutable ordered sequence of elements. It is similar to a list, but tuples cannot be modified once they are created. Tuples are created using parentheses, and elements can be of any data type.
How do you create a tuple in Python?
To create a tuple in Python, you simply list the elements inside a pair of parentheses. For example, the following code creates a tuple with two elements:my_tuple = (1, "Hello")
You can also create a tuple with a single element, but you must include a comma after the element to indicate that it is a tuple. For example, the following code creates a tuple with a single element:my_tuple = (1,)
What are the benefits of using tuples in Python?
Tuples are immutable, which means that they cannot be modified once they are created. This makes them useful for storing data that needs to be protected from accidental modification. Additionally, tuples are more efficient than lists in terms of memory usage.
How do you access elements of a tuple in Python?
To access elements of a tuple in Python, you use the same square bracket notation [ ] that you use to access elements of a list. For example, the following code accesses the first element of the my_tuple tuple:first_element = my_tuple[0]
You can also use negative indexes to access elements from the end of a tuple. For example, the following code accesses the last element of the my_tuple tuple:last_element = my_tuple[-1]
How do you iterate over a tuple in Python?
To iterate over a tuple in Python, you can use a for loop. For example, the following code iterates over the my_tuple tuple and prints each element to the console:for element in my_tuple:
print(element)