Python Print is a powerful and essential function in the Python programming language. It allows programmers to display output messages and information on the screen. With just a few lines of code, Python Print enables developers to communicate essential data, debug their programs, and provide user feedback. Whether you are a beginner or an experienced programmer, understanding how to use the Python print() function effectively is crucial for writing clear and informative code.
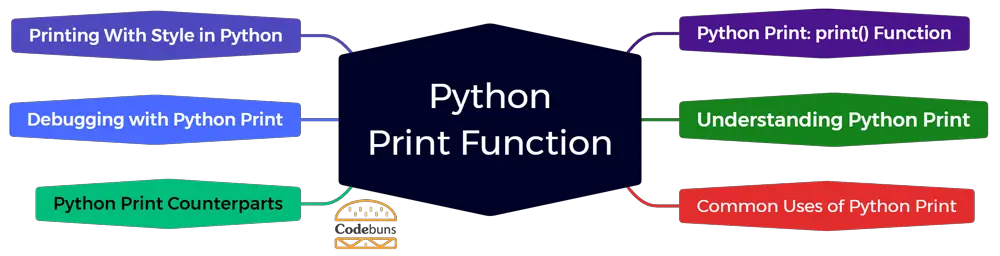
Table of Contents | |
Python Print: print() Function
The Python print() function is a built-in function used in Python programming to display output on the screen. It is commonly used for debugging, displaying user instructions or messages, and printing data values during program execution. Beginners often learn this function early on as it is essential for creating interactive programs.
Python Print Syntax
The syntax for the print() function is straightforward and easy to remember. It takes in one or more arguments, separated by commas, and prints them to the standard output or screen. The general syntax of the print() function is as follows:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
Explanation of Python Print Parameters
Python Print parameters provide control over how the output appears:
- print(): The primary function that displays output in Python.
- Multiple Objects (*objects): This is the input value or values that must be displayed on the screen. It can take multiple arguments, separated by commas.
- Separator (sep=’ ‘): The separator between objects. By default, it is set to a single space character ‘ ‘. We can change this value to any string we want (default is a space).
- End Character (end=’\n’): The end parameter sets the string at the end of the output. By default, it is set to a new line character \n (default is a newline).
- Output File (file=sys.stdout): The file parameter allows us to specify an object where we want our output printed. It defaults to sys.stdout, meaning the output will be shown on the screen (default is the console).
- Flush (flush=False): The flush parameter specifies whether or not we want to flush the output buffer. It defaults to False and is typically used when displaying our output immediately without waiting for a newline character (default is buffered).
Python Print Example 1
Let’s consider a simple example to illustrate the use of print() in Python:
print("Hello", "World", sep="_", end="*", file=sys.stdout, flush=True)
Explanation
- print(): The primary function that displays output in Python.
- *objects: Accepts any number of objects to be printed, separated by the specified sep.
- sep=’ ‘: Defines the character or string used to separate printed objects (default is a space).
- end=’\n’: Specifies what’s added at the end of the printed output (default is a newline).
- file=sys.stdout: Determines the output destination (default is the console).
- flush=False: Controls whether output is immediately displayed or buffered (default is buffered).
Python Print Example 2
The print() function in Python doesn’t return any value. Its purpose is to output information to the console or another output stream. Here’s an example to illustrate its use:
name = "Alice" age = 30 print(name, age, sep=':', end='!')
Explanation
- name = “Alice”: Stores the string “Alice” in the variable name.
- age = 30: Stores the number 30 in the variable age.
- print(name, age, sep=’:’, end=’!’): Prints the values of name and age with customizations:
- print(): The function responsible for displaying output.
- name, age: The variables to be printed.
- sep=’:’: Specifies a colon as the separator between them.
- end=’!’: Replaces the default newline with an exclamation mark.
- The output will be Alice:30!
Understanding Python Print
Python’s print() function is an integral part of the language and is widely used for displaying information. Its evolution from Python 2 to Python 3 reflects a shift towards simplicity and flexibility, making it more consistent with other functions in Python.
Print Is a Function in Python 3
In Python, the print () function has replaced the print statement. This change was made in Python 3 to improve the consistency and readability of the code.
Syntax
print(value(s), sep=' ', end='\n', file=sys.stdout, flush=False)
Explanation
- The syntax now requires parentheses, indicating its function status.
- It allows multiple values, separated by commas, and lets you customize separators, end characters, and output streams.
Example
print("Hello", "world", sep="-")
Explanation
- “Hello”, “world”: Two string values to be printed.
- sep=”-“: Custom separator, a hyphen, used between “Hello” and “world“.
- This code outputs: Hello-world, demonstrating the use of sep in print().
Print Was a Statement in Python 2
In Python 2, it was possible to write multiple expressions within a single print statement by separating them with commas. This feature allowed developers to conveniently display multiple values or variables in a single line of code, making it easier to debug and understand the program’s output.
Syntax
print value(s)
Explanation
- print: The fundamental statement for displaying output.
- value(s): Represents the values or expressions to be printed.
Key Points
- Automatic Space: Python 2 adds a space between printed values by default.
- No Parentheses: Unlike Python 3, parentheses aren’t required around value(s).
- Commas for Separation: Use commas to separate multiple values within the print statement.
Example
print "Hello world"
Explanation
- print: The statement that commands Python to display output.
- “Hello world”: The string to be printed, enclosed in double quotes.
Key Points for Python 2
- No Parentheses Needed: In Python 2, parentheses aren’t required around the string to be printed.
- Automatic Whitespace: Python 2 automatically adds a newline character at the end, creating a visual break.
Common Uses of Python Print
Python’s print() function is widely used for its simplicity and effectiveness in displaying information. It caters to various needs, from outputting simple messages to complex formatted data, making it an indispensable tool in a Python programmer’s toolkit.
Double Quotes Use
Double quotes within the print() function are used to enclose strings that need to be displayed as-is. This is particularly useful when dealing with strings that contain single quotes or apostrophes. By using double quotes, we can avoid syntax errors and ensure the correct interpretation of the string. It’s important to note that both single and double quotes can be used interchangeably in Python, providing flexibility and convenience for programmers.
Example
print("She said, 'Hello!'")
“She said, ‘Hello!'”: The string to be printed contains a quote within quotes.
Single Quotes Use
Using single quotes within the print() function allows us to include strings containing double quotes without causing any syntax errors. This is particularly useful when displaying a message or output that includes quotation marks. We can avoid any conflicts with the double quotes used to define the string itself by using single quotes.
Example
print('A "quoted" word')
Explanation
- ‘A “quoted” word’: This is a string, a sequence of characters enclosed in quotes. Python allows for both single (‘) and double (“) quotes to define strings.
- Notice the use of double quotes “ inside the single-quoted string. This is how Python allows mixing quote types to include quotes as part of the string without causing syntax errors.
- When the above line of code is executed, it displays the text A “quoted” word in the output, with the word “quoted” enclosed in double quotes.
Triple Quotes Use
Triple quotes are also known as multiline strings, allowing us to print multiple lines of text without using the \n line break character. This can save time and make our code more readable.
In addition, multiline strings also preserve any whitespace within the text, making it easier to format our output exactly how we want it. We can even include single or double quotes within triple quotes without causing any errors.
Another benefit of using triple quotes in the print() function is that we can define docstrings, which are useful for providing documentation within our code. These docstrings can be accessed using the doc attribute on a function or class.
Syntax
print('''Line 1 Line 2 Line 3''')
Explanation
Triple Quotes (”’): Create a string that spans multiple lines, preserving formatting:
- Opening triple quotes signal the start of the multi-line string.
- Each line within the quotes is treated as a separate line.
- Closing triple quotes mark the end of the string.
Example
print('''First line Second line Third line''')
Prints a multi-line string exactly as formatted within the triple quotes.
Printing Strings
In Python, strings are a sequence of characters enclosed in single (”) or double (“”) quotes. They can contain letters, numbers, symbols, and even spaces. To display the contents of a string on the screen, we use the print() function.
Example
print("Hello, World!")
This line prints the classic “Hello, World!” message to the console.
String Concatenation
In Python, string concatenation refers to the process of combining two or more strings together. It allows us to create a new string by joining multiple strings. This can be achieved using the “+” operator.
Syntax
print(string1 + string2)
Explanation
- The + operator is used to concatenate strings.
- This method is used to combine multiple strings into a single output.
Example
print("Hello, " + "World!")
Concatenates and prints “Hello, ” and “World!” as “Hello, World!“.
Printing Variables
Python Print is also commonly used to display the values of variables. This is particularly useful for debugging, helping programmers understand how variables change and behave during execution.
Syntax
print(variable)
Explanation
- We simply replace variable with the name of the variable we want to display.
- The function outputs the current value of the specified variable.
Example
user_age = 25 print(user_age)
Explanation
- user_age = 25: Stores the value 25 in a variable named user_age.
- print(user_age): Displays the value of user_age to the console.
Formatted Output: Formatted String Literals (f-strings)
In Python, a formatted string literal, or f-string, is a way to create formatted output. It allows us to insert dynamic values into a string without using concatenation or string formatting methods such as format() and %. F-strings were introduced in Python 3.6 and have become a popular feature due to their simplicity and readability.
To use f-strings, we start the string with the letter f followed by quotes. We can include curly braces {} containing expressions or variables inside the quotes. When the f-string is executed, these expressions are evaluated, and their values are inserted into the string.
Syntax
print(f"String text {variable}")
Explanation
- f”String text {variable}”: An f-string that combines text and a variable:
- f before the opening quote designates an f-string.
- “String text” is the static text string.
- {variable} is a placeholder where the variable’s value will be inserted.
Example
name = "Alice" age = 30 print(f"{name} is {age} years old.")
Explanation
- name = “Alice”: Stores the string “Alice” in the variable name.
- age = 30: Stores the number 30 in the variable age.
- print(f”{name} is {age} years old.”): Creates a dynamic message using f-strings:
- f before the opening quote signals an f-string.
- {name} and {age} embed variable values directly within the string.
Printing Integer
To print integers in Python, simply pass the integer or an integer variable to print().
Syntax
print(integer_variable)
Explanation
- The function displays the integer value.
- Integers can be directly printed without any additional formatting.
Example
age = 25 print(age)
This line prints the integer value stored in age, which is 25.
Printing Exponential
To use f-strings with the print() function, we must use the f prefix before the string. This indicates that the string contains expressions that must be evaluated and inserted into the final output. F-strings also allow us to format our output by specifying how we want our values displayed.
To print exponential values using f-strings, we can use the e-format specifier. This tells Python to convert our value into exponential form and print it accordingly.
Syntax
print(f"{variable:e}")
OR
print("{:e}".format(variable))
Explanation
- variable: A placeholder for the variable containing the number to be formatted.
- {:e}: The format specifier that instructs Python to display in scientific notation:
- : signals formatting instructions within f-strings or the format() method.
- e stands for “exponential,” representing scientific notation.
Methods
- f-strings (Python 3.6+): – f”{variable:e}”: Concise syntax for embedding formatting directly within strings.
- format() Method: – “{:e}”.format(variable): Versatile method compatible with earlier Python versions.
Example
number = 0.0000123 print(f"{number:e}")
Explanation
- number = 0.0000123: Assigns a small number to the variable number.
- print(f”{number:e}”): Prints number in scientific notation using an f-string:
- f”{number:e}”: Combines text and formatting within the f-string.
- {number}: Inserts the value of number.
- :e: Specifies scientific notation for compact and readable output.
Printing Float
Printing a number as a float involves simply passing a float or float variable to print() function.
Syntax
print(float_variable)
The function displays the floating-point number.
Example
price = 19.99 print(price)
Printing Multiple Objects
One helpful feature of the print() function is that it can display multiple objects at once. Instead of using multiple print statements for each object, we can separate them with a comma inside the print() function.
Syntax
print(object1, object2, ...)
Explanation
- We can insert multiple objects to print, separated by commas.
- The function displays each object in order, separated by a space (default separator).
Example
name = "Alice" age = 30 print("Name:", name, "Age:", age)
Explanation
- name = “Alice”: Assigns the string “Alice” to the variable name.
- age = 30: Assigns the number 30 to the variable age.
- print(“Name:”, name, “Age:”, age): Prints a formatted message using commas:
- “Name:”: Static text to provide context.
- name: Variable holding the person’s name.
- “Age:”: Additional static text for clarity.
- age: Variable containing the numerical age value.
Custom Separators: Separating Multiple Arguments
There may be situations where we want to use a different separator, such as a comma, a dash, or even a custom string. This is where the concept of custom separators comes into play.
Custom separators in the Python print() function allow us to specify a specific character or string for the separator between multiple arguments. This gives us more control over how our output is displayed and makes it easier for users to understand the information being printed.
To use a custom separator, we need to add the “sep” parameter in our print() function, followed by an equal sign and the desired separator enclosed in quotes. For example, if we want to use a dash as the separator between two strings, our code would look like this: print(“Hello”, “World”, sep=”-“).
Syntax
print(object1, object2, sep='your_separator') print(arg1, arg2, ..., sep='separator')
Explanation
- The sep parameter allows us to define what character or string should separate the arguments.
- By default, sep is set to a space (‘ ‘) but can be changed to any character or string.
Example 1
print("A", "B", "C", sep='|')
Explanation
- This line prints the letters “A“, “B“, and “C“, separated by the pipe character |.
- It demonstrates using a custom separator, resulting in the output “A|B|C“.
- sep=’|’: The separator argument, customizing how elements are joined:
- sep determines the character or string used to separate values.
- ‘|’ specifies a vertical bar (|) as the separator.
Example 2
print("Hello", "World", sep=", ")
Explanation
- Print “Hello” and “World” with a comma and a space as a separator.
- The output will be: “Hello, World“.
Preventing Line Break
Python Print function automatically adds a new line at the end of the printed text. This can be inconvenient in certain situations where we want to print multiple items on the same line or have control over the line breaks in our output. Fortunately, a few ways exist to prevent this automatic line break behavior.
One option is to use the end parameter when calling the print() function. By default, this parameter is set to the new line character \n, but we can change it to any other string or even an empty string to prevent the line break.
Another way to prevent line breaks is by using the sep parameter. This allows us to specify a different separator between multiple items that are being printed. By setting this parameter to an empty string, all items will be printed without any additional characters between them, effectively preventing a new line from being added at the end.
We can use Python 3.6 or above to use the new end and sep syntax for the print function, allowing us to pass them as keyword arguments without worrying about their order. This can make our code more readable and easier to understand.
Syntax
print("Your text", end='your_ending')
Explanation
- “Your text”: The text string to be printed.
- end=’your_ending’: The end argument, crafting a custom ending:
- end determines what follows the printed text, replacing the default newline (\n).
- ‘your_ending’ specifies the desired ending characters or string.
Example
print("Hello, World!", end='')
Explanation
- “Hello, World!”: The string to be presented to the user.
- end=”: The crucial argument that prevents an automatic newline:
- end dictates what follows the printed text.
- Setting it to an empty string (”) suppresses the usual newline (\n).
Printing to a File
Printing to a file using the file parameter in the Python print() function allows us to write the output of our program directly into a specified file. This is useful when we want to save the results of our program for future reference or when working with large amounts of data.
To use this feature, add an argument to the print() function, specifying the file name and mode in which we want to open it. For example, if we’re going to write the output to a new file called “output.txt“, we would use print(“Hello File!”, file=open(“output.txt”, “w”)).
One thing to note is that this method will overwrite any existing data in the output file, so be careful when selecting file name and mode.
Syntax
print(argument, file=file_object)
Explanation
- argument: The item to be printed, which can be a string, number, or another data type.
- file=file_object: The optional argument that redirects output to a file:
- file determines the destination for the printed content.
- file_object represents a file object that has been previously opened for writing.
Example
with open('output.txt', 'w') as file: print("Hello, File!", file=file)
Explanation
- with open(‘output.txt’, ‘w’) as file:: Establishes a secure connection to a file:
- with open(): Opens the file named ‘output.txt’ in write mode (‘w’).
- as file:: Assigns the file object to the variable file for interaction.
- print(“Hello, File!”, file=file): Writes a message directly to the file:
- print(): Performs the writing action.
- “Hello, File!”: The text to be written.
- file=file: Redirects output to the file object instead of the console.
Buffering print() Calls
Buffering in Python refers to a temporary storage area used to store data before it is processed or displayed. By default, the print() function does not buffer its output, which means it displays each line of text as soon as it is received.
To improve performance and speed up the execution, we can enable buffering in the print() function by using the argument flush=False. This tells the function to store the output in a buffer and only display it when necessary.
By buffering print calls, we can reduce the number of system calls made and improve performance. It is especially useful when dealing with large amounts of data or continuous printing within a loop.
Syntax
print(argument, flush=True_or_False)
Explanation
- argument: The content to be printed, whether text, numbers, or other data.
- flush=True_or_False: An optional argument controlling immediate output flushing:
- flush dictates whether output is instantly displayed or buffered.
- True forces immediate flushing, ensuring visibility without delay.
- False (default) allows buffering, potentially optimizing performance.
Example
print("Buffered Output", flush=True)
This prints “Buffered Output” and immediately flushes the output buffer.
Printing Custom Data Types
To print custom data types, such as objects of a class, in a more readable format, we can use special methods called dunder methods. Dunder stands for double underscore, and these special methods allow us to customize how the object is printed when used with the print() function.
For example, by defining a str() method within our class, we can specify how the object should be represented as a string when printed using the print() function. This allows for better readability and understanding of the data stored in the object.
Example
class Student: def __init__(self, name, age): self.name = name self.age = age def __str__(self): return f"Student Name: {self.name}\nStudent Age: {self.age}" student1 = Student("John", 21) print(student1) # Output: # Student Name: John # Student Age: 21
Explanation
- class Student:: Establishes a blueprint for creating students.
- __init__(self, name, age):: The constructor, crafting each student:
- self: References the student object being built.
- name: Stores the student’s name.
- age: Preserves the student’s age.
- __str__(self):: Determines how a student is displayed as a string:
- Returns a formatted message containing essential student details.
- student1 = Student(“John”, 21): Welcomes a new student named “John“, age 21.
- print(student1): Showcases the student’s information.
Printing With Style in Python
Python’s print() function, known for its simplicity, can be used creatively to enhance the output of our program, making it visually appealing and interactive. From pretty-printing complex data structures to adding colors and animations, print() can be employed in various inventive ways to elevate the user experience.
Pretty-Printing Nested Data Structures
Pretty-printing is a method to display nested or complex data structures like lists, dictionaries, or JSON in a readable and formatted way.
Syntax
print(json.dumps(your_data_structure, indent=number_of_spaces))
Explanation
- json.dumps(): The translator converting data into a readable format:
- json: The Python library handling JSON data exchange.
- dumps(): The function responsible for serializing data into JSON strings.
- your_data_structure: The information to be transformed into JSON.
- indent=number_of_spaces: The optional argument for visually pleasing output:
- Controls the number of spaces used for indentation, enhancing readability.
Example
import json data = {"name": "Alice", "age": 30, "hobbies": ["Reading", "Cycling"]} print(json.dumps(data, indent=4))
Explanation
- import json: Imports the json module.
- data = {“name”: “Alice”, “age”: 30, “hobbies”: [“Reading”, “Cycling”]}: Constructs a Python dictionary containing personal details:
- Stores a person’s name, age, and hobbies.
- print(json.dumps(data, indent=4)): Transforms the data into a clear and presentable JSON format:
- json.dumps(): The translator converts the Python dictionary into a JSON string.
- indent=4: The stylist ensures well-formatted output with four spaces of indentation.
Adding Colors With ANSI Escape Sequences
ANSI escape sequences are special characters that can be used to manipulate the formatting of text in a terminal or console. These sequences start with the character \x1b, followed by a square bracket and a series of numbers and/or characters. In Python, we can use these escape sequences inside the print() function to change the color of our output.
To add colors to the output, we need to use two specific escape sequences – \x1b[ and m. The sequence \x1b[ is used as a prefix before specifying the color code, while the sequence m marks the end and resets the formatting back to default. For example, if we want to print a word in red color, we can write it as print(“\x1b[31mHello\x1b[m”).
Syntax
print("\033[ColorCode;StylemYour Text\033[0m")
Explanation
- \033[ColorCode;Stylem: The magic sequence for text styling:
- \033: Signals the terminal to interpret formatting codes.
- ColorCode: Determines the desired text color (e.g., 31 for red).
- Style: Optionally sets text styles like bold (1) or italics (3).
- Your Text: The message to be printed in the chosen style.
- \033[0m: The reset code, returning to default formatting:
- Ensures subsequent text appears in standard colors and styles.
Example
print("\033[31;1mRed Text\033[0m")
We are using two ANSI escape sequences in the above example – \033[31;1m and \033[0m. The sequence \033[31;1m specifies the color code as 31 (which represents red) and 1 (which makes the text bold). This means that our output will be printed in bold red color. Then, the sequence \033[0m marks the end of our formatting and resets it back to default. Therefore, the output will only have “Red Text” in bold red color on a plain background.
Building Console User Interfaces
The print() function can be creatively used to build simple console user interfaces, providing a basic yet effective user interaction.
Example
print("1. View Item\n2. Add Item\n3. Exit") # Output: # 1. View Item # 2. Add Item # 3. Exit
The above code creates a simple menu interface in the console. Each option is displayed on a new line, offering a clear choice to the user.
Living It Up With Cool Animations
The print() function has various parameters that can be used to customize the output, including the end parameter. The end=’\r’ parameter allows for a carriage return (or ‘CR’) at the end of the printed message, essentially moving the cursor back to the beginning of the line.
This may seem like little, but it can create cool animations and effects when combined with other parameters and techniques. Using a loop and changing the end parameter each time, one can print out multiple messages on the same line, creating a sense of movement or change.
Syntax
print("Animation Frame", end='\r')
Explanation
- Use the end=’\r’ to return the cursor to the start of the line, enabling overwriting of text.
- Combine with time delays to create an animation effect.
Example
import time for i in range(10): print(f"\rLoading... {i*10}%", end='') time.sleep(0.5) # Output: # Loading... 90%
Explanation
- import time: Imports time module.
- for i in range(10):: Orchestrates a loop that repeats 10 times:
- i: Serves as the counter, keeping track of progress.
- print(f”\rLoading… {i*10}%”, end=”): Dynamically displays a loading message:
- \r: Resets the line, ensuring a clean update each time.
- f-string: Embeds the current percentage calculated as i*10.
- end=”: Suppresses the default newline, keeping text on one line.
- time.sleep(0.5): Instructs the program to pause for half a second:
- Creates a visual delay, simulating a loading process.
Making Sounds With print() Function
To make a beep sound with the print() function, we need to use the \a escape sequence. We can also control the duration of the beep by adding a number after \a, like so: \a3. This will make the beep sound last for 3 seconds. Using different escape sequences and numbers, we can also create other sounds, such as a bell or a whistle.
For example, if we want to make a beep sound that lasts for 2 seconds and is followed by a bell sound, we can use the following code: print(“\a2\07”). This will first create a 2-second long beep sound and then immediately follow it with a bell sound. We can experiment with different combinations of escape sequences and numbers to create our unique sounds.
Example
print('\a')
This line attempts to produce a beep sound using the ASCII Bell character.
Note: The ability to make a sound depends on the operating system and terminal settings.
Debugging with Python Print
Debugging is a crucial part of programming, and Python’s print() function is a simple yet effective tool for this purpose. It allows developers to trace code execution, log values at different stages, and debug issues by printing out necessary information in an easily understandable format.
Tracing
Tracing with print() involves displaying the flow of execution and the state of variables at various points in our code.
Syntax
print("Trace: ", variable)
Example
for i in range(5): print("Trace: Loop iteration", i) # Output: # Trace: Loop iteration 0 # Trace: Loop iteration 1 # Trace: Loop iteration 2 # Trace: Loop iteration 3 # Trace: Loop iteration 4
Explanation
- Each iteration of the loop prints the current value of i.
- This trace helps in understanding the loop’s execution and the changing value of i.
Logging
Using print() for logging involves recording events or values that occur during the execution of a program.
Syntax
print("Log: ", event_description)
Example
if user_login_successful: print("Log: User logged in successfully")
Explanation
- This prints a log message when a user logs in successfully.
- The log helps in tracking user activity and successful authentication.
Debugging
The print() function can be effectively used for debugging by printing variable values, function calls, and error messages.
Example
try: result = some_function() print("Debug: Function result", result) except Exception as e: print("Debug: Error occurred", e) # Output: # Debug: Error occurred name 'some_function' is not defined
Explanation
- try:: This keyword starts the block of code that might raise an exception.
- result = some_function(): The mission, focused on executing a function:
- Attempts to call a function named some_function().
- Stores its return value in the result variable, if successful.
- print(“Debug: Function result”, result): The victory lap, sharing success:
- Displays a message with the function’s result, indicating a smooth operation.
- except Exception as e:: This line catches any exception that inherits from the base Exception class:
- Activated if some_function() encounters an exception.
- Captures the exception details in the variable e.
- print(“Debug: Error occurred”, e): The post-incident report, revealing the issue:
- Prints a message acknowledging the error and its details.
Python Print Counterparts
In Python, while the built-in print() function is widely used for outputting data, there are also alternative methods both within the standard library and in third-party packages. These counterparts offer extended functionality and customization, catering to specific needs or preferences in different programming scenarios.
Built-In
Python’s standard library provides several built-in counterparts to print(), such as logging and file writing, which are suited for more structured output.
The logging module in Python provides a way to output detailed and organized information for debugging purposes. With the logging.info() method, developers can display informational messages to the console or log file as an alternative to the print() function.
Syntax for Logging
logging.info("Your message")
Explanation
- The logging module allows for different levels of message severity, providing a more structured and configurable output compared to print().
- For file writing, the syntax file.write(“Your message”) is used, directing the output to a file instead of the console.
Example
import logging logging.basicConfig(level=logging.INFO) logging.info("This is an info message")
Explanation
- import logging: Imports the logging module, a powerful tool for logging information in Python.
- logging.basicConfig(level=logging.INFO): Sets up the logging system:
- Configures basic logging settings.
- Specifies level=logging.INFO, meaning messages of level INFO and above will be logged.
- logging.info(“This is an info message”): Logs a message with the level “INFO” to the default output stream (usually the console).
Third-Party
There are also third-party libraries like rich and prettyprinter that offer enhanced printing capabilities, such as colorized and formatted output.
Syntax for Rich
rich.print("Your message")
Example
from rich import print as rich_print rich_print("[bold red]Alert![/bold red] This is a styled message")
Explanation
- The first line imports the print function from the rich library, renaming it to rich_print.
- The second line prints a message with styling – bold and red text – an enhanced and visually appealing output, far beyond the capabilities of the standard print() function.
Steps
- The rich library is installed in our Python environment. If it’s not installed, we can install it using pip: pip install rich
- Run the above code in an environment where the rich library’s features are supported, like a command-line interface or a terminal that supports ANSI escape sequences for styling.
When executed, it will display the word “Alert!” in bold red, followed by “This is a styled message” in the default text style.
Conclusion
The print() function is a powerful and versatile tool for displaying output and debugging. It enables developers to display results, gain insights into variables and data structures, and handle complex outputs. Understanding and effectively utilizing this function is essential for efficient coding in Python. Embrace the print() function as your go-to method for displaying information in your programs.
Python Reference
FAQ
How do I use the print() function in Python?
The print() function is used to display text or variables to the console. Simply enclose the content you want to print in parentheses, like print(“Hello, World!”).
Can I print multiple variables and strings in one print() statement?
Yes, you can print multiple items by separating them with commas, as in print(var1, var2).
How can I prevent print() from going to a new line after each statement?
Use the end parameter, like print(“Hello”, end=””), to prevent print() from adding a newline character at the end.
Is it possible to format strings within the print() function?
Yes, Python supports formatted strings, or f-strings, where you can embed expressions inside string literals, like print(f”Value: {value}”).
Can print() output be redirected to a file?
Absolutely, by using the file parameter. For example, print(“Hello, file!”, file=open_file) writes to the open_file file object.