In this article, we’ll dive deep into one of the most fundamental constructs in programming – the while loop. Specifically, we’ll focus on the Python while loop, exploring its syntax, flow diagram, and various use cases. Let’s start now!
Table of Contents | |
In Python, loops are used for repetitive tasks. There are two types of loops in Python: for loop and while loop.
What is while Loop in Python?
In Python, a while loop is a control flow structure that allows code to be executed repeatedly based on a Boolean condition. You can think of the while loop as a repeating if statement. The code within the loop, often known as the loop’s body, is repeated as long as a given condition remains True.
It’s important to ensure that the condition eventually becomes False to prevent an infinite loop. This usually involves updating variables inside the loop that affect the condition.
Here’s an example of a simple while loop in Python:
Example
count = 0 while count < 5: print("Current count value:", count) count += 1
Note that you can combine the print and increment statements into one line (making the loop literally a “single statement while loop“):
count = 0 while count < 5: print("Current count value:", count); count += 1
Please note that although Python allows writing multiple statements on the same line using semicolons, it is not considered good practice as it affects readability of the code. Python’s philosophy emphasizes readability and simplicity, so usually it’s better to write code in the standard multiline format.
The output will be:
Current count value: 0
Current count value: 1
Current count value: 2
Current count value: 3
Current count value: 4
This loop will print the value of count and increment it by 1 in each iteration. When count reaches 5, the condition count < 5
becomes False, and the loop stops executing.
The execution order would be:
- Check if count is less than 5 (initially, it is).
- Enter the loop and print the current value of count.
- Increment the count by 1.
- Go back to step 1 and check the condition again. If true, repeat steps 2-4; otherwise, exit the loop.
Python while Loop Flow Diagram
A flow diagram visually represents the steps involved in a process, such as a programming construct like a while loop. Here’s a flow diagram for a Python while loop:
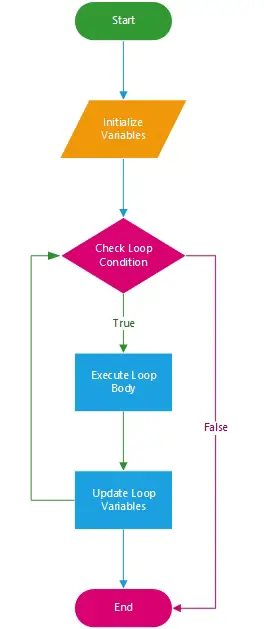
- Start: The beginning of the while loop.
- Initialize Variables: Variables used in the loop condition and the loop body are initialized.
- Check Loop Condition: The loop condition is checked. If it’s true, the loop body will be executed, and if it’s false, the loop will be exited.
- Execute Loop Body: The code inside the loop body is executed.
- Update Loop Variables: The variables used in the loop condition and the loop body are updated as required.
- End: The point where the loop finishes and the program continues with the following lines of code.
This flow will repeat steps 3-5 until the loop condition is no longer true.
Python while Loop Syntax
In Python, the syntax for a while loop is as follows:
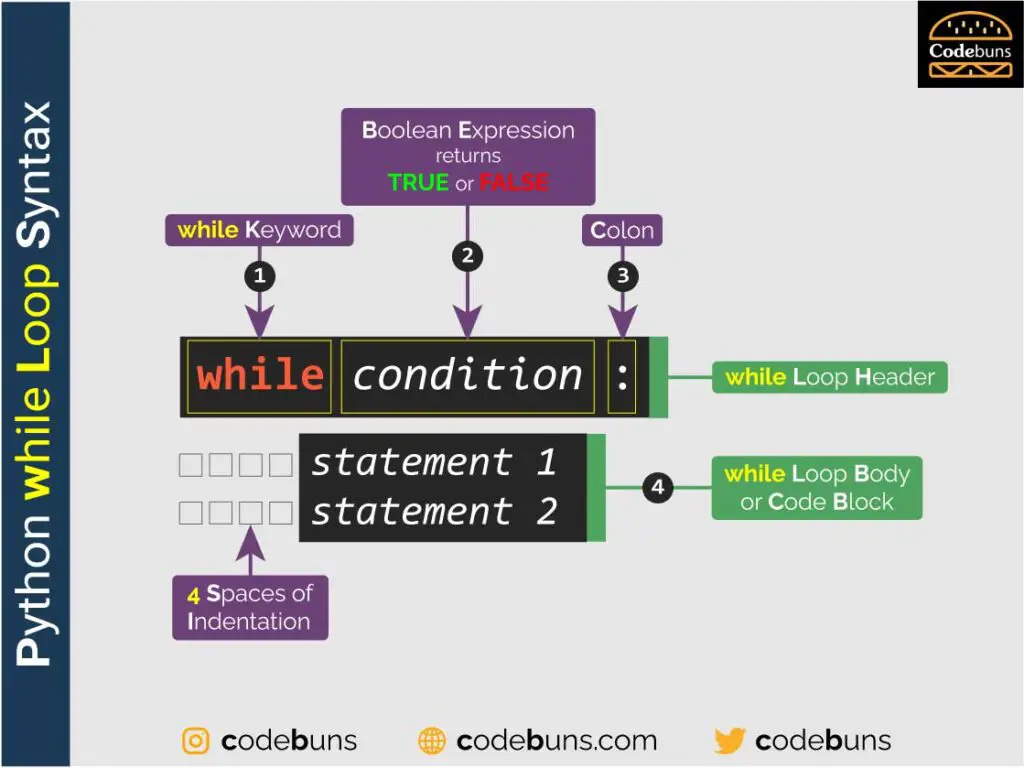
Syntax
while condition: # Code to execute while the condition is True
Here’s a breakdown of the while loop syntax:
- The keyword while indicates the start of the loop.
- The condition is a Boolean expression that is evaluated before each iteration of the loop. If the condition is true, the loop continues executing the code block. If it’s false, the loop stops and the program continues executing the code following the loop.
- A colon ( : ) indicates the end of the condition and the beginning of the code block to be executed.
- The code block to be executed should be indented, typically by 4 spaces.
Python while Loop with else Clause
In Python, the while loop can have an optional else clause which is executed when the loop terminates naturally (i.e. when the condition specified in the while statement becomes False). This is different from an else clause in an if statement, which is executed when the if condition is False.
The syntax for using the else clause in a while loop is as follows:
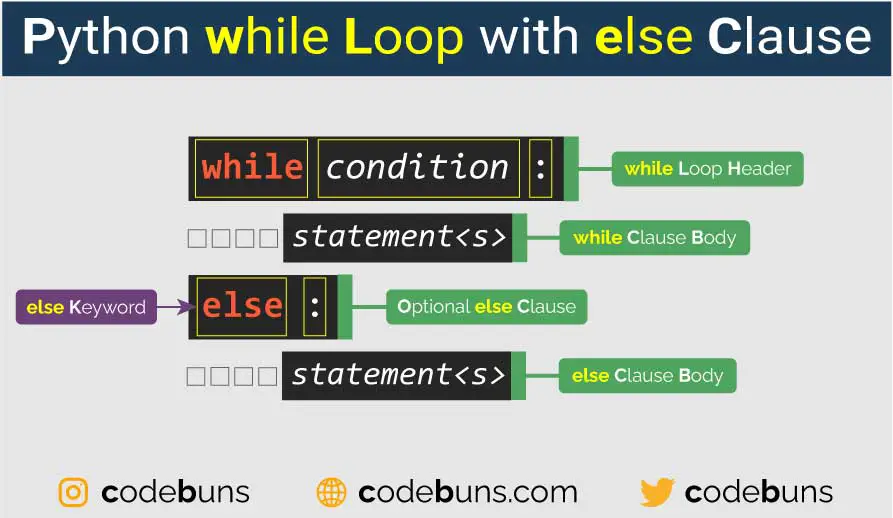
Syntax
while condition: # Code to execute while the condition is True else: # Code to execute when the condition becomes False
Here’s an example to demonstrate the use of the else clause in a while loop:
Example
count = 0 while count < 5: print(f"Count is {count}") count += 1 else: print("The loop has completed.")
Output
Count is 0
Count is 1
Count is 2
Count is 3
Count is 4
The loop has completed.
In this example, the while loop will continue executing as long as count is less than 5, printing out the current value of count in each iteration. When count reaches 5, the loop condition becomes False, and the code block in the else clause will be executed, printing “The loop has completed.”
It’s important to note that the else clause will not be executed if you use a break statement to exit the while loop. The else clause only executed when the loop terminates naturally due to its condition becoming False.
Python while Loop with break Statement
The break keyword in a Python while loop is used to exit the loop prematurely when a certain condition is met. It stops the loop iteration and continues with the next statement after the loop. The break statement is often combined with an if statement to evaluate the condition.
Here’s the syntax for using the break statement within a while loop:
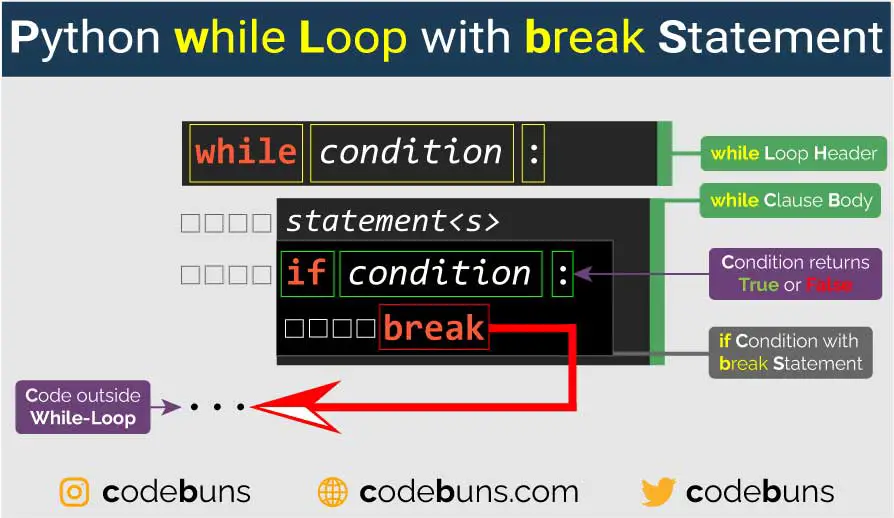
Syntax
while condition: # Your code here if condition_2: break # Your code after the break statement
In this example, the while loop will continue to execute as long as the condition is true. When the condition_2 inside the loop becomes true, the break statement will be executed, and the program will exit the loop immediately, skipping any remaining code within the loop.
Here’s a simple example demonstrating the use of the break statement within a while loop:
Example
count = 0 while True: # This creates an infinite loop count += 1 print("Count is:", count) if count >= 5: print("Breaking out of the loop!") break # Exits the loop when count is greater than or equal to 5 print("Outside the loop")
In this example, the while loop will keep running indefinitely because the condition is always True. However, the break statement will be executed when count reaches 5 or more, causing the loop to exit early. The output will be:
Count is: 1
Count is: 2
Count is: 3
Count is: 4
Count is: 5
Breaking out of the loop!
Outside the loop
The break statement can be very useful for controlling the flow of your loops based on specific conditions, especially when working with infinite loops or when the loop termination condition is not known in advance.
Python while Loop with continue Statement
In Python, the continue statement is used within a loop (such as a while loop or a for loop) to skip the remaining part of the current iteration and proceed to the next iteration of the loop. It is useful when you want to skip specific iterations based on a condition and continue with the rest of the loop.
Here’s the syntax for using the continue statement within a while loop:
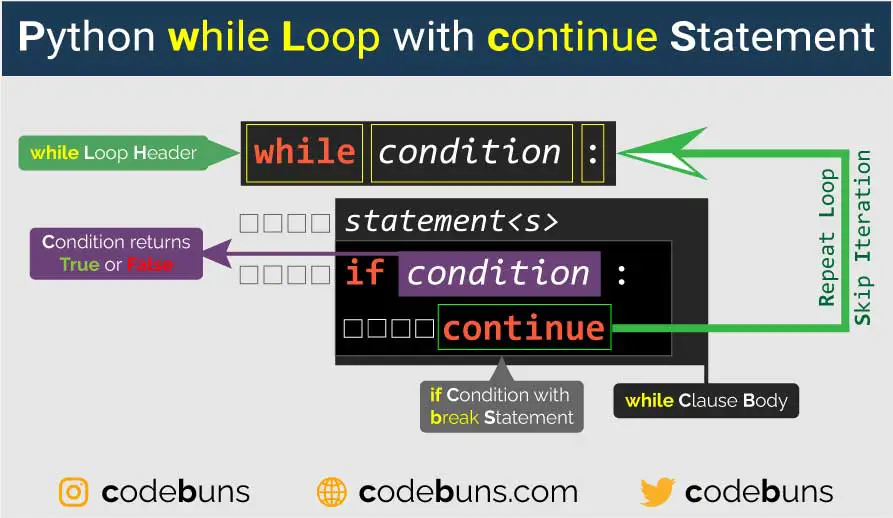
Syntax
while condition: # statement(s) if condition_2: continue # statement(s)
In this structure, if condition_2 is true, then the continue statement is executed. This will immediately skip to the next iteration of the loop, bypassing any code for the current iteration that is located beneath the continue statement.
Here’s an example of how to use the continue statement with a while loop:
Example
num = 0 while num < 10: num += 1 if num == 5: continue print(num)
In this code, the while loop will iterate from 1 to 10. However, when the value of num equals 5, the continue statement is executed, and the current iteration of the loop is terminated. This means that 5 will not be printed by the print(num)
command, as it is skipped over by the continue statement.
So, the output of the above code will be:
1
2
3
4
6
7
8
9
10
You can see that the number 5 is missing from the sequence, due to the use of the continue statement.
Python while Loop with pass Statement
In Python, the pass statement is a null operation that does nothing when it is executed. It is used as a placeholder when a statement is required syntactically, but you don’t want any action to be performed.
Here’s the syntax for using the pass statement within a while loop:
Syntax
while condition: pass
This is a while loop that does nothing as long as the condition is True. Once the condition is False, the loop will exit.
Here’s an example to illustrate this:
Example
count = 0 while count < 5: # Do nothing, just pass pass count += 1
Output: Program continues running without doing anything for 5 iterations.
In this example, the while loop will execute 5 times, but nothing will happen inside the loop because of the pass statement. The loop will still run and increment the count variable until the condition count < 5
is no longer true.
The use of pass is not necessary here, but it can help to clarify your intention that nothing should happen in a specific case.
Remember that the above example is not typical or advised; it’s just to demonstrate how pass behaves within a while loop. In a real program, you’d want your loop to do something meaningful and a pass statement is generally used as a placeholder for functionality to be added in the future.
Nested Python while Loops
In Python, you can nest one loop inside another loop, which means you can have a while loop inside another while loop. This is called nested looping.
The syntax for nested while loops in Python is as follows:
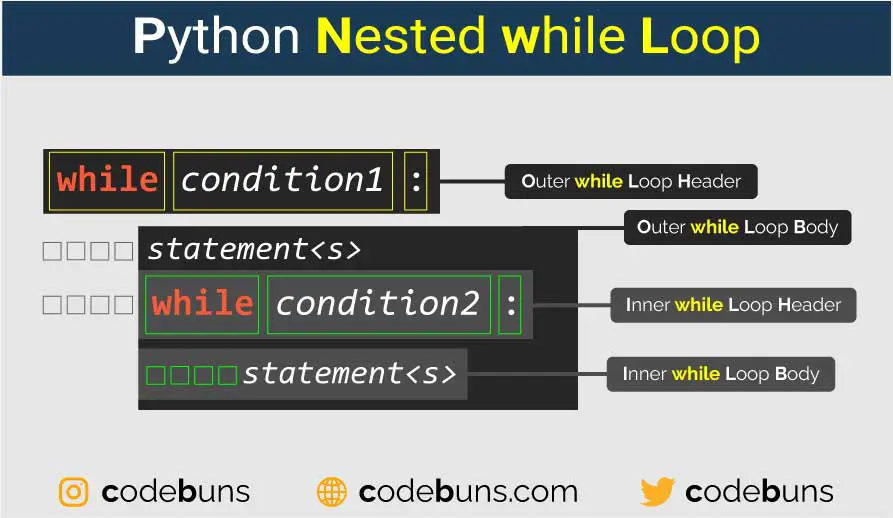
Syntax
while condition1: # code block for Outer Loop while condition2: # code block for Inner Loop
- The outer loop keeps iterating as long as condition1 evaluates to True.
- Within each outer loop iteration, the inner loop iterates as long as condition2 evaluates to True.
- Once condition2 becomes False, the rest of the outer loop’s code (if any) runs before the outer loop’s condition is checked again.
- If condition1 then evaluates to False, the entire nested loop structure completes.
Here is an example of using nested while loops:
Example
outer_counter = 1 while outer_counter <= 3: print(f"Outer loop iteration: {outer_counter}") inner_counter = 1 while inner_counter <= 5: print(f" Inner loop iteration: {inner_counter}") inner_counter += 1 outer_counter += 1
In this example, there are two while loops: the outer loop and the inner loop. The outer loop iterates 3 times, while the inner loop iterates 5 times. Each time the outer loop iterates, the inner loop will iterate completely, resulting in a total of 3 * 5 = 15
iterations for the inner loop.
The output of this code will be:
Outer loop iteration: 1
Inner loop iteration: 1
Inner loop iteration: 2
Inner loop iteration: 3
Inner loop iteration: 4
Inner loop iteration: 5
Outer loop iteration: 2
Inner loop iteration: 1
Inner loop iteration: 2
Inner loop iteration: 3
Inner loop iteration: 4
Inner loop iteration: 5
Outer loop iteration: 3
Inner loop iteration: 1
Inner loop iteration: 2
Inner loop iteration: 3
Inner loop iteration: 4
Inner loop iteration: 5
Nested loops can be used for various purposes, such as iterating over multi-dimensional arrays, generating combinations, or solving complex problems.
Infinite Python while Loop
An infinite while loop is a loop that runs indefinitely without a condition to break out of it. In Python, you can create an infinite while loop by using the while statement with the condition that is always True.
The basic syntax for creating an infinite while loop in Python is:
Syntax
while True: # Code to execute indefinitely
In the above syntax, while True creates an infinite loop because the condition True is always true, and the loop will continue to execute indefinitely.
To exit the infinite while loop, you can use the break statement to break out of the loop when a certain condition is met. For example:
Syntax
while True: # code to be executed indefinitely if condition: break
In the above example, condition is a placeholder for a condition that you want to check. When condition becomes true, the break statement is executed, and the loop is exited.
Here’s an example of an infinite while loop in Python:
Example
i = 0 while True: print(i) i += 1 if i >= 5: break
In this script, we initiate a counter variable i to 0. Then, we enter an infinite while loop. Inside the loop, we print the current value of i and increment it by 1. We keep doing this until i is equal to or greater than 5. When i reaches 5, we hit the break statement which exits the loop.
The output of this script would be:
0
1
2
3
4
Caution should be used when creating infinite while loops as they can cause your program to consume large amounts of resources and even crash if they aren’t appropriately managed.
To avoid the pitfalls of an infinite loop, it is important to include a way to exit the loop, such as a break statement or pressing CTRL+C on the keyboard to stop the program.
Looping through list
In Python, you can use a while loop to iterate through a list by using a loop control variable (e.g., an index) to access each element in the list. Here’s an example:
Example
# List of Elements numbers = [1, 2, 3, 4, 5] # Initialize index index = 0 # Use a while loop to iterate over the list while index < len(numbers): # Access the current element print(numbers[index]) # Move to the next index index += 1
This code will output:
1
2
3
4
5
Here is the step-by-step explanation:
- A list of integers named numbers is initialized with the values
[1, 2, 3, 4, 5]
. - An integer variable named index is initialized with a value of 0. This variable will be used to access each element of the list numbers by its index.
- A while loop is created, which will run until the value of index is less than the length of the list numbers. The
len(numbers)
function returns the number of elements in the list numbers. - Inside the while loop, the
print()
function is used to display the value of the element at the current index of the list numbers. This is achieved by using the indexing operationnumbers[index]
. - After printing the current element, the index is incremented by 1 with the
index += 1
statement, effectively moving to the next element in the list for the subsequent iteration. - Once the index becomes equal to or larger than the length of the list, the condition for the while loop (index < len(numbers)) is no longer satisfied, and the loop terminates.
- Overall, the code prints out each element in the numbers list, one at a time, from the first element to the last one.
Remember, while while loops can be used to iterate over lists, for loops are generally more concise and readable for this task. while loops are better suited for situations where you don’t know in advance how many times you’ll need to loop.
Conclusion
In this easy-to-understand guide, we covered various aspects of the Python while loop, from its syntax and flow diagram to its use cases. We also showed how to use the while loop with different control statements (break, continue, pass, and else), as well as nested loops. Additionally, we discussed how to use the while loop to cycle through lists.