Table of Contents | |
What is Set in Python?
Python set is an unordered collection of unique elements. This means that the order of the elements does not matter, there are no indexes, and duplicate elements are removed. Sets are mutable, meaning that elements can be added and removed, but the elements themselves must be immutable. Sets are particularly useful when we want to store unique items.
Python Set Syntax
The basic syntax for creating a set in Python is using curly braces { } or the built-in set() function.
- Using curly braces ({ }):
- {element1, element2, …, elementN}
- element1, element2, …, elementN: The elements of the set.
- Using the built-in set() function:
- set(iterable)
- iterable: Any iterable object, such as a list, tuple, string, or dictionary.
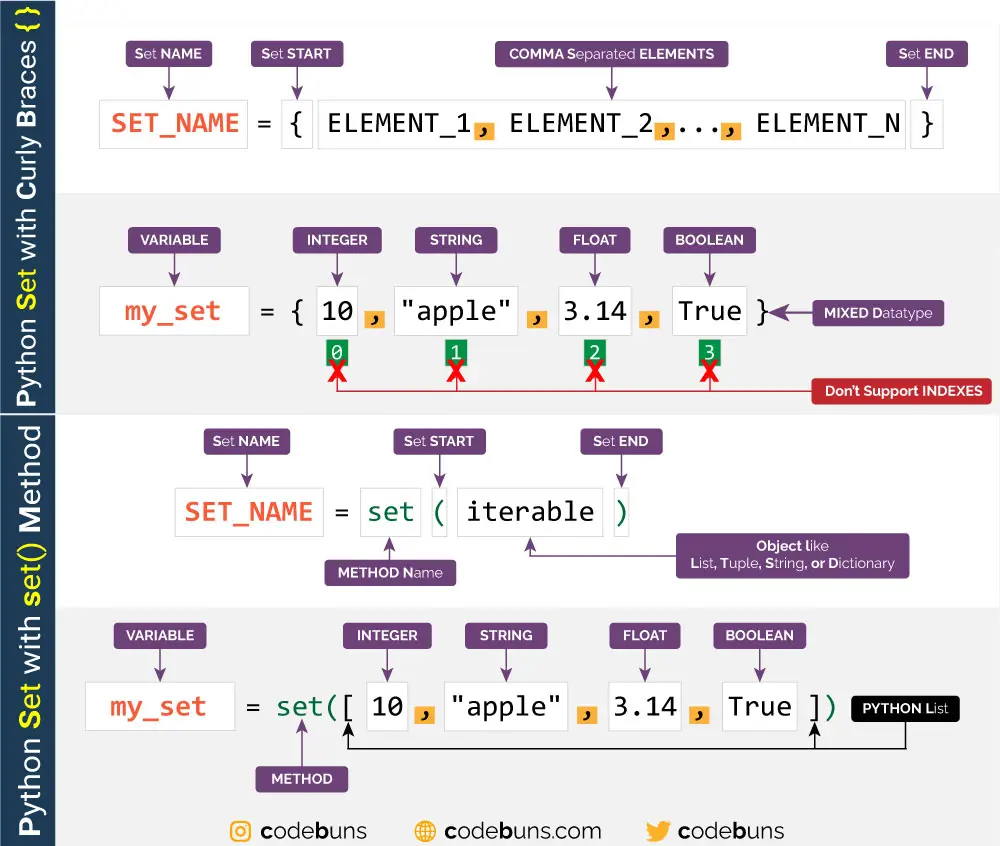
Example of Python Set
# Create a set from curly braces
my_set = {10, "Apple", 3.14, True}
# Create a set from a list
my_list_set = set([10, "Apple", 3.14, True])
# Create a set from a tuple
my_tuple_set = set((10, "Apple", 3.14, True))
# Create a set from a string
my_string_set = set("Apple")
# Create a set from a dictionary
my_dict = {1:10, 2:"Apple", 3:3.14, 4:True}
my_dict_keys_set = set(my_dict.keys())
# Print the sets
print(my_set)
print(my_list_set)
print(my_tuple_set)
print(my_string_set)
print(my_dict_keys_set)
Output
{3.14, 10, True, ‘Apple’}
{3.14, 10, True, ‘Apple’}
{3.14, 10, True, ‘Apple’}
{‘l’, ‘e’, ‘p’, ‘A’}
{3.14, 10, True, ‘Apple’}
Explanation
{ }
– The curly braces are used to create a set from multiple elements.set(...)
– The built-in function creates a new set object.
Note: Sets are often used to eliminate duplicate values from a list or tuple, or to perform mathematical set operations like union, intersection, and difference.
Characteristics of Python Set
Python Set is a powerful data structure that can be used for various tasks, such as removing duplicate elements from a list, performing set operations, and creating unique identifiers. Python set is characterized by the following properties:
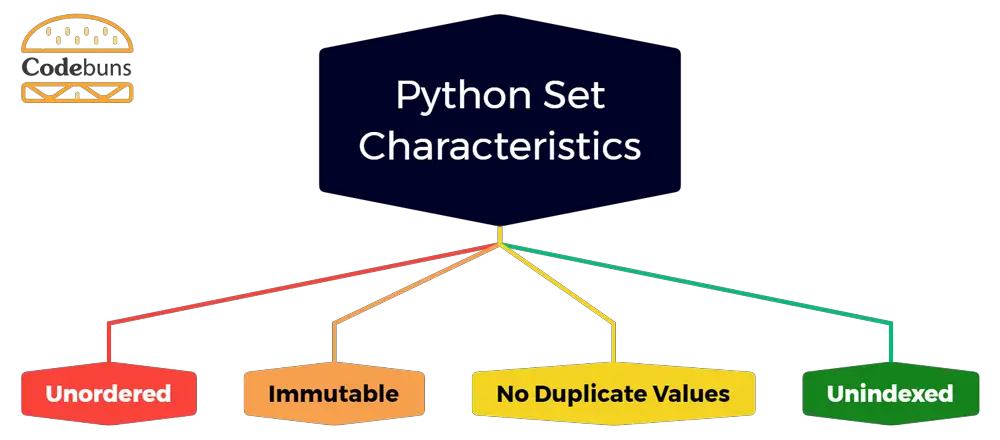
Unordered
- Explanation: Sets are unordered. This means that the elements of a set are not stored in any particular order.
- Example:
sample_set = {3, 1, 2}
print(sample_set) # Output {1, 2, 3}
- Even if we add items in the sequence 3, 1, 2, there’s no guarantee 3 will be the first element when accessing the set.
Immutable
- Explanation: The elements of a set can be changed (mutable), but the set itself cannot be changed. This means that we can add or remove elements from a set, but we cannot change the order of the elements or the elements themselves (immutable).
- Example:
immutable_example = {1, 2, 3}
# immutable_example[0] = 4 # This will raise an error
- We can add an integer or string, but not a list.
- Add using:
immutable_example.add(4)
- Remove using:
immutable_example.remove(3)
No Duplicate Values
- Explanation: A set can only contain unique elements. Duplicate elements are removed when a set is created.
- Example:
duplicate_set = {1, 2, 2, 3, 3}
print(duplicate_set)
- duplicate_set will only store {1, 2, 3}
Unindexed
- Explanation: We cannot access the elements of a set by using an index or key. Instead, we must iterate over the set to access the elements.
- Example:
sample_set[0] will raise an error.
Creating a Set in Python
There are two main ways to create Python set:
- Using set() Function
- Using Curly Braces
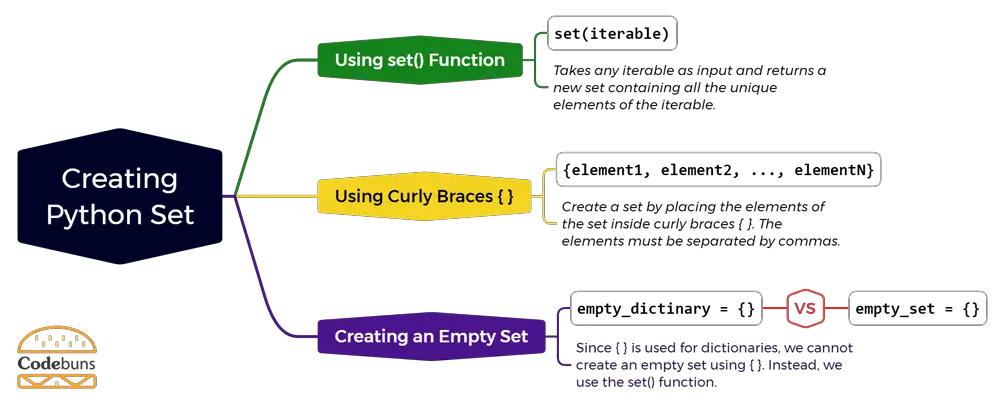
Using set()
Function
The set() function takes any iterable as input and returns a new set containing all the unique elements of the iterable. The iterable can be any sequence of elements, such as a list, tuple, string, or any other iterable object.
Example
# Create an empty set
my_empty_set = set()
# Create a set from a list
my_list = [1, 2, 3, 4, 5]
my_set = set(my_list)
# Create a set from a tuple
my_tuple = (1, 2, 3, 4, 5)
my_set = set(my_tuple)
# Create a set from a string
my_string = "Hello, world!"
my_set = set(my_string)
This is the preferred way to create an empty set in Python because it is more explicit and less likely to lead to errors.
The set() function will also remove duplicate elements from the iterable. For example, the following code creates a set containing the numbers 1, 2, and 3, even though the list passed to the set() function contains the number 3 twice:
my_set = set([1, 2, 3, 3])
Using Curly Braces {}
We can also create a set by placing the elements of the set inside curly braces ({}). The elements must be separated by commas.
For example, the following code creates the same set as the previous example:
Example
my_set = {1, 2, 3, 4, 5}
Note that when creating a set using curly braces, duplicate elements will be automatically removed.
# Create a set with duplicate elements
my_set = {1, 2, 3, 4, 5, 1, 2, 3}
# Print the set
print(my_set)
#Output: {1, 2, 3, 4, 5}
As we can see, the duplicate items have been removed from the set.
We can also use a combination of the two methods to create a set. For example, the following code creates a set containing the numbers 1, 2, 3, and 4 and the string “hello“:
my_set = set({1, 2, 3, 4, "hello"})
Creating an Empty Set
This can be a source of confusion for new Python programmers. In Python, both dictionaries and sets use braces ({}) as their delimiters. However, there’s a key difference in how we declare an empty set versus an empty dictionary:
Empty Dictionary
empty_dict = {}
This creates an empty dictionary. We can confirm its type using the type() function:
print(type(empty_dict)) # Output:
Empty Set
Since {} is used for dictionaries, we cannot create an empty set using {}. Instead, we use the set() function.
empty_set = set()
Confirming its type:
print(type(empty_set)) # Output:
To avoid this confusion, it is best to use the set() function to create empty sets and the dict() function to create empty dictionaries.
Adding Elements in a Python Set
One of the fundamental operations is the addition of elements. Python offers multiple methods for this task, ensuring flexibility and convenience.
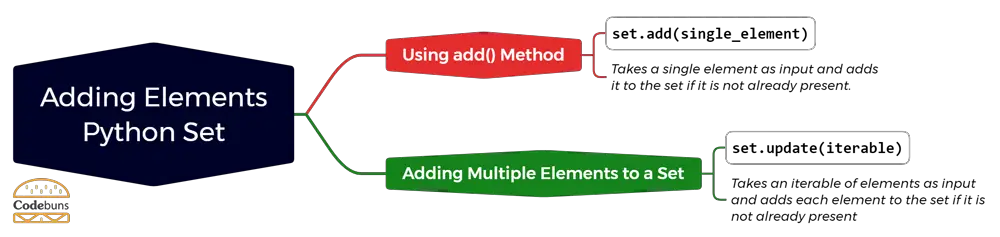
Using add()
Method
To add elements to a Python set, we can use the add() method. The add() method takes a single element as input and adds it to the set if it is not already present.
Syntax
set.add(single_element)
single_element: The element we want to add.
The set.add(element) operation adds the element element to the set set. If the element is already present in the set, the operation does nothing.
Example
colors = set()
# Add an element to the set
colors.add('green')
# Print the set
print(colors)
#Output:{'green'}
Explanation
set(...)
: The built-in function that creates a new empty set.colors.add(1)
: Adds the element ‘green’ to the set colors.print(colors)
: Prints the set colors to the console.
While we can’t modify an individual element in a set (since they’re immutable), we can update the contents of a set by adding or removing elements.
Adding Multiple Elements to a Set
To add multiple elements to a set, we can use the update() method. The update() method takes an iterable of elements as input and adds each element to the set if it is not already present.
Syntax
set.update(iterable)
iterable: An iterable of elements to add to the set.
Example
colors = set()
# Add multiple elements to the set
colors.update(['red', 'blue', 'green'])
# Print the set
print(colors)
#Output:{'red', 'blue', 'green'}
Explanation
colors = set()
: Creates a new empty set.colors.update(['red', 'blue', 'green'])
: Adds the elements ‘red’, ‘blue’, and ‘green’ to the set.print(colors)
: Prints the set.
Updating Elements in a Python Set
There’s no direct method to update an element in a set due to its unordered nature. But one can remove an element and then add a new one.
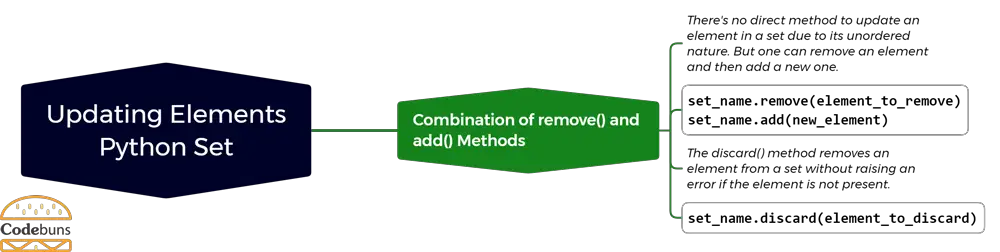
Combination of remove()
and add()
Methods
Syntax
set_name.remove(element_to_remove)
set_name.add(new_element)
Explanation
- set_name is the name of the set.
- element_to_remove: The element we want to remove from the set.
- new_element: The new element we want to add to the set.
Example
colors = {'red', 'blue', 'green'}
colors.remove('red')
colors.add('yellow')
print(colors)
#Output:{'blue', 'green', 'yellow'}
Explanation
colors = {'red', 'blue', 'green'}
: Creates a set with the elements red, blue, and green.colors.remove('red')
: Removes the element red from the set colors.colors.add('yellow')
: Adds the element yellow to the set colors.print(colors)
: Prints the set colors to the console.
To add multiple elements (from lists, sets, or other iterable data types) to a set, use the update() method.
An alternative way to remove an element from a set, but it doesn’t raise an error if the element doesn’t exist
Syntax
set_name.discard(element_to_discard)
Explanation
- set_name is the name of the set.
- element_to_discard: The item we wish to remove.
Example
numbers = {1, 2, 3, 4}
numbers.discard(5) # No error even if 5 doesn't exist in the set
numbers.discard(3)
print(numbers)
#Output:{1, 2, 4}
Explanation
numbers = {1, 2, 3, 4}
: Creates a set with the elements 1, 2, 3, and 4.numbers.discard(5)
: Removes the element 5 from the set numbers. Since 5 is not present in the set, the method does nothing.numbers.discard(3)
: Removes the element 3 from the set numbers.print(numbers)
: Prints the set numbers to the console.
Finding an Element in a Python Set
Finding an element in a Python set is a very simple operation. The in operator can be used to check if an element is present in a set. This operation is very efficient and can be used to quickly find elements in a set.
Checking if an Element Exists in a Python Set(in
and not in
Operator)
In Python, the in and not in operators can be used to check if an element exists in a set. The in operator returns True if the element is present in the set and False if it is not present. The not in operator returns the opposite of the in operator, meaning that it returns True if the element is not present in the set and False if it is present.
Syntax
element_in_set = element in set
Explanation
- element: The element to check if it is present in the set.
- set: The set to check the element against.
- element_in_set: A boolean value indicating whether the element is present in the set.
Example
set = {1, 2, 3, 4, 5}
element = 2
# Check if the element 2 is present in the set
element_in_set = element in set
# Print the result
print(element_in_set)
# Output: True
Explanation
set = {1, 2, 3, 4, 5}
: Create a set with the elements 1, 2, 3, 4, and 5.element = 2
: Define the element to check if it is present in the set.element_in_set = element in set
: Check if the element is present in the set and assign the result to the variableelement_in_set
.print(element_in_set)
: Print the result of the in operator to the console.
Deleting Elements from a Python Set
There are two ways to delete elements from a Python set:
- Using the remove() Method.
- Using the discard() Method.
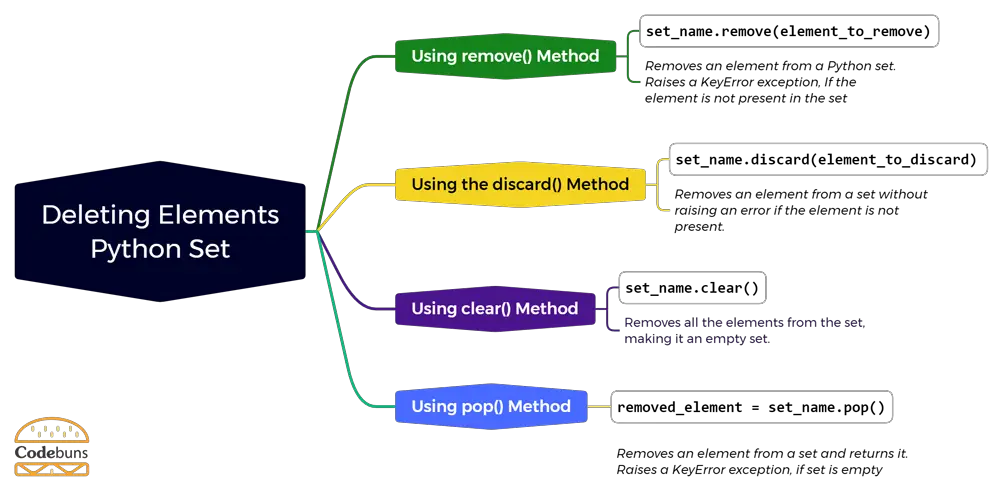
Using remove()
Method
The remove() method removes an element from a Python set. If the element is not present in the set, the remove() method raises a KeyError exception.
Syntax
set_name.remove(element_to_remove)
Explanation
- set_name: The set from which we want to delete.
- element_to_remove: The specific element to delete.
- Note: Raises a KeyError if the element doesn’t exist.
Example
colors = {'red', 'blue', 'green'}
colors.remove('blue')
print(colors)
# Output: {'green', 'red'}
Using discard()
Method
The discard() method removes an element from a set if it is present in the set. Otherwise, it does nothing.
Syntax
set_name.discard(element_to_discard)
Explanation
- set_name is the name of the set.
- element_to_discard: The item we want to remove.
Example
colors = {'red', 'blue', 'green'}
colors.discard('black') # No error if 'black' doesn't exist
colors.discard('blue')
print(colors)
# Output: {'green', 'red'}
In addition to the two main ways, there are other ways to delete elements of Python list, such as:
Using clear()
Method
The clear() method removes all the elements from the set, making it an empty set.
Syntax
set_name.clear()
Example
colors = {'red', 'blue', 'green'}
colors.clear()
print(colors)
# Output: {}
Using pop()
Method
The pop() method removes an element from a set and returns it. If the set is empty, the pop() method raises a KeyError exception. This method is useful for removing a single element from a set without knowing its value.
Syntax
removed_element = set_name.pop()
Note: Since sets are unordered, the popped element is unpredictable.
Example
colors = {'red', 'blue', 'yellow'}
popped_color = colors.pop()
print(popped_color)
# Output: blue
Remove Duplicates from a Python Set
The set() constructor is a simple and efficient way to remove duplicates from a Python set. The constructor creates a new set from the original set, removing all duplicates.
Syntax
new_set = set(original_set)
Explanation
- original_set: The set to remove duplicates from.
- new_set: The new set without duplicates.
Example
# Create a set with duplicate elements
original_set = {1, 2, 3, 1, 4, 2}
# Remove duplicates from the set
new_set = set(original_set)
print(new_set)
# Output: {1, 2, 3, 4}
Explanation
original_set = {1, 2, 3, 1, 4, 2}
: Creates a set with the elements 1, 2, 3, 1, 4, and 2.new_set = set(original_set)
: Creates a new set from the original set, removing all duplicates.print(new_set)
: Prints the new set to the console.
Iterating Over a Python Set
Iterating over a Python set is a simple and efficient way to access each element of the set. The for loop is the most common way to iterate over a set.
Syntax
for element in set:
# Do something with the element
Explanation
- set: The set to iterate over.
- element: The element in the set.
Example
my_set = {1, 2, 3, 4}
for element in my_set:
print(element)
Output
1
2
3
4
Explanation
my_set = {1, 2, 3, 4}
: Creates a set with the elements 1, 2, 3, and 4.for element in my_set
: Iterates over the set my_set and assigns each element to the variable element.print(element)
: Prints element to the console.
How to Get the Length of a Python Set
Determining the length or the number of elements in a set is a routine task in Python programming. Thankfully, Python makes it straightforward with the use of a built-in function len().
Syntax
length = len(set)
Explanation
- set: The set to get the length of.
- length: The length of the set.
Example
my_set = {1, 2, 3, 4}
# Get the length of the set
length = len(my_set)
print(length)
# Output: 4
Explanation
my_set = {1, 2, 3, 4}
: Creates a set with the elements 1, 2, 3, and 4.length = len(my_set)
: Gets the length of the set my_set and assigns it to the variable length.print(length)
: Prints the length of the set to the console.
Python Frozenset
A frozenset is a set that is immutable, meaning its elements cannot be modified after creation. This distinct feature ensures that frozensets can be used as keys in dictionaries, a task regular sets cannot perform due to their mutable nature.
Syntax
frozen_set = frozenset(iterable)
Explanation
- iterable: Any iterable object, such as a list, tuple, or set.
- frozen_set: The new frozenset object.
Example
# Create a frozenset from a list
my_list = [1, 2, 3, 4]
frozen_set = frozenset(my_list)
# Try to add an element to the frozenset
frozen_set.add(5)
# This will raise a TypeError exception because frozensets are immutable
Explanation
my_list = [1, 2, 3, 4]
Creates a list with the elements 1, 2, 3, and 4.frozen_set = frozenset(my_list)
Creates a new frozenset object from the list my_list.frozen_set.add(5)
Tries to add the element 5 to the frozenset frozen_set.
This will raise a TypeError exception because frozensets are immutable and cannot be modified.
Operations on Python Set
Set operations are operations that can be performed on two or more sets. Some of the most common set operations include union, intersection, difference, and symmetric difference.
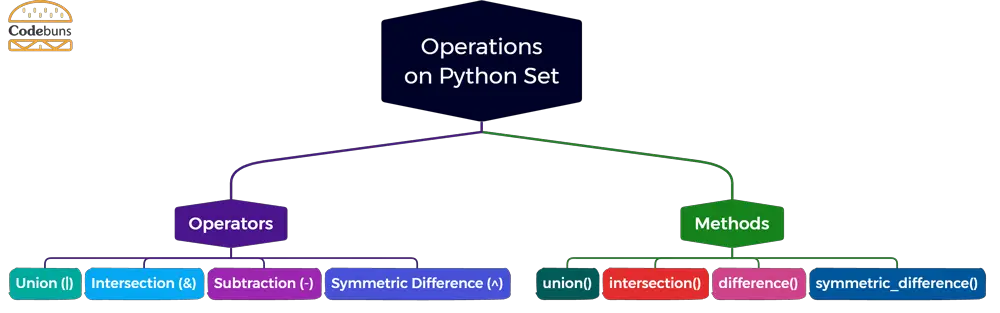
Using Union (|
) Operator
The union operator (|) is a simple and efficient way to combine two sets into a new set that contains all the elements of both sets. The union operator is a binary operator, which means that it takes two sets as input and returns a new set as output. The union operator removes duplicate elements from the new set.
Example
# Create two sets
set1 = {1, 2, 3}
set2 = {4, 5, 6}
# Combine the two sets using the union operator
new_set = set1 | set2
print(new_set) # {1, 2, 3, 4, 5, 6}
Explanation
set1 = {1, 2, 3}
: Creates a set with the elements 1, 2, and 3.set2 = {4, 5, 6}
: Creates a set with the elements 4, 5, and 6.new_set = set1 | set2
: Combines the two sets set1 and set2 using the union operator and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
Using union()
Method
The union() method in Python is used to combine two or more sets into a new set that contains all the elements of all the sets. It is a member of the set class and takes one or more sets as input and returns a new set as output. The union() method removes duplicate elements from the new set.
Example
# Create two sets
set1 = {1, 2, 3}
set2 = {4, 5, 6}
# Combine the two sets using the union() method
new_set = set1.union(set2)
print(new_set) # {1, 2, 3, 4, 5, 6}
Explanation
set1 = {1, 2, 3} and set2 = {4, 5, 6}
: Create two sets with the elements 1, 2, 3, and 4, 5, 6, respectively.new_set = set1.union(set2)
: Combines the two sets set1 and set2 using the union() method and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
Using Intersection (&
) Operator
The intersection operator (&) in Python is used to combine two sets into a new set that contains only the elements that are present in both sets. It is a binary operator, which means that it takes two sets as input and returns a new set as output.
Example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Find the intersection of the two sets using the intersection operator
new_set = set1 & set2
# Print the new set
print(new_set)
# Output: {4, 5}
Explanation
set1 = {1, 2, 3, 4, 5}
andset2 = {4, 5, 6, 7, 8}
: Create two sets with the elements 1, 2, 3, 4, 5 and 4, 5, 6, 7, 8, respectively.new_set = set1 & set2
: Combines the two sets set1 and set2 using the intersection operator and assigns the result to the variable new_set.print(new_set
): Prints the variable new_set to the console.
Using intersection()
Method
The intersection() method in Python is used to combine two or more sets into a new set that contains only the elements that are present in all of the sets. It is a member of the set class and takes one or more sets as input and returns a new set as output.
Example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Find the intersection of the two sets using the intersection() method
new_set = set1.intersection(set2)
# Print the new set
print(new_set)
# Output: {4, 5}
Explanation
set1 = {1, 2, 3, 4, 5}
andset2 = {4, 5, 6, 7, 8}
: Create two sets with the elements 1, 2, 3, 4, 5 and 4, 5, 6, 7, 8, respectively.new_set = set1.intersection(set2)
: Combines the two sets set1 and set2 using the intersection() method and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
Using Subtraction (-
) Operator
The subtraction operator (-) in Python is used to combine two sets into a new set that contains all the elements of the first set that are not present in the second set. It is a binary operator, which means that it takes two sets as input and returns a new set as output.
Example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Subtract the second set from the first set using the subtraction operator
new_set = set1 - set2
# Print the new set
print(new_set)
# Output: {1, 2, 3}
Explanation
set1 = {1, 2, 3, 4, 5}
andset2 = {4, 5, 6, 7, 8}
: Create two sets with the elements 1, 2, 3, 4, 5 and 4, 5, 6, 7, 8, respectively.new_set = set1 - set2
: Subtracts the second set set2 from the first set set1 and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
The subtraction operator can also be used to subtract elements from a list or a set. For example, the following code subtracts the element 2 from the list list1:
list1 = [1, 2, 3, 4, 5]
# Subtract the element 2 from the list list1 using the subtraction operator
list1.remove(2)
# Print the list list1
print(list1)
# Output: [1, 3, 4, 5]
The subtraction operator can also be used to negate a number. For example, the following code negates the number 5:
x = 5
# Negate the number 5 using the subtraction operator
x = -x
# Print the number x
print(x)
# Output: -5
Using difference()
Method
The difference() method in Python is used to combine two or more sets into a new set that contains all the elements of the first set that are not present in any of the other sets. It is a member of the set class and takes one or more sets as input and returns a new set as output.
Example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Subtract the second set from the first set using the difference() method
new_set = set1.difference(set2)
# Print the new set
print(new_set)
# Output: {1, 2, 3}
Explanation
set1 = {1, 2, 3, 4, 5}
andset2 = {4, 5, 6, 7, 8}
: Create two sets with the elements 1, 2, 3, 4, 5 and 4, 5, 6, 7, 8, respectively.new_set = set1.difference(set2)
: Subtracts the second set set2 from the first set set1 and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
The difference() method can also be used to subtract elements from a list or a set. For example, the following code subtracts the element 2 from the list list1:
list1 = [1, 2, 3, 4, 5]
# Subtract the element 2 from the list list1 using the difference() method
list1.remove(2)
# Print the list list1
print(list1)
# Output: [1, 3, 4, 5]
The difference() method can also be used to find the unique elements in a set. For example, the following code finds the unique elements in the set set1:
set1 = {1, 2, 3, 4, 5, 5, 6}
# Find the unique elements in the set set1 using the difference() method
unique_elements = set1.difference(set1.intersection({5, 6}))
# Print the unique elements
print(unique_elements)
# Output: {1, 2, 3, 4}
Using Symmetric Difference (^
) Operator
The symmetric difference operator (^) in Python is used to combine two sets into a new set that contains all the elements that are present in either set but not in both sets. It is a binary operator, which means that it takes two sets as input and returns a new set as output.
Example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Find the symmetric difference of the two sets using the symmetric difference operator
new_set = set1 ^ set2
# Print the new set
print(new_set)
# Output: {1, 2, 3, 6, 7, 8}
Explanation
set1 = {1, 2, 3, 4, 5}
andset2 = {4, 5, 6, 7, 8}
: Create two sets with the elements 1, 2, 3, 4, 5 and 4, 5, 6, 7, 8, respectively.new_set = set1 ^ set2
: Combines the two sets set1 and set2 using the symmetric difference operator and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
Using symmetric_difference()
Method
The symmetric_difference() method in Python is used to combine two or more sets into a new set that contains all the elements that are present in either set but not in both sets. It is a member of the set class and takes one or more sets as input and returns a new set as output.
Example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Find the symmetric difference of the two sets using the symmetric_difference() method
new_set = set1.symmetric_difference(set2)
# Print the new set
print(new_set)
# Output: {1, 2, 3, 6, 7, 8}
Explanation
set1 = {1, 2, 3, 4, 5}
andset2 = {4, 5, 6, 7, 8}
: Create two sets with the elements 1, 2, 3, 4, 5 and 4, 5, 6, 7, 8, respectively.new_set = set1.symmetric_difference(set2)
: Combines the two sets set1 and set2 using the symmetric_difference() method and assigns the result to the variable new_set.print(new_set)
: Prints the variable new_set to the console.
Note: The symmetric_difference() method is only available for sets in Python.
Conclusion
Python Set is a powerful and versatile data structure in Python. It allows for the storage of unique elements and provides efficient operations like adding, removing, and checking for membership. With its simplicity and flexibility, Set in Python proves to be a valuable tool for various programming tasks. Whether we need to eliminate duplicates from a list or perform set operations like union, intersection, or difference, Python Set offers an effective solution. By understanding and utilizing the capabilities of Python Set, programmers can enhance their code efficiency and achieve better results in their projects.
Python Reference
FAQ
What is a Python set?
A Python set is a collection of unique elements. The elements in a set can be of any type, such as numbers, strings, or even other sets. Sets are unordered, meaning that the order of the elements in a set does not matter.
How do you create a Python set?
There are two ways to create a Python set: using the set() function or using curly braces ({ }). The set() function takes an iterable as input and returns a new set containing all of the unique elements of the iterable. Curly braces can be used to create a new set from a list of elements.
How do you add or remove elements from a Python set?
To add an element to a set, use the add() method. To remove an element from a set, use the remove() method.
How do you check if an element is in a Python set?
To check if an element is in a set, use the in operator.
How do you combine two Python sets?
There are two ways to combine two Python sets: using the union() method or using the | operator. The union() method takes two sets as input and returns a new set containing all of the unique elements of the two sets. The | operator can be used to combine two sets in a similar way to the union() method.
How does a Set in Python differ from a List or Tuple?
The primary differences lie in their properties and functionalities. Lists are ordered, allow duplicate values, and are mutable. Tuples are ordered and allow duplicates but are immutable. Sets, on the other hand, are unordered, mutable, and ensure all items are unique, making them perfect for tasks where item uniqueness is important.