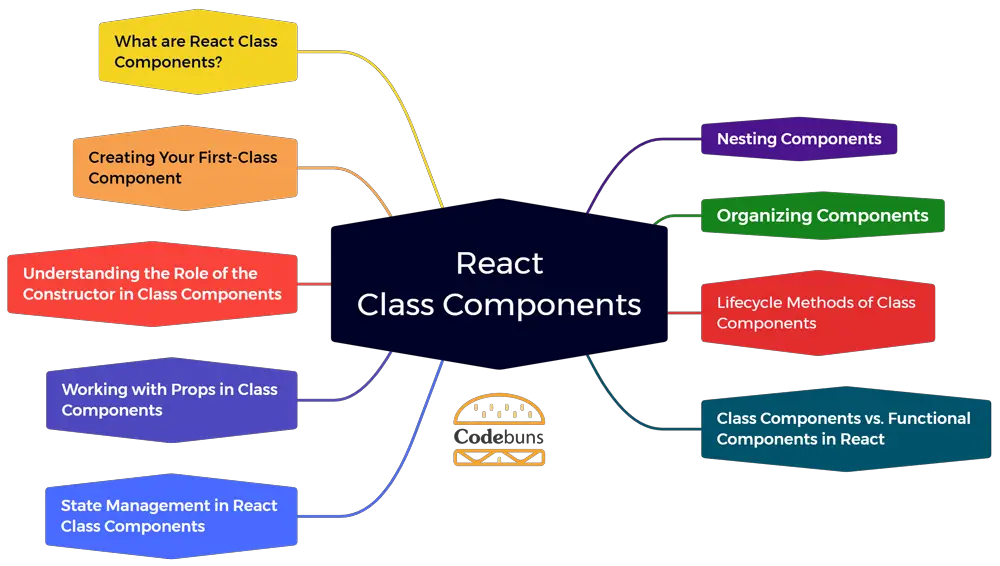
Table of Contents | |
What are React Class Components?
In the early days of React, class components were the primary way to create reusable UI elements. They are built upon JavaScript classes, offering a structured approach to managing state and defining component behavior. While functional components are now recommended for new projects, understanding class components remains valuable, especially for existing codebases.
Example of a React Class Component
class Greeting extends React.Component {
render() {
return (
<div>
<h1>Hello from a React Class Component!</h1>
</div>
);
}
}
Explanation
class Greeting extends React.Component {...}
: This line defines a class component namedGreeting
that inherits functionalities fromReact.Component
.render() {...}
: This method is required in React components. It defines what the component will render on the screen.return (...)
: Therender
method returns JSX that defines the component’s structure. In this case, adiv
element containing a heading with the greeting message.
Creating Your First-Class Component
Creating your first React class component is fundamental to understanding React development. These components offer a structured approach to building reusable UI elements with state management capabilities. Here’s a breakdown of the essential steps:
- Define the Class: Use the
class
keyword followed by a name (e.g.,MyComponent
) and extendReact.Component
to inherit core functionalities. - Constructor (Optional): The constructor (
constructor(props) {...}
) is a special method for initializing state or binding event handlers. - Render Method: Every React component needs a
render()
method. This method returns JSX code that defines the component’s visual structure. - Return JSX: The
render()
method should return JSX elements representing the UI content your component will display.
Example
class Message extends React.Component {
constructor(props) {
super(props); // Call the parent constructor (optional)
}
render() {
return (
<div>
<h1>{this.props.message}</h1>
</div>
);
}
}
Explanation
class Message extends React.Component {...}
: Defines a class component namedMessage
inheriting fromReact.Component
.constructor(props) {...}
: An optional constructor that can be used for initialization. Here,super(props)
calls the parent constructor (optional in this case).render() {...}
: The requiredrender
method that defines what the component will display.return (...)
: Therender
method returns JSX containing adiv
element with an<h1>
tag displaying the message passed as a prop (this.props.message
).
Understanding the Role of the Constructor in Class Components
The constructor, a special method within React class components, plays a crucial role in initialization tasks. While not mandatory for every component, it provides a designated space for setting up essential elements before your component renders for the first time.
- Initializing State: The constructor is a common place to initialize the component’s initial state using the
this.state
object. State allows your component to manage data that can change over time. - Binding Event Handlers: If you’re defining methods that handle user interactions (events) within your class component, you might need to bind
this
to those methods inside the constructor to ensure they have access to the component’s state and functionality.
Example of How to Use the Constructor to Initialize State in a React Class Component
class Counter extends React.Component {
constructor(props) {
super(props); // Call the parent constructor (optional)
this.state = { count: 0 }; // Initialize state with initial count
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
</div>
);
}
}
Explanation
constructor(props) {...}
: The constructor is defined.super(props)
: This line (optional here) calls the constructor of the parent class (React.Component
) to ensure proper inheritance.this.state = {count: 0};
: The state object is initialized with acount
property set to 0.
- The
render
method displays the currentcount
value from the state.
Working with Props in Class Components
React components rely on props for communication. Props are a way to pass data down from parent components to their child components. This one-way data flow promotes better organization and maintainability within your React applications.
- Read-Only Values: Props are read-only within the receiving component. This means child components cannot modify the values passed as props.
- Flexibility: Props allow you to customize the behavior and appearance of child components based on the data they receive.
- Reusability: Using props effectively, you can create reusable components that can adapt to different use cases based on the provided data.
Example of How to Pass Props to a React Class Component
function UserGreeting(props) {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
}
class App extends React.Component {
render() {
return (
<div>
<UserGreeting name="Mark" />
</div>
);
}
}
Explanation
UserGreeting(props)
: This functional component accepts props as an argument.props.name
: This line accesses thename
prop passed from the parent component.
App.js
: The parent component,App
, renders theUserGreeting
component.<UserGreeting name="Mark" />
: Thename
prop is passed with the value “Mark” to the child component.
State Management in React Class Components
State management is a core concept in building interactive React applications. React class components provide a structured way to manage data that can change over time within a component. Here’s a step-by-step breakdown of state management in class components:
- Initializing State: In the constructor, use
this.state = {...}
to define the initial state object with properties representing the component’s data. - Updating State: Use the
setState
method (this.setState({...})
) to modify the state. This method triggers a re-render of the component whenever the state changes. - Accessing State: Within the component’s methods (like
render
), usethis.state
to access and display the current state values.
Example
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 }; // Initialize state with count
}
handleClick = () => {
this.setState({ count: this.state.count + 1 }); // Update state on click
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
Explanation
constructor(props) {...}
: The constructor initializes the state withcount: 0
.handleClick = () => {...}
: This method defines how the state is updated on button click.this.setState({ count: this.state.count + 1 })
: This line usessetState
to update thecount
property by adding 1.
render() {...}
: The component displays the currentcount
value from the state and callshandleClick
on button click.
Nesting Components
Nesting components is a fundamental technique for building complex and well-organized user interfaces in React. It allows you to break down your UI into smaller, reusable components. Each component can manage its state and logic, promoting better maintainability and readability of your codebase.
- Modular Design: Nesting components encourages a modular approach, where complex UIs are composed of smaller, independent building blocks.
- Code Reusability: By nesting reusable components, you can avoid code duplication and ensure consistency across your application.
- Improved Organization: Nesting helps structure your components logically, making your code easier to understand and manage as your project grows.
Example
class ProductCard extends React.Component {
render() {
return (
<div className="product-card">
<img src={this.props.imageUrl} alt={this.props.productName} />
<h3>{this.props.productName}</h3>
<p>{this.props.description}</p>
<ProductPrice price={this.props.price} /> {/* Nested ProductPrice component */}
</div>
);
}
}
class ProductPrice extends React.Component {
render() {
return (
<span className="price">Price: ${this.props.price}</span>
);
}
}
Explanation
ProductCard
: This component displays product information.- It renders an image, heading, description, and nests a
ProductPrice
component to display the price.
- It renders an image, heading, description, and nests a
ProductPrice
: This nested component focuses solely on formatting and displaying the product price.
Organizing Components: Best Practices for File Structure
As your React project grows, maintaining a well-structured codebase becomes crucial. Here are key practices for organizing React class components in separate files:
- Component-Per-File: Each class component ideally resides in its file named after the component (e.g.,
ProductCard.js
). This improves code readability and makes components easier to locate within your project. - Grouping by Feature: Organize components logically by grouping them into folders based on features or functionalities they represent. For instance, components related to user profiles can be grouped within a
/components/UserProfile
folder. - Colocation of Styles: Keep related stylesheets or CSS Modules in the same folder as their corresponding components for better organization and maintainability.
- Separation of Concerns: If you have a large project, consider separating components further based on their role:
- Container Components: Components responsible for managing state and data fetching.
- Presentational Components: Components primarily focused on rendering UI and handling user interactions.
ProductCard.js
class ProductCard extends React.Component {
render() {
return (
<div className="product-card">
{/* ... (rest of the component's JSX) */}
</div>
);
}
}
export default ProductCard;
UserProfile/index.js
// Import related components from within the folder
import UserAvatar from './UserAvatar';
import UserDetails from './UserDetails';
export { UserAvatar, UserDetails };
Explanation
ProductCard.js
: This file holds the code for theProductCard
component.UserProfile/index.js
: This file acts as an entry point for theUserProfile
feature, importing and exporting related components likeUserAvatar
andUserDetails
for cleaner usage in other parts of your application.
Lifecycle Methods of Class Components
React class components have a well-defined lifecycle, allowing you to control their behavior at different stages. These lifecycle methods provide hooks to perform specific actions at key points during a component’s existence. Here is the example and brief explanation of each method:
- Mounting: When a component is first created and inserted into the DOM, methods like
constructor
(for initialization) andcomponentDidMount
(for side effects) are called. - Updating: If a component’s state or props change, methods like
shouldComponentUpdate
(optional optimization),getDerivedStateFromProps
(optional state updates based on props), andcomponentDidUpdate
(for actions after updates) are triggered. - Unmounting: When a component is removed from the DOM, the
componentWillUnmount
method is called to perform any necessary cleanup tasks.
Example of a React Class Component That Demonstrates All the Major Lifecycle Methods
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0, step: props.step }; // Assuming initial step from props to maintain consistency
}
componentDidMount() {
// Simulate fetching data from an API after mount
setTimeout(() => {
this.setState({ count: 10 });
}, 1000);
}
shouldComponentUpdate(nextProps, nextState) {
// Only update if the count value actually changes
return nextState.count !== this.state.count;
}
static getDerivedStateFromProps(nextProps, prevState) {
// Simulate updating state based on a prop change (here, for demonstration)
if (nextProps.step !== prevState.step) {
return {
count: prevState.count + nextProps.step,
step: nextProps.step // Update step in state to ensure future comparisons are correct
};
}
return null; // No change to state
}
componentDidUpdate(prevProps, prevState) {
// Log any state or prop changes for debugging purposes
console.log("Component Updated:", this.state, prevProps, prevState);
}
componentWillUnmount() {
// Cleanup any subscriptions or timers before unmounting
console.log("Cleaning up...");
}
handleClick = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
Explanation
- componentDidMount(): This method is called after the component is inserted into the DOM.
- shouldComponentUpdate(nextProps, nextState): This optional method allows you to optimize performance by controlling when the component should re-render.
- getDerivedStateFromProps(nextProps, prevState): This method is used to update the state based on changes in props.
- componentDidUpdate(prevProps, prevState): This method is called after the component has updated.
- componentWillUnmount(): This method is called when the component is about to be removed from the DOM.
Functionality
- Counter with a Step: This code implements a counter component in React that allows increments with a configurable
step
value, defined as a prop for flexibility. - Fetched Initial Value: It simulates fetching an initial value (10) for the counter after it has been added to the DOM (
componentDidMount
). - Conditional Updates: The component optimizes re-renders by only updating when the
count
value actually changes (shouldComponentUpdate
). - Dynamic Step Adjustment: The component intelligently updates its internal state based on changes to the
step
prop (getDerivedStateFromProps
).
Explanation of Key Parts
- constructor(props)
- Initializes the state with the initial
count
(0) and thestep
(extracted from props).
- Initializes the state with the initial
- handleClick = () => { … }
- This method handles button clicks, incrementing the count by 1 using
setState
.
- This method handles button clicks, incrementing the count by 1 using
- render(): This method defines the component’s UI structure and returns JSX elements to be displayed.
- componentDidMount()
- Simulates fetching data after mounting by setting the count state to 10 after a 1-second timeout.
- shouldComponentUpdate(nextProps, nextState)
- Prevents unnecessary re-renders by returning
true
only if thenextState.count
differs fromthis.state.count
.
- Prevents unnecessary re-renders by returning
- static getDerivedStateFromProps(nextProps, prevState)
- Updates the component’s state if the
step
prop changes. It calculates the new count based on the previous state and the new step value. Keeps thestep
in state updated for consistent calculations.
- Updates the component’s state if the
- componentDidUpdate(prevProps, prevState)
- Logs changes in component state and props for debugging.
- componentWillUnmount()
- Logs a cleanup message for demonstration, typically used for clearing timers, subscriptions, etc.
Class Components vs. Functional Components in React
React offers two main approaches for building components: class components and functional components. Each has its own strengths and use cases:
- Class Components (Stateful): Well-suited for components that manage their own state (data that can change) and utilize lifecycle methods for actions at different stages of a component’s existence. They provide more control and structure for complex components.
- Functional Components (Stateless): Ideal for simpler presentation components that primarily display data and handle user interactions without internal state management. They are generally more concise and easier to reason about.
Class Component (Counter)
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
handleClick = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
Explanation
- This class component manages its own state (
count
) and provides ahandleClick
method to update it.
Functional Component (Greeting)
function Greeting(props) {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
}
Explanation
- This functional component receives a
name
prop and displays a greeting message.
Conclusion
While functional components with hooks are the recommended approach for modern React development, understanding class components remains valuable. This foundation is essential for working with existing codebases and provides insight into React’s evolution and core concepts. Class components introduce state management, lifecycle methods, and a structured approach to building components. Understanding them deepens your overall React knowledge and helps you tackle both legacy and new projects with confidence, solidifying your mastery of the React framework.