Level up your React development with ES6! Write cleaner, more efficient code using arrow functions, classes, template literals, and more. Build modern, maintainable web applications with ease.
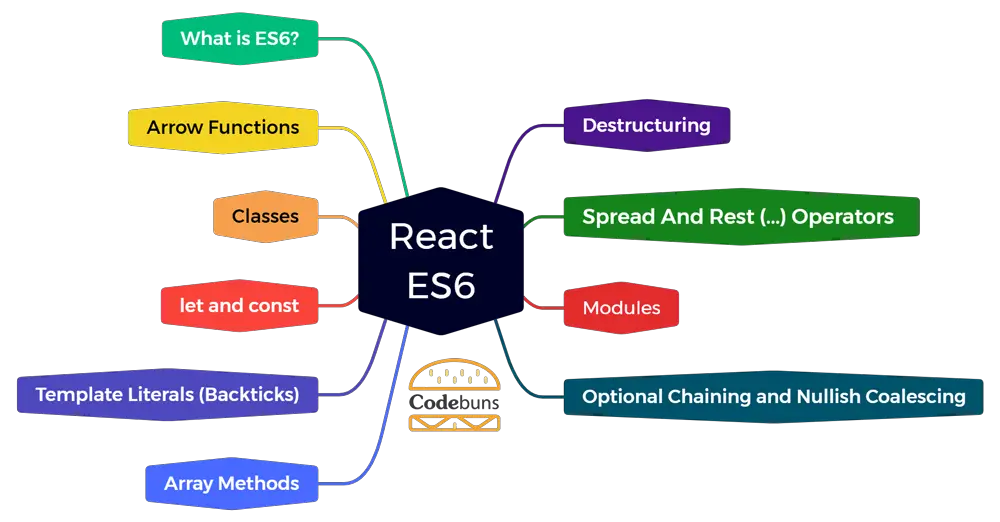
Table of Contents | |
What is ES6?
ES6, also known as ECMAScript 2015, signifies a significant upgrade to the JavaScript language. It introduced many new features that enhance code readability, maintainability, and expressiveness. But why is ES6 particularly important for React development?
- Modernization: ES6 aligns perfectly with React’s clean and concise code philosophy. Features like arrow functions and template literals streamline your React components, making them easier to write and understand.
- Enhanced Readability: ES6 introduces
let
andconst
for variable declarations, improving code clarity and preventing unintended variable reassignments. This is crucial for managing state and props effectively within React components. - Streamlined Data Handling: Destructuring and the spread operator empower you to work with complex data structures like objects and arrays more efficiently within React components. This simplifies prop and state management, leading to cleaner and more maintainable code.
Simple Example of React ES6
function Greeting(name) { const message = () => { // Arrow function for conciseness return `Hello, ${name}!`; }; return ( <div> <h1>{message()}</h1> {/* Calling the arrow function */} </div> ); }
Explanation
function Greeting(name) {...}
: Defines aGreeting
component that accepts aname
prop.const message = () => {...}
: An arrow function is defined to create the greeting message.- The component returns JSX that displays the message returned by the
message
function.
Arrow Functions in React ES6
Arrow functions, introduced in ES6, offer a concise and readable alternative to traditional function expressions in React. They not only improve code clarity but also address a common challenge related to the this
keyword within React class components.
- Concise Syntax: Arrow functions use a shorter syntax compared to traditional functions. This can significantly improve the readability of your React components, especially when dealing with simple logic or callback functions.
- Resolving “this” Puzzle: Traditional functions can introduce ambiguity regarding the
this
keyword within React class components. Arrow functions, however, inherit thethis
value from their surrounding context, eliminating this confusion and simplifying event handling within classes.
Example of Arrow Function in React Class Component
class Button extends React.Component { handleClick = () => { console.log(this.props.message); // Accessing props using "this" }; render() { return ( <button onClick={this.handleClick}>Click Me</button> ); } }
Explanation
class Button extends React.Component {...}
: Defines aButton
class component.handleClick = () => {...}
: An arrow function is defined to handle button clicks.console.log(this.props.message)
: Inside the arrow function,this
refers to theButton
component instance, allowing access to props likemessage
.- The
render
method returns JSX for the button, withonClick
bound to thehandleClick
arrow function.
Let’s compare arrow function example with how the same functionality might be implemented using a traditional function and how it affects the this
behavior:
Traditional Function
class Button extends React.Component { handleClick() { console.log(this.props.message); // Potential 'this' issue } render() { return ( <button onClick={this.handleClick}>Click Me</button> // Notice: no .bind() here! ); } }
Explanation of the Problem
- With traditional functions, the
this
keyword’s value can change depending on the context in which the function is called. In the event handler context (onClick
),this
might not refer to theButton
component instance, leading to errors when accessingthis.props.message
.
Common Solutions with Traditional Functions
1. Using .bind()
in the constructor
constructor(props) { super(props); this.handleClick = this.handleClick.bind(this); // Binding 'this' }
2. Using .bind()
inline
<button onClick={this.handleClick.bind(this)}>Click Me</button>
Key Differences
- Syntax: Traditional functions use the
function
keyword, while arrow functions use the=>
syntax. this
behavior: Arrow functions inheritthis
from the enclosing scope (often the component instance), while traditional functions have variablethis
depending on how they’re called.- Handling
this
: Arrow functions provide a built-in solution to thethis
issue common with React classes, while traditional functions might require additional work using.bind()
.
Classes in React ES6
React offers two main approaches to building components: functional and class components. Introduced before the rise of functional components, class components used ES6 classes to provide a structured way to manage component state and lifecycle.
- State Management: Classes allow you to define and manage internal data specific to the component using the
state
property. This state can be updated based on user interactions or other events. - Component Lifecycle: Classes provide lifecycle methods like
componentDidMount
andcomponentWillUnmount
that hook into different stages of a component’s existence, enabling you to perform actions at specific points in time. - Inheritance (Optional): Classes enable inheritance, allowing you to create new components that inherit properties and behaviors from existing ones, promoting code reusability.
While React now leans towards functional components, understanding classes with ES6 features remains valuable, especially for existing codebases. Here’s a basic example of a class component that simply displays a greeting:
class Greeting extends React.Component { render() { return ( <div> <h1>Hello from a React Class Component!</h1> </div> ); } }
Explanation
class Greeting extends React.Component {...}
: Defines aGreeting
class component inherited fromReact.Component
.render() {...}
: This method is required in React components. It defines what the component will render on the screen.return (...)
: Therender
method returns JSX that defines the component’s UI structure.<div>...</div>
: Create adiv
element containing the greeting message.<h1>Hello from a React Class Component!</h1>
: Defines a heading element (h1
) displaying the greeting message.
let
and const
in ES6
ES6 introduced let
and const
as powerful alternatives to the traditional var
keyword for variable declarations in JavaScript. These enhancements improve code clarity, prevent errors, and make your React components more maintainable.
- Block-Level Scope: Unlike
var
, which has function scope,let
andconst
have block-level scope. This means they are only accessible within the code block (like an if statement or loop) where they are declared, preventing accidental variable conflicts. - Reassignment Control:
const
enforces immutability, meaning the value assigned to a constant variable cannot be changed after its declaration. This promotes predictable behavior and helps avoid unintended side effects in your React components. - Clarity and Readability: Using
let
andconst
explicitly conveys the intent behind a variable.const
for fixed values andlet
for variables that might need to change, which improves code readability and maintainability.
Example of let
and const
in a React Component
function Greeting(name) { const message = "Hello, "; // Constant message let fullName = message + name; // Let for changeable full name return ( <div> <h1>{fullName}</h1> </div> ); }
Explanation
const message = "Hello, ";
: A constant variablemessage
is declared with a fixed greeting.let fullName = message + name;
: Alet
variablefullName
is declared and assigned the combined message and name using string concatenation.- The component returns JSX that displays the
fullName
variable’s value.
Template Literals (Backticks) in React ES6
Introduced in ES6, template literals offer a more flexible and readable way to create strings than traditional concatenation. They are particularly useful for building dynamic strings within React components.
- Enhanced Readability: Template literals allow you to embed expressions directly within strings using
${expression}
syntax. This improves readability, especially when dealing with complex or dynamic string construction. - Multi-Line Strings: Backticks (
`
) delimit template literals, enabling you to create multi-line strings without cumbersome concatenation or extra escape characters. This can significantly improve code clarity when dealing with formatted text or multi-line content. - Interpolation: The
${expression}
syntax allows you to seamlessly integrate variable values or expressions into your strings. This eliminates the need for explicit concatenation, making your code more concise and easier to maintain.
Example of a Template Literal in a React Component
function Greeting(name) { const message = `Hello, ${name}! How are you today?`; return ( <div> <h1>{message}</h1> </div> ); }
Explanation
const message = \
Hello, ${name}! How are you today?`;: A constant variable${name}
within the string: This expression inserts the value of thename
prop into the greeting message.- The component returns JSX that displays the
message
variable, showcasing the dynamic greeting.
Array Methods in React ES6
ES6 brought a wealth of array methods that streamline working with lists in JavaScript. These methods empower you to efficiently manipulate, filter, and transform your data within React components.
- Concise Data Handling: Array methods like
map
,filter
, andreduce
offer concise syntax for processing arrays. This can significantly improve code readability and maintainability compared to traditional loops. - Improved Performance: Many array methods are optimized for performance, especially with large datasets. This can benefit the overall responsiveness of your React applications.
- Declarative Approach: These methods promote a declarative style of coding. You describe what you want to achieve with the data, and the method handles the underlying logic, making your code easier to understand and reason about.
Example of map
Method to Create a List of Greetings in a React Component
function UserList(users) { const greetings = users.map(user => ( <li key={user.id}>Hello, {user.name}!</li> )); return ( <ul> {greetings} </ul> ); }
Explanation
const greetings = users.map(user => (...))
: Themap
method iterates over theusers
array and creates a new array (greetings
) containing JSX elements.user => (...)
: An arrow function defines the logic for each element.<li key={user.id}>Hello, {user.name}!</li>
: This JSX creates a list item with a greeting for each user, using theuser.id
for the key anduser.name
for the message.
- The component returns JSX, which displays an unordered list (
<ul>
) containing the elements of thegreetings
array.
Destructuring in React ES6
Destructuring, a powerful ES6 feature, allows you to unpack values from objects and arrays more concisely and readable. This is particularly beneficial when dealing with props and state within React components.
- Cleaner Prop Access: Destructuring enables you to extract specific properties from props objects passed to your React components. This eliminates the need for repetitive prop name references, improving code readability.
- State Management: Destructuring can streamline working with state objects within React components. By unpacking specific state properties, you can access and update them more efficiently.
- Enhanced Readability: Destructuring promotes cleaner and more readable code, especially when dealing with complex objects or nested data structures within your React components.
Example of Destructuring Props in a React Component
function UserDetails({ name, email }) { return ( <div> <h1>{name}</h1> <p>Email: {email}</p> </div> ); }
Explanation
function UserDetails({ name, email }) {...}
: The component accepts props and destructures them directly in the function parameters. This meansname
andemail
props can be accessed directly within the component without the need forprops.name
orprops.email
.- The component returns JSX that displays the destructured
name
andemail
props, demonstrating how destructuring simplifies prop access.
Spread And Rest (...
) Operators
The spread (...
) and rest (...
) operators, introduced in ES6, offer versatile tools for working with data in JavaScript. These operators prove particularly useful when manipulating arrays and objects within React components.
- Spreading the Elements: The spread operator (
...
) efficiently expands an iterable (like an array or object) into individual elements. This can be helpful when creating copies of arrays or objects or when passing elements as separate arguments to functions. - Collecting the Rest: The rest operator (
...
) acts as a collector, gathering remaining elements from an iterable into a new array. This is useful when working with function arguments where you might not know the exact number of arguments in advance. - React Applications: Both spread and rest operators can streamline data handling within React components. They simplify tasks like copying state objects, merging data, and handling variable-length prop lists.
Spread Operator (...
)
When used within another data structure, the spread operator efficiently expands iterables (like arrays or objects) into individual elements.
Example
import React, { useState } from 'react'; // Added useState import function ItemList(initialItems) { const [items, setItems] = useState(initialItems); // Initial state with items const addItem = (newItem) => { setItems([...items, newItem]); // Spread operator to add a new item }; return ( <div> <ul> {items.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> <button onClick={() => addItem({ id: 3, name: "New Item" })}> Add Item </button> </div> ); }
Explanation
- The component has an
items
state array initialized withinitialItems
. - The
addItem
function takes anewItem
object as an argument. - Inside
setItems
, the spread operator (...
) creates a new array. It spreads the existingitems
array and then appends thenewItem
object, ensuring the originalitems
array remains unchanged. - When the button is clicked, the
addItem
function is called with a new item object, effectively adding it to the list.
Rest Operator (...
)
The rest operator (...
) gathers remaining elements from an iterable into a new array. It’s useful when working with an unknown number of arguments.
Example
function UserCard({ name, role, ...otherInfo }) { // Destructuring with rest return ( <div> <h1>{name}</h1> <p>Role: {role}</p> {otherInfo.email && <p>Email: {otherInfo.email}</p>} // Using otherInfo </div> ); } // Example usage when calling the component: <UserCard name="Alice Johnson" role="Developer" email="alice@example.com" location="New York City" />
Explanation
- Passing Additional Props: When calling the
UserCard
component, we now pass additional properties:email
andlocation
. - Conditional Rendering: We conditionally render an email element inside the component if the
otherInfo.email
property exists. This demonstrates how the rest operator can help handle optional or extra props.
How the Rest Operator Works Here
The rest operator (...otherInfo
) in the function parameters collects all additional properties passed to the UserCard
component (in this case, email
and location
) and stores them within the otherInfo
object. This object can then be used flexibly within the component.
Modules in React ES6
As your React projects grow, code organization becomes crucial. ES6 modules offer a powerful way to break down your code into reusable and manageable pieces. This promotes better code structure, maintainability, and collaboration.
- Improved Structure: Modules allow you to group related functions, components, and data into separate files. This enhances code readability and makes it easier to find specific functionalities within your React application.
- Reusability: By exporting functions and components from modules, you can reuse them across different parts of your application. This reduces code duplication and promotes consistency.
- Collaboration: Modules facilitate collaboration on larger projects. Different developers can work on separate modules without worrying about conflicts in the global namespace.
Example of Module for Formatting Greetings in React (ES6)
greetings.js (Module File)
export function formatGreeting(name) { return `Hello, ${name}!`; }
Explanation
export function formatGreeting(name) {...}
: Defines a function namedformatGreeting
that takes aname
argument.export
: This keyword marks the function as available for export from the module.
App.js (Component File)
import { formatGreeting } from './greetings.js'; // Import the function function App() { const userName = "Mark"; const greeting = formatGreeting(userName); return ( <div> <h1>{greeting}</h1> </div> ); }
Explanation
import { formatGreeting } from './greetings.js'
: Imports theformatGreeting
function from thegreetings.js
module.- The component then uses the imported function to format a greeting with the
userName
and displays it in the JSX.
Optional Chaining and Nullish Coalescing in React ES6
Modern JavaScript introduces two features that help you handle potential errors when working with nested objects or properties that might be null or undefined: Optional Chaining (?.
) and Nullish Coalescing (??
). These tools improve code readability and prevent runtime errors in your React applications.
- Safe Navigation: Optional chaining (
?.
) allows you to safely access properties of nested objects without worrying if intermediate objects in the chain are null or undefined. It gracefully returnsundefined
if any part of the chain is nullish, preventing errors. - Default Values: Nullish coalescing (
??
) provides a way to specify a default value in case an expression evaluates tonull
orundefined
. This eliminates the need for complex conditional checks and improves code conciseness. - Focus on React: While both features are generally useful in JavaScript, they become especially valuable in React when dealing with potentially nullish data coming from props or state.
Example
function UserAvatar(props) { const avatarUrl = props.user?.avatarUrl ?? "default-avatar.png"; return ( <img src={avatarUrl} alt="User Avatar" /> ); }
Explanation
const avatarUrl = props.user?.avatarUrl ?? "default-avatar.png";
:props.user?.avatarUrl
: Optional chaining is used to access theavatarUrl
property of theprops.user
object. Ifprops.user
is null or undefined, it returnsundefined
without causing an error.?? "default-avatar.png"
: Nullish coalescing checks ifprops.user?.avatarUrl
is nullish. If it is, the default value “default-avatar.png” is assigned toavatarUrl
.
- The component returns JSX that displays an image using the
avatarUrl
, ensuring a default image is used if the user doesn’t have a profile picture.
Conclusion
Mastering ES6 features is essential for building modern and robust React applications. ES6 advancements like arrow functions, classes, template literals, destructuring, and more streamline your development process. These enhancements lead to cleaner, more readable, and maintainable code, making your React components easier to understand and manage. Understanding how to use array methods, the spread and rest operators, and modules empowers you to handle data effectively and organize your projects intelligently. As your React development skills progress, these ES6 tools will become second nature, allowing you to focus on creating truly dynamic and user-friendly interfaces.