React JSX: Simplify your React development by writing familiar HTML-like structures directly within your JavaScript code. JSX makes component creation intuitive and improves code readability, allowing you to focus on the core functionality of your components.
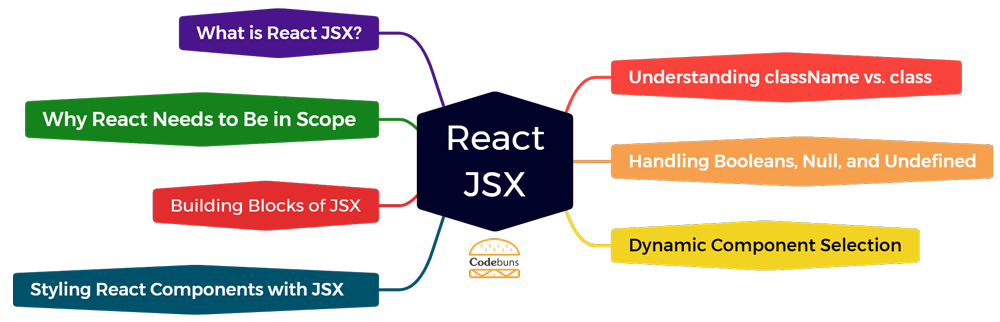
Table of Contents | |
What is React JSX?
React JSX (JavaScript Syntax Extension) is a special syntax that allows you to write HTML-like code directly inside your JavaScript files. It’s not actual HTML but rather a way to describe what your UI components will look like. JSX makes React code easier to read and understand because it keeps your component’s structure and logic together in one place. While JSX is optional, most React developers find it useful for building user interfaces.
Example: JSX inside a functional React component
function MyComponent() { // This line returns a JSX element return <h1>Hello, world!</h1>; }
Explanation
- function MyComponent() { … }: Defines a functional React component named MyComponent.
- return <h1>Hello, world!</h1>;: Returns a JSX element. The <h1> tag defines a heading element, and the text content inside it is “Hello, world!”. This JSX element describes the UI that this component will render.
React JSX follows specific capitalization conventions for components and HTML elements. These conventions ensure consistency and clarity in your React codebase.
Components
Component names should always start with a capital letter (PascalCase). This helps differentiate components from built-in HTML elements and avoids naming conflicts with JavaScript variables.
HTML Elements
Built-in HTML elements used within JSX should be written in lowercase ( kebab-case is not recommended for HTML elements in JSX). This aligns with standard HTML conventions and improves readability.
- MyComponent starts with uppercase letters (PascalCase).
- h1 element is written in lowercase, following standard HTML conventions within JSX.
Why React Needs to Be in Scope
In React, JSX allows you to write code that looks like HTML but gives you the power of JavaScript. However, for JSX to work properly, React must be in scope. Here’s why:
JSX Transformation
React treats JSX code differently than normal JavaScript. When you write JSX, it undergoes a transformation during the build process. This transformation converts the JSX syntax into regular JavaScript function calls that React understands.
React.createElement
The React.createElement function is a core building block in React. It takes component names, props, and child elements as arguments and returns a React element object that describes your UI. JSX gets transformed into calls to React.createElement behind the scenes.
Without React in scope
If React isn’t available, the JSX transformation fails. You’ll encounter errors because the code that interprets and handles JSX syntax won’t be found.
Example: React not in Scope
// This will cause an error because React is not imported function MyComponent() { return <h1>Hello, world!</h1>; }
The code will throw an error because React is not imported, making React.createElement unavailable for JSX transformation.
Example: React in Scope
import React from 'react'; // Import React from 'react' function MyComponent() { return <h1>Hello, world!</h1>; }
import React from ‘react’;: Imports the React library from the react package. This makes the React object available within your code.
With React imported, the JSX code gets transformed during the build process. The transformation creates a call to React.createElement similar to:
return React.createElement('h1', null, 'Hello, world!');
This transformed code defines a React element object that React understands and can use to render the UI. When your application runs, React will render the “Hello, world!” message on the screen.
Building Blocks of JSX
Building Blocks of JSX refer to the fundamental elements and concepts that make up the JSX syntax. These building blocks include:
- Nesting Elements
- Embedding JavaScript Expressions
- String Literals
- Comments
Nesting Elements
React JSX allows you to create complex UIs by nesting elements within each other, similar to how HTML works. You can combine smaller JSX elements to represent more intricate UI components. This nesting capability lets you organize your UI in a hierarchical way, making it easier to manage as your components grow.
Example: Nesting JSX elements
function ProfileCard() { // This line returns a JSX element with nested elements return ( <div className="profile-card"> <h2>John Doe</h2> <p>Software Engineer</p> </div> ); }
Explanation
- return ( … ): Returns a JSX element, in this case, a <div> with the class name “profile-card”.
- <div className=”profile-card”> … </div>: This div element acts as a container and groups the nested elements.
- <h2>John Doe</h2>: Creates an <h2> element (heading) with the text content “John Doe”.
- <p>Software Engineer</p>: Creates a <p> element (paragraph) with the text content “Software Engineer”. We build a structured profile card component by nesting these elements within the div.
Embedding JavaScript Expressions
React JSX lets you add dynamic behavior to your UI by embedding JavaScript expressions in curly braces { } within your JSX code. These expressions are evaluated before rendering, allowing you to include variables, function calls, or complex logic directly inside your component’s structure. This powerful feature makes JSX code adaptable and data-driven, ensuring your UI reflects the latest information in your application.
Example: Embedding a JavaScript Expression within JSX
function Greeting(props) { // Embed a variable 'name' from props using curly braces const name = props.name; return ( <h1>Hello, {name}!</h1> ); }
Explanation
- const name = props.name;: Declares a variable named name and assigns the value of the name prop passed to the component.
- <h1>Hello, {name}!</h1>: This JSX element includes the name variable wrapped in curly braces {}. When the component renders, the value of name is inserted into the string, creating a greeting message.
String Literals
React JSX allows you to define attributes for your elements using string literals. These attributes provide additional information or functionalities to your UI components.
- Key-Value Pairs: Attributes are specified as key-value pairs within the opening tag of a JSX element.
- String Values: String literals are commonly used for attribute values that represent static text, IDs, or class names.
- Dynamic Values: You can also use curly braces {} to embed JavaScript expressions within string literals for dynamic attribute values based on props or variables.
Example: String Literals within JSX
function Button(props) { // Button text from props const text = props.text; return ( <button className="primary-button" onClick={() => alert("Clicked!")}> {text} </button> ); }
Explanation
- className=”primary-button”: Attribute sets the CSS class for styling.
- onClick={() => alert(“Clicked!”)}: Attribute defines an event handler using an arrow function that displays an alert message when clicked.
Comments
Adding comments to your React JSX code improves readability and maintainability. Comments explain the purpose of specific code sections or provide notes for future developers. There are two ways to add comments in React:
- Regular JavaScript comments: Use // for single-line comments or /* */ for multi-line comments outside JSX elements.
- JSX comments: Wrap your comment within curly braces {/* comment */} when commenting directly inside JSX code.
Example
function TodoItem(props) { // Destructure props for clarity (commented out for explanation) // const { text, completed } = props; // Text content for the todo item const text = props.text; // Flag indicating completion status const completed = props.completed; return ( <li /* Comment: Todo item element */ className={completed ? "completed" : ""}> {/* Comment: Display todo text */} {text} </li> ); }
Explanation
- Lines commented out with //: Demonstrate destructuring props for readability, but they are commented out to focus on explaining comments.
- /* Comment: Todo item element */: Explains the purpose of the <li> element, which represents a single todo item.
- {/* Comment: Display todo text */}: Clarifies the purpose of displaying the text variable inside the <li> element.
Styling React Components with JSX
Styling React components with JSX refers to the various methods you can use to apply visual styles and customize the appearance of your React components. This includes techniques like:
- Inline styles
- CSS stylesheets
- CSS Modules
- Styled-Components
Inline Styles
React JSX allows you to apply styles directly to your UI elements using inline styles. This approach involves defining a JavaScript object containing styles as key-value pairs and assigning it to the style attribute within your JSX element. While inline styles can be convenient for simple styling, they can become cumbersome for complex components or managing styles across multiple components.
Example
function Heading(props) { // Set the heading size based on props (default is 1) const size = props.size || 1; return ( <h{size} style={{ color: "blue", fontSize: size * 2 + "em" }}> {props.children} </h{size}> ); }
Explanation
- const size = props.size || 1;: Defines a variable size that retrieves the size prop value or defaults to 1 if not provided.
- <h{size} … >: JSX element dynamically selects the heading level (h1, h2, etc.) based on the size variable.
- style={{ color: “blue”, fontSize: size * 2 + “em” }}: This inline style object sets the font color to blue and adjusts the font size based on the size value (multiplied by 2 and appended with “em” unit).
- {props.children}: Displays the content passed to the component using props.children, allowing you to customize the heading text.
CSS Classes
You can leverage CSS classes to create more maintainable and reusable styles in React JSX. This approach involves creating separate CSS files containing your styles and referencing those classes within your JSX elements using the className attribute. This separation of concerns keeps your component code clean and your styles organized in one location.
Example
import styles from "./styles.module.css"; // Import styles from a CSS module function Button(props) { return ( <button className={styles.primaryButton}> {props.text} </button> ); }
Explanation
- import styles from “./styles.module.css”;: Imports styles from a CSS module named styles.module.css. CSS modules ensure style names are unique within your component, preventing naming conflicts.
- <button className={styles.primaryButton}>: JSX element defines a button and assigns the CSS class named primaryButton from the imported styles to the className attribute.
- {props.text}: Displays the button text passed as a prop within the button element.
CSS Modules
CSS Modules offer a way to manage component-specific styles in React JSX. They help prevent naming conflicts between CSS classes used across different parts of your application. Here’s how it works:
- CSS Module File: Create a CSS file with the .module.css extension (e.g., MyComponent.module.css).
- Class Name Generation: During the build process, CSS Modules automatically generate unique class names based on the filename and class name defined within the CSS file.
- Importing and Applying Styles: Import the CSS module file into your React component. Use the generated class names within your JSX elements to apply styles.
Example
import React from 'react'; import styles from './MyComponent.module.css'; // Import CSS Module function MyComponent() { return ( <div className={styles.container}> <h2 className={styles.heading}>This is a title</h2> <p className={styles.content}>This is some content.</p> </div> ); }
Explanation
- import styles from ‘./MyComponent.module.css’;: Imports styles from the CSS Module file.
- className={styles.container} … className={styles.heading} … className={styles.content}: Uses the generated class names (styles.container, styles.heading, etc.) to apply styles to specific elements within the JSX.
Styled-Components (CSS-in-JS)
Styled-Components is another popular approach for styling React components using JSX. It offers a CSS-in-JS solution that allows you to write CSS code directly within your JavaScript components. Here’s a breakdown of Styled-Components:
- Styled Components: You define styled components using template literals with special syntax. These components behave like regular React components but return styles instead of JSX.
- Component Styling: The styles defined within the styled component are scoped to that specific component, reducing the risk of style conflicts.
- Props and Theming: Styled-components allow you to use props and themes to dynamically adjust styles based on your needs.
Example
import React from 'react'; import styled from 'styled-components'; const Button = styled.button` background-color: #4CAF50; /* Green */ border: none; color: white; padding: 10px 20px; text-align: center; text-decoration: none; display: inline-block; font-size: 16px; margin: 4px 2px; cursor: pointer; `; function MyComponent() { return ( <div> <Button>Click me</Button> </div> ); }
Explanation
- const Button = styled.button**: Defines a styled component named Button that extends the built-in button element.
- background-color: #4CAF50; … : Defines the styles applied to the Button component using CSS syntax within template literals.
- The MyComponent function uses the Button styled component as a regular React component.
Understanding className vs. class
In React JSX, you might encounter two terms for applying CSS classes to elements: className and class. While they might seem interchangeable, there’s a crucial distinction.
className
This is the correct attribute for assigning CSS classes to JSX elements. React uses this attribute to avoid conflicts with the built-in JavaScript keyword class.
class
Although it might seem valid, using class inside JSX is not recommended. It can lead to errors and unexpected behavior. Always use className for CSS class assignments in React components.
Example
function Button(props) { // Set button type based on props (default is 'primary') const type = props.type || "primary"; return ( <button className={`btn btn-${type}`}> {props.children} </button> ); }
Explanation
- const type = props.type || “primary”;: Defines a variable type that retrieves the type prop value or defaults to “primary” if not provided.
- <button className={btn btn-${type}}>: JSX element defines a button element. The className attribute uses template literals (“`) to dynamically construct the class name based on the type variable (e.g., “btn btn-primary” or “btn btn-secondary”).
- {props.children}: Displays the content passed to the component using props.children, allowing you to customize the button text.
Handling Booleans, Null, and Undefined
When working with JSX, you might encounter situations where your data includes boolean values, null, or undefined. React treats these values differently within JSX elements.
Boolean values (true or false)
By default, React treats true as true and renders the content within the JSX element. Conversely, false is treated as false, and the content will not be rendered.
null and undefined
Including null or undefined directly in JSX elements will result in nothing being rendered. This is because React considers them false values.
To conditionally render content based on these data types, you can use techniques like ternary operators or short-circuiting with logical operators (&&).
Example
function IsLoggedIn(props) { // isLoggedIn prop indicates login status const isLoggedIn = props.isLoggedIn; return ( <div> {isLoggedIn ? ( <p>Welcome, you are logged in!</p> ) : ( <p>Please log in to continue.</p> )} </div> ); }
Explanation
- const isLoggedIn = props.isLoggedIn;: Retrieves the login status from the isLoggedIn prop.
- {isLoggedIn ? ( … ) : ( … )}: This ternary operator conditionally renders content based on the isLoggedIn value. If true (truthy), it displays a welcome message. If false (false), it displays a login prompt.
Dynamic Component Selection
React JSX allows you to select which component to render dynamically based on certain conditions. This is useful when you have multiple components with similar functionality but variations in their appearance or behavior. Here’s how you can achieve this:
Conditional Rendering
Use techniques like if statements or ternary operators to evaluate a condition and render the appropriate component based on the result.
Component Lookup
Create a JavaScript object that stores different components as key-value pairs. Use the current state or props to determine the key, then access the corresponding component from the lookup object.
Example
function ProductDisplay(props) { // Product type from props const type = props.type; // Component lookup object const productComponents = { "electronics": ElectronicsProduct, "clothing": ClothingProduct, }; // Select the component based on product type const SelectedProductComponent = productComponents[type]; // Render the selected component if it exists return SelectedProductComponent ? ( <SelectedProductComponent {...props} /> ) : ( <p>Invalid product type</p> ); } function ElectronicsProduct(props) { // ... Specific content and logic for electronic products } function ClothingProduct(props) { // ... Specific content and logic for clothing products }
Explanation
- productComponents object stores references to ElectronicsProduct and ClothingProduct components.
- SelectedProductComponent uses the type prop to retrieve the corresponding component from the lookup object.
- The conditional rendering ensures that only the selected component is rendered, displaying an error message if the type is invalid.
Conclusion
React JSX provides a powerful and convenient way to create the structure and layout of your React components. Its blend of HTML-like syntax with the flexibility of JavaScript makes defining UIs simpler and more intuitive. By allowing you to nest elements, embed dynamic content using expressions, and customize elements with attributes, JSX enables you to build rich and interactive experiences within your React applications. While it might require an initial learning curve, mastering React JSX will make your development journey faster, smoother, and more enjoyable.