Python DateTime is a powerful module that allows programmers to work with dates and times in their Python programs. It provides a simple way to manipulate, format, and compare dates and times. With Python DateTime, you can easily perform operations such as adding or subtracting time intervals, extracting specific components from a date or time, and converting between different date formats. Whether you need to calculate the duration between two events or display the current date and time in a specific format, Python DateTime has got you covered.
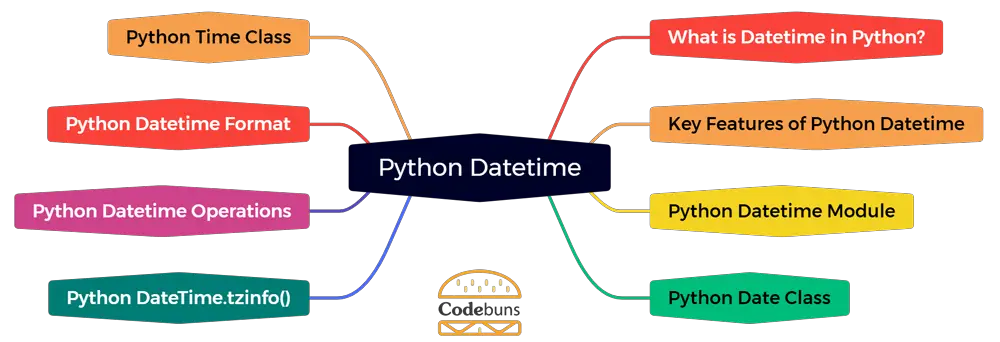
Table of Contents | |
What is Datetime in Python?
Datetime in Python is a module that provides classes for manipulating dates and times. It allows for date and time arithmetic, formatting, and parsing. The module includes fundamental classes such as datetime, date, time, and timedelta. These classes can handle time zones, leap seconds, and various calendar systems.
By leveraging the power of Python’s datetime module, developers can save significant time and effort when dealing with time-related operations in their projects. Whether you are building a web application that requires precise scheduling or analyzing data that involves temporal aspects, Python’s datetime module is an invaluable tool in your arsenal.
Python Datetime Example
import datetime # Current date and time: now = datetime.datetime.now() # Specific date and time: specific_datetime = datetime.datetime(2023, 12, 20, 15, 45, 0) print(specific_datetime) # Output: "2023-12-20 15:45:00"
Explanation
- import datetime: Imports the datetime module.
- datetime.datetime(): The constructor for creating datetime objects.
- .now(): Captures the current date and time.
- (year, month, day, hour, minute, second): Specify components for a custom datetime.
Accessing Datetime Components
Once you have a datetime object, you can access its individual components using dot notation:
Example
year = now.year # Extract the year month = specific_datetime.month # Retrieve the month print(year) # Output: "2023" print(month) # Output: "12"
Explanation
- now.year: Accesses the year attribute of the now datetime object, extracting its year value.
- specific_datetime.month: Retrieves the month attribute of the specific_datetime object, obtaining its month value.
Key Features of Python Datetime
These are just some of the key features that make Python DateTime a versatile and powerful tool for handling dates and times in your projects.
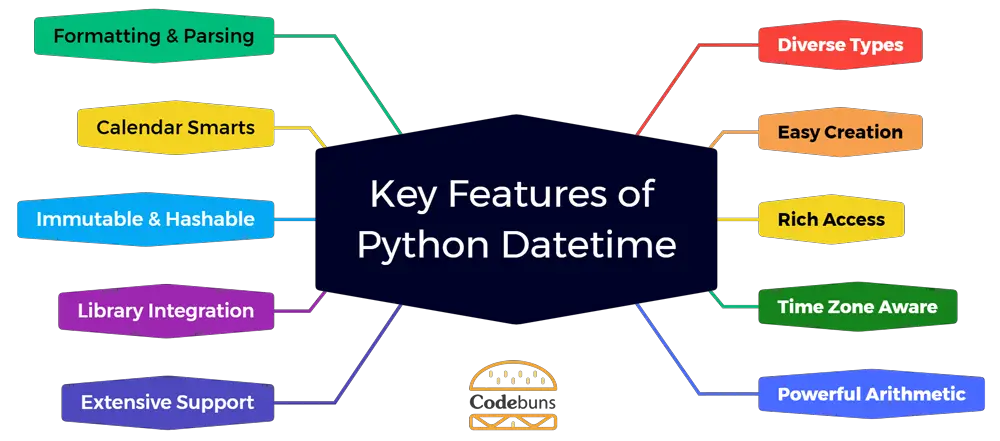
- Diverse Types: date, time, datetime, and timedelta for flexible date & time handling.
- Easy Creation: Build objects with individual components or parse strings in various formats.
- Rich Access: Extract specific parts like year, month, day, or even weekday/week number.
- Time Zone Aware: Handle dates with or without time zones and seamlessly convert between them.
- Powerful Arithmetic: Add, subtract, and multiply dates & durations to calculate time differences.
- Formatting & Parsing: Convert dates & times to human-readable strings and back with customizable formats.
- Calendar Smarts: Access detailed calendar information like month names, leap years, and day of week.
- Immutable & Hashable: Ensures data integrity and allows the use of dates as dictionary keys.
- Library Integration: Works seamlessly with popular data analysis libraries like Pandas and NumPy.
- Extensive Support: Comprehensive documentation and vibrant online communities for learning & help.
Python Datetime Module
Python Datetime module offers classes to manipulate date and time data with functions for creating, parsing, and formatting these objects. The module supports operations like comparing dates, calculating durations, and handling time zones. It’s essential for tasks involving scheduling, logging, and timestamps in Python programming.
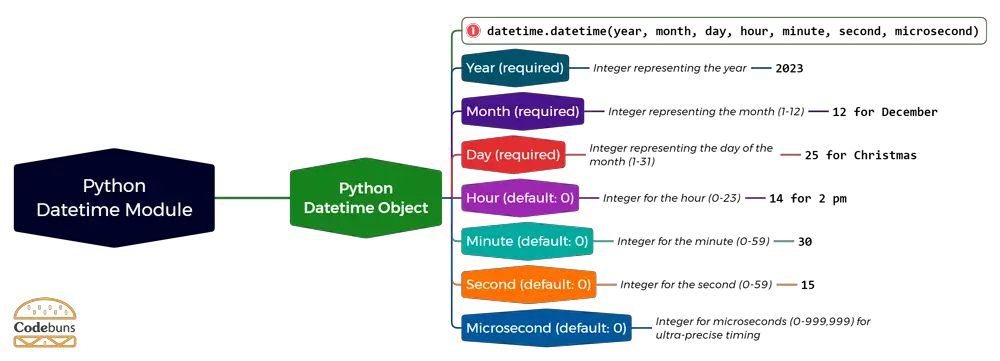
Importing the Datetime Module
To access the datetime functionalities, the datetime module must be imported into your Python script. This module provides classes for manipulating dates and times in both simple and complex ways.
Example
import datetime
import keyword is used to include the datetime module in your Python script.
Datetime Objects
A datetime object in Python represents a specific moment in time, defined by attributes like year, month, day, hour, minute, second, and microsecond.
Syntax of Python Datetime
datetime.datetime(year, month, day, hour, minute, second, microsecond)
Explanation
- datetime.datetime is the constructor for creating datetime objects.
- Parameters like year, month, day, hour, minute, second, microsecond specify the date and time.
Creating a DateTime Object
- Year (required): Integer representing the year, e.g., 2023.
- Month (required): Integer representing the month (1-12), e.g., 12 for December.
- Day (required): Integer representing the day of the month (1-31), e.g., 25 for Christmas.
Optional Arguments
- Hour (default: 0): Integer for the hour (0-23), e.g., 14 for 2 pm.
- Minute (default: 0): Integer for the minute (0-59), e.g., 30.
- Second (default: 0): Integer for the second (0-59), e.g., 15.
- Microsecond (default: 0): Integer for microseconds (0-999,999) for ultra-precise timing.
Key Points
- All arguments must be integers.
- Days must be valid for the given month and year (e.g., February 29th is not valid unless it’s a leap year).
- Hours, minutes, and seconds cannot exceed their respective limits.
Example of Python Datetime
import datetime specific_datetime = datetime.datetime(2023, 12, 1, 15, 30, 45, 123456) print(specific_datetime) # Output: "2023-12-01 15:30:45.123456"
Creates a datetime object for 1st December 2023 at 15:30:45 with 123456 microseconds.
Explanation
- Imports: datetime module provides tools for working with dates and times.
- Object Creation
- specific_datetime: Variable name for the created object.
- datetime.datetime: Constructor call for a datetime object.
- Arguments
- year=2023: Sets the year to 2023.
- month=12: Sets the month to December (12th).
- day=1: Sets the day to the 1st of the month.
- hour=15: Sets the hour to 3 PM (15 in 24-hour format).
- minute=30: Sets the minute to 30.
- second=45: Sets the second to 45.
- microsecond=123456: Sets the microsecond to 123456 (optional, defaults to 0).
- print(specific_datetime) displays the created datetime object.
Datetime Today: datetime.today() Function
The datetime.today() function is a straightforward method in the datetime module for getting the current date in the local timezone. It returns a datetime object with the current date and a time component set to zero.
Example
import datetime today_date = datetime.datetime.today() print(today_date) # Output: "2023-12-20 15:24:38.481000"
today() is a method of the datetime class that returns the current local date, such as 2023-12-20.
Python Date Class
The Python Date class, part of the datetime module, specifically handles dates. It provides methods to create, manipulate, and retrieve date information, independent of time and time zones. This class allows for operations like comparing dates, calculating differences, and formatting dates for display. The Date class supports calendar-related functions, including leap year checks. It’s essential in Python for any date-specific data handling and analysis tasks.
The Date class includes various methods such as today(), fromtimestamp(), isoformat(), and more. These methods allow for operations like getting the current date, converting timestamps to dates, and formatting dates.
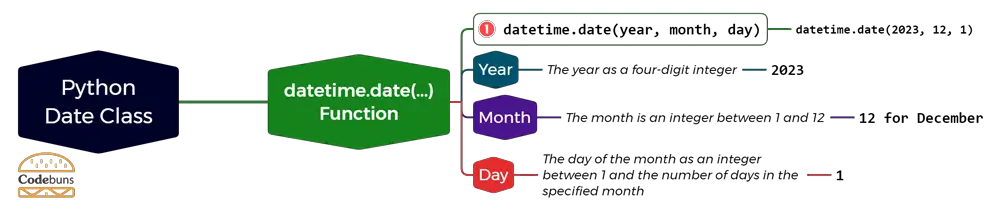
Creating a Date: datetime.date() Function
To create a specific date, the datetime.date() function is used, which accepts year, month, and day as arguments.
Syntax
datetime.date(year, month, day)
Explanation
- datetime.date: Constructor calls for a date object from the datetime module.
- Arguments
- year: The year as a four-digit integer (e.g., 2023).
- month: The month is an integer between 1 and 12.
- day: The day of the month as an integer between 1 and the number of days in the specified month.
Example
import datetime date_object = datetime.date(2023, 12, 1) print(date_object) # Output: "2023-12-01"
Creates a date object representing December 1, 2023.
Explanation
- Import: datetime module provides tools for handling dates and times.
- Object Creation
- date_object: Variable name for the created object.
- datetime.date(2023, 12, 1): Creates a date object using the constructor.
- Arguments
- year=2023: Sets the year to 2023.
- month=12: Sets the month to December (12th).
- day=1: Sets the day to the 1st of the month.
- print(date_object) displays the information stored in the created date object.
Get Current Date: date.today() Function
The date.today() function is a straightforward way to get the current date from the system’s local date.
Example
import datetime current_date = datetime.date.today() print(current_date) # Output: "2023-12-20"
today() is a method that returns the current local date.
Get Date from Timestamp
To convert a timestamp to a date, the fromtimestamp() method of the Date class is utilized.
Syntax
datetime.date.fromtimestamp(timestamp)
Explanation
- datetime.date: Accesses the date class within the datetime module.
- .fromtimestamp(timestamp): Static method to convert a timestamp to a date object.
- timestamp: A floating-point number representing the number of seconds since the Unix epoch (January 1, 1970 00:00:00 UTC).
Example
import datetime date_from_timestamp = datetime.date.fromtimestamp(1672527600) print(date_from_timestamp) # Output: "2023-01-01"
Explanation
- Variable Assignment
- date_from_timestamp: Variable to store the extracted date.
- datetime.date.fromtimestamp(1672527600): Converts the provided timestamp to a date object.
- Arguments
- timestamp=1672527600: Represents a specific point in time (Thursday, December 28, 2023 00:00:00 UTC).
- Function Call
- fromtimestamp(timestamp): Extracts the date portion from the provided timestamp.
- Output
- print(date_from_timestamp) displays the extracted date as a date object (2023-12-28).
Print Today’s Year, Month, and Day
To print individual components of today’s date, such as year, month, and day, one can access these attributes directly from the date object.
Example
import datetime today = datetime.date.today() print(today.year, today.month, today.day) # Output: "2023 12 20"
Explanation
- Variable Assignment
- today: Variable to store the current date.
- datetime.date.today(): Retrieves the current date as a date object.
- It automatically considers your local system’s time zone.
- Accessing Components
- today.year, today.month, and today.day: Access individual components (year, month, and day) from the date object using dot notation.
Convert Date Object to String
To convert a date object to a string, methods like strftime() or isoformat() are used for customizable formatting.
Syntax
date_object.strftime(format)
Explanation
- date_object: A valid datetime object containing the date you want to convert to a string.
- .strftime(format): Method call on the date_object to format it as a string.
- format: A string specifying the desired format for the output.
Key Points
- This syntax allows you to customize the string representation of your date_object using various format codes.
- Common format codes include:
- %Y: Year (4 digits)
- %y: Year (2 digits)
- %m: Month (number)
- %b: Month (abbreviation)
- %d: Day of the month (number)
- %a: Day of the week (abbreviation)
- %H: Hour (24-hour format)
- %M: Minute
- %S: Second
- You can combine these codes to create more complex formats (e.g., “%Y-%m-%d” for 2023-12-19).
Example
import datetime date_object = datetime.date(2023, 12, 1) date_string = date_object.strftime("%Y-%m-%d") print(date_string) # Output: "2023-12-01"
Explanation
- Date Object Creation
- date_object: Variable to store the created date object.
- datetime.date(2023, 12, 1): Creates a date object with specific components (year, month, day).
- Formatting
- date_string: Variable to store the formatted string.
- date_object.strftime(“%Y-%m-%d”): Applies the strftime() method to the date_object with a specific format string.
- Format String
- %Y: Represents the year with four digits (2023).
- %m: Represents the month as a number (12).
- %d: Represents the day of the month (1).
- Output
- print(date_string) displays the converted string containing the formatted date.
Python Time Class
The Python Time class, a component of the datetime module, focuses exclusively on time, independent of any date. It provides precision to microseconds and includes attributes like hour, minute, second, and microsecond. This class offers functionality for creating, manipulating, and formatting time objects. Time class operations include comparing times, time arithmetic, and time representation in various formats. It is especially useful in Python for tasks requiring precise time handling without the context of a specific date.
The Time class in Python includes various methods such as isoformat(), strftime(), and attributes like hour, minute, second, and microsecond. These methods and attributes are essential for managing and formatting time data.
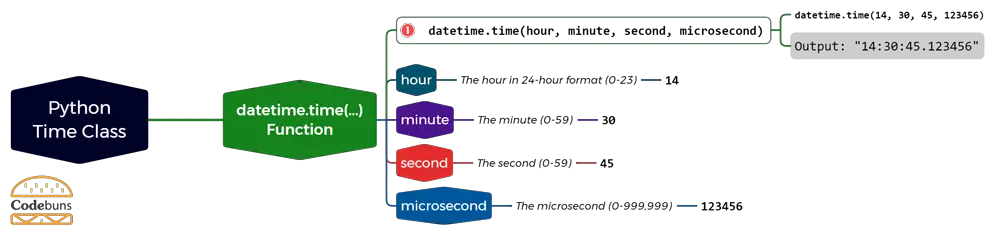
Creating a Time: datetime.time() Function
The datetime.time() function is used to create a time object, which can include hour, minute, second, and microsecond components.
Syntax
datetime.time(hour, minute, second, microsecond)
Explanation
- datetime.time: Constructor calls for a time object from the datetime module.
- Arguments (optional with default values)
- hour: The hour in 24-hour format (0-23).
- minute: The minute (0-59).
- second: The second (0-59).
- microsecond: The microsecond (0-999,999).
Key Points
- This syntax creates a time object, focusing solely on the time components (hour, minute, second, microsecond).
- It excludes date information like year, month, and day.
- Useful for tasks like representing time intervals, calculating durations, or setting timers.
Example
import datetime time_object = datetime.time(14, 30, 45, 123456) print(time_object) # Output: "14:30:45.123456"
This code creates a time object for 14:30:45 with 123456 microseconds.
Explanation
- Import: datetime module provides tools for handling dates and times.
- Object Creation
- time_object: Variable to store the created time object.
- datetime.time(14, 30, 45, 123456): Creates a time object using the constructor.
- Arguments
- hour=14: Sets the hour to 2 PM (14 in 24-hour format).
- minute=30: Sets the minute to 30.
- second=45: Sets the second to 45.
- microsecond=123456: Sets the microsecond to 123456 (optional, defaults to 0).
Get Current Time
While the Time class itself doesn’t provide a direct method to get the current time, this can be achieved by using datetime.now() and extracting the time.
Example
import datetime current_time = datetime.datetime.now().time() print(current_time) # Output: "16:30:49.871000"
Explanation
- Chain of Operations
- datetime.datetime.now(): Gets the current date and time as a datetime object.
- .time(): Extracts the time portion from the datetime object.
- Variable Assignment
- current_time: Stores the extracted time as a time object.
- Output
- print(current_time) Displays the current time information.
Print Hour, Minute, Second, and Microsecond
Individual components of a time object, such as hour, minute, second, and microsecond, can be accessed and printed.
Example
import datetime time_object = datetime.time(12, 15, 30, 987654) print(time_object.hour, time_object.minute, time_object.second, time_object.microsecond) # Output: "12 15 30 987654"
Explanation
- Object Creation
- time_object: Variable to store the created time object.
- datetime.time(12, 15, 30, 987654): Creates a time object using the constructor.
- Arguments
- hour=12: Sets the hour to 12 PM (in 24-hour format).
- minute=15: Sets the minute to 15.
- second=30: Sets the second to 30.
- microsecond=987654: Sets the microsecond to 987654 (optional, defaults to 0).
- Accessing Components
- time_object.hour, time_object.minute, time_object.second, time_object.microsecond: Separate calls to access individual components (hour, minute, second, microsecond) using dot notation.
Convert Time Object to String
To convert a time object to a string, the strftime() method is used, allowing for flexible formatting based on the programmer’s requirements.
Syntax
time_object.strftime(format)
strftime(format) is used to format a time object as a string.
Example
import datetime time_object = datetime.time(20, 45, 30) time_string = time_object.strftime("%H:%M:%S") print(time_string) # Output: "20:45:30"
Explanation
- Time Object Creation
- time_object: Variable to store the created time object.
- datetime.time(20, 45, 30): Creates a time object with specific components (hour, minute, second).
- Arguments
- hour=20: Sets the hour to 8 PM (20 in 24-hour format).
- minute=45: Sets the minute to 45.
- second=30: Sets the second to 30.
- Formatting
- time_string: Variable to store the formatted string.
- time_object.strftime(“%H:%M:%S”): Applies the strftime() method to the time_object with a specific format string.
- Format String
- %H: Represents the hour in 24-hour format with two digits (20).
- %M: Represents the minute with two digits (45).
- %S: Represents the second with two digits (30).
- Output
- print(time_string) Displays the converted string containing the formatted time: “20:45:30“.
Python Datetime Format
Formatting datetime objects to strings in Python is a common task involving converting date and time information into a readable or standardized format.
Datetime to String
Datetime to string in Python refers to the conversion of a datetime object into a string representation. This process is typically achieved using the strftime() method in the datetime module. It allows for custom formatting date and time components into readable text formats. This conversion is essential for displaying datetime information in user-friendly formats or serialization. Understanding this conversion is crucial for effective date-time handling in Python applications.
Python strftime() Method
The strftime() method in Python is used to convert datetime objects to their string representation based on specified format codes.
Syntax
datetime_object.strftime(format)
Explanation
- datetime_object: A datetime object containing date and/or time information to be formatted.
- .strftime(format): Method calls on the datetime_object to convert it into a string with a specified format.
- format: A string specifying the desired output format using special format codes.
Format Codes (Common Examples)
- Date Components
- %Y: Four-digit year (e.g., 2023)
- %m: Month as a number (01-12)
- %b: Month abbreviation (e.g., Dec)
- %d: Day of the month (01-31)
- %a: Weekday abbreviation (e.g., Wed)
- Time Components
- %H: Hour in 24-hour format (00-23)
- %I: Hour in 12-hour format (01-12)
- %M: Minute (00-59)
- %S: Second (00-59)
- %p: AM/PM indicator
Examples
- “%Y-%m-%d %H:%M:%S”: 2023-12-19 15:32:45
- “%A, %B %d, %Y”: Tuesday, December 19, 2023
- “%I:%M %p”: 03:32 PM
Example
import datetime now = datetime.datetime.now() formatted_string = now.strftime("%Y-%m-%d %H:%M:%S") print(formatted_string) # Output: "2023-12-20 15:55:00"
Formats the current datetime into a string with the format “YYYY-MM-DD HH:MM:SS”.
Python Datetime: Understanding Format Codes
Understanding the format codes in the Python datetime module is crucial for effectively formatting and parsing date and time strings. Each code represents a specific component of date or time.
Python’s strftime() method supports a variety of format codes. Here’s a detailed explanation of the common format codes:
Date Components
- %a: Weekday as a three-letter abbreviation (e.g., “Wed”).
- %A: Full weekday name (e.g., “Wednesday”).
- %w: Weekday as a number (0-6, Sunday is 0).
- %d: Day of the month as a zero-padded decimal (01-31).
- %b: Month as a three-letter abbreviation (e.g., “Dec”).
- %B: Full month name (e.g., “December”).
- %m: Month as a zero-padded decimal (01-12).
- %y: Year without century as a zero-padded decimal (00-99).
- %Y: Year with century as a decimal (e.g., 2023).
Time Components
- %H: Hour in 24-hour format (00-23).
- %I: Hour in 12-hour format (01-12).
- %p: AM/PM indicator (e.g., “AM”).
- %M: Minute as a zero-padded decimal (00-59).
- %S: Second as a zero-padded decimal (00-59).
- %f: Microsecond as a decimal (000000-999999).
Time Zone Information
- %z: UTC offset in the form ±HHMM (e.g., “+0530”).
- %Z: Time zone name (e.g., “Asia/Kolkata”).
Other Components
- %j: Day of the year as a zero-padded decimal (001-366).
- %U: Week number of the year (Sunday as the first day of the week) as a zero-padded decimal (00-53).
- %W: Week number of the year (Monday as the first day of the week) as a zero-padded decimal (00-53).
- %c: Locale’s appropriate date and time representation (e.g., “Wed Dec 20 15:55:00 2023”).
- %C: Century as a decimal (00-99).
- %x: Locale’s appropriate date representation (e.g., “12/20/2023”).
- %X: Locale’s appropriate time representation (e.g., “15:55:00”).
- %%: Literal percent sign.
- %G: ISO 8601 year with century as a decimal (e.g., 2023).
- %u: Weekday as a number (1-7, Monday is 1).
- %V: ISO 8601 week number of the year (Monday as the first day of the week) as a zero-padded decimal (01-53).
Example
import datetime now = datetime.datetime.now() print(now.strftime("%a")) # e.g., 'Wed'
String to Datetime
In Python, String to datetime involves converting a string formatted as a date or time into a datetime object. This is accomplished using the strptime method from the datetime module. The method requires the string and the specific format code that matches the string’s layout. This conversion is vital for processing and analyzing dates and times stored as text.
Python strptime() Method
The Python strptime() method converts a string representation of a date or time into a datetime object, making it easier to manipulate and work with dates and times in your code. With the strptime() method, you can specify the input string format using various directives, such as %Y for year, %m for month, %d for day, and so on. This method is particularly useful when dealing with data that is stored or received as strings but needs to be processed as datetime objects in Python.
Syntax
datetime.datetime.strptime(date_string, format)
Explanation
- datetime.datetime: Accesses the datetime class for date and time operations.
- .strptime(date_string, format): Method call for string parsing.
- date_string: The string containing date and/or time information to be parsed.
- format: A string specifying the format of date_string, using designated codes.
Example
import datetime datetime_object = datetime.datetime.strptime("2023-12-20 14:30", "%Y-%m-%d %H:%M") print(datetime_object) # Output: "2023-12-20 14:30:00"
Raises a ValueError exception if parsing fails due to an incorrect format.
Python fromisoformat() Method
The Python fromisoformat() method allows you to convert a string representation of a date and time in ISO 8601 format into a datetime object. This method is particularly useful when dealing with stored, or transmitted data in this standard format. Using the fromisoformat() method, you can easily manipulate and perform calculations on datetime objects in your Python programs.
Example
import datetime datetime_object = datetime.datetime.fromisoformat("2023-01-30T12:15:30") print(datetime_object) # Output: "2023-01-30 12:15:30"
Explanation
- datetime_object = datetime.datetime.fromisoformat(“2023-01-30T12:15:30”): Calls the fromisoformat() method to construct a datetime object from a string in ISO 8601 format:
- “2023-01-30T12:15:30”: The string holding the date and time information in ISO 8601 format.
- ISO 8601 Format
- International standard for date and time notation.
- Structure: YYYY-MM-DDTHH:MM:SS.mmmmmm
- Ensures consistent and unambiguous representation.
Python isoformat() Method
The Python isoformat() method is a built-in function that allows you to format a datetime object into a string representation following the ISO 8601 standard. It provides a standardized way of representing dates and times, making it easier to work with and exchange data across different systems. With the isoformat() method, you can easily convert datetime objects into strings with the desired format for various applications and data processing tasks in Python.
Example
import datetime datetime_object = datetime.datetime.now() iso_formatted = datetime_object.isoformat() print(iso_formatted) # Output: "2023-12-20T12:34:09.952000"
Explanation
- datetime_object = datetime.datetime.now(): Captures the current date and time as a datetime object and stores it in the variable datetime_object.
- iso_formatted = datetime_object.isoformat(): Calls the isoformat() method to convert the datetime_object into a string adhering to ISO 8601 format, a widely used international standard for date and time representation.
- ISO 8601 Format
- Consists of year, month, day, hour, minute, second, and microsecond components.
- Separates date and time with a “T” character.
- Includes microseconds for high precision.
Python Datetime Operations
Python datetime module provides extensive capabilities for manipulating and operating on date and time data. A key component of this module is the timedelta class, which facilitates operations like addition, subtraction, and comparison of datetime objects.
Timedelta: Explanation of the timedelta Class
The timedelta class in Python’s datetime module represents a duration, the difference between two dates or times.
Syntax
datetime.timedelta(days=0, seconds=0, microseconds=0, milliseconds=0, minutes=0, hours=0, weeks=0)
Explanation
- datetime.timedelta: Accesses the timedelta class, designed for representing time differences.
- (args): Constructor accepts keyword arguments to specify time intervals:
- days=0: Number of days (default is 0).
- seconds=0: Number of seconds (default is 0).
- microseconds=0: Number of microseconds (default is 0).
- milliseconds=0: Number of milliseconds (converted to microseconds).
- minutes=0: Number of minutes (converted to seconds).
- hours=0: Number of hours (converted to seconds).
- weeks=0: Number of weeks (converted to days).
Example
import datetime ten_days = datetime.timedelta(days=10) print(ten_days) # Output: "10 days, 0:00:00"
Creates a timedelta object representing a duration of 10 days.
Add Days to DateTime Object
Adding days to a datetime object is a common operation, easily achieved with the timedelta class.
Example
import datetime current_datetime = datetime.datetime.now() ten_days_later = current_datetime + datetime.timedelta(days=10) print(ten_days_later) # Output: "2023-12-30 12:57:08.661000"
Adds 10 days to the current datetime.
Difference Between Two DateTime
Calculating the difference between two datetime objects results in a timedelta object, representing the time interval between them.
Example
datetime1 = datetime.datetime(2023, 1, 1) datetime2 = datetime.datetime(2023, 1, 10) difference = datetime2 - datetime1 print(difference) # Output: "9 days, 0:00:00"
Calculates the difference between January 1 and January 10, 2023.
Operations Supported by Timedelta Class
The timedelta class supports operations like addition, subtraction, and multiplication, providing flexibility in date-time calculations.
Syntax
timedelta_object1 +/- timedelta_object2, timedelta_object * number
Explanation
- timedelta_object1 + timedelta_object2: Combines two timedelta objects, resulting in a new timedelta object representing their cumulative duration.
- timedelta_object1 – timedelta_object2: Subtracts one timedelta object from another, yielding a new timedelta object signifying the difference between their durations.
- timedelta_object * number: Multiplies a timedelta object by a number (integer or float), creating a new timedelta object with the duration scaled by the specified factor.
Example
import datetime ten_days = datetime.timedelta(days=10) doubled_duration = ten_days * 2 print(doubled_duration) # Output: "20 days, 0:00:00"
Doubles the duration of ten_days timedelta object.
Format DateTime in Python
Formatting datetime objects into readable strings is done using the strftime() method, which accepts various format codes.
Syntax
datetime_object.strftime(format)
Explanation
- datetime_object: Represents the datetime object you want to format. This object holds information about a specific date and time.
- .strftime(format): The method called on the datetime object to perform the formatting.
- format: A string containing special format codes that dictate the desired output structure.
Example
import datetime formatted_date = current_datetime.strftime("%Y-%m-%d %H:%M:%S") print(formatted_date) # Output: "2023-12-20 12:57:08"
Formats the current datetime as a string.
Difference Between Two Timedelta Objects
The difference between two timedelta objects is calculated by subtracting one from another, resulting in a new timedelta object.
Example
import datetime timedelta1 = datetime.timedelta(days=5) timedelta2 = datetime.timedelta(days=3) difference = timedelta1 - timedelta2 print(difference) # Output: "2 days, 0:00:00"
Calculates the difference as 2 days.
Time Duration in Seconds
Converting a timedelta duration to seconds is straightforward, using the total_seconds() method.
Example
import datetime ten_days = datetime.timedelta(days=10) seconds = ten_days.total_seconds() print(seconds) # Output: "864000.0"
Converts the ten_days timedelta object to seconds.
Compare Datetime: Discuss methods for comparing two datetime objects
Comparing two datetime objects is a straightforward task that is essential for various applications like event scheduling, data logging, or time-sensitive calculations. The datetime module, a part of Python’s standard library, provides the necessary tools for this comparison. The most common approach is to use comparison operators (like <, >, ==, !=, <=, >=) directly on datetime objects.
Syntax
datetime_object1 <operator> datetime_object2
Explanation
- <operator>: Placeholder for standard comparison operators. You can use < (less than), > (greater than), == (equal to), != (not equal to), <= (less than or equal to), or >= (greater than or equal to).
- datetime_object1 and datetime_object2: These are the two datetime objects you want to compare.
Comparison Operators
- Equality (==): Determines if two datetime objects represent the exact same point in time.
- Inequality (!=): Checks if two datetime objects differ in their date or time components.
- Less Than (<): Assesses if one datetime object occurs earlier than another.
- Less Than or Equal to (<=): Determines if one datetime object is either earlier or the same as another.
- Greater Than (>): Evaluates if one datetime object occurs later than another.
- Greater Than or Equal to (>=): Determines if one datetime object is either later or the same as another.
Example
from datetime import datetime datetime1 = datetime(2023, 12, 1, 12, 0) datetime2 = datetime(2023, 12, 1, 18, 0) result = datetime1 < datetime2 print(result) # Output: "True"
Explanation
- from datetime import datetime: Imports the datetime class from the datetime module.
- datetime1 = datetime(2023, 12, 1, 12, 0): Here, datetime1 is set to December 1, 2023, at 12:00 pm.
- datetime2 = datetime(2023, 12, 1, 18, 0): Creates another datetime object, datetime2, set to December 1, 2023, at 6:00 pm.
- result = datetime1 < datetime2: Compares datetime1 and datetime2. Since datetime1 is earlier than datetime2, result will be True.
Python DateTime.tzinfo()
Python’s datetime module provides robust support for time zone handling through tzinfo objects, which is essential for building applications sensitive to various time zones. The tzinfo abstract base class in Python is used to deal with time zone-related information, and various third-party libraries like pytz and dateutil offer concrete implementations of these objects. Using tzinfo objects, developers can represent time zones, handle daylight-saving time transitions, and perform conversions between different time zones.
Syntax
datetime.datetime(year, month, day, hour, minute, second, microsecond, tzinfo)
Explanation
- tzinfo is an optional parameter in the datetime constructor.
- Represents Time Zone Awareness: Incorporates time zone information into a datetime object, crucial for accurate time representation across different regions.
- Abstract Base Class: tzinfo is an abstract base class, meaning you typically use a subclass like pytz for specific time zones.
Example
import datetime import pytz # External library for time zone data eastern = pytz.timezone('US/Eastern') aware_datetime = datetime.datetime(2023, 1, 1, 12, 0, tzinfo=eastern) print(aware_datetime) # Output: "2023-01-01 12:00:00-04:56"
Explanation
- Imports the pytz library, which provides time zone information.
- Creates a timezone-aware datetime object for January 1, 2023, at 12:00 PM in the US Eastern time zone.
- Aware vs. Naive Datetimes: datetime objects with tzinfo are considered “aware,” while those without are “naive.”
- Awareness for Accurate Calculations: Aware datetimes are essential for precise time calculations and comparisons involving different time zones.
How to Get a Particular Timezone in Python Using datetime.now() and pytz
Using datetime.now() with pytz, you can create a datetime object in a specific timezone.
Example
import datetime import pytz pacific = pytz.timezone('US/Pacific') pacific_time = datetime.datetime.now(pacific) print(pacific_time) # Output: "2023-12-20 01:22:46.179000-08:00"
Explanation
- pytz.timezone(‘US/Pacific’) obtains the Pacific timezone object.
- datetime.datetime.now(pacific) gets the current time in the Pacific timezone.
Using dateutil to Add Time Zones to Python datetime
The dateutil library is another option for handling time zones in Python. It can be used to add time zone information to naive datetime objects.
Example
import datetime from dateutil import tz naive_datetime = datetime.datetime.now() aware_datetime = naive_datetime.replace(tzinfo=tz.gettz('Asia/Tokyo')) print(aware_datetime) # Output: "2023-12-20 14:24:52.734000+09:00"
Explanation
- Import Necessary Modules
- import datetime: Imports the datetime module for working with dates and times.
- from dateutil import tz: Imports the tz module from dateutil for time zone functionalities.
- Create a Naive Datetime Object
- naive_datetime = datetime.datetime.now(): Captures the current date and time as a naive datetime object, lacking time zone awareness.
- Attach Time Zone Information
aware_datetime = naive_datetime.replace(tzinfo=tz.gettz('Asia/Tokyo'))
:- naive_datetime.replace(…): Creates a new datetime object with modified attributes.
- tzinfo=tz.gettz(‘Asia/Tokyo’): Assigns the time zone ‘Asia/Tokyo’ using tz.gettz().
- Output
- print(aware_datetime): Displays the time-zone-aware datetime object, now reflecting the specified time zone (e.g., “2023-12-20 14:24:52.734000+09:00”).
- Naive vs. Aware Datetimes
- Naive datetimes lack time zone information, potentially leading to errors in time-related operations across different regions.
- Aware datetimes incorporate time zone awareness for accurate calculations and comparisons.
- dateutil’s Flexibility
- dateutil‘s tz module offers a convenient way to add time zone information to existing datetime objects.
- Supports a wide range of time zone names and abbreviations.
Alternatives to Python datetime and dateutil
Beyond datetime and dateutil, alternative libraries like pendulum and arrow offer enhanced functionalities for datetime manipulation, including more intuitive timezone handling.
Example
import pendulum # Get the current time in Tokyo, Japan, with time zone awareness: tokyo_time = pendulum.now('Asia/Tokyo') print(tokyo_time) # Output (e.g.): "2023-12-20T14:45:00+09:00" # Format the time in a human-readable way: print(tokyo_time.to_formatted_date_string()) # Output: "Dec 20, 2023"
Explanation
- import pendulum: Imports the Pendulum library, offering enhanced time zone handling and a user-friendly API.
tokyo_time = pendulum.now('Asia/Tokyo')
:- pendulum.now(): Obtains the current date and time as a Pendulum datetime object.
- (‘Asia/Tokyo’): Specifies the desired time zone, ensuring time zone awareness from the outset.
Conclusion
Python datetime module empowers developers to effectively manipulate and manage dates and times within their applications. Its core components, including the datetime class, timedelta class, and strftime/strptime methods, provide a robust toolkit for various time-related tasks.
While the built-in module offers a comprehensive foundation, third-party libraries like dateutil and Pendulum extend its capabilities, particularly in time zone handling and user-friendly interactions. These libraries simplify complex operations and offer intuitive APIs for seamless time-based development.
Understanding Python datetime functionalities is crucial for programmers working with date and time data. By leveraging these tools appropriately, developers can construct applications that accurately track events, schedule tasks, analyze temporal patterns, and deliver time-sensitive information in a clear and adaptable manner.
Also Read
15+ Popular Python IDE and Code Editors
Python Reference
FAQ
How do I create a datetime object in Python?
To create a datetime object representing the current date and time, use datetime.datetime.now(). Alternatively, provide specific date and time components as arguments to the datetime constructor.
How do I format a datetime object as a string?
Use the strftime() method and specify format codes to customize the output string. Common codes include %Y (year), %m (month), %d (day), %H (hour), %M (minute), and %S (second).
How do I parse a string into a datetime object?
Employ the strptime() method, providing the string and format codes matching its structure to convert it into a datetime object.
How do I calculate time differences in Python?
Utilize the timedelta class to represent time differences. Create timedelta objects using keywords like days, seconds, and microseconds, and perform arithmetic operations with them or with datetime objects.
How do I handle time zones in Python?
While Python’s built-in datetime module offers tzinfo, consider using third-party libraries like dateutil or Pendulum for enhanced time zone support, including conversions, calculations, and localization.
What is the difference between date, time, and datetime in Python?
The datetime module provides three types: date (for year, month, day), time (for hour, minute, second, microsecond), and datetime (combination of both date and time).
How can I format a datetime object into a more readable string?
Apply the strftime method on a datetime object to format it into a readable string. For example, datetime.now().strftime(“%Y-%m-%d %H:%M:%S”).
Is it possible to calculate the time difference between two dates in Python?
Yes, subtract one datetime object from another to get a timedelta object, which represents the difference in time.
How can I handle timezones in Python using datetime?
Use the pytz library along with datetime to handle timezone-aware datetime objects. Assign a timezone to a datetime object using pytz.timezone.