Python Operators are essential components that allow us to perform various operations on data. They enable us to manipulate and combine values, making our code more dynamic and functional. By understanding how operators work in Python, we can effectively perform tasks such as arithmetic calculations, logical comparisons, and string manipulations. This article will explore different types of operators available in Python and how they can be used to enhance our code.
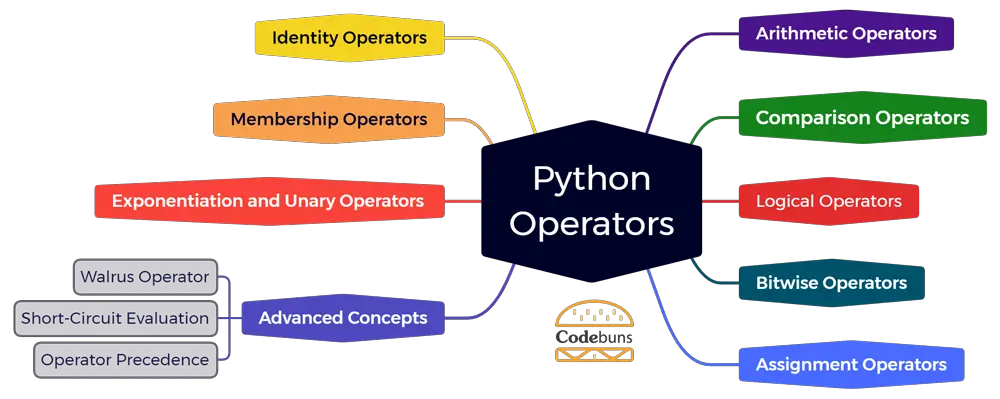
Table of Contents | |
Python Operators
Python Operators are special built-in symbols that represent specific operations to be performed on one or more operands. They are an essential part of the Python language, used to manipulate data and perform calculations. Python offers various operators, including arithmetic, assignment, comparison, logical, and identity operators. Arithmetic operators are used for basic mathematical operations such as addition, subtraction, multiplication, and division. Assignment operators assign values to variables. Comparison operators compare values and return either True or False based on the comparison result. Logical operators combine multiple conditions using logical operations such as AND, OR, and NOT. Identity operators check if two objects have the same identity or not. Other commonly used Python operators include membership and bitwise operators.
When using arithmetic operators in Python, it’s important to remember that certain operators have precedence over others. For example, the multiplication (*) and division (/) operators take precedence over addition (+) and subtraction (–). This means that expressions are evaluated based on these rules unless specified otherwise using parentheses.
Furthermore, Python offers the flexibility to define custom operator behavior through operator overloading. This powerful feature allows developers to redefine how standard built-in operators behave when applied to user-defined objects or classes. By leveraging this capability, developers can create custom data types with intuitive mathematical operations tailored to specific application requirements. Understanding how these different types of Python operators work together provides an essential foundation for writing efficient code in Python.
Examples of Python Operators
# Arithmetic operators a = 10 b = 4 c = 2 print(a + b) # Addition print(a - b) # Subtraction print(a * b) # Multiplication print(a / b) # Division print(a // b) # Floor division print(a % b) # Modulus print(a ** b) # Exponentiation # Assignment operators a += 5 # Add and assign b -= 2 # Subtract and assign c *= 3 # Multiply and assign a /= 2 # Divide and assign c **= 2 # Exponentiate and assign # Comparison operators print(a == b) # Equality print(a != b) # Inequality print(a > b) # Greater than print(a < b) # Less than print(a >= b) # Greater than or equal to print(a <= b) # Less than or equal to # Logical operators print(True and False) # AND print(True or False) # OR print(not True) # NOT # Identity operators x = [1, 2, 3] y = x # Both variables refer to the same object z = [1, 2, 3] # Different object with the same values print(x is y) # True, both refer to the same object print(x is not z) # True, different objects # Bitwise operators print(a & b) # Bitwise AND print(a | b) # Bitwise OR print(a ^ b) # Bitwise XOR print(~a) # Bitwise NOT print(a << 1) # Left shift print(a >> 1) # Right shift # Membership operators print(2 in x) # True, 2 is in the list print(5 not in x) # True, 5 is not in the list
Understanding Operands in Python
Operands in Python are the values or variables that operators act upon to produce results. These operands can be numbers, strings, objects, variables, constants, or even expressions that evaluate to a single value. Considering the expression a + b = c, a and b are the operands, and + is the operator. By understanding how operands work in Python, developers can manipulate data and perform various operations such as arithmetic calculations, string concatenation, and comparison operations.
Understanding Expressions in Python
Python Expressions are a combination of values, variables, operators, and function calls that result in a new value. They can be as simple as adding numbers or as complex as manipulating strings or performing mathematical calculations. Expressions allow you to perform various operations, from basic arithmetic calculations to more advanced manipulations of values, variables, operators, and function calls. You can use Python expressions to solve mathematical problems, concatenate strings, compare values using logical operators, etc. The flexibility and versatility of Python expressions make it a powerful tool for data manipulation and problem-solving.
Examples of Python Expressions
- Arithmetic: 2 + 3 * 5 evaluates to 17.
- String: ‘Hello ‘ + ‘World!’ evaluates to ‘Hello World!’.
- Logical: True and False evaluates to False.
- Function Call: len(‘Python’) evaluates to 6 (the length of the string ‘Python’).
Explanation of Arithmetic Expression
- 3 * 4 is a multiplication operation.
- + 5 is an addition operation.
- The entire expression 3 * 4 + 5 is first evaluated as 12 + 5 (since multiplication takes precedence over addition).
- Finally, result 17 is stored in the variable result.
Here are some key points about expressions in Python:
Basic Elements
- Values and Variables: A value can be a number, a string, or any other type of data. Variables are symbols that can store values.
- Operators: These include arithmetic operators like +, –, *, /, logical operators like and, or, not, and comparison operators like ==, !=, <, >.
- Function Calls: An expression can include calls to functions, which return values.
Evaluation
When Python evaluates an expression, it computes the value. This process includes performing calculations, executing functions, and so forth.
Complex Expressions
Complex expressions can be formed by combining simpler expressions using operators and functions. Python follows the PEMDAS rule, which stands for Parentheses, Exponential, Multiplication and Division, and Addition and Subtraction in evaluating these expressions.
Arithmetic Operators in Python
Arithmetic operators are symbols or characters that represent certain mathematical operations such as addition, subtraction, multiplication, division, and more. In Python, these operators follow the same basic rules and syntax as other programming languages, making learning easy for beginners.
However, one unique aspect of Python is its ability to use the floor division operator (//), which returns the integer value of a division rather than the decimal value. This can be useful in certain mathematical operations where only whole numbers are needed.
Another important operator in Python is the modulus operator (%), which returns the remainder when one number is divided by another. This can help determine if a number is even or odd, as an even number divided by 2 will have a remainder of 0.
Apart from the basic arithmetic operators like addition and subtraction, there is also the power operator (**). This operator is handy when you need to raise a number to a certain power, allowing you to calculate exponential values or even find square roots effortlessly.
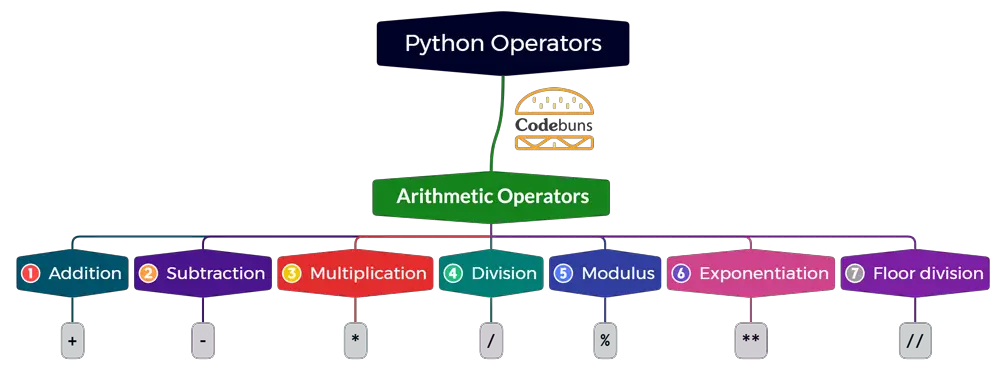
List of Arithmetic Operators in Python:
- Addition (+)
- Subtraction (–)
- Multiplication (*)
- Division (/)
- Modulus (%)
- Exponentiation Operator: Power (**)
- Floor division (//)
Addition Operator (+)
- Syntax: operand1 + operand2
- Adds operand1 and operand2.
Subtraction Operator (-)
- Syntax: operand1 – operand2
- Subtracts operand2 from operand1.
Multiplication Operator (*)
- Syntax: operand1 * operand2
- Multiplies operand1 and operand2.
Division Operator: Returns Decimal Value (/)
- Syntax: operand1 / operand2
- Divides operand1 by operand2.
Modulus Operator: Returns Remainder (%)
- Syntax: operand1 % operand2
- Returns the remainder of the division of operand1 by operand2.
Exponentiation Operator: Power (**)
- Syntax: operand1 ** operand2
- Raises operand1 to the power of operand2.
Floor Division Operator: Returns Integer Value (//)
- Syntax: operand1 // operand2
- Divides operand1 by operand2 and returns the integer part of the quotient.
Examples of Arithmetic Operators
Let’s look at a simple example of how these operators work in Python:
# Arithmetic operations in Python a = 10 b = 5 # Addition sum = a + b # Adds a and b print(sum) # Subtraction difference = a - b # Subtracts b from a print(difference) # Multiplication product = a * b # Multiplies a and b print(product) # Division quotient = a / b # Divides a by b print(quotient) # Modulus remainder = a % b # Finds the remainder of a divided by b print(remainder) # Exponentiation power = a ** b # Raises a to the power of b print(power) # Floor Division floor_division = a // b # Performs floor division on a and b print(floor_division)
Explanation
- a = 10 and b = 5 assign the values 10 and 5 to variables a and b, respectively, for use in calculations.
- Addition: sum = a + b # Calculates the sum of a and b. The result is 15.
- Subtraction: difference = a – b # Subtracts b from a. The result is 5.
- Multiplication: product = a * b # Multiplies a and b. The result is 50.
- Division: quotient = a / b # Divides a by b. The result is 2.
- Modulus: remainder = a % b # Finds the remainder of a divided by b. The result is 0.
- Exponentiation: power = a ** b # Raises a to the power of b. The result is 100000.
- Floor Division: floor_division = a // b # Divides a by b and rounds down to the nearest whole number. The result is 2.
Comparison Operators in Python
Comparison operators in Python are used to compare two values and determine the relationship between them. They return a Boolean True or False value based on the comparison result. These operators allow us to check for equality, inequality, greater than, less than, greater than or equal to, and less than or equal to condition. These operators are used extensively in conditional statements, such as if-else, while loops, and for loops.
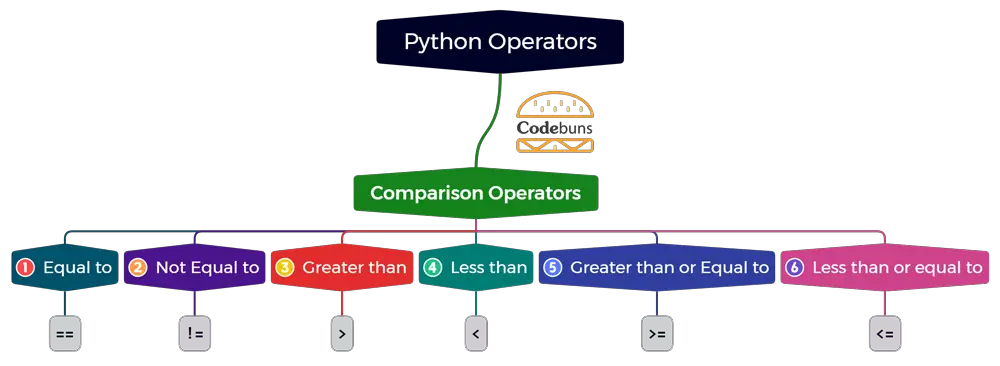
List of Comparison Operators in Python:
- Equal to (==)
- Not Equal to (!=)
- Greater than (>)
- Less than (<)
- Greater than or Equal to (>=)
- Less than or equal to (<=)
Equal Operator (==)
- Syntax: operand1 == operand2
- Operator checks whether operand1 is equal to operand2.
Not Equal Operator (!=)
- Syntax: operand1 != operand2
- Operator checks whether operand1 is not equal to operand2.
Greater Than Operator (>)
- Syntax: operand1 > operand2
- Operator checks if operand1 is greater than operand2.
Less Than Operator (<)
- Syntax: operand1 < operand2
- Operator checks if operand1 is less than operand2.
Greater Than or Equal To Operator (>=)
- Syntax: operand1 >= operand2
- Operator checks if operand1 is greater than or equal to operand2.
Less Than or Equal To Operator (<=)
- Syntax: operand1 <= operand2
- Operator checks if operand1 is less than or equal to operand2.
Examples of Comparison Operators
# Comparison operators in Python x = 10 y = 20 # Check if x is equal to y is_equal = x == y print(is_equal) # Check if x is not equal to y is_not_equal = x != y print(is_not_equal) # Check if x is greater than y is_greater = x > y print(is_greater) # Check if x is less than y is_less = x < y print(is_less) # Check if x is greater than or equal to y is_greater_or_equal = x >= y print(is_greater_or_equal) # Check if x is less than or equal to y is_less_or_equal = x <= y print(is_less_or_equal)
Explanation
- x = 10 and y = 20 assign the values 10 and 20 to variables x and y, respectively, for comparison.
- Equality: is_equal = x == y # Checks if x is equal to y (False in this case).
- Inequality: is_not_equal = x != y # Checks if x is not equal to y (True here).
- Greater Than: is_greater = x > y # Checks if x is greater than y (False as 10 is not greater than 20).
- Less Than: is_less = x < y # Checks if x is less than y (True since 10 is less than 20).
- Greater Than or Equal To: is_greater_or_equal = x >= y # Checks if x is greater than or equal to y (False in this scenario).
- Less Than or Equal To: is_less_or_equal = x <= y # Checks if x is less than or equal to y (True as 10 is indeed less or equal to 20).
Logical Operators in Python
Python Logical operators are an essential part of programming that allows us to combine multiple conditions and evaluate them as a single expression. There are three logical operators in Python: “and“, “or“, and “not“. These operators allow us to evaluate multiple conditions at once, making our code more efficient and concise. The “and” operator returns True only if both operands are True, otherwise it returns False. The “or” operator returns True if any of the operands are True, otherwise it returns False. Finally, the “not” operator is used to invert a boolean value. In other words, it will return True if the operand is False and vice versa. Logical operators play an important role in control flow statements like if-else and while loops. They help us make decisions based on multiple conditions and control the flow of our code accordingly.
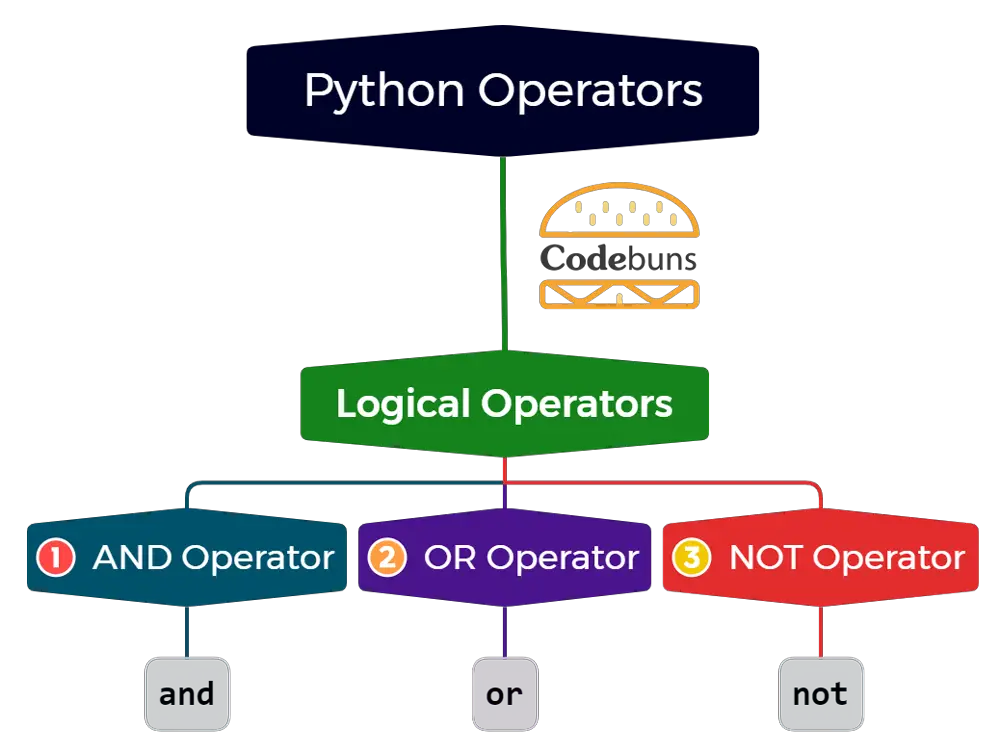
List of Logical Operators in Python:
- Logical AND Operator (and)
- Logical OR Operator (or)
- Logical NOT Operator (not)
The and Operator
- Syntax: operand1 and operand2
- The “and” operator returns True only if both operands are True, otherwise it returns False.
The or Operator
- Syntax: operand1 or operand2
- The “or” operator returns True if any of the operands are True, otherwise it returns False.
The not Operator
- Syntax: not operand
- The “not” operator is used to invert a boolean value and return the opposite.
Examples of Logical Operators
# Logical operators in Python a = True b = False # Using 'and' operator result_and = a and b # Returns False print(result_and) # Using 'or' operator result_or = a or b # Returns True print(result_or) # Using 'not' operator result_not = not a # Returns False print(result_not)
Explanation
- a = True and b = False assign the boolean values True and False to variables a and b, respectively, for logical operations.
- AND (and): result_and = a and b # Returns True only if both a and b are True
- OR (or): result_or = a or b # Returns True if either a or b (or both) are True
- NOT (not): result_not = not a # Reverses the boolean value of a
Bitwise Operators in Python
Python Bitwise operators are used to perform operations at the bit level. These operators work on individual bits of a number, converting them into binary format and then performing logical operations on them. This allows for efficient manipulation of bit patterns, making it useful for tasks such as encryption, compression, and graphics processing. The bitwise operators in Python include AND, OR, XOR, NOT, and left shift and right shift, each with its unique functionality. They are often combined to manipulate and compare bits, providing powerful tools for developers working with binary data.
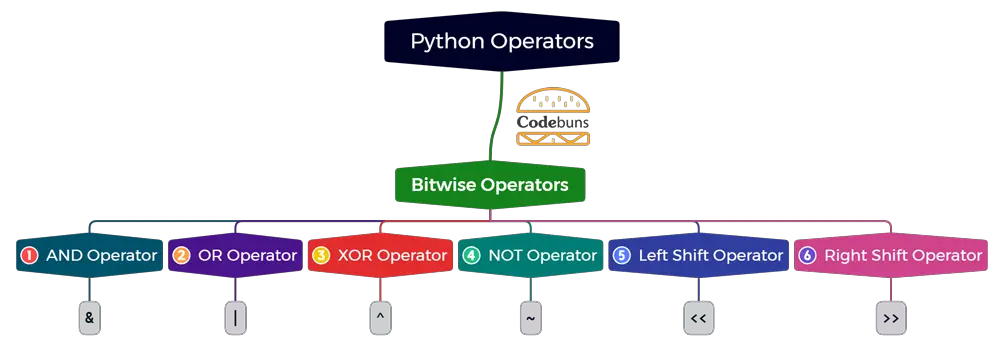
List of Bitwise Operators in Python:
- Binary AND Operator (&)
- Binary OR Operator (|)
- Binary XOR Operator (^)
- Binary NOT Operator (~)
- Binary Left Shift Operator (<<)
- Binary Right Shift Operator (>>)
Bitwise AND Operator (&)
- Syntax: operand1 & operand2
- The NOT operator flips all the bits of a value, returning its complement. This is equivalent to subtracting the value from -1.
Bitwise OR Operator (|)
- Syntax: operand1 | operand2
- The OR operator performs a logical OR operation on two values, returning 1 if either of the bits is 1 and 0 otherwise.
Bitwise XOR Operator (^)
- Syntax: operand1 ^ operand2
- Exclusive OR or XOR operator returns 1 when the bits are different and 0 otherwise.
Bitwise NOT Operator (~)
- Syntax: ~operand
- The NOT operator flips all the bits of a value, returning its complement. This is equivalent to subtracting the value from -1.
Bitwise Left Shift Operator (<<)
- Syntax: operand << shift_amount
- Shifts the bits of operand to the left by shift_amount positions. Vacant positions are filled with zeros.
Bitwise Right Shift Operator (>>)
- Syntax: operand >> shift_amount
- Shifts the bits of operand to the right by shift_amount positions. The behavior for filling vacant positions depends on the sign and implementation.
Examples of Bitwise Operators
# Bitwise operators in Python a = 4 # 0100 in binary b = 5 # 0101 in binary # Bitwise AND and_result = a & b # 0100 print(and_result) # Bitwise OR or_result = a | b # 0101 print(or_result) # Bitwise XOR xor_result = a ^ b # 0001 print(xor_result) # Bitwise NOT not_result = ~a # -0101 print(not_result) # Left Shift left_shift = a << 1 # 1000 print(left_shift) # Right Shift right_shift = a >> 1 # 0010 print(right_shift)
Explanation
- a = 4 and b = 5 assign the values 4 (0100 in binary) and 5 (0101 in binary) to variables a and b, respectively, for bitwise operations.
- AND (&): and_result = a & b # Performs a bitwise AND, resulting in 0100 (4)
- OR (|): or_result = a | b # Performs a bitwise OR, resulting in 0101 (5)
- XOR (^): xor_result = a ^ b # Performs a bitwise XOR, resulting in 0001 (1)
- NOT (~): not_result = ~a # Inverts the bits of a, resulting in -0101 (-5 in two’s complement)
- Left Shift (<<): left_shift = a << 1 # Shifts the bits of a one position to the left, resulting in 1000 (8)
- Right Shift (>>): right_shift = a >> 1 # Shifts the bits of a one position to the right, resulting in 0010 (2)
Assignment Operators in Python
Python Assignment operators are essential for assigning values to variables. They allow us to store data in variables and manipulate them as needed. These operators are essential for performing basic operations such as addition, subtraction, multiplication, and division of variables. The most commonly used assignment operator is the equal sign (=), which assigns the value on the right-hand side of the operator to the variable on the left-hand side.
Python also has special assignment operators such as (:=), which was introduced in version 3.8. These are known as the Walrus Operator, allowing us to assign values to variables within an expression. This can be particularly useful when working with conditional statements or list comprehensions.
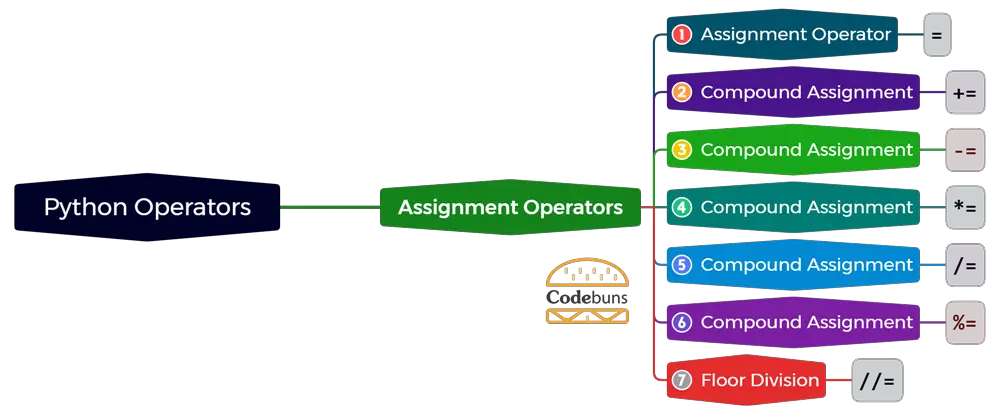
List of Assignment Operators in Python:
- Assignment Operator (=)
- Compound Assignment Operator (+=)
- Compound Assignment Operator (-=)
- Compound Assignment Operator (*=)
- Compound Assignment Operator (/=)
- Compound Assignment Operator (%=)
- Floor division/integer Division Assigned Value to a Variable (//=)
Basic Assignment Operator (=)
- Syntax: variable = value
- Assigns the value on the right to the variable on the left.
Add and Assign Operator (+=)
- Syntax: variable += value
- Adds the right operand to the variable and assigns the result back to the variable.
Subtract and Assign Operator (-=)
- Syntax: variable -= value
- Subtracts the right operand from the variable and assigns the result back to the variable.
Multiply and Assign Operator (*=)
- Syntax: variable *= value
- Multiplies the variable by the right operand and assigns the result back to the variable.
Divide and Assign Operator (/=)
- Syntax: variable /= value
- Divides the variable by the right operand and assigns the result back to the variable.
Modulus and Assign Operator (%=)
- Syntax: variable %= value
- Takes modulus using the variable and the right operand and assigns the result back to the variable.
Exponent and Assign Operator (**=)
- Syntax: variable **= value
- Raises the variable to the power of the right operand and assigns the result back to the variable.
Floor Divide and Assign Operator (//=)
- Syntax: variable //= value
- Performs floor division using the variable and the right operand and assigns the result back to the variable.
Examples of Assignment Operators
# Assignment operators in Python num = 10 # Using add and assign num += 5 # num is now 15 print(num) # Using subtract and assign num -= 3 # num is now 12 print(num) # Using multiply and assign num *= 2 # num is now 24 print(num) # Using divide and assign num /= 4 # num is now 6 print(num) # Using modulus and assign num %= 4 # num is now 2 print(num) # Using exponent and assign num **= 3 # num is now 8 print(num) # Using floor divide and assign num //= 3 # num is now 2 print(num)
Explanation
- num = 10 assigns the initial value 10 to the variable num.
- Add and Assign (+=): num += 5 # Adds 5 to num and stores the result back in num (now 15)
- Subtract and Assign (-=): num -= 3 # Subtracts 3 from num and stores the result (now 12)
- Multiply and Assign (*=): num *= 2 # Multiplies num by 2 and stores the result (now 24)
- Divide and Assign (/=): num /= 4 # Divides num by 4 and stores the result (now 6)
- Modulus and Assign (%=): num %= 4 # Calculates the remainder of num divided by 4 and stores it (now 2)
- Exponent and Assign (**=): num **= 3 # Raises num to the power of 3 and stores the result (now 8)
- Floor Divide and Assign (//=): num //= 3 # Divides num by 3 and rounds down to the nearest integer, storing the result (now 2)
Identity Operators in Python
Python Identity operators are used to compare the memory location of two objects. These operators do not compare the values of the objects but instead determine if they are the same object with the same memory address. The two identity operators in Python are “is” and “is not“. The “is” operator returns true if both variables point to the same object, while the “is not” operator returns true if both variables point to different objects. This is useful when working with mutable objects, such as lists or dictionaries, where two objects may have the same values but are actually stored in different memory locations. By using identity operators, you can determine if two variables reference the same object and avoid unintended side effects in your code.
Identity operators are important in Python, as they help differentiate objects with similar values but different memory locations. This is crucial when working with complex data structures and comparing the behavior of different objects within your code. So, it is always recommended to use identity operators when comparing objects in Python to ensure accurate results.
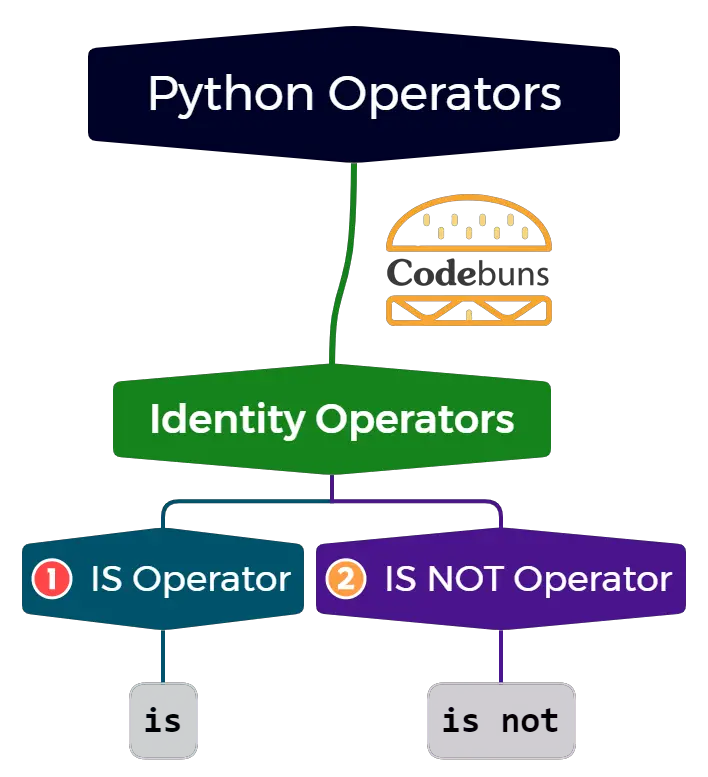
List of Identity operators in Python:
- Identity operator (is)
- Identity operator (is not)
The is Operator
- Syntax: operand1 is operand2
- Evaluates to True if both operand1 and operand2 refer to the same object.
The is not Operator
- Syntax: operand1 is not operand2
- Evaluates to True if operand1 and operand2 do not refer to the same object.
Examples of Identity Operators
# Identity operators in Python a = [1, 2, 3] b = a c = [1, 2, 3] # Using 'is' operator is_same = b is a # True, as b and a refer to the same object print(is_same) # Using 'is not' operator is_not_same = b is not c # True, as b and c do not refer to the same object print(is_not_same)
Explanation
- a = [1, 2, 3] creates a list and assigns it to variable a.
- b = a assigns the reference of a (not a copy) to variable b. Both now refer to the same list object in memory.
- c = [1, 2, 3] creates a separate, identical list and assigns it to variable c.
- is Operator:
- is_same = b is a # True, because b and a refer to the exact same object in memory.
- is not Operator:
- is_not_same = b is not c # True, because b and c refer to different objects, even though their values are identical.
Membership Operators in Python
Python Membership Operators are used to test whether a value is present or not in a sequence. In other words, they help us check if an element exists in a particular data structure, such as lists, tuples, strings, and dictionaries. These operators include “in” and “not in“, and they return a boolean value of either True or False depending on the result of the operation. These operators are useful when working with conditional statements and loops, allowing us to quickly check for the presence of a specific element in a data structure and take appropriate actions based on the result.
It is important to note that these membership operators only work with iterable objects or containers. This means that the data structure being tested must have a defined sequence or order, and each element can be accessed through an index.
For example, the “in” operator wouldn’t work with a dictionary as it doesn’t have a specified order for its key-value pairs. However, we can still use these operators with dictionaries by specifying which data type to check for membership. For instance, the statement “5 in {1: ‘apple’, 2: ‘banana’, 3: ‘orange’}” would return False, but the statement “‘banana’ in {1: ‘apple’, 2: ‘banana’, 3: ‘orange’}” would return True.
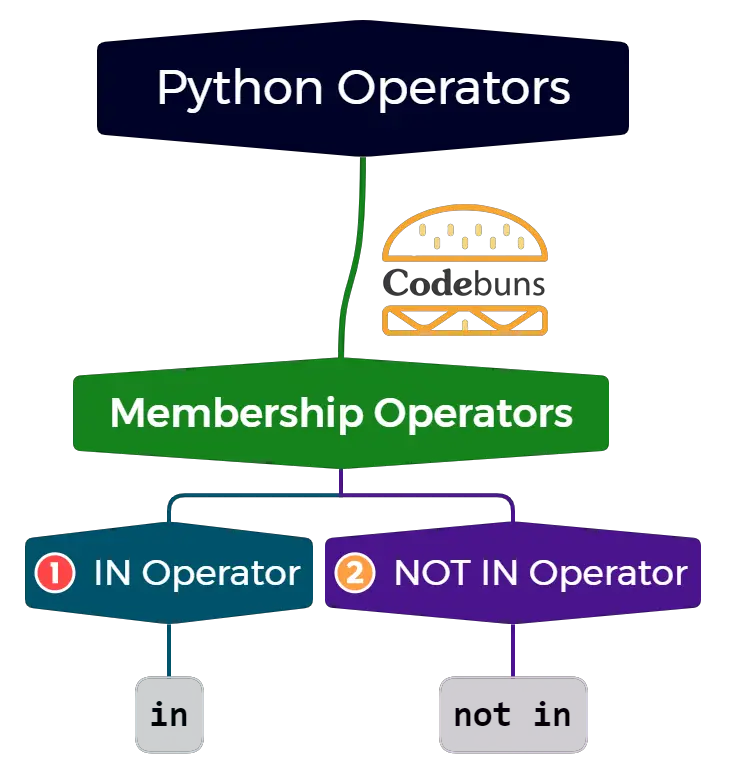
List of Membership Operators in Python:
- Membership Operator (in)
- Membership Operator (not in)
The in Operator
- Syntax: value in sequence
- Returns True if the specified value is present in the given sequence.
The not in Operator
- Syntax: value not in sequence
- Returns True if the specified value is not found in the given sequence.
Examples of Membership Operators
# Membership operators in Python my_list = [1, 2, 3, 4, 5] # Using 'in' operator is_present = 3 in my_list # True, as 3 is in my_list print(is_present) # Using 'not in' operator is_not_present = 6 not in my_list # True, as 6 is not in my_list print(is_not_present)
Explanation
- my_list = [1, 2, 3, 4, 5] creates a list containing the numbers 1 to 5.
- in Operator:
- is_present = 3 in my_list # True, because the value 3 is present within my_list.
- not in Operator:
- is_not_present = 6 not in my_list # True, because the value 6 is not present within my_list.
Exponentiation and Unary Operators in Python
Python Exponentiation and Unary Operators are used to perform mathematical operations on variables or values. Exponentiation involves raising a number to a power, while unary operators are symbols used to change the sign of a variable from positive to negative or vice versa. In Python, there are several operators that can be used for exponentiation, such as the double asterisk (**), which is used to raise a number to a power, and the caret (^) operator, which can also be used for exponentiation in certain versions of Python.
Python Unary operators include the plus (+) and minus (–) signs, commonly used to denote positive and negative numbers. These operators can also be applied to variables or expressions to change their sign. Another unary operator in Python is the tilde (~), which is used for bitwise inversion – a process that flips all bits in a binary number from 0 to 1 or vice versa. Unary operators are handy when working with conditional statements, as they can be used to toggle the truth value of an expression.
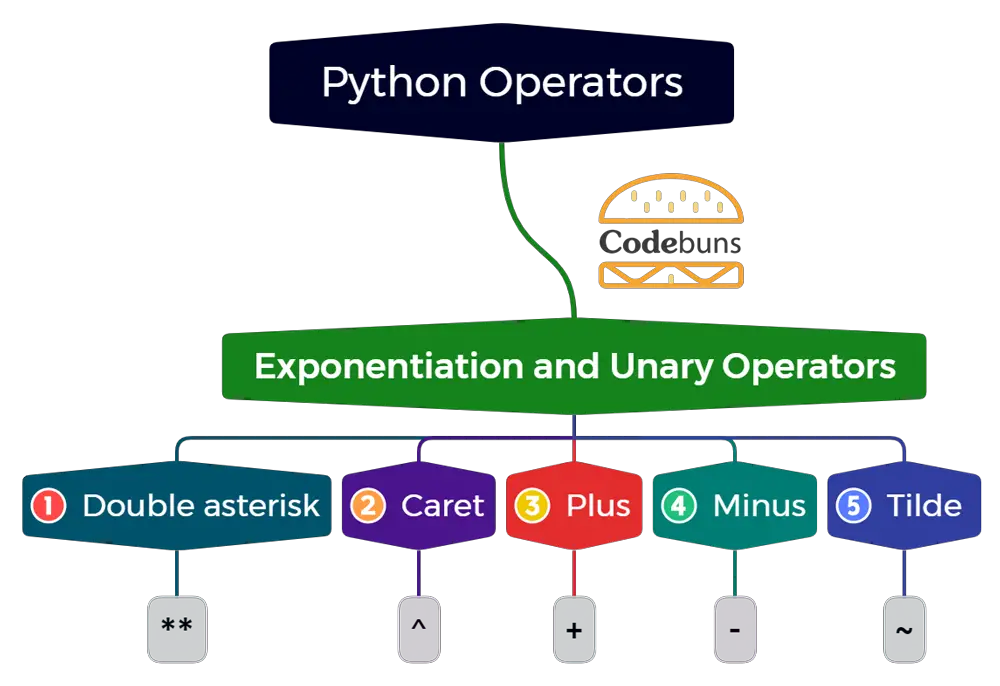
List of Exponentiation and Unary Operators in Python:
- Double asterisk (**)
- Caret (^)
- Plus (+)
- Minus (–)
- Tilde (~)
Exponent Operator (**)
- Syntax: base ** exponent
- This operator is used to raise the base to the power of exponent.
- It’s versatile and capable of handling both power and root calculations depending on the exponent value.
Example of Exponentiation
# Calculating powers using exponentiation result = 2 ** 3
Explanation
- 2 is the base number.
- 3 is the exponent.
- The expression is evaluated as 2 raised to the power of 3, meaning 2 multiplied by itself 3 times: 2 * 2 * 2.
- The result, 8, is assigned to the variable result.
Caret Operator (^)
The Caret (^) operator in Python is used for exponentiation, which involves raising a number to a power. It is similar to the double asterisk (**) operator and can also be used for this purpose, depending on the Python version. However, some versions of Python may interpret the caret differently, such as using it for bitwise operations instead of exponentiation.
Example of Caret Operator
- 2 ^ 3 = 8 (exponentiation using caret operator)
- 2 ** 3 = 8 (equivalent expression using double asterisk operator)
Unary Plus and Minus Operators
Unary operators in Python are used to either retain or change the sign of their operand.
Unary Plus Operator (+)
- Syntax: +operand
- This operator does not affect the operand’s value. It’s more of a formality in code to indicate positive values.
Unary Minus Operator (-)
- Syntax: -operand
- It negates the value of its operand, effectively reversing its sign.
Example of Unary Plus and Minus
# Demonstrating unary operators positive_num = +3 negative_num = -3
- Unary Plus (+):
- positive_num = +3 explicitly assigns the positive value 3 to the variable positive_num.
- While not strictly necessary for positive numbers, it can be used for clarity or emphasis.
- Unary Minus (–):
- negative_num = -3 negates the value 3, making it negative, and assigns it to negative_num.
Unary Tilde Operator (~)
The tilde Operator (~) in Python can be used for bitwise inversion, which can be useful when working with binary numbers in Python. For example, the expression ~1101 will result in -14, as it flips all bits from 0 to 1 or vice versa. This can come in handy when dealing with complex algorithms or data structures that require manipulation of binary values.
Advanced Concepts of Python Operators
Python programming is not just about writing code; it’s about writing it efficiently and understandably. Advanced concepts like the Walrus Operator introduced in Python 3.8, Short-Circuit Evaluation, and understanding Operator Precedence play a crucial role in achieving this. These aspects of Python operators can significantly enhance how you write and understand Python code.
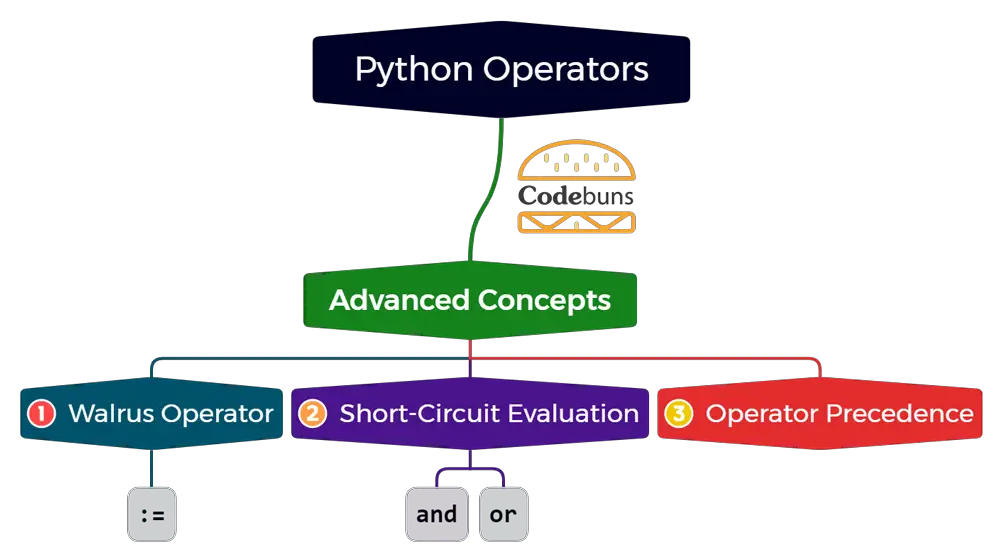
Walrus Operator in Python
The Walrus Operator, or the Assignment Expression, is a new operator introduced in Python 3.8. It allows the assignment of values to variables within an expression itself rather than having to create temporary variables. This eliminates the need for multiple lines of code and makes it easier to write more concise and readable code.
This operator is represented by the symbol “:=“, hence its nickname as the “walrus” operator. It is mainly used to improve the readability and efficiency of code, as it allows for the creation of more compact and concise expressions.
Syntax
variable := expression
Explanation
- This operator assigns the result of the expression to variable and simultaneously returns the value.
- This operator is particularly useful in loops and conditional expressions as it reduces the need for additional lines of code.
Example of Walrus Operator
# Using the Walrus Operator if (n := 10) > 5: print(f"Number {n} is greater than 5")
Explanation
if (n := 10) > 5: # Assigns 10 to n and immediately checks if it’s greater than 5.
- The value 10 is assigned to n using =: within the condition itself.
- The condition is then evaluated, resulting in True (10 is indeed greater than 5).
- The print statement within the if block executes as a result.
Python Short-Circuit Evaluation
Short-Circuit Evaluation in Python is a feature that allows logical expressions to be evaluated only until the final result can be determined. In other words, if the final result of an expression can be determined by evaluating only part of it, then the rest of the expression will not be evaluated. This feature is implemented through short-circuit operators such as `and` and `or`. These operators are also known as Boolean operators and are used to combine logical expressions.
In Python, the `and` operator returns `True` if both operands are evaluated to be true; otherwise, it returns `False`. Similarly, the `or` operator returns `True` if either of the operands is evaluated to be true; otherwise, it returns `False`. This feature saves time and resources by skipping unnecessary evaluations, making the code more efficient.
Example of Short-Circuit Evaluation
result = (5 > 3) and (10 / 0) # Division by zero is not evaluated print(result)
Prints False since the second expression is not evaluated due to the first one being false.
result = (5 < 3) or (10 / 0) # Division by zero is not evaluated print(result)
Prints True since the second expression is not evaluated due to the first one being true.
Operator Precedence in Python
Operator Precedence in Python refers to the order of operations in which operators are evaluated. This means that when multiple operators are used in a single expression, some operators have higher precedence over others and will be evaluated first.
For example, multiplication has a higher precedence than addition, so the expression 2 + 3 * 4 would be evaluated as 14 instead of 20.
In Python, operator precedence is determined by the type of operators used and can be modified using parentheses to group operations. Knowing operator precedence in Python is important for writing efficient, accurate code that produces expected results.
Operators with the same precedence will be evaluated left-to-right unless parentheses specify a different order. This knowledge can also help avoid potential errors and bugs in code by ensuring that operations are performed in the intended order.
Example
x = 2 + 3 * 4
Evaluates as 14 due to multiplication having higher precedence than addition.
y = (2 + 3) * 4
Evaluates as 20 because parentheses are used to group addition first.
Operators and their precedence in Python include:
- Arithmetic Operators (e.g., +, –, *, /) have higher precedence than Logical Operators (e.g., and, or)
- Comparison Operators (e.g., ==, !=) have higher precedence than Assignment Operators (e.g., =)
- Unary Operators (e.g., +x, -x), which perform operations on a single operand, have the highest precedence.
Conclusion
Python operators play a crucial role in programming, allowing us to manipulate and perform operations on variables and values. From arithmetic operators for mathematical calculations to comparison operators for making logical comparisons, Python offers a wide range of operators to suit different needs. These operators provide flexibility and efficiency in writing code, making it easier to perform complex tasks with minimal effort. Whether assigning values, combining strings, or evaluating conditions, understanding and utilizing Python operators is essential for any programmer. So, master these operators to unlock the full potential of Python programming.
Python Reference
FAQ
What are Python Operators and How are They Used?
Python operators are special symbols that carry out arithmetic or logical computation. They are used to perform operations on variables and values, enabling tasks like mathematical calculations, logical comparisons, and manipulating data stored in data structures.
How Do Assignment Operators Work in Python?
Assignment operators in Python are used to assign values to variables. The basic assignment operator is =, and there are also compound assignment operators like +=, -=, *=, which combine an arithmetic operation with an assignment. These make the code more concise and readable.
Can You Explain the Difference Between == and = in Python?
In Python, = is the assignment operator used to assign a value to a variable, like x = 10. On the other hand, == is the equality operator used to compare two values and returns True if they are equal, as in if (x == 10).
What are the Logical Operators in Python and How are They Used?
Logical operators in Python include and, or, and not. They are used to perform logical operations like logical AND (and), logical OR (or), and logical NOT (not). These operators are fundamental in making decisions in code, especially in conditional statements and loops.
How Do Bitwise Operators Function in Python?
Bitwise operators in Python, such as & (AND), | (OR), ^ (XOR), and ~ (NOT), perform operations on binary digits (bits) of integers. They are used in lower-level programming, like system programming or cryptography, where manipulation of individual bits of data is required.