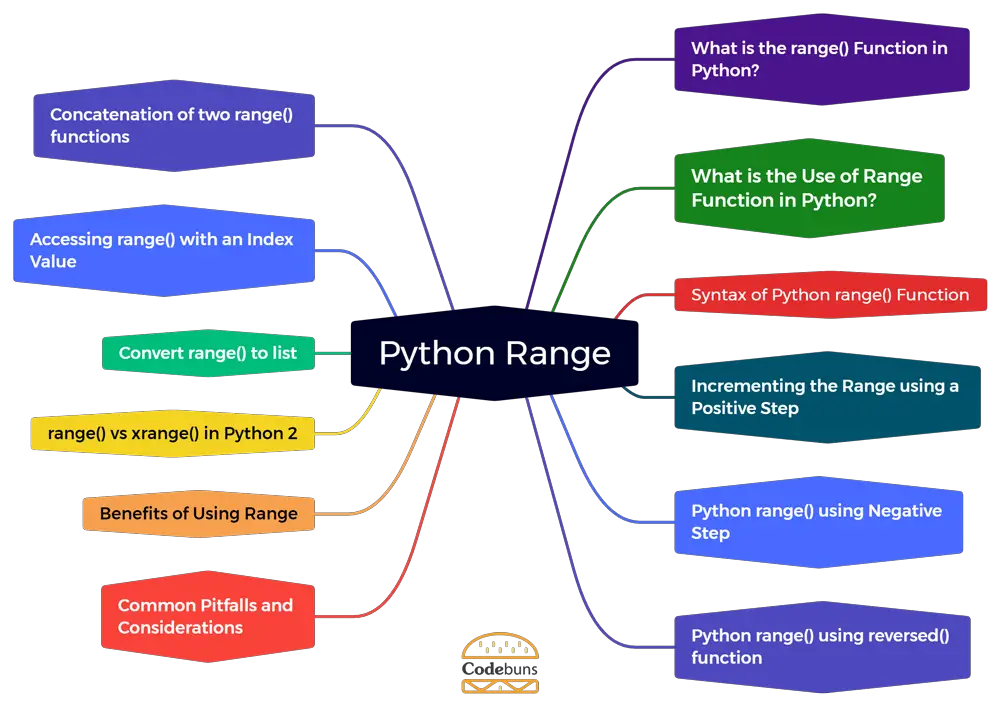
Table of Contents | |
What is the range()
Function in Python?
Python range() function is a built-in function that generates a sequence of numbers. It is commonly used in for loops to iterate over a specific range of values. The range() function takes three parameters: start, stop, and step. The start parameter specifies the starting point of the sequence, the stop parameter defines the endpoint (exclusive), and the step parameter determines the increment between each number in the sequence. This versatile function allows programmers to easily create loops that iterate over a desired range of values in their Python programs.
Example of Python Range
for i in range(3, 10, 2): # Generates 3, 5, 7, 9
print(i)
Explanation
for i in range(3, 10, 2):
:- for: Initiates a loop that iterates through a sequence of numbers.
i in range(3, 10, 2)
: Specifies the sequence usingrange()
:- 3: Starting number (inclusive).
- 10: Endpoint (exclusive, not included).
- 2: Increment (steps by 2 in each iteration).
print(i)
: Prints the current value of i in each loop iteration.
What is the Use of Range Function in Python?
Python Range function is a valuable tool for programmers. It allows you to generate a sequence of numbers within a specific range effortlessly, making loops and iterations more efficient. By mastering the range function, you can greatly enhance the functionality and flexibility of your Python programs.
Here are some of the uses of Python range() function:
- Creating Number Sequences: Generates a sequence of numbers within a specified range, ideal for iteration and indexing.
- Powering for Loops: Primarily used to control the number of iterations in for loops, ensuring precise repetition.
- Slicing Lists and Strings: Enables extracting specific elements from lists and strings using start, stop, and step values.
- Generating Indices for Iteration: Provides a convenient way to iterate over indices of iterables, accessing elements by position.
- Creating Equally Spaced Values: Produces evenly spaced numbers for tasks like graphing, data distribution, or numerical simulations.
- Building List Comprehensions: Simplifies list comprehension syntax by generating numerical sequences for concise element creation.
- Memory-Efficient Iteration: Avoids creating large lists in memory, optimizing performance for large sequences.
Syntax of Python range()
Function
The syntax of the Python range() function is simple and straightforward. It takes three arguments: start, stop, and step. The start argument represents the starting value of the sequence, while the stop argument represents the exclusive end value. The step argument determines the increment between each value in the sequence.
Syntax of Python Range
range(start, stop, step)
Explanation
- start:
- The first number in the sequence (inclusive).
- Defaults to 0 if not specified.
- stop:
- The first number is not included in the sequence (exclusive).
- Required for range() to function.
- step:
- The increment between numbers in the sequence.
- Defaults to 1 if not specified.
- Can be positive or negative to control direction.
Example: Python range(stop)
for i in range(5):
print(i) # Iterates through the sequence 0, 1, 2, 3, 4
Python range(stop) Parameter: range(stop) generates a sequence from 0 to stop-1.
Explanation
for i in range(5):
:- for: Initiates a loop that iterates through a sequence of numbers.
i in range(5)
: Specifies the sequence usingrange()
:- start: Implicitly defaults to 0, so the sequence starts at 0.
- stop: 5, indicating the endpoint (not included).
- step: Implicitly defaults to 1, so the sequence increments by 1 in each step.
- print(i): Prints the current value of i in each loop iteration.
Example: Python range(start, stop)
for i in range(2, 6):
print(i) # Prints 2, 3, 4, 5
Python range(start, stop) Parameters: range(start, stop) generates a sequence from start to stop-1.
Explanation
for i in range(2, 6):
:i in range(2, 6)
: Specifies the sequence usingrange()
.- 2: The starting number of the sequence (inclusive).
- 6: The endpoint of the sequence (exclusive, not included).
- step: Implicitly defaults to 1, meaning the sequence increments by 1 in each step.
Example: Python range(start, stop, step)
for i in range(1, 10, 2):
print(i) # Prints 1, 3, 5, 7, 9
Python range(start, stop, step) Parameters: range(start, stop, step) generates a sequence with defined increments.
Explanation
for i in range(1, 10, 2):
:i in range(1, 10, 2)
: Specifies the sequence usingrange()
:- 1: Starting number (inclusive).
- 10: Endpoint (exclusive, not included).
- 2: Increment (steps by 2 in each iteration).
Incrementing the Range using a Positive Step
Python Range function allows you to generate a sequence of numbers. By default, it starts from 0 and increments by 1. However, you can customize the range by specifying a positive step value. For example, if you use a step value of 2, the range will generate every second number in the sequence. This flexibility in incrementing the range using a positive step is particularly useful when iterating over specific elements or skipping certain values in our code.
Example
for i in range(0, 10, 2):
print(i) # Prints 0, 2, 4, 6, 8
Positive step increases the sequence incrementally.
Explanation
for i in range(0, 10, 2):
:- for: Initiates a loop that iterates through a sequence of numbers.
i in range(0, 10, 2)
: Sets the sequence usingrange()
:- 0: Starting number (inclusive).
- 10: Endpoint (exclusive, not included).
- 2: Increment (steps by 2 in each iteration).
Python range()
using Negative Step
Python Range function allows you to specify the direction in which the sequence should be generated using negative step values. This feature enables you to create sequences in reverse order, starting from a higher number and decrementing by a specified step value. The range() function with negative steps offers flexibility and convenience when dealing with scenarios that require iterating through a sequence backward or generating decreasing sequences in Python programming.
Example
for i in range(5, 0, -1):
print(i) # Prints 5, 4, 3, 2, 1
Negative step generates a sequence in reverse.
Explanation
for i in range(5, 0, -1):
:- for: Initiates a loop that iterates through a sequence of numbers.
i in range(5, 0, -1)
: Sets the sequence usingrange()
:- 5: Starting number (inclusive).
- 0: Endpoint (exclusive, not included).
- -1: Decrement (steps downward by 1 in each iteration).
Python range()
using reversed()
function
By combining the range() function with the reversed() function, you can reverse the order of the generated sequence. This allows you to iterate over a range of numbers in descending order.
Syntax
reversed(range(start, stop, step))
Explanation
- range(…): Creates the initial sequence of numbers.
- reversed(…): Reverses the order of elements in an iterable (like a sequence or range).
Example
for i in reversed(range(5)):
print(i) # Prints 4, 3, 2, 1, 0
Explanation
for i in reversed(range(5)):
:- for: Initiates a loop that iterates through a sequence of numbers.
reversed(range(5))
:range(5)
: Creates a sequence from 0 to 4 (excluding 5).reversed()
: Reverses the order of this sequence.
Concatenation of two range()
functions
In Python, the concatenation of two range() functions allows for creating a single sequence encompassing multiple ranges. This can be achieved by using the list() function to convert each range into a list and then combining them using the addition operator (+).
Syntax
import itertools
concatenated = list(itertools.chain(range(start1, stop1), range(start2, stop2), ...))
Explanation
import itertools
: Imports the itertools module for working with iterators.concatenated = list(itertools.chain(...))
:itertools.chain()
: Combines multiple iterables (like ranges) into one.list(...)
: Converts the combined iterator into a list for storage and manipulation.
- range(start1, stop1), range(start2, stop2), …: Separate range() objects for each desired segment.
Example
import itertools
concatenated = list(itertools.chain(range(3), range(4, 7)))
print(concatenated) # Prints [0, 1, 2, 4, 5, 6]
Use itertools.chain() to concatenate two ranges.
Explanation
- Combines range(0, 3) (0, 1, 2) with range(4, 7) (4, 5, 6).
- Skips the number 3.
- Creates the final list [0, 1, 2, 4, 5, 6].
Accessing range()
with an Index Value
Python Range function allows you to generate a sequence of numbers. You can retrieve an element from the range using its corresponding index position. By accessing range() with an index value, you can easily manipulate and work with specific elements within the sequence.
Example
r = range(10)
print(r[3]) # Prints 3
Access elements in a range using indexing.
Convert range to a List
r = list(range(10))
: Creates a list containing the numbers 0 to 9.print(r[3])
: Accesses and prints the 4th element (index 3), which is 3.
Use a Loop
for i in range(10):
: Iterates through the range.if i == 3:
: Checks for the desired index.print(i)
: Prints the value 3 when found.
Convert range()
to list
In Python, the range() function is commonly used to generate a sequence of numbers. However, if you want to convert this sequence into a list, you can easily do so by using the list() function. By passing the range object as an argument to the list() function, it will create a list containing all the numbers within the specified range. This can be useful when you need to perform operations or manipulate the sequence of numbers in a more flexible manner. So, whether you need to iterate through the numbers or access them individually, converting range() to a list provides you with greater control and flexibility in your Python programming endeavors.
Syntax
list(range(start, stop, step))
Convert a range to a list to visualize or manipulate the sequence.
Explanation
- range(start, stop, step): Generates a sequence of numbers based on parameters:
- start: Inclusive starting number (defaults to 0 if omitted).
- stop: Exclusive endpoint (not included in the generated sequence).
- step: Increment or decrement between numbers (defaults to 1).
- list(…): Converts the range object into an actual list, allowing for list operations.
Example
numbers = list(range(5))
print(numbers) # Prints [0, 1, 2, 3, 4]
Explanation
range(5)
: Creates a sequence from 0 to 4 (stops before 5).list(...)
: Converts this sequence into [0, 1, 2, 3, 4].
Skipping Numbers
- To skip numbers, adjust the step value:
- numbers = list(range(0, 10, 2)): Creates [0, 2, 4, 6, 8], skipping every other number.
- numbers = list(range(10, 0, -2)): Creates [10, 8, 6, 4, 2], descending with skips.
range()
vs xrange()
in Python 2
In Python 2, two functions are available for generating a sequence of numbers: range() and xrange(). While both functions serve a similar purpose, they have a key difference. Python range() function returns a list containing all the numbers in the specified range, which can consume a considerable amount of memory for large ranges. On the other hand, the xrange() function returns an iterator object that generates numbers on-the-fly as needed, resulting in more efficient memory usage. Therefore, if you are working with large ranges and memory efficiency is crucial, using xrange() instead of range() can be advantageous in Python 2.
In Python 3, the xrange() function was removed, and range() now behaves like the efficient xrange() from Python 2.
Syntax (Both)
range(start, stop, step)
xrange(start, stop, step) # Python 2 only
Example
# range() creates a list
numbers = range(10) # Generates a list [0, 1, 2, ..., 9]
print(numbers[3]) # Prints 3
# xrange() generates numbers as needed
numbers = xrange(10) # Generates numbers on-the-fly
for num in numbers: # Iterates without creating a full list
print(num)
Explanation
numbers = range(10)
: Creates a generator for numbers 0 to 9 (efficient).print(numbers[3])
: Raises an error in Python 3, as generators aren’t subscriptable. To access elements, convert to a list:numbers_list = list(numbers)
: Creates the list [0, 1, 2, …, 9].print(numbers_list[3])
: Prints 3 (accessing the 4th element).
for num in numbers:
: Iterates directly through the generator without creating a list, saving memory for large ranges.
Example
# Python 2
numbers_list = range(1000000) # Consumes memory for all 1 million numbers
numbers_generator = xrange(1000000) # Efficient, generates numbers as needed
Key Differences
Memory Usage
- range(): Creates a full list of numbers in memory, potentially consuming significant space for large ranges.
- xrange(): Generates numbers on-the-fly, more memory-efficient for large ranges.
Type
- range(): Returns a list, allowing direct access and slicing.
- xrange(): Returns an xrange object, not subscriptable (can’t use [] for access).
Performance
- range(): Can be slower for large ranges due to list creation overhead.
- xrange(): Often faster for large ranges due to on-demand generation.
Best Practices
- Python 2: Use xrange() for large ranges to optimize memory usage.
- Python 3: Use range() without concerns, as it offers both efficiency and list-like functionality.
Benefits of Using Range
Python’s built-in function, range(), offers a range of advantages for programmers. Whether you’re working on a small script or a complex program, harnessing the power of range() can greatly enhance your coding experience. From simplifying loops to optimizing memory usage, the benefits of using range() are numerous and impactful.
Here are some of the Benefits of Python Range:
- Efficient way to generate a sequence of numbers in Python.
- Saves memory as it generates numbers on the fly, rather than storing them all at once.
- Allows for easy iteration over a specific range of values.
- Provides flexibility by accepting start, stop, and step arguments to define the range.
- Supports negative step values for iterating in reverse order.
- Can be combined with other Python functions like len() or list() to further manipulate the generated sequence.
Common Pitfalls and Considerations
When working with the Python Range function, it’s important to be aware of common pitfalls and considerations. Understanding these nuances can help prevent errors and ensure accurate results.
Common Pitfalls and Considerations When Using Python range() Function:
- Misunderstanding the behavior of the Python range function can lead to unexpected results.
- Forgetting that the end value in the range function is exclusive and not inclusive.
- Not considering the step argument in the range function, which allows for iterating over a sequence with a specific interval.
- Overlooking the fact that negative values can be used as arguments in the range function to iterate backward.
Conclusion
Python Range function is valuable for any programmer seeking to streamline their code and improve efficiency. With its simplicity and versatility, the range function has become an essential component in many Python programs. It offers a convenient way to iterate over a sequence of numbers without manually creating them. By effectively using the range function, programmers can enhance their code and improve its readability.
Also Read
15+ Popular Python IDE and Code Editors
Python Reference
FAQ
What is the purpose of the range() function in Python?
It efficiently generates sequences of numbers, often used for iterating through loops or creating lists without consuming excessive memory.
How does range() work in Python 2 compared to Python 3?
In Python 2, range() creates a full list, while xrange() is a generator. Python 3 unifies them, making range() behave like the efficient xrange().
What are the parameters of the range() function?
start: Inclusive beginning number (defaults to 0).
stop: Exclusive endpoint (not included in the sequence).
step: Increment or decrement between numbers (defaults to 1).
Can I access elements of a range() object directly?
No, range() objects aren’t subscriptable. Convert to a list using list(range(…)) for direct element access.
How do I create a reversed range in Python?
Use reversed(range(…)) to generate a reversed sequence of numbers, conserving memory compared to creating a reversed list.