Table of Contents | |
Python Conditional Statements
Python conditional statements are used to make decisions in code based on whether a certain condition is met. The three main types of conditional statements are:
- if statement
- if-else statement
- if-elif-else statement
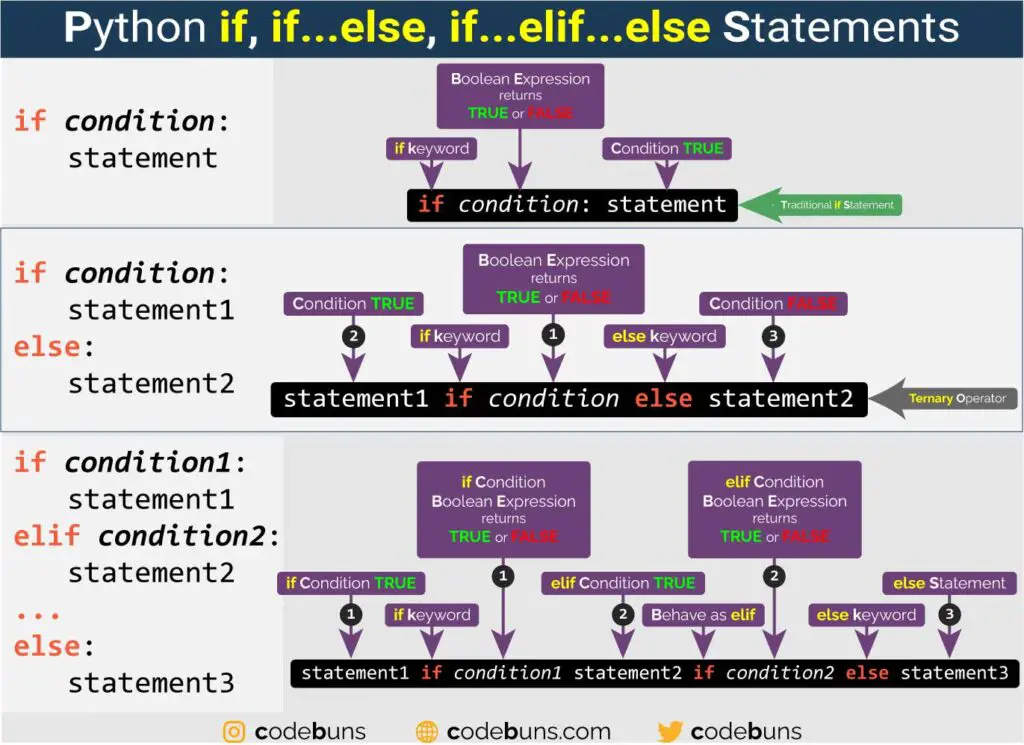
Python if statement
The if statement in Python is a fundamental control structure used for conditional execution of code. The action under the if statement is performed if the condition is true. This makes your code perform different actions depending on the situation, making it more versatile and efficient.
The basic syntax of an if statement is as follows:
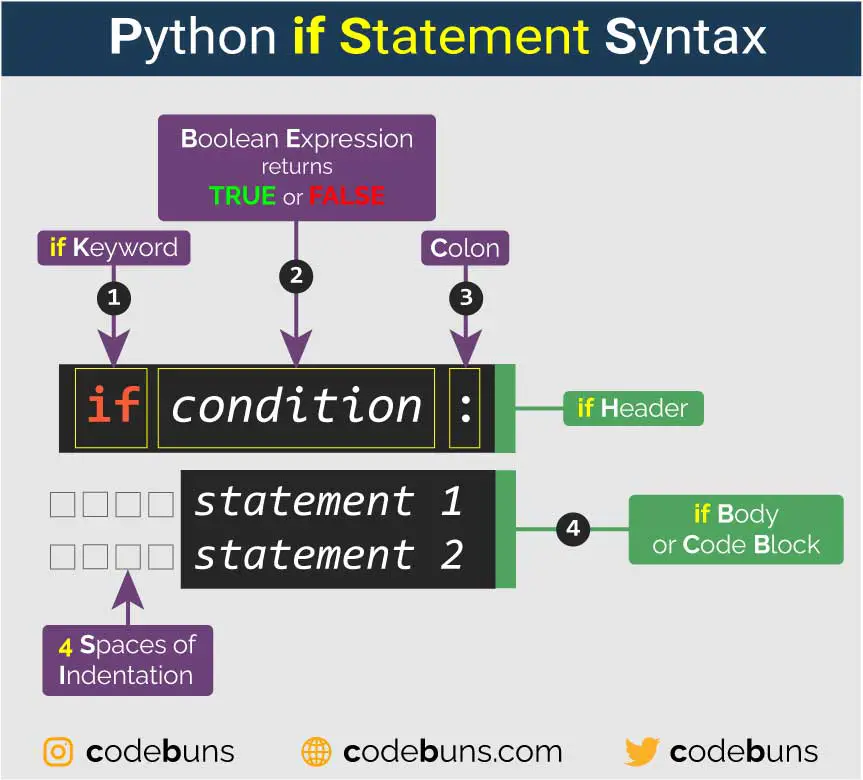
if condition:
# Code Block to execute if the condition is true
- The if keyword starts the if statement.
- The condition is a Boolean expression that evaluates to either True or False.
- The colon (:) signifies the end of the condition and the beginning of the code block that will be executed if the condition is true.
- The code block that follows the if statement must be indented using spaces or a tab. The indentation is important because Python uses indentation to determine the scope of the code block.
Python if Statement Flow Diagram
The flow of if condition can be illustrated with the following diagram:
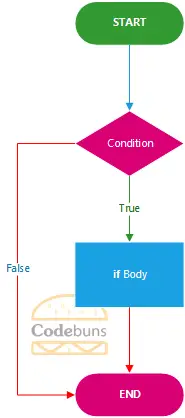
- START: Begin the if statement.
- Condition: Any Boolean expression that evaluates to either True or False. If it’s true, control passes to the if statement body. If false, the control directly jumps to step 4.
- if Body: The block of code that will be executed if the condition is True.
- END: The end of the if statement. The interpreter will continue running any subsequent code that isn’t part of the if statement.
Here’s a simple example:
x = 5
if x > 0:
print("x is a positive number.")
# Output:
# x is a positive number.
Here’s what each line does:
x = 5
: This is a variable assignment. It assigns the integer value 5 to the variable x.(if x > 0:)
: This is a conditional if statement. It checks whether the condition inside the parentheses (x > 0) is True or False. In this case, it’s checking whether x is greater than 0.print("x is a positive number.")
: This line of code is indented under the if statement, which means it’s a part of the if block. This line will be executed if the condition of the if statement is True. The print function in Python outputs the specified message to the screen. Here, it will print “x is a positive number.”
Python if Statement in One Line
Python allows if statements to be written in a single line for conciseness.
Example
x = 5
if x > 0: print("x is a positive number.")
We shortened the previous example into one line. The result is the same.
The above code first assigns the value 5 to the variable x. Then, it uses an if statement to check if x is greater than 0. If it is, the code prints the message “x is a positive number.” If x is not greater than 0, the code does nothing.
Multiple Conditions in Python if Statement
We can check multiple conditions within a single if statement using logical operators such as and, or, and not.
Example
x = 5
y = 10
if x > 0 and y > 0:
print("Both x and y are positive numbers.")
Here, the print statement is executed if both conditions are true – that is, if both x and y are greater than 0.
Python if…else Statement
The Python if…else statement is a conditional statement that allows you to execute different blocks of code based on the value of a Boolean expression. The Boolean expression can be any value that can be evaluated as True or False. If the Boolean expression is True, the block of code under the if statement will be executed. If the Boolean expression is False, the block of code under the else statement will be executed. The basic syntax for an if…else statement is:
Syntax
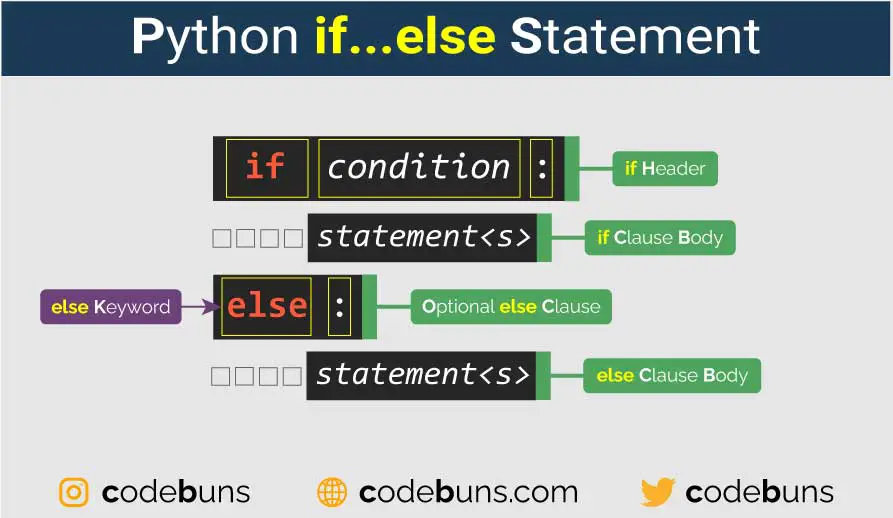
if condition:
# Code Block to execute if the condition is true
else:
# Code Block to execute if the condition is false
In this syntax:
- The if keyword is used to start the if statement.
- The condition is a Boolean expression that is evaluated.
- If the condition is true, the code block indented under the if keyword is executed.
- If the condition is false, the code block indented under the else keyword is executed.
- The else keyword is optional. If it is not present, and the condition is false, nothing happens.
Python If…else Statement Flow Diagram
The flow of if…else can be illustrated with the following diagram:
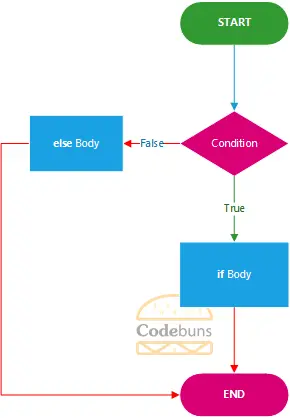
- START: Begin the if…else statement.
- Condition: The condition within the if clause is evaluated. This condition must be a Boolean Expression that results in either True or False.
- if Body: If the condition from step 2 is True, the program will execute the code block within the if statement.
- else Body: If the condition from step 2 is False, the program will execute the code within the else block. The else block only executes when the if condition fails.
- END: After either the if block or the else block is executed, the control will move to the next part of your program, signifying the end of the if…else construct.
Here’s a short example:
x = -5
if x > 0:
print("x is a positive number.")
else:
print("x is not a positive number.")
# Output:
# x is not a positive number.
In this example, the value of x is not greater than 0. Therefore, the string “x is not a positive number.” will be printed to the console. If the value of x were greater than 0, the string “x is a positive number.” would be printed instead.
Python if…else Statement in One Line
You can write Python if…else statements in one line using a ternary conditional expression, also known as the conditional operator.
Example
x = -5
print("x is a positive number.") if x > 0 else print("x is not a positive number.")
This one-liner produces the same result as the previous example.
The code first assigns the value -5 to the variable x. Then, it uses the ternary operator to check if x is a positive number. The ternary operator is a shorthand for an if-else statement. In this case, the ternary operator evaluates the expression x > 0. If the expression is True, the value “x is a positive number.” is printed. If the expression is False, the value “x is not a positive number.” is printed.
In this case, the expression x > 0 is False because -5 is less than 0. Therefore, the value “x is not a positive number.” is printed.
Python if…elif…else Statement (elif Ladder)
The if…elif…else statement allows us to check multiple conditions and execute a specific block of code as soon as one of the conditions is true.
Syntax
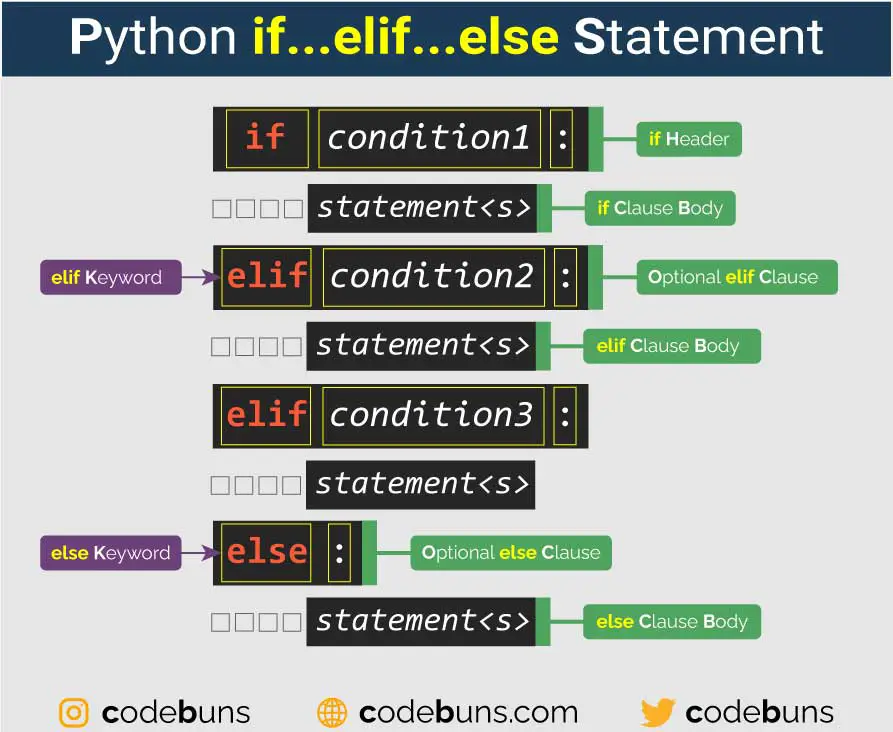
if condition1:
# this code block will be executed if condition1 is true
elif condition2:
# this code block will be executed if condition1 is false but condition2 is true
elif condition3:
# this code block will be executed if both condition1 and condition2 are false, but condition3 is true. You can have as many elif parts as you need.
else:
# this code block will be executed if all the above conditions are false
You should note that:
- The conditions are evaluated from top-to-bottom. As soon as one condition is true, its code block is executed, and the rest are not evaluated.
- The elif and else parts are optional. An if statement can have zero or more elif parts and it can end with an else part or not.
- Python uses indentation to define code blocks. The code block associated with each condition should be indented.
- Each condition is a Boolean Expression that results in True/False.
Python if…elif…else Statement Flow Diagram
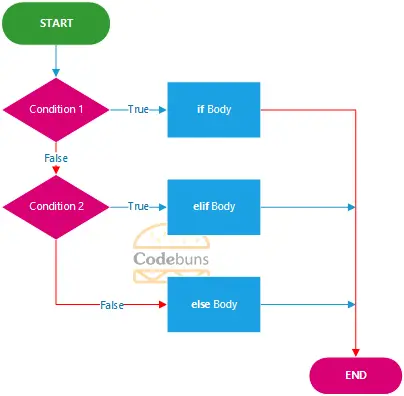
- START: The initial point of execution of the Python code.
- Condition: The condition or expression that is evaluated by the if statement. If the condition is true, the code in the if body is executed. If the condition is false, the code in the elif body or the else body is executed, depending on whether there are any elif conditions.
- if Body: The block of code that is executed if the condition is true.
- elif Body: The block of code that is executed if the elif condition is true. If there are multiple elif conditions, the code in the first true elif body is executed.
- else Body: The block of code that is executed if none of the conditions are true.
- END: The end of the if…elif…else construct.
Example
x = 0
if x > 0:
print("x is a positive number.")
elif x == 0:
print("x is zero.")
else:
print("x is a negative number.")
# Output:
# x is zero.
Here, the second condition is true, so x is zero. is printed to the console.
Python elif Statement in One Line
You can use a one-liner ternary conditional expression to achieve a similar effect to an elif statement.
Example
x = 0
print("x is a positive number.") if x > 0 else print("x is zero.") if x == 0 else print("x is a negative number.")
Same result as before.
It’s important to note that this one-liner is not as readable as the traditional if-elif-else block, and it can become difficult to understand as the conditions and expressions grow more complex. Use it only when the expressions are simple and straightforward.
Nested if Statements
Nested if statements refer to using one or more if statements inside another if statement. These nested structures allow for more complex decision-making in your code, as they enable you to test multiple conditions in a hierarchical manner.
The syntax of nested if statements is as follows:
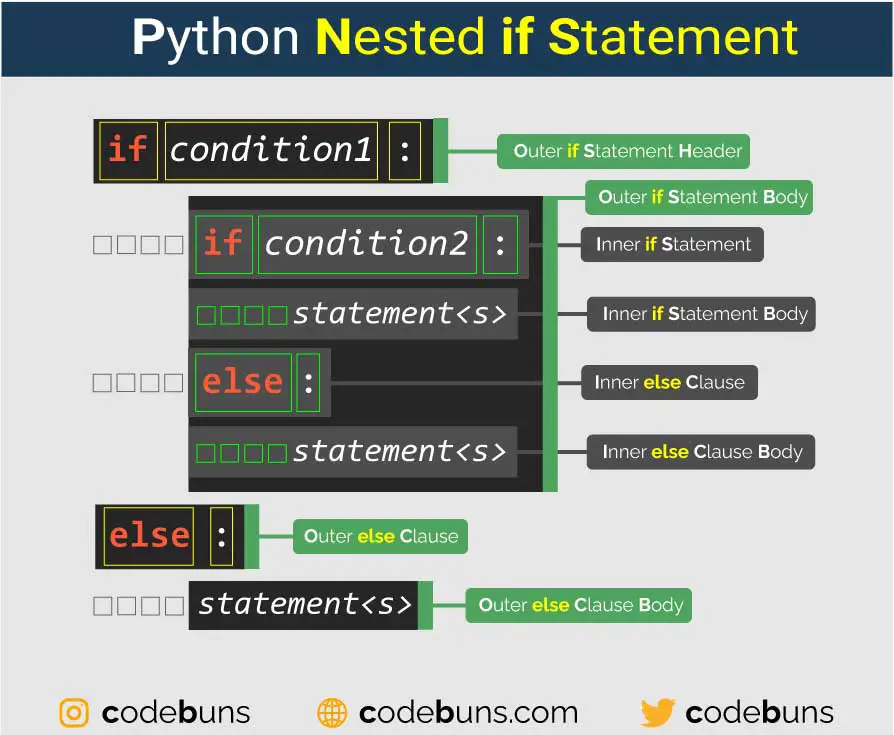
if condition1:
if condition2:
# Code block executed if both conditions are true
else:
# Code block executed if condition2 is false but condition1 is true
else:
# Code block executed if condition1 is false
Here’s a step-by-step explanation of how nested if statements work:
- The first if statement checks the condition1. If the condition1 is true, the second if statement is executed.
- The second if statement checks the condition2. If the condition2 is true, the code block inside the if statement is executed.
- If the condition2 is false, the else block of the second if statement is executed.
- If the condition1 is false, the else block of the first if statement is executed.
Let’s look at an example to illustrate nested if statements:
x = 5
if x >= 0:
if x == 0:
print("x is zero.")
else:
print("x is a positive number.")
else:
print("x is a negative number.")
# Output:
# x is a positive number.
In the above example, the first if statement checks if the x variable is greater than or equal to 0. If it is, the second if statement is executed. This second if statement checks if the x variable is equal to 0. If it is, the “x is zero.” message is printed. If it is not, the “x is a positive number.” message is printed.
If the first if statement is not true, the else block is executed. This block prints the “x is a negative number.” message.
Nested if statements can be used to check multiple conditions and execute different code blocks based on the results of these tests. They can be a powerful tool for controlling the flow of your program.
Python ShortHand Statements
Python shorthand statements are a way to write code in a more concise and compact way. They are often used to replace longer, more verbose statements. Some of the most common shorthand if statements in Python include:
- ShortHand if Statement
- ShortHand if…else Statement
ShortHand if Statement
A one-line if statement is a traditional way to write an if statement that can be used to execute a block of code if a condition is true.
Syntax
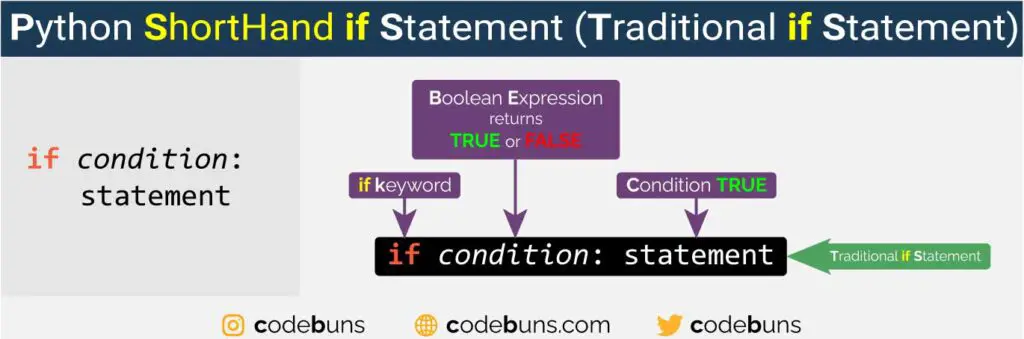
if condition: statement_if_true
In this syntax:
- The if keyword is used to start an if statement.
- The condition is a Boolean expression that evaluates to either True or False.
- The colon (:) after the condition marks the end of the condition clause.
- The statement is a Python statement that is executed if the condition evaluates to True.
Example
x = 10
if x > 0: print("Positive number")
# Output:
# Positive number
In this example, if the condition (x > 0) is true, then the print statement is executed.
ShortHand if…else Statement
Ternary operators, also known as conditional operators, are a shorthand way to write an if-else statement on a single line. They are used to evaluate something based on a condition being true or false.
Syntax
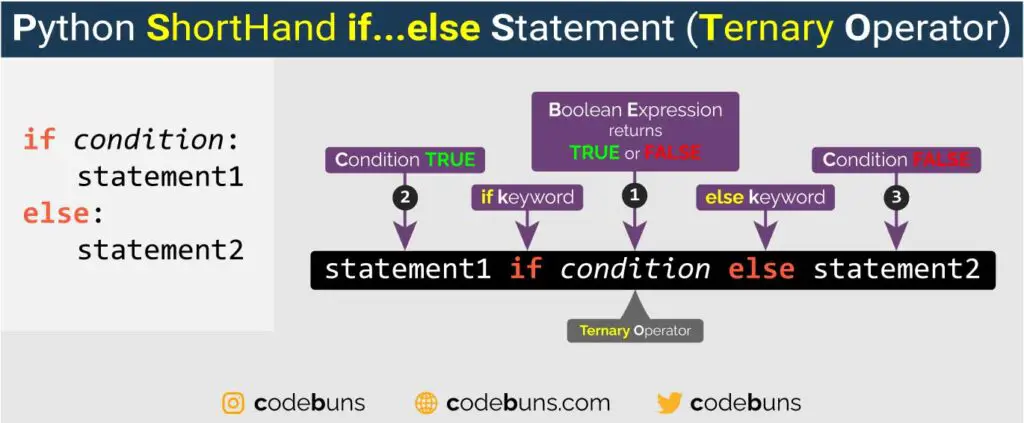
statement1 if condition else statement2
The above syntax is a ternary operator, also known as a conditional expression.
The syntax consists of three parts:
- The condition is the Boolean expression that is evaluated as either True or False.
- The statement1 is the expression that is evaluated if the condition is true.
- The statement2 is the expression that is evaluated if the condition is false.
Example
x = 10
print("Positive number") if x > 0 else print("Non-positive number")
# Output:
# Positive number
In this example, if the condition (x > 0) is true, “Positive number” is printed. If the condition is false, “Non-positive number” is printed. This is equivalent to:
x = 10
if x > 0:
print("Positive number")
else:
print("Non-positive number")
# Output:
# Positive number
Note that the conditional expression in Python includes an else part, which is mandatory. It is evaluated when the condition is false. In the traditional if statement, the else part is optional.
ShortHand if…elif…else Statement
The if…elif…else statement allows you to make decisions based on the value of a variable or expression. However, it can sometimes be long and cumbersome to write out all of the code for each condition. In these cases, you can use the shorthand if…else statement to write your code on a single line.
The shorthand if…else statement uses the ternary operator, which is a special operator that can be used to evaluate a conditional expression and return one of two values depending on the outcome.
Syntax
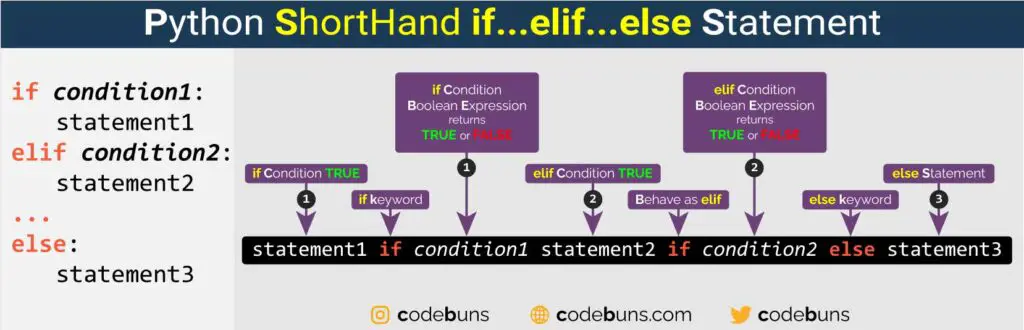
statement1 if condition1 statement2 if condition2 else statement3
Here is how syntax works, step-by-step.
- statement1 if condition1: This is the first statement that will be executed if the condition1 evaluates to True.
- statement2 if condition2: This is the second statement that will be executed if the condition2 evaluates to True.
- else statement3: This is the statement that will be executed if neither condition1 nor condition2 evaluates to True.
- The condition can be any expression that evaluates to a Boolean value.
- The statement1, statement2, and statement3 can be any valid Python code.
Example
x = 0
print("x is a positive number.") if x > 0 else print("x is zero.") if x == 0 else print("x is a negative number.")
# Output:
# x is zero.
This is the same as:
x = 0
if x > 0:
print("x is a positive number.")
elif x == 0:
print("x is zero.")
else:
print("x is a negative number.")
Output:
x is zero.
Short Circuit Evaluation
When using logical operators such as and and or in your if…else statements, Python employs a technique called short circuit evaluation to optimize the evaluation process. This means that Python evaluates conditions from left to right and stops evaluating them as soon as the outcome is determined.
Let’s put it another way, the and and or operators use a short-circuit evaluation technique. This means that if the first condition in an and expression is false, the second condition is not evaluated. Similarly, if the first condition in an or expression is true, the second condition is not evaluated.
Short circuit evaluation can be used to your advantage when writing if…else statements, as it can help you avoid unnecessary computation and potential errors.
Example
x = 0
y = 2
# Using 'and'. If the first argument is False, the entire statement is False
if x and y:
print("This won't print.")
# Using 'or'. If the first argument is True, the entire statement is True
if x or y:
print("This will print.")
In the first example, Python doesn’t need to evaluate the second argument of the and expression (y), because x is False, and False and anything is False. Therefore, the “This won’t print.” line will not be printed.
Since x is False in the second example, Python only needs to determine whether y is True in order for the complete expression to be True. The “This will print.” line will be printed because y is True.
Python Operators (And, Or, Not)
In Python, you can use multiple conditions in if statements by using logical operators such as and, or, and not. Here’s a brief explanation of each:
- and: Both conditions must be true for the whole expression to be true.
- or: At least one of the conditions must be true for the whole expression to be true.
- not: Negates the truth value of the condition that follows it.
Let’s briefly cover logical operators used in Python conditional statements.
And Operator
The and operator checks if all specified conditions are true.
Example
x = 5
y = 10
if x > 0 and y > 0:
print("Both x and y are positive numbers.")
# Output:
# Both x and y are positive numbers.
In this case, since both conditions are true, the print statement is executed.
Or Operator
The or operator checks if at least one of the specified conditions is true.
Example
x = -5
y = 10
if x > 0 or y > 0:
print("At least one of x or y is a positive number.")
# Output:
# At least one of x or y is a positive number.
Here, since one of the conditions is true (y > 0), the print statement is executed.
Not Operator
The not operator negates the truth value of the condition.
Example
x = -5
if not x > 0:
print("x is not a positive number.")
# Output:
# x is not a positive number.
In this case, the condition x > 0 is false. However, the not operator negates this, making the overall condition true, so the print statement is executed.
You can combine multiple logical operators in a single if statement but be careful. This can make your code harder to read and understand. To avoid this, use parentheses to group conditions and control the order of evaluation.
Conclusion
The Python if…else statement is a fundamental and powerful for controlling the flow of your code. It is essential to master conditional statements to become a proficient Python programmer. By understanding the syntax and best practices for using if…else statements, as well as how to combine them with loops, functions, list comprehensions, and ternary operators, you can write more efficient and readable code.