Python try-except is a powerful error-handling mechanism that allows developers to handle exceptions gracefully. With the try-except block, developers can anticipate and catch potential errors in their code, preventing them from crashing the program. This feature is handy when dealing with unpredictable inputs or external dependencies. By using try-except in Python, developers can ensure that their programs continue to run smoothly even in the face of unexpected errors. Whether catching specific exceptions or creating a catch-all block, Python try-except provides a flexible and robust approach to error handling in Python programming.
Another term often used interchangeably with try-except in Python is “try-catch,” which refers to the same concept of catching and handling exceptions.
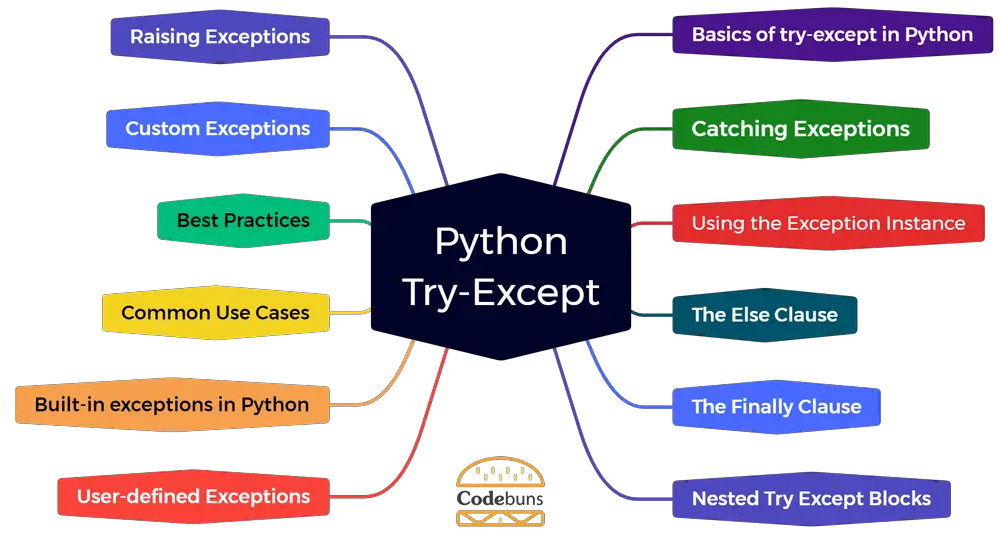
Table of Contents | |
Python try-except
Python try-except, also known as try-catch in other programming languages, is a powerful error-handling mechanism in Python. It allows developers to anticipate and handle potential exceptions or errors that may occur during the execution of a program. The try block contains the code that might raise an exception, while the except block specifies the actions to be taken if an exception is raised. Using try-except Python, developers can gracefully handle errors and prevent their programs from crashing or producing unexpected results. This construct is essential for writing robust and reliable code in Python.
Syntax of Python try-except
The syntax for a basic Python try-except block is as follows:
try: # Code that might cause an exception except ExceptionType: # Code to handle the exception
Explanation
- try block: Contains code that might raise exceptions.
- # Code block: This is where you write the code that might cause an exception.
- except blocks: Handle specific exception types, executing only if a matching exception occurs.
- ExceptionType: (Optional) Specifies the type of exception to handle (e.g., ValueError).
- # Code to handle the exception: This is where you handle the exception, often with error messages or fallback procedures.
Example of Python try-except
Let’s look at a practical example to understand how the try-except block works in Python:
try: result = 10 / 0 # This line will raise a ZeroDivisionError print("Result:", result) except ZeroDivisionError: print("Cannot divide by zero!")
Explanation
- try:: The try block is initiated.
- result = 10 / 0: This line of code attempts to divide 10 by zero, which is an operation that will result in a ZeroDivisionError.
- except ZeroDivisionError:: The except statement is used here to specifically catch the ZeroDivisionError.
- print(“Cannot divide by zero!”): If the ZeroDivisionError occurs, this line of code will be executed, printing a message to the user.
Python try-except block effectively prevents the program from crashing due to the zero division error. Instead, it provides a user-friendly message, demonstrating how exception handling can make your Python applications more error-tolerant and user-centric.
Basics of try-except in Python
The Python try-except block is a fundamental construct that allows you to handle exceptions elegantly. Here’s a breakdown of its components:
- Purpose: The primary purpose of the Python try-except block is to handle exceptions and errors that might occur during the execution of a program. It’s a proactive approach to programming, allowing you to anticipate and manage potential issues.
- try Block: This is where you place the code that could cause an error. It’s a protective layer that monitors the enclosed code for any exceptions.
- except Block: When an exception is thrown from the try block, it’s caught by the except block. This segment is crucial as it contains code that dictates how to handle the caught exception. It’s the response mechanism to the identified issue.
Catching Exceptions in Python
Catching exceptions in Python involves specifying what should happen when an error occurs in your code. There are different ways to catch exceptions, each with its purpose and use case.
Specific Exceptions: Catch Specific Errors to Handle Them Differently
Catching specific exceptions allows you to handle different types of errors differently. This is critical for responding appropriately to various error conditions.
Syntax
try: # Code that might cause an exception except SpecificException: # Handle the specific exception
Explanation
- try block: Encloses code that might raise exceptions.
- except block: Catches and handles specific exceptions.
- SpecificException: Optional placeholder for a targeted exception type.
Multiple Exceptions: A Single Except Block Can Handle Multiple Exceptions
Sometimes, you should handle several exceptions in the same way. Python allows you to catch multiple exceptions in a single except block.
Syntax
try: # Code that might cause multiple exceptions except (FirstException, SecondException): # Handle multiple exceptions
Explanation
- Single except block handles multiple exceptions:
- Enclose exception types in a tuple: (FirstException, SecondException)
- Order of exceptions matters:
- Handles exceptions from left to right within the tuple.
You can handle multiple exceptions by specifying multiple except blocks.
try: # Code that might cause multiple exceptions except FirstException: # Handle the first exception except SecondException: # Handle the second exception
Explanation
- Individual except blocks: Each handles a specific exception type.
- Sequential execution: Python checks except blocks in order until a match is found.
Wildcard Exception: Catch All Possible Exceptions (Not Recommended Due to Potential for Masking Errors)
Using a wildcard to catch all exceptions is generally not recommended, as it can hide errors and make debugging difficult. However, it can be used to ensure that your program does not fail under any circumstance.
Syntax
try: # Code that might cause any exception except: # Handle all exceptions
Explanation
- except block without a specified exception type: Captures any exception.
- Use sparingly: Provides less control and potential to mask errors.
Example of Catching Specific and Multiple Exceptions
Let’s illustrate catching specific and multiple exceptions with an example:
try: value = int(input("Enter a number: ")) result = 10 / value except ValueError: print("Please enter a valid integer.") except (ZeroDivisionError, OverflowError): print("Math error occurred.")
Explanation
- value = int(input(“Enter a number: “)): Attempts to convert user input to an integer, which can raise a ValueError.
- result = 10 / value: Attempts a division that can raise ZeroDivisionError or OverflowError.
- except ValueError:: Catches and handles the ValueError if the input conversion fails.
- except (ZeroDivisionError, OverflowError):: Catches and handles both ZeroDivisionError and OverflowError.
Example of Multiple Exceptions
try: user_input = input("Enter a number: ") number = int(user_input) # Potential for ValueError if input isn't a number result = 10 / number # Potential for ZeroDivisionError if number is 0 print("Result:", result) except ValueError: print("Invalid input. Please enter a valid integer.") except ZeroDivisionError: print("Cannot divide by zero!")
Explanation
- Potential for Errors: The code within the try block attempts to:
- Convert user input to an integer (int(user_input))
- Perform division (10 / number)
- Exception Handling:
- If the input isn’t a valid integer, ValueError is raised and handled by the first except block.
- If the input is 0, ZeroDivisionError is raised and handled by the second except block.
- Specific Handling: Each except block provides a tailored response to its respective exception, ensuring informative feedback for the user.
- Successful Execution: If no exceptions occur, the code within the try block executes successfully, and the result is printed.
Example of Catch-All Exception
try: number = int(input("Enter a number: ")) # Potential for ValueError result = 10 / number # Potential for ZeroDivisionError print("Result:", result) except: # Catches any exception that might occur print("An unexpected error occurred. Please try again.")
Explanation
- If an exception occurs, the code within the except block executes, providing a generic error message in this example.
- Using a catch-all except block can be helpful for unexpected errors during development or debugging but use it with caution in production code as it can mask potential issues.
Using Python try-except, ensures that different types of errors are handled in a way that’s appropriate for each, maintaining the stability and usability of your Python application. By mastering the art of catching exceptions in Python, you can significantly enhance the quality and reliability of your code.
Using the Exception Instance
In Python, the try-except block handles exceptions and errors detected during execution. A common practice is to use the exception instance to access and display the error message. This approach enhances the debugging process and clarifies what went wrong.
Syntax
except SomeException as e: print("Error occurred:", e)
Explanation
- except SomeException as e:: Catches an exception of type SomeException. The as e clause assigns the exception object to a variable (e) for inspection.
- print(“Error occurred:”, e): Prints the error message. The variable e represents the exception instance, which usually contains a description of the error.
Example
try: result = 10 / 0 except ZeroDivisionError as e: print("Error occurred:", e) print("Error type:", type(e)) print("Traceback:", e.__traceback__)
Explanation
- try: Starts the try block where you place the code that might raise an exception.
- result = 10 / 0: Code attempts to divide 10 by zero, which will raise a ZeroDivisionError.
- except ZeroDivisionError as e: Here, the ZeroDivisionError is caught, and the instance is stored in e.
- print(“Error occurred:”, e): Prints the error message, explaining why the exception was raised.
The Else Clause
In a Python try-except block, the else clause plays a significant role. It is executed when the try block does not raise an exception. Including an else clause can make the code more readable and better structured by separating the error-prone code from the code that runs only if no errors occur.
Syntax
try: # Code that might raise an exception except SomeException: # Code that runs if an exception occurs else: # Code that runs if no exception occurs
Explanation
- try Block: Encapsulates code that has the potential to raise exceptions.
- except Blocks: Designed to intercept and manage specific exceptions, ensuring program resilience.
- else Block: Executes exclusively when the try block completes without encountering exceptions, enabling conditional execution.
Example
try: result = 10 / 2 except ZeroDivisionError: print("Division by zero is not allowed.") else: print("Result:", result)
Explanation
- try: Begins the try block.
- result = 10 / 2: A division operation that does not raise an exception.
- except ZeroDivisionError: Catches a ZeroDivisionError. In this case, it is not executed since no error occurs.
- else: This block executes because no exception was raised, and it prints the result of the division.
Understanding and effectively using the Python try-except block, including accessing error messages through the exception instance and correctly implementing the else clause, is vital for writing error-resistant and clearer Python code.
The Finally Clause
The purpose of the finally clause in Python try-except blocks is to ensure that specific code runs irrespective of whether an exception is raised or not. It’s particularly useful for resource management tasks, like closing files or network connections, which need to be executed regardless of the success or failure of the try block.
Syntax
try: # Risky code that might raise an exception except SomeException: # Code to handle the exception finally: # Code that always executes
Explanation
- try Block: Begins the block where you place the code that might raise an exception.
- except SomeException: Catches specific exceptions that occur within the try block.
- finally Block: Executes regardless of exceptions, guaranteeing code runs.
Example
try: file = open("example.txt", "r") data = file.read() except FileNotFoundError: print("File not found.") finally: file.close() print("File closed.")
Explanation
- try: The try block starts. It attempts to open and read a file.
- file = open(“example.txt”, “r”): This line tries to open a file named “example.txt” in read mode.
- except FileNotFoundError: If the file is not found, this exception block catches the FileNotFoundError.
- print(“File not found.”): Prints an error message if the file is not found.
- finally: This block is executed after the try and except blocks.
- file.close(): Regardless of whether the file was successfully opened or not, this line ensures the file is closed.
- print(“File closed.”): This line indicates that the file has been closed, showing the execution of the finally block.
The finally clause in the Python try-except block is a powerful tool for resource management and ensuring the execution of essential cleanup activities. By incorporating this clause, Python programmers can write more reliable and fault-tolerant code.
Using Else and Finally
When handling exceptions in Python, the keywords else and finally play a crucial role. The else block is executed if no exception occurs within the try block, allowing you to perform additional operations. On the other hand, the finally block is always executed, regardless of whether an exception occurred or not, making it ideal for cleanup tasks like closing files or releasing resources. By utilizing these keywords in your try-except blocks, you can ensure more robust and reliable code execution in Python.
Syntax
try: # Code that might cause an exception except ExceptionType: # Handle the exception else: # Code that runs if no exception occurs finally: # Code that runs no matter what
Explanation
- try Block: – Contains code that might raise exceptions. – Acts as a testing ground for potential errors.
- except Block(s): – Catch and handle specific exception types. – Execute only if a matching exception occurs within the try block.
- else Block: – Executes only if no exceptions arise within the try block. – Facilitates code execution conditional on successful operations.
- finally Block: – Executes unconditionally, regardless of exceptions. – Guarantees critical code execution, often used for resource cleanup or essential tasks.
Example
try: number = int(input("Enter a number: ")) result = 100 / number # Potential ZeroDivisionError except ZeroDivisionError: print("Cannot divide by zero!") except ValueError: print("Invalid input. Please enter a number.") else: print("The result is:", result) finally: print("This line always executes, regardless of exceptions.")
Explanation
- try Block: Attempts to divide 100 by user input.
- except ZeroDivisionError: Handles division by zero errors.
- except ValueError: Manages invalid input types (e.g., non-numbers).
- else Block: Executes only if no exceptions occur, printing the result.
- finally Block: Unconditionally executes, often for cleanup tasks or logging.
Output Scenarios
- Valid Number: Prints “The result is: …” and “This line always executes…”
- Zero Input: Prints “Cannot divide by zero!” and “This line always executes…”
- Non-Number Input: Prints “Invalid input. Please enter a number.” and “This line always executes…”
Example of Python Try-Except with Multiple Exceptions, Else, and Finally
Consider an example where you’re trying to convert user input to an integer:
try: num = int(input("Enter a number: ")) except ValueError: print("That's not a number!") except KeyboardInterrupt: print("No input received.") else: print(f"You entered {num}") finally: print("Attempted to read a number.")
Explanation
- try: Attempts to convert the input to an integer.
- except ValueError: Catches and handles a ValueError if the input is not a valid number.
- except KeyboardInterrupt: Catches and handles the case where the user interrupts the input operation.
- else: Executes if there are no exceptions, confirming successful input conversion.
- finally: Executes regardless of the outcome, indicating the completion of the attempt.
By effectively using the Python try-except block, along with else and finally, and knowing how to raise specific exceptions, you can significantly improve the robustness and user-friendliness of your Python programs. This approach is a cornerstone of professional Python development, ensuring applications can handle unexpected scenarios smoothly.
Nested Try Except Blocks
Nested try-except Blocks in Python enable handling exceptions more granularly, especially within functions. This structure is particularly useful when a piece of code within a function may throw an exception, and you need to handle it within that function, possibly performing some specific recovery or cleanup operations.
Syntax
try: # Outer try block try: # Inner try block except SomeException: # Handle exceptions in the inner block except AnotherException: # Handle exceptions in the outer block
OR
def some_function(): try: # Code that might raise an exception except SomeException: # Handle exception try: some_function() except AnotherException: # Handle different exceptions that might be raised by the function
Explanation
- Defining a function with a try block: The function some_function() contains its own try-except block.
- try within the function: This block in the function tries to execute code that might raise SomeException.
- Handling exceptions in the function: If SomeException occurs, it’s handled within the function itself.
- Calling the function in another try block: When some_function() is called within another try block, it allows handling other exceptions that might be raised by the function.
Key Points
- Layered Exception Handling: Enable handling potential exceptions from multiple code sections.
- Structure: try-except blocks can be nested within one another.
- Propagation: If an inner block doesn’t handle an exception, it propagates to outer blocks.
Example
def divide_numbers(x, y): try: result = x / y except ZeroDivisionError: print("Division by zero!") try: divide_numbers(10, 0) except Exception as e: print("An error occurred:", e)
Explanation
- divide_numbers function with a try block: It attempts to divide two numbers.
- Handling ZeroDivisionError in the function: If division by zero occurs, it prints a specific message.
- Calling the function within another try block: This allows catching other potential exceptions from the function.
Raising Exceptions
Raising exceptions in Python is a powerful feature that allows programmers to trigger exceptions when certain conditions are met manually. This is done using the raise keyword. It’s particularly useful for enforcing certain constraints in your code or when you need to notify the calling code that something unusual or unexpected has happened.
Syntax
You can raise exceptions using the raise keyword.
raise ExceptionType("Error message") if some_condition: raise SomeException("Error message")
Explanation
- Conditional check: The if statement checks for a specific condition.
- raise keyword: Used to throw an exception when the condition is met.
- Creating an exception: SomeException(“Error message”) creates an instance of SomeException with a custom error message.
Example
def set_age(age): if age < 0: raise ValueError("Age cannot be negative") print(f"Age set to {age}") try: set_age(-5) except ValueError as e: print(e)
Explanation
- set_age function: Checks if the age is negative.
- raise ValueError: Throws a ValueError if the age is negative.
- Try-except block when calling set_age: Catches the ValueError raised by the function and prints the error message.
Developers can create more resilient, error-proof applications by mastering Nested try-except Blocks and Raising Exceptions in Python. These features of the Python try-except mechanism help prevent crashes, enforce logical constraints, and ensure that the code behaves as expected under all circumstances.
Custom Exceptions
Custom Exceptions in Python allow programmers to define exception classes that cater to specific application error scenarios. This is particularly useful when built-in exceptions do not adequately describe the error situation. You can make your code more readable and maintainable by creating custom exceptions.
Syntax
class MyCustomException(Exception): pass try: # Some code that raises a custom exception raise MyCustomException("Error message") except MyCustomException as e: # Handle the custom exception
Explanation
- Defining a Custom Exception Class: class MyCustomException(Exception): Creates a new exception class named MyCustomException which inherits from the base Exception class.
- raise MyCustomException: This line throws the custom exception with a specific error message.
- Catching the Custom Exception: The except MyCustomException as e: Block catches the custom exception and allows for handling it.
Example
class InvalidAgeException(Exception): pass def set_age(age): if age < 0: raise InvalidAgeException("Age cannot be negative") try: set_age(-1) except InvalidAgeException as e: print("Caught an invalid age exception:", e)
Explanation
- InvalidAgeException Class: Defines a custom exception for invalid age scenarios.
- raise InvalidAgeException: In set_age, if the age is negative, it raises the custom exception.
- Handling Custom Exception: The except block specifically catches InvalidAgeException and prints the associated error message.
How to Print an Exception with try-except
Printing an exception in Python is a common way to understand the nature of an error that has occurred. By catching and printing exceptions, programmers can diagnose the cause of issues in their code.
Syntax
try: # Code that may raise an exception except Exception as e: print("An exception occurred:", e)
Explanation
- try Block: Contains the code that might raise an exception.
- Catching the Exception: except Exception as e: catches any exception.
- Printing the Exception: print(“An exception occurred:”, e) prints the exception details.
Example
try: result = 10 / 0 except Exception as e: print("An exception occurred:", e)
Explanation
- Attempting Division by Zero: This operation will raise a ZeroDivisionError.
- Catching the Exception: The except block catches the ZeroDivisionError.
- Printing the Error Message: The error message “division by zero” is printed, indicating the nature of the exception.
How to Print the Exception Name
Sometimes, it’s useful to know the type of exception that occurred, rather than its message. This can be done by printing the name of the exception class.
Syntax
try: # Code that may raise an exception except Exception as e: print("Exception type:", type(e).__name__)
Explanation
- try Block: Contains the potentially error-raising code.
- Catching the Exception: except Exception as e: is a general way to catch any exception.
- Printing the Exception Type: type(e).__name__ extracts and prints the name of the exception class.
Example
try: result = 10 / 0 except Exception as e: print("Exception type:", type(e).__name__)
Explanation
- Code Causing an Exception: The division by zero is a sure way to cause a ZeroDivisionError.
- Catching the Exception: The except block is ready to catch any exception.
- Printing the Exception Name: It prints “ZeroDivisionError“, which is the type of the exception raised.
Through the use of Custom Exceptions and understanding how to print exceptions and their names, Python developers can significantly improve the debugging and error-handling capabilities of their applications. These techniques are integral to writing clean, efficient, and reliable Python code.
Best Practices
Understanding and implementing best practices for using Python try-except can greatly enhance the reliability and robustness of your Python code. By effectively utilizing try-except, you can gracefully handle unexpected errors, prevent program crashes, and ensure a smooth execution flow.
Exception Hierarchy in Python
Python’s exception hierarchy plays a crucial role in catching and handling exceptions. Being familiar with this hierarchy allows you to write more specific and appropriate exception-handling code. This knowledge ensures that you catch only those exceptions you are prepared to handle while others are propagated up the call stack.
Syntax
try: # Code that might raise an exception except SpecificException: # Handle specific exception except MoreGeneralException: # Handle more general exceptions
Explanation
- Specific Exception First: Catching more specific exceptions before more general ones ensures that each exception is handled as precisely as possible.
- General Exceptions Later: More general exceptions are caught later, which allows for broader error handling if specific exceptions are not raised.
Example
try: # Some risky operation number = int("abc") except ValueError: # Handle specific ValueError print("Invalid number format") except Exception: # Handle any other exceptions print("An error occurred")
Explanation
- Invalid Conversion Attempt: Trying to convert a non-numeric string to an integer will raise a ValueError.
- Handling ValueError: Specifically catches and handles the ValueError.
- Fallback Exception Handler: Catches any other exceptions that are not ValueError.
Minimal Try Blocks
Keeping the code within try blocks minimal is recommended in Python try-except blocks. This approach minimizes the risk of catching and silently handling exceptions that you are not explicitly checking for, which can lead to bugs that are difficult to diagnose.
Syntax
try: # Only the risky code except SomeException: # Handle the exception
Explanation
- Minimal Code in Try Block: Include only the code that might raise the exception you want to handle.
- Catch Specific Exceptions: Aim to catch only those exceptions that you expect could be raised by the code in the try block.
Example
denominator = 0 try: # Minimal code: Just the operation that might fail result = 1 / denominator except ZeroDivisionError: # Handle the specific exception print("Cannot divide by zero")
Explanation
- Single Risky Operation: Only the division operation, which might raise a ZeroDivisionError, is inside the try block.
- Handling Specific Exception: Catches and handles the ZeroDivisionError.
Logging Exceptions
Always logging an exception before handling or re-raising it is another best practice in Python. Logging records the error, which is invaluable for debugging and auditing.
Syntax
import logging try: # Risky code except SomeException as e: logging.error("Exception occurred", exc_info=True) # Handle or re-raise the exception
Explanation
- Import Logging Module: To log exceptions, the logging module is used.
- Log the Exception: logging.error logs the exception details, including a stack trace.
Example
import logging try: # Perform some risky operation open("nonexistent_file.txt") except FileNotFoundError as e: logging.error("File not found error occurred", exc_info=True) # Additional exception handling logic
Explanation
- Attempting to Open a Non-existent File: This operation raises a FileNotFoundError.
- Logging the Exception: Before handling the exception, it’s logged with details for future reference.
Best Practices for Python try-except Blocks
- Use try-except blocks to handle potential errors in your Python code.
- Place the code that might raise an exception inside the try block.
- Specify the type of exception you want to handle using the except keyword followed by the exception name.
- Include a generic except block at the end to catch any unexpected exceptions.
- Use multiple except blocks to handle different types of exceptions separately.
- Use finally block after try-except blocks to execute code regardless of whether an exception occurred or not.
- Avoid using bare except statements without specifying the exception type, as it can hide crucial errors and make debugging difficult.
- Handle specific exceptions first before handling more general ones.
- Raising custom exceptions using the raise keyword can be useful for handling errors in specific scenarios.
Implementing these best practices in Python try-except blocks — understanding exception hierarchy, keeping try blocks minimal, and logging exceptions — significantly enhances the effectiveness of your error-handling strategy. It leads to cleaner, more maintainable, and debuggable code, aligning well with professional Python development standards.
Common Use Cases
Python try-except offers a robust solution for addressing common pitfalls in software development, from handling input validation errors to dealing with file operations and network connections. In this section, you will explore some of the most common use cases for Python try-except and how it can enhance the reliability and stability of your code.
Input Validation
Input validation is a critical aspect of programming where Python’s try-except blocks shine. They ensure user input meets the expected format or type, gracefully handling anomalies.
Syntax
try: # Code to process user input except InputValidationError: # Handle invalid input
Explanation
- Process User Input: The try block contains code that attempts to process or convert user input.
- Handle Input Errors: The except block catches specific input-related exceptions and handles them, such as displaying an error message to the user.
Example
try: age = int(input("Enter your age: ")) except ValueError: print("Invalid input. Please enter a numeric value.")
Explanation
- User Input for Age: The user is prompted to enter their age, which is expected to be a number.
- Conversion to Integer: The code attempts to convert the input to an integer.
- Handling Non-Numeric Input: If the input is not numeric, a ValueError is raised and caught, prompting an appropriate message.
File Handling
File handling is another area where Python’s try-except blocks are handy. They manage Input/Output (IO) operations, ensuring the graceful handling of file-related errors like files not found or denied access.
Syntax
try: # Code to open and read/write a file except IOError: # Handle file-related errors
Explanation
- File IO Operations: The try block encloses code that attempts to open, read, or write to a file.
- Handling IO Errors: The except block is designed to catch IOError, which covers a range of file-related issues, including file not found or access problems.
Example
try: with open("example.txt", "r") as file: data = file.read() except FileNotFoundError: print("File not found.")
Explanation
- Attempting to Open a File: The code tries to open example.txt in read mode.
- Reading the File: If the file exists, it reads the content.
- File Not Found: If example.txt does not exist, a FileNotFoundError is raised and caught, with an error message displayed.
Network Communication
In network communication, handling exceptions is vital due to the unpredictable nature of network connectivity and data transmission. Python try-except blocks provide a robust mechanism to deal with these uncertainties.
Syntax
try: # Code to perform network operations except NetworkError: # Handle errors in network connectivity or data transmission
Explanation
- Network Operations: The try block contains code that performs operations like sending or receiving data over a network.
- Handling Network Errors: The except block catches exceptions related to network issues, such as connectivity problems or timeouts.
Example
try: response = requests.get("https://example.com") except requests.ConnectionError: print("Failed to connect to the server.")
Explanation
- Making a Network Request: The code attempts to retrieve data from https://example.com.
- Catching Connection Errors: If there is a network connectivity issue, requests.ConnectionError is raised and handled, informing the user of the problem.
In each of these common scenarios—input validation, file handling, and network communication—Python’s try-except blocks prove invaluable. They not only prevent the program from crashing but also provide a mechanism for handling errors gracefully and informing users or developers of the issues encountered. By effectively utilizing the Python try-except feature, programmers can significantly enhance their applications’ reliability and user experience.
Built-in exceptions in Python
Python offers a comprehensive set of built-in exceptions designed to signal specific error scenarios. By familiarizing yourself with common exceptions like ZeroDivisionError, ValueError, and TypeError, you can proactively implement exception-handling strategies using try-except blocks to prevent program crashes and provide informative feedback to users.
- ArithmeticError: Base class for numeric errors (e.g., ZeroDivisionError, OverflowError)
- AssertionError: Raises when an assert statement fails
- AttributeError: Occurs when trying to access an attribute that doesn’t exist
- EOFError: Signals reaching the end of file prematurely
- ImportError: Arises when importing modules fails
- IndexError: Raised when attempting to access a list or sequence index out of range
- KeyError: Occurs when trying to access a non-existent dictionary key
- KeyboardInterrupt: Generated when the user interrupts execution (e.g., Ctrl+C)
- NameError: Triggers when referencing an undefined variable or name
- OSError: Base class for operating system errors (e.g., FileNotFoundError, PermissionError)
- TypeError: Signals operations on incompatible data types
- ValueError: Indicates invalid arguments for a function or operation
- ZeroDivisionError: Occurs when attempting division by zero
Example of Built-in Exceptions in Python
try: # Division operation result = 10 / 0 except ZeroDivisionError: # Handling division by zero print("Cannot divide by zero.")
Explanation
- Division Operation: This line attempts to divide 10 by zero, which is not possible.
- Handling ZeroDivisionError: The except block catches the ZeroDivisionError and prints an appropriate message.
Understanding Python’s built-in exceptions and properly utilizing them in Python try-except blocks is fundamental for effective error handling. These exceptions provide a comprehensive way to deal with various error scenarios, making Python a powerful and user-friendly language for programmers of all levels.
User-defined Exceptions
User-defined exceptions are created by defining a new class derived from Python’s built-in Exception class. The syntax for creating a user-defined exception is simple and consistent with how other classes are defined in Python.
Syntax
class MyCustomError(Exception): pass
Explanation
- Defining a New Exception Class: class MyCustomError(Exception): creates a new exception class named MyCustomError.
- Inheriting from Exception: By inheriting from Python’s base Exception class, MyCustomError gains all the functionalities of a standard Python exception.
Example
class InvalidLevelError(Exception): """Exception raised for errors in the input level.""" def __init__(self, level, message="Level is not valid"): self.level = level self.message = message super().__init__(self.message) try: level = -1 if level < 0: raise InvalidLevelError(level) except InvalidLevelError as e: print(f"Error: {e.message} - Level: {e.level}")
Explanation
- Defining InvalidLevelError: This custom exception is defined with an additional level attribute and a default message.
- Initializing the Exception: The __init__ method initializes the exception with the provided level and message, then calls the super().__init__ method to initialize the base Exception class.
- Raising the Exception: In the try block, a condition checks if level is less than 0. If it is, InvalidLevelError is raised with the offending level value.
- Handling the Exception: The except block catches InvalidLevelError and prints the error message along with the level that caused the error.
User-defined exceptions in Python are powerful, allowing developers to create custom error-handling mechanisms. They provide clarity and specificity in error reporting, making debugging and maintaining the application easier. By using the Python try-except block with user-defined exceptions, programmers can ensure their applications handle errors in a user-friendly and specific way to the application’s context.
Conclusion
Python try-except blocks are essential for building strong and reliable code. By learning how to use them effectively, you can create programs that gracefully handle errors, keeping them running smoothly and preventing unexpected crashes. This leads to better user experiences and makes your code easier to maintain. As you continue your Python journey, remember the importance of try-except blocks for creating resilient code that anticipates and handles potential problems. This approach builds confidence in programmers and users, ensuring programs work as expected, even in unexpected challenges.
Also Read
15+ Popular Python IDE and Code Editors
Python Reference
FAQ
What is try-except in Python?
Python try-except is a fundamental error-handling mechanism that safeguards code execution from unexpected errors, preventing abrupt program crashes and ensuring smoother user experiences. It empowers developers to manage exceptions and gracefully provide informative feedback or alternative actions.
How does try-except work in Python?
The try block encapsulates potentially error-prone code. If an exception occurs within the try block, Python’s interpreter halts execution and seeks a matching exception block. If a match is found, the code within the except block handles the exception, often providing informative messages or actions to rectify the issue.
Can you catch multiple exceptions in Python?
Yes, Python supports catching multiple exceptions using either separate except blocks for each type or a single except block that handles a tuple of multiple exception types. This flexibility allows for tailored responses to different error scenarios.
What is the purpose of the else clause in try-except?
The else clause executes exclusively when the code within the try block completes successfully without encountering any exceptions. It’s often used for tasks conditional on error-free execution, promoting code organization and clarity.
Is it necessary to specify an exception type in the except block?
While not strictly mandatory, specifying the exception type in the except block is highly recommended for precise exception handling and informative error messages. It enables targeted responses to specific error scenarios, enhancing code clarity and maintainability.
What is the role of the “finally” block in Python’s error handling?
The “finally” block guarantees the execution of its code regardless of whether exceptions occur or not. This feature is invaluable for essential tasks like closing files, releasing resources, or logging events, ensuring data integrity and code reliability.
When is it appropriate to use a custom exception in Python?
Crafting custom exceptions is often beneficial when you need to signal specific error conditions or enforce application-level rules beyond the scope of built-in exceptions. This approach enhances code clarity, maintainability, and tailored error responses.