Python dictionaries are like a treasure map: they store data in key-value pairs, where the key is like the name of the treasure and the value is like the location of the treasure. This makes dictionaries very efficient for storing and retrieving data, as you can quickly look up a value by its key. Dictionaries are also very flexible, as you can add, remove, and update key-value pairs as needed.
If you are new to Python, dictionaries can be a bit tricky to understand at first. However, once you get the hang of them, you will find that they are a very powerful tool that can be used to solve a wide variety of problems.
Table of Contents | |
What is a Python dictionary?
A Python dictionary is a built-in data type in Python that is mutable and unordered. It’s designed to hold key-value pairs, where each key is unique and associated with a value. A key can be any immutable type, such as strings, numbers, or tuples, whereas values can be of any type including mutable and immutable types.
Python dictionary data structure is similar to “associative arrays” in other languages. One of its key features is the ability to quickly retrieve, insert, delete, or update values using their corresponding keys.
Basic syntax to define a Python dictionary:
dict_name = { "key1": "value1", "key2": "value2", "key3": "value3", # ... }
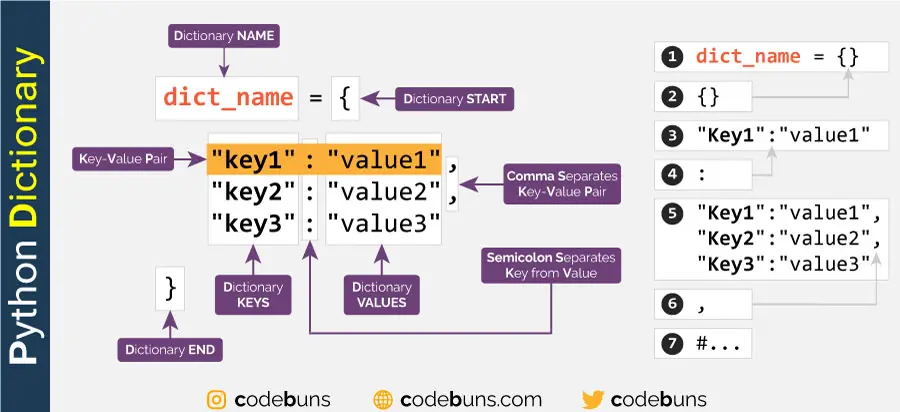
Explanation
- This line initializes a new dictionary in Python and assigns it to the variable named dict_name.
- The dictionary is enclosed in curly braces ({}). Curly braces mark the start and end of the dictionary.
- A key-value pair is the primary way data is stored in a dictionary. Each pair consists of a key and a value. In the example provided, “key1” is the key and “value1” is the value.
- A Semicolon (:) separates the key from its associated value.
- These are additional key-value pairs following the same pattern as the first.
- A comma (,) separates each pair.
- This line is a comment in Python. It indicates that the pattern of key-value pairs could continue.
Restrictions on Python Dictionary Keys and Values
Python dictionaries are a flexible and powerful data type. However, there are some restrictions on what can be used as dictionary keys and values.
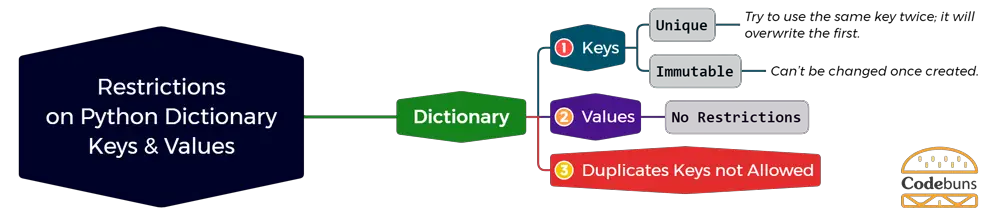
Dictionary Keys
Unique: Keys in a Python dictionary must be unique. If you try to use the same key twice, the second value will overwrite the first. No error will be raised in this case.
Immutable: Keys in a Python dictionary must be immutable, meaning they can’t be changed after they’re created. Immutable types in Python include integers, floats, strings, tuples, and frozensets. Mutable types (like lists, sets, or other dictionaries) can’t be used as dictionary keys because they can be modified in place and thus their hash values can change.
Dictionary Values
No restrictions: Values in Python dictionary can be any type of object and there are no restrictions on what types can be used. This includes mutable types, like lists or dictionaries, and immutable types, like integers, strings, or tuples. Values in a dictionary do not need to be unique.
Example of a Python dictionary that illustrates these restrictions:
# defining the dictionary my_dict = { 'name': 'Jim Brown', # string key, string value 32: 'Answer', # integer key, string value (1, 2): [4, 5], # tuple key, list value } print(my_dict)
In this example, the dictionary my_dict is initially created with three entries. The keys are a string (‘name’), an integer (32), and a tuple ((1, 2)) – all of which are immutable types. The values are a string (‘Jim Brown’), another string (‘Answer’), and a list ([4, 5]) – showcasing the flexibility of values in a dictionary.
# trying to use a list as a key, this will raise an error try: my_dict[[1, 2]] = 'value' except TypeError as error: print(error)
When we attempt to use a list ([1, 2]) as a key, a TypeError is raised because lists are mutable and can’t be hashed, and thus they cannot be used as dictionary keys.
Example2
# Valid dictionary valid_dict = {1: "one", 2: "two", 3: "three"} # Invalid dictionary: Repeated keys invalid_dict = {1: "one", 1: "two", 2: "two"}
Duplicates Not Allowed
Here’s an example of a Python dictionary:
student_grades = {"Alice": 90, "Bob": 85, "Charlie": 88}
In this example, “Alice“, “Bob“, and “Charlie” are keys and 90, 85, and 88 are their corresponding values. Now, suppose you try to add another entry with “Alice” as a key:
student_grades = {"Alice": 90, "Bob": 85, "Charlie": 88, "Alice": 95}
In this case, the second “Alice” will overwrite the first “Alice“. So, the resulting dictionary will be:
{"Alice": 95, "Bob": 85, "Charlie": 88}
The original grade for “Alice” (90) is lost and replaced by the new value (95). This is because Python dictionaries do not allow for duplicate keys. Each key must be unique. If a duplicate key is found during the dictionary creation, the last assignment will overwrite the previous ones. This is an important characteristic to remember when working with dictionaries in Python.
Creating a Python Dictionary
Python dictionaries can be created using the {} or dict().
Creating a Python Dictionary with {}
(Curly Braces)
Creating a Python dictionary with {} involves defining a set of unique keys and their corresponding values enclosed in curly braces {}. Each key-value pair is separated by a colon (:), and the pairs are divided by commas. The created dictionary can be accessed and manipulated using various Python built-in methods and operations.
Syntax
dict_name = {'key1': 'value1', 'key2': 'value2', ...}
Explanation
- dict_name: Name of the dictionary you want to create.
- {}: The dictionary is initialized using curly braces.
- ‘key’: Represents the unique key in the dictionary.
- ‘value’: Associated value with each key.
Example
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'grape': 'purple'} print(fruit_colors) # Outputs {'apple': 'red', 'banana': 'yellow', 'grape': 'purple'}
Creating a Python Dictionary with dict()
Function
Creating a Python dictionary with the dict() function involves instantiating a dictionary object using this built-in Python function. The dict() function provides a flexible and dynamic way to create dictionaries either from key-value pairs, a list of tuples, or other iterable objects.
Syntax
dict_name = dict(key1='value1', key2='value2', ...)
Or
dict_name = dict([('key1', 'value1'), ('key2', 'value2'), ...])
Above syntax allows you to create a dictionary in two common ways: by directly passing key-value pairs or by passing an iterable containing pairs of items. These dictionaries are mutable and can hold different types of data, including numbers, strings, lists, and other dictionary objects, and can be modified, manipulated, or accessed according to needs.
Explanation
- dict(): The dict() function is used to create the dictionary.
- key=’value’: It represents a key-value pair in the dictionary.
- (‘key’, ‘value’): In the second syntax, the key-value pairs are represented as tuples inside a list.
Example1
fruit_colors = dict(apple='red', banana='yellow', grape='purple') print(fruit_colors) # Outputs {'apple': 'red', 'banana': 'yellow', 'grape': 'purple'}
Example2
fruit_colors = dict([('apple', 'red'), ('banana', 'yellow'), ('grape', 'purple')]) print(fruit_colors) # Outputs {'apple': 'red', 'banana': 'yellow', 'grape': 'purple'}
In both methods, the dictionaries store data as key-value pairs.
The dict()
Constructor
You can also create a dictionary using the dict() constructor.
fruits = dict(Apple=50, Banana=100, Cherry=150)
Python Dictionary dict()
Constructor
The dict() constructor in Python creates a dictionary. Dictionaries in Python are a type of data structure that allows you to store key-value pairs. The keys in a dictionary must be unique, and the key-value pairs are unordered.
Syntax
dict(keyword arguments)
Or
dict(iterable)
Or
dict(mapping)
Explanation:
- keyword arguments: Pairs of values separated by an equal sign (=). The name of the argument becomes the dictionary key and the value becomes the dictionary value.
- iterable: This can be a list, set, or any other iterable Python object which contains pairs of values that can be used as key-value pairs in the dictionary. The first item of each pair becomes the dictionary key, and the second item becomes the dictionary value.
- mapping: Another dictionary or a type of mapping object. The keys and values of the mapping object become the keys and values of the new dictionary, respectively.
Example:
Here’s an example of using dict() with keyword arguments:
person = dict(name="John", age=30, city="New York") print(person) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
In this example:
- We’re using the dict() constructor with keyword arguments to create a dictionary.
- The names of the arguments (name, age, city) become the keys in the dictionary.
- The values of the arguments (“John“, 30, “New York“) become the values in the dictionary.
- The print() function is used to display the contents of the dictionary.
We can also create a dictionary from an iterable of pairs. For example:
list_of_pairs = [("one", 1), ("two", 2), ("three", 3)] numbers = dict(list_of_pairs) print(numbers) # Output: {'one': 1, 'two': 2, 'three': 3}
In this example:
- We start with a list of pairs, where each pair contains a string and a number.
- We use the dict() constructor with the list of pairs to create a dictionary.
- The first element of each pair becomes a key in the dictionary, and the second element of each pair becomes the corresponding value.
- The print() function is used to display the contents of the dictionary.
Add Items to a Python Dictionary
Adding items to a Python dictionary is quite straightforward. Here’s how you do it:
dictionary_name[key] = new_value
Above is the syntax used for creating a new key-value pair in a dictionary. Let’s break it down:
- dictionary_name: Name of the dictionary you’re working with.
- [key]: This represents the key for which you want to set a value. The key should be unique in each dictionary. In Python, dictionary keys can be of any immutable type, like integers, strings, tuples etc.
- new_value: This is the value you want to set for the key. Unlike keys, dictionary values can be of any type, including mutable types.
Example
# Creating an empty dictionary my_dict = {} # Adding a key-value pair to the dictionary my_dict["fruit"] = "apple" print(my_dict) # Output {'fruit': 'apple'}
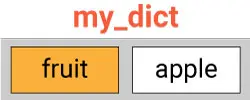
This example shows you how to add a key-value pair to a dictionary. Here’s a step-by-step walkthrough:
- my_dict = {} : An empty dictionary is created and assigned to the variable my_dict.
- my_dict[“fruit”] = “apple” : This line adds a key-value pair to the dictionary my_dict. The key is “fruit” and the associated value is “apple”.
- print(my_dict) : This line prints the contents of the dictionary my_dict to the console.
- Finally, the output {‘fruit’: ‘apple’} shows the current state of the dictionary after executing the code. It has one key-value pair where “fruit” is the key and “apple” is its corresponding value.
You can use second method, which is quite convenient. With this method, values can be assigned all at once. This syntax is useful when you are creating a python dictionary of a known size and want to quickly specify the initial values.
This action is usually done by redefining the dictionary variable entirely with a new set of key-value pairs.
Example
fruits = {"apple": 1, "banana": 2, "cherry": 3} print(fruits) # Output {'apple': 1, 'banana': 2, 'cherry': 3}
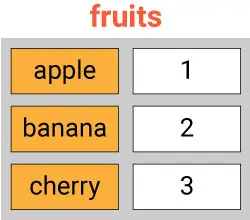
In this example, fruits is the name of the dictionary. “apple”, “banana”, “cherry” are the keys and 1, 2, 3 are their corresponding values. This dictionary assignment happens all at once, assigning the three key-value pairs to fruits in one line of code.
Update Python Dictionary Items
To update Python dictionary items, there are several methods you can use. Here’s a summary of the primary methods:
Direct Assignment
Direct assignment is a method used to assign a specific value to a particular key in a Python dictionary. This direct assignment operation is essential for modifying dictionaries in Python.
Syntax
dictionary_name[key] = new_value
Above syntax is used to add a new key-value pair to a dictionary or update the value for an existing key in the dictionary.
Example
# Initializing a dictionary my_dict = {"apple": 1, "banana": 2} print(my_dict) # Output: {'apple': 1, 'banana': 2} # Using the syntax to add a new key-value pair my_dict["cherry"] = 3 print(my_dict) # Output: {'apple': 1, 'banana': 2, 'cherry': 3} # Using the syntax to update the value of an existing key my_dict["apple"] = 4 print(my_dict) # Output: {'apple': 4, 'banana': 2, 'cherry': 3}
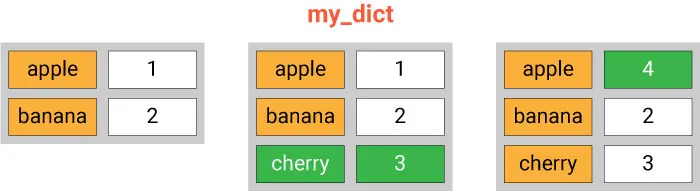
In the example above, we first create a dictionary my_dict with two key-value pairs. We then use the dictionary[key] = new_value syntax to add a new key-value pair with the key “cherry” and the value 3. Lastly, we use the same syntax to update the value of the key “apple” to 4.
Using the update()
method
The update() method in Python is an inbuilt function that adds or updates existing key-value pairs in a dictionary. If a key is already present in the dictionary, the update() function will update its value; if the key is not present, the method will add the key-value pair to the dictionary.
Syntax
dictionary_name.update({key: value})
This is another way of adding a key-value pair to a dictionary. The update() method takes in a dictionary as an argument. The dictionary inside the parentheses is the key-value pair you want to add to your dictionary.
When you use .update({key: value}), you are essentially creating a new temporary dictionary with {key: value}, and then merging this temporary dictionary into dictionary_name. If key already exists in dictionary_name, the value for that key in the dictionary is updated to the new value. If key does not already exist, a new key-value pair is added to the dictionary.
Example
Let’s consider a Python dictionary called “fruit_basket” that represents different fruits and their respective quantities.
fruit_basket = {"apple": 5, "banana": 4, "grape": 10}
Now, suppose we have just bought some oranges and we want to add them to our fruit basket. Using the update() method, we can add the key-value pair “orange: 3” to the fruit_basket dictionary like this:
fruit_basket.update({"orange": 3})
After this line of code executes, the fruit_basket dictionary will become:
print(fruit_basket) # Output # {'apple': 5, 'banana': 4, 'grape': 10, 'orange': 3}
Using dictionary comprehension
Python dictionary comprehension is a powerful and concise way to create dictionaries in Python. This technique uses a single line of code to create a new dictionary by iterating over an iterable, applying an optional condition, and associating keys and values. It’s syntactically similar to python list comprehension, but with key-value pairs.
Syntax
{key_expression : value_expression for item in iterable}
Explanation
- { } are used to denote a dictionary.
- key_expression is an expression that forms the key of the dictionary.
- value_expression is an expression that forms the value for the keys in the dictionary.
- for item in iterable is a loop that iterates over each element in the iterable.
Additional conditionals can be added:
{key_expression : value_expression for item in iterable if condition}
if condition is used to apply a condition for filtering items.
Suppose you have a list of numbers and you want to create a dictionary where the numbers are keys and their squares are values.
Example
numbers = [1, 2, 3, 4, 5] squares = {number: number**2 for number in numbers} {k: new_value if k == key else v for k, v in dictionary.items()} print(squares) # Output # {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example:
- numbers is our iterable.
- number is the item taken from the iterable each iteration.
- number (before the colon) is our key_expression which is the same as the item.
- number**2 is our value_expression, which squares the item.
- The result is a dictionary where each key is a number from the list and each value is the square of the respective number.
Conditionals can be used to filter out some elements. For example, to include only odd numbers:
Example2
numbers = [1, 2, 3, 4, 5] odd_squares = {number: number**2 for number in numbers if number % 2 != 0} print(odd_squares) # Output # {1: 1, 3: 9, 5: 25}
In this example, only the squares of the odd numbers are included due to the if number % 2 != 0 condition.
Access Python Dictionary Items
You can access a dictionary item by its key or use the get() method.
Accessing by key
This is the most straightforward way to access an item in a dictionary. You simply call the key within square brackets after the dictionary name.
Here’s the general syntax for dictionary value access:
dictionaryName[keyName]
Explanation
- dictionaryName is the name of the dictionary you’re working with.
- keyName is the key whose corresponding value you want to access from the dictionary.
If you use this syntax and the key is present in the dictionary, Python will return the value that is associated with that key. If the key is not present in the dictionary, Python will raise a KeyError exception.
Example
# Define a dictionary my_dict = {'name': 'John', 'age': 30, 'job': 'Developer'} # Access a value by key print(my_dict['name']) # Output: John
In the example:
- A dictionary my_dict is defined with three key-value pairs.
- The line
print(my_dict['name'])
accesses the value associated with the key ‘name’ in the dictionary my_dict, and prints that value to the console. The output of this line is John because ‘John’ is the value associated with the key ‘name’ in the dictionary.
Using the get()
method
The Python Dictionary get() method is a built-in function that retrieves the value of a specified key from a dictionary. This method provides a way to safely access the value associated with a key without raising a KeyError if the key does not exist, which would be the case if you tried to access the key directly.
Syntax
dictionary_name.get('key')
or
dictionary_name.get('key', default_value)
or
dictionary.get(key, default=None)
Takes two parameters: the key to be looked up, and an optional default value that is returned if the key is not found in the dictionary. By default, if the key is not present in the dictionary and no default value is provided, the get() method returns None.
Example
# Defining a Python dictionary student = {'name': 'John', 'age': 22, 'grade': 'A'} # Accessing dictionary items using get() print(student.get('name')) # Outputs: John # Accessing a non-existent key with a default value print(student.get('major', 'Not Found')) # Outputs: Not Found
In the above example:
- student is our Python dictionary.
- We used get(‘name’) to access the value associated with the key ‘name’ in the dictionary, which returns ‘John’.
- We used get(‘major’, ‘Not Found’) to attempt to access the value associated with the key ‘major’. Since ‘major’ doesn’t exist in our dictionary, it returns the default value ‘Not Found’.
Remove Python Dictionary Items
You can remove items from a dictionary using the del keyword, pop() method, and clear() method.
Syntax for removing items from a Python dictionary.
Using the del
statement
The del statement in Python is used to delete items from a dictionary. This keyword removes the key-value pair corresponding to the specified key from the dictionary, if it exists. If you try to use it with a key that’s does not present in the dictionary, Python will raise a KeyError. To avoid this, it’s common to check if the key exists in the dictionary before trying to delete it.
Syntax
del dictionary[key]
Using the pop()
method
The Python Dictionary pop() method is a built-in function that removes an item with the provided key and returns the value of the item.
Syntax
dictionary.pop(key[, default])
Takes two parameters: the key of the element to be removed and an optional default value. If the key is found in the dictionary, the method removes the key-value pair and returns the value. If the key is not found and a default value is specified, the method returns the default value. However, if the key is not found and no default value is provided, it raises a KeyError.
Using the popitem()
method
The Python Dictionary popitem() method is a built-in function that removes and returns the last element (key-value pair) as a tuple from the dictionary. The items are returned in a LIFO (Last In First Out) order, which has been a standard behavior since Python 3.7. If the dictionary is empty, calling popitem() will raise a KeyError.
Syntax
dictionary.popitem()
Using the clear()
method
The Python Dictionary clear() method is a built-in function that removes all items, i.e., key-value pairs, from a given dictionary, thereby leaving it empty. The method does not take any parameters nor return any value. After invoking clear(), the size of the dictionary becomes zero, but the dictionary itself remains, now just as an empty dictionary. The operation does not delete the dictionary object, but merely clears its content.
Syntax
dictionary.clear()
Example
# Initialize a dictionary my_dict = {'Name': 'Zophie', 'Species': 'cat', 'Age': 7} # Use del to remove an item del my_dict['Age'] print(my_dict) # Outputs: {'Name': 'Zophie', 'Species': 'cat'} # Use pop to remove an item and get its value popped_item = my_dict.pop('Species') print(popped_item) # Outputs: 'cat' print(my_dict) # Outputs: {'Name': 'Zophie'} # Use popitem to remove the last item (or an arbitrary item in older Python versions) last_item = my_dict.popitem() print(last_item) # Outputs: ('Name', 'Zophie') in Python 3.7 and later # Clear the dictionary my_dict.clear() print(my_dict) # Outputs: {}
We first create a dictionary with three items. We then remove items using del, pop, and popitem, and finally, we clear the entire dictionary.
Python Dictionary Length
The Python Dictionary len() function is a built-in function in Python that is used to return the number of key-value pairs in a dictionary. It accepts the dictionary as its argument and gives the total count of items present in the dictionary as output. If the dictionary is empty, the len() function will return 0.
Syntax
len(dictionary_name)
dictionary_name is the name of the dictionary whose length you want to determine.
Example
fruits = {"Apple": 50, "Banana": 100, "Cherry": 150} print(len(fruits)) # Output: 3
When you run this code, the output will be 3, indicating that there are 3 key-value pairs in the dictionary, which represents the 3 fruits.
Operators in
and not
with Python Dictionary
You can use in and not operators with a dictionary to check if a key is present.
fruits = {"Apple": 50, "Banana": 100, "Cherry": 150} print("Apple" in fruits) # Output: True print("Mango" not in fruits) # Output: True
in
Operator with Python Dictionary
The “in” operator in Python checks if a key is present in the dictionary. It returns True if the key is in the dictionary, and False otherwise.
Syntax
key in dictionary
When the in operator is used, Python checks only in the dictionary keys, not the values.
not
Operator with Python Dictionary
The “not” operator checks if a key is not present in the dictionary. It returns True if the key is not in the dictionary, and False otherwise. It operates by scanning through the keys of the dictionary, and does not consider the values.
Syntax
key not in dictionary
Example
# Define a dictionary person = { "name": "Alice", "age": 30, "city": "New York" } # Check if "name" is in the dictionary if "name" in person: print("'name' is in the dictionary.") else: print("'name' is not in the dictionary.") # Check if "height" is not in the dictionary if "height" not in person: print("'height' is not in the dictionary.") else: print("'height' is in the dictionary.")
If you run the above code, it would print:
# Output # 'name' is in the dictionary. # 'height' is not in the dictionary.
This is because name is a key in the person dictionary, and height is not.
Built-in Python Dictionary Functions & Methods
Python provides a number of built-in functions and methods for dictionaries.
Using the keys()
method
The “keys()” method in a Python Dictionary is a built-in function that returns a view object containing the dictionary’s keys. This object can be iterated over, used in for loops, or converted to a list or set to perform operations that require a sequence. It does not take any parameters and the keys are presented in the order they were first added to the dictionary. If a dictionary’s keys are modified while iterating over this view object, the changes will be reflected in the view as well.
Syntax
dictionary.keys()
Note that the returned object is a view, not a separate list, so it doesn’t involve creating a new collection of keys.
Example
person = {'name': 'John', 'age': 30, 'city': 'New York'} keys = person.keys() print(keys)
Explanation
- person dictionary with keys ‘name’, ‘age’, and ‘city’.
- We use keys = person.keys() to retrieve the keys from the dictionary and assign them to the variable keys.
- Then we print the keys, which will output: dict_keys([‘name’, ‘age’, ‘city’])
Using the values()
method
The Python Dictionary values() method is a built-in function in Python that returns a view object containing the values of the dictionary. The returned values object is not a list, but it can be converted to a list or iterated over using loops. This method is primarily used for accessing all the values in a dictionary without referring to their respective keys.
Syntax
dictionary.values()
Note that the order of the values will match the order in which the keys were inserted into the dictionary. However, if any modifications are made to the dictionary, the view object will be dynamically updated, reflecting those changes.
Example
person = { "name": "John", "age": 30, "country": "USA" } print(person.values())
In this example, the dictionary person has three keys: “name“, “age“, and “country“, each with their respective values. The values() method is used to print all the values of the dictionary, so the output will be:
# Output # dict_values(['John', 30, 'USA'])
This is a dict_values object, which is an iterable view on the dictionary’s values. You can convert this view into a list by using the list() function if you need to.
Using the items()
method
The Python Dictionary items() method is a built-in function that returns a view object, which is a dynamic set-like object containing all the dictionary’s key-value tuple pairs. This view object allows for iteration over its elements, and reflects any changes to the dictionary in real-time. It’s typically used in loops or other iterative contexts where both the keys and values of the dictionary are required.
Syntax
dictionary.items()
The items() method doesn’t take any parameters.
Example
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3} print(my_dict.items()) # Output # dict_items([('apple', 1), ('banana', 2), ('cherry', 3)])
Explanation
- We first define a dictionary called “my_dict” with three key-value pairs.
- When we call my_dict.items(), it returns a dict_items object that includes all of the dictionary’s key-value pairs as tuples, where the first element of each tuple is the key and the second is the corresponding value.
- We then print this dict_items object. In our case, it’s a list-like object that contains tuples: (‘apple’, 1), (‘banana’, 2), and (‘cherry’, 3).
Using the copy()
method
The Python Dictionary copy() method is a built-in function that creates and returns a shallow copy of the existing dictionary. This method does not take any parameters. When invoked on a dictionary, it generates a new dictionary that duplicates the key-value pairs of the original dictionary. It’s important to note that this is a shallow copy, meaning that it only copies the references to the objects stored as values in the dictionary, not the objects themselves. Therefore, changes made to mutable elements (like lists or dictionaries) in the copied dictionary will also be reflected in the original dictionary, and vice versa.
For a deep copy, where even the elements inside mutable objects get their own copies, you would need to use the copy module’s deepcopy() function.
Syntax
dictionary.copy()
Example
original_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} copied_dict = original_dict.copy() print("Original Dictionary: ", original_dict) print("Copied Dictionary: ", copied_dict) # Output # Original Dictionary: {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} # Copied Dictionary: {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
Explanation
- We first create a dictionary called original_dict with keys ‘key1‘, ‘key2‘, ‘key3‘ and corresponding values ‘value1‘, ‘value2‘, ‘value3‘.
- We then use the copy() method to create a copy of original_dict, and we store this copy in a new dictionary called copied_dict.
- When we print both original_dict and copied_dict, we can see that they contain the same keys and values. This shows that copy() has successfully created a copy of the original dictionary.
Looping Through a Python Dictionary
You can loop through a dictionary by its keys, values, or items (key-value pairs).
Looping through Keys
The Python dictionary keys() method returns a view object that displays a list of all the keys in the dictionary. This method does not take any parameters and directly returns the keys stored in the dictionary.
Syntax
for key in dictionary: # process key or dictionary[key]
Explanation
- In the syntax, for starts a for loop. This type of loop repeats a block of code once for each item in a sequence or collection.
- key is a variable that takes on the value of each successive key in the dictionary for each iteration of the loop.
- in keyword in Python can be used to check if a value exists in a sequence or collection, or to iterate over a sequence or collection in a for loop.
- dictionary is the dictionary through which the loop iterates.
Example
fruits = {'apple': 1, 'banana': 2, 'cherry': 3} # Loop over the keys for key in fruits: print(f'The key is {key} and its value is {fruits[key]}')
In this example, fruits is a dictionary that maps strings to integers. The for loop iterates over each key in the fruits dictionary. For each key, it prints a formatted string that includes the key and the value associated with that key in the dictionary. The {key} and {fruits[key]} inside the f-string will be replaced by the current key and its corresponding value, respectively.
This for loop will output:
# Output # The key is apple and its value is 1 # The key is banana and its value is 2 # The key is cherry and its value is 3
In Python, when we loop through a dictionary without specifying a method, it defaults to iterating over the keys. Hence, for key in fruits
is equivalent to for key in fruits.keys()
.
Looping through Values
The values() method in Python is used to return a new view object that contains all the values of a dictionary. This method does not take any parameters and directly returns the values stored in the dictionary.
Syntax
for value in dictionary.values(): # actions
Explanation
- dictionary: It represents the dictionary through which you are looping. Replace it with the name of your dictionary.
- .values(): This is a built-in Python method specifically for dictionaries. It returns an iterable view object that displays all of the values in the dictionary.
- for value in: This is the beginning of a Python for loop. The value variable represents each value that the loop pulls from the dictionary in turn. You can use any name for this variable.
- # actions: This is where you put what you want to do with each value.
Example
student_grades = {"John": 90, "Sara": 85, "Peter": 88} # Loop over the values for grade in student_grades.values(): print(grade)
In this example, student_grades is a dictionary that maps student names (the keys) to their grades (the values).
The for loop iterates over the values in the student_grades dictionary using the .values() method. On each iteration, it assigns the current value to the variable grade and then prints the grade.
The output of this script would be:
# Output # 90 # 85 # 88
This output is the list of grades that each student received. This script doesn’t tell us which grade belongs to which student, because it only prints the values, not the keys. If we wanted to also know which student received each grade, we would need to loop through both the keys and the values of the dictionary.
Looping through Items
The Python dictionary items() method returns a view object that displays a list of dictionary’s key-value tuple pairs. This can be used for iteration or checking if a specific key-value pair is present in the dictionary.
Syntax
for key, value in dict.items(): # perform operation
Explanation
- dict is the dictionary you are looping through.
- The items() method is a built-in Python function that returns a view object. This view object contains the key-value pairs of the dictionary as tuples in a list.
- The for keyword is a loop operator in Python.
- key and value are variables that stand for the key and value elements of each key-value pair. You can actually use any names instead of key and value; these are just common choices because they’re descriptive.
- in is a keyword that checks if a value exists in the specified sequence and, in the context of a for loop, iterates over that sequence.
Example
student_grades = {"Tom": 87, "Jerry": 92, "Spike": 78} # Loop over the key-value pairs for student, grade in student_grades.items(): print(f"{student} scored {grade} marks.")
In this example, student_grades is a dictionary that contains names of students as keys and their grades as values. The for loop iterates over each key-value pair in the student_grades dictionary. On each iteration, student is the key and grade is the value of the current item. The print statement inside the loop displays a string that includes the student’s name and their grade. The f-string (formatted string literal) is a Python feature that allows for embedded expressions inside string literals, enclosed in curly braces {}.
Therefore, when this code is run, it will print the names of the students along with their grades. For instance, the first line of output would be “Tom scored 87 marks.” The loop will continue to print such statements until it has iterated through all the items in the dictionary.
The output of this script would be:
# Output # 90 # 85 # 88
Python Nested Dictionaries
A nested dictionary in Python is a dictionary within a dictionary. It’s a collection of dictionaries into one single dictionary. It allows you to store data in a structured and convenient way.
Defining a Nested Dictionary
This can be done by using curly brackets {}. The key-value pairs are separated by a colon : and each pair is separated by commas.
nested_dict = { 'dictA': {'key_1': 'value_1'}, 'dictB': {'key_2': 'value_2'} }
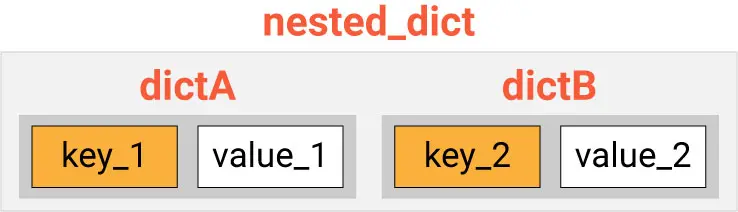
Accessing Elements
Elements can be accessed using the keys. First, you specify the outer key to get to the inner dictionary and then specify the key of the element in the inner dictionary.
print(nested_dict['dictA']['key_1']) # Outputs: 'value_1'
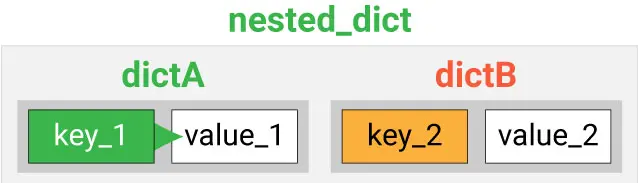
Modifying Elements
The values can be modified by using the keys.
nested_dict['dictA']['key_1'] = 'new_value'
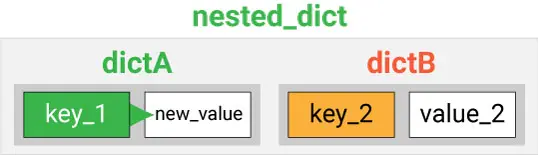
Adding Elements
You can add a new dictionary to the nested dictionary or add a new key-value pair to an existing inner dictionary.
nested_dict['dictC'] = {'key_3': 'value_3'} nested_dict['dictA']['key_4'] = 'value_4'
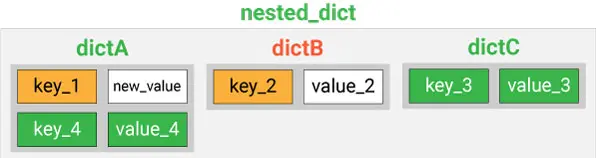
Removing Elements
del statement is used to remove an item or an inner dictionary.
del nested_dict['dictB'] del nested_dict['dictA']['key_4']
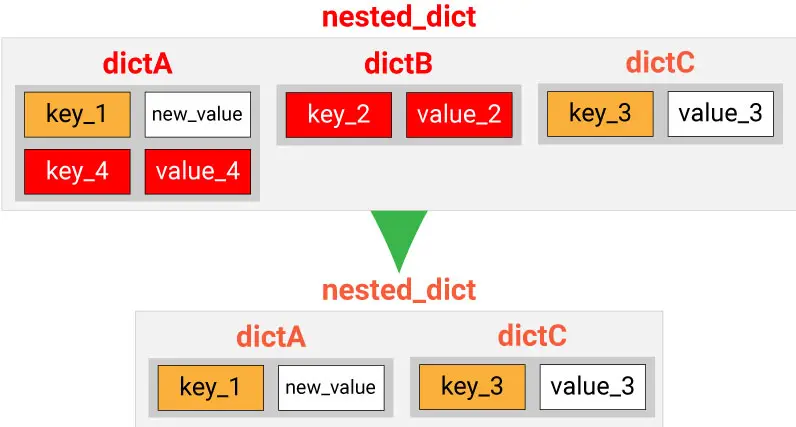
Complete Code
# Defining a Nested Dictionary nested_dict = { 'dictA': {'key_1': 'value_1'}, 'dictB': {'key_2': 'value_2'} } print(nested_dict) # Outputs: {'dictA': {'key_1': 'value_1'}, 'dictB': {'key_2': 'value_2'}} # Accessing Elements print(nested_dict['dictA']['key_1']) # Outputs: value_1 # Modifying Elements nested_dict['dictA']['key_1'] = 'new_value' print(nested_dict) # Outputs: {'dictA': {'key_1': 'new_value'}, 'dictB': {'key_2': 'value_2'}} # Adding Elements nested_dict['dictC'] = {'key_3': 'value_3'} nested_dict['dictA']['key_4'] = 'value_4' print(nested_dict) # Outputs: {'dictA': {'key_1': 'new_value', 'key_4': 'value_4'}, 'dictB': {'key_2': 'value_2'}, 'dictC': {'key_3': 'value_3'}} # Removing Elements del nested_dict['dictB'] del nested_dict['dictA']['key_4'] print(nested_dict) # Outputs: {'dictA': {'key_1': 'new_value'}, 'dictC': {'key_3': 'value_3'}}
Example2
student_data = { 'student1': { 'name': 'Alice', 'age': 20, 'grades': {'math': 90, 'science': 80, 'english': 85} }, 'student2': { 'name': 'Bob', 'age': 22, 'grades': {'math': 85, 'science': 95, 'english': 90} } } print(student_data['student1']['name']) # Outputs: Alice print(student_data['student1']['grades']['math']) # Outputs: 90
In the example above, we have a dictionary student_data, where each key is a string that identifies a student, and the corresponding value is another dictionary that stores data about the student.
In the nested dictionaries, there are keys for name, age, and grades. The value for the grades key is another dictionary that contains subject names as keys and corresponding grades as values.
You can access, modify, add, and remove elements in this nested dictionary in the same way as described in the syntax explanation. For instance, student_data['student1']['name']
would give you Alice, student_data['student1']['grades']['math']
would give you 90, you can modify these values or add more students or grades or remove any of them using the appropriate syntax. This way of storing data makes it highly readable and easy to manipulate.
Looping through Python Nested Dictionaries
Sure, I will provide an explanation and example of how to loop through nested dictionaries in Python.
Define the dictionary
First, a dictionary must be defined. In Python, dictionaries are defined with curly brackets {}. In nested dictionaries, a dictionary contains another dictionary(s) as a value(s).
nested_dict = { 'dictA': {'key_1': 'value_1'}, 'dictB': {'key_2': 'value_2'}}
Access nested dictionary
To access the nested dictionary, you need to use the key for the outer dictionary and then the key for the inner dictionary.
nested_dict['dictA']['key_1'] This will return: `'value_1'`
Loop through the dictionary
You can loop through a dictionary using a for loop.
for key, value in dictionary.items(): # do something
Loop through nested dictionaries
If a dictionary is nested, you will need to use a nested for loop.
for outer_key, outer_value in nested_dict.items(): for inner_key, inner_value in outer_value.items(): # do something
Example
nested_dict = {'first': {'a': 1, 'b': 2}, 'second': {'c': 3, 'd': 4}} for outer_key, outer_value in nested_dict.items(): print(f"Outer Key: {outer_key}") for inner_key, inner_value in outer_value.items(): print(f"Inner Key: {inner_key}, Inner Value: {inner_value}")
In this example, we have a dictionary called nested_dict which contains two other dictionaries. The for loop iterates over the outer dictionary’s keys and values (which are also dictionaries). For each outer key-value pair, we print the outer key, then start another for loop to iterate over the inner dictionary’s keys and values. For each inner key-value pair, we print the inner key and its corresponding value. This allows us to access and print all keys and values in the nested dictionary.
Python Dictionary vs List: Which Is Better?
Both Python dictionaries and lists are powerful data structures, but they are used for different purposes and have some important differences. The choice between the two often depends on your specific use case.
Python List
- A Python list is a built-in data type which can be used to store multiple items in a single variable.
- Lists are created by placing a comma-separated sequence of items inside square brackets [].
- Lists are ordered, changeable, and allow duplicate items.
- Each item in a list has an assigned index value.
- It’s important to note that the index of the first item is 0, not 1.
- Lists can contain any data type: integers, strings, other lists, etc.
- Python lists are great to use when order matters in the collection of items.
Syntax
- Declaration:
listName = [item1, item2, item3]
- Access:
listName[index]
- Update:
listName[index] = newValue
- Addition:
listName.append(item)
- Deletion:
del listName[index]
Example
# List declaration fruits = ['apple', 'banana', 'cherry'] # Accessing an item print(fruits[1]) # Output: 'banana' # Updating an item fruits[1] = 'blueberry' print(fruits) # Output: ['apple', 'blueberry', 'cherry'] # Adding an item fruits.append('dragonfruit') print(fruits) # Output: ['apple', 'blueberry', 'cherry', 'dragonfruit'] # Deleting an item del fruits[0] print(fruits) # Output: ['blueberry', 'cherry', 'dragonfruit']
Python Dictionary
- A Python dictionary is also a built-in data type which is used to store data in key/value pairs.
- Dictionaries are created by placing a comma-separated sequence of key:value pairs inside curly braces {}.
- Dictionaries are unordered, changeable, and do not allow duplicates in keys.
- The key acts as an identifier for the value, meaning you can access the value by referring to its key.
- Dictionaries can contain any data types as keys or values, but keys must be immutable.
- Python dictionaries are ideal when you need to associate a set of values with keys—to look up items by name or property, rather than by index.
Syntax
- Declaration:
dictName = {key1: value1, key2: value2}
- Access:
dictName[key]
- Update/Addition:
dictName[key] = newValue
- Deletion:
del dictName[key]
Example
# Dictionary declaration fruit_colors = {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'} # Accessing a value print(fruit_colors['banana']) # Output: 'yellow' # Updating a value fruit_colors['banana'] = 'green' print(fruit_colors) # Output: {'apple': 'red', 'banana': 'green', 'cherry': 'red'} # Adding a key-value pair fruit_colors['blueberry'] = 'blue' print(fruit_colors) # Output: {'apple': 'red', 'banana': 'green', 'cherry': 'red', 'blueberry': 'blue'} # Deleting a key-value pair del fruit_colors['apple'] print(fruit_colors) # Output: {'banana': 'green', 'cherry': 'red', 'blueberry': 'blue'}
In terms of performance, dictionary operations like retrieval, deletion, and insertion are faster because they use hashing to locate items. On the other hand, these operations can be slower with lists, especially when the list grows in size, because the search operation runs through each element in the list.
That being said, lists are more straightforward for simple, iterable collections of data, and they maintain the order of the elements. Dictionaries are more suitable when you need a logical association between a key:value pair. Also, dictionaries provide a more efficient way to look up data.
Keep in mind that neither data structure is “better” than the other; the best choice depends on the specific situation and requirements of your problem.
Conclusion
Python dictionaries are an extremely useful data structure for handling data efficiently. Understanding their nuances allows you to tackle a wide range of programming problems with ease. Keep practicing and exploring this powerful Python feature!