Python is an incredibly versatile and powerful programming language, used by programmers worldwide for various applications. When writing code, it’s essential to document your work and make it easier for others (or even yourself) to understand. One way to achieve this is through the use of Python comments. In this article, we will discuss:
Table of Contents | |
In Python, comments are text that is not executed by the interpreter. They serve as a way for programmers to explain their code, make notes, or temporarily disable parts of the code without deleting it. Python supports two types of comments: single-line comments and multi-line comments.
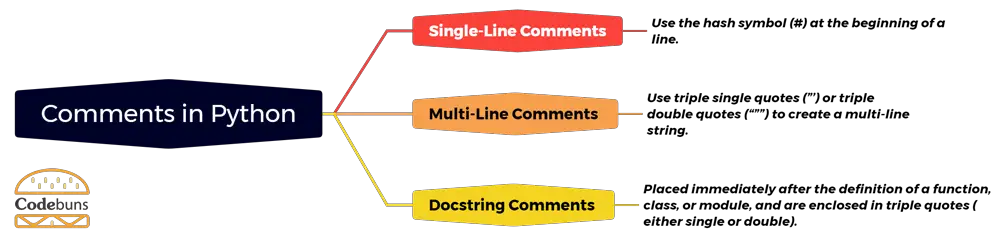
Python Single-Line Comments
Single-line comments are used to provide explanations or notes within the code, making it more readable and maintainable. To create a single-line comment, you can use the hash symbol (#) at the beginning of a line, followed by the text of the comment. The Python interpreter ignores everything after the hash symbol on that line, treating it as a comment.
Here’s an example of a single-line comment in Python:
# This is a single-line comment print("Python Comments")
In this example, the interpreter will ignore the text after the hash symbol, and only execute the print statement.
Python Multi-Line Comments
Multi-line comments are useful for providing more detailed explanations or for temporarily disabling a block of code during development. While Python doesn’t have a dedicated syntax for multi-line comments, you can use triple single quotes (”’) or triple double quotes (“””) to create a multi-line string, which can serve as a multi-line comment. Since the string isn’t assigned to a variable or used in an expression, it will be ignored by the interpreter.
Here’s an example of a multi-line comment in Python:
Triple Single Quotes Example
''' This is a multi-line comment ''' print("Python Single Quote Comments")
Triple Double Quotes Example
""" This is a multi-line comment. It can span multiple lines and provide more detailed explanations. """ print("Python Double Quote Comments")
In this example, the interpreter will ignore the text between the triple double quotes or triple double quotes and only execute the print statement.
It’s important to note that using docstrings (triple-quoted strings) to provide explanations for functions, classes, and modules is a common practice in Python. While they are not strictly comments, they serve a similar purpose. In general, it’s better to use consecutive single-line comments if you want to include a lengthy comment in your code.
Here is an example:
# first line # second line # third line print("Consecutive Single-Line Comments")
In this case, every line is handled as a single comment, and all of them are ignored.
Python Docstring Comments
Docstring comments, or simply docstrings, are a specific type of comment in programming languages like Python that are used to provide detailed documentation for functions, classes, and modules. They serve as a convenient way to describe the purpose, usage, and behavior of code components, making it easier for other developers to understand and maintain the code.
Docstrings are placed immediately after the definition of a function, class, or module, and are enclosed in triple quotes (either single or double), like this:
Example
def multiply(a, b): """ Multiply two numbers and return the result. Args: a (int): The first number. b (int): The second number. Returns: int: The multiplication of a and b. """ return a * b
Here’s a detailed breakdown of the docstring components:
- Brief description: The first line provides a short, concise explanation of what the function or class does.
- Extended description (optional): Following the brief description, you can add a more detailed explanation, if necessary. This part is optional, and it can span multiple lines if needed.
- Arguments (Args): This section documents the input parameters, or arguments, of the function. For each argument, provide its name, type, and a brief description of its purpose.
- Returns: This section describes the expected output of the function, including the type and a description of the returned value.
- Raises (optional): If the function or method can raise any exceptions, this section is used to document them. This part is also optional.
Different Types of Comments in Python
Comments in Python can be categorized into the following types:
Descriptive Comments
These comments explain the purpose of a specific line or block of code. They are useful for providing context and making the code more readable.
Example
# Calculate the area of a circle area = 3.14159 * (radius ** 2)
Inline Comments
These are short comments placed on the same line as the code they describe. They should be used sparingly and only when necessary to clarify complex or non-obvious code.
Example
x += 1 # Increment x by 1
Function/Method Comments
These comments describe the purpose, parameters, and return values of a function or method. They are typically written as docstrings (multi-line comments) immediately following the function or method definition.
Example
def addition(a, b): """ Adds two numbers and returns the result. Parameters: a (int): The first number to add b (int): The second number to add Returns: int: The sum of the two numbers """ return a + b
Class Comments
These comments provide a high-level overview of a class, explaining its purpose, properties, and methods. Like function/method comments, they are typically written as docstrings.
Example
class Circle: """ Represents a circle with a specified radius. Attributes: radius (float): The radius of the circle """ def __init__(self, radius): self.radius = radius
Python Commenting Best Practices
To ensure your Python comments are effective and beneficial, follow these best practices:
- Use descriptive comments for complex or non-obvious code: If a particular line or block of code is difficult to understand at first glance, use a comment to provide a brief explanation.
- Limit the use of inline comments: Inline comments can make your code appear cluttered, especially if used excessively. Reserve them for cases where the code’s purpose or behavior is unclear.
- Write clear and informative docstrings for functions, methods, and classes: Include information about the purpose, parameters, and return values. This makes your code more readable and easier to maintain.
- Comment out code sparingly: If you need to temporarily disable a portion of your code, use comments to do so. However, avoid leaving large amounts of commented-out code in your final project, as it can be confusing and distracting.
- Avoid redundant comments: Don’t write comments that simply restate what the code is doing. Instead, focus on providing context or explaining the reasoning behind the code.
Python Commenting Worst Practices
To avoid creating confusing or unhelpful comments, steer clear of these worst practices:
- Writing overly verbose comments: Lengthy comments can be difficult to read and may cause the reader to lose focus. Aim for brevity and clarity in your comments.
- Using cryptic or ambiguous language: Comments should be easy to understand, even for someone who is not intimately familiar with your code or the problem it solves. Avoid using jargon, slang, or abbreviations that may be unclear to the reader.
- Commenting on every line or block of code: Excessive commenting can make your code harder to read and maintain. Focus on commenting only where it adds value or clarity.
- Ignoring outdated or incorrect comments: Failing to update comments when the code changes can lead to confusion and incorrect assumptions about how the code works. Regularly review and update your comments to ensure they remain accurate and relevant.
- Using comments to justify bad code: Instead of using comments to explain or justify poorly written code, take the time to refactor and improve the code itself. Comments should not be a substitute for writing clear, efficient, and maintainable code.
Conclusion
In conclusion, writing effective and informative comments is an essential skill for any Python programmer. By following the best practices and avoiding the worst practices outlined in this article, you can make your code more readable, maintainable, and understandable for others (and yourself). Remember to focus on clarity, brevity, and relevance when crafting your comments, and your Python code will be easier to work with and appreciate by everyone who encounters it.