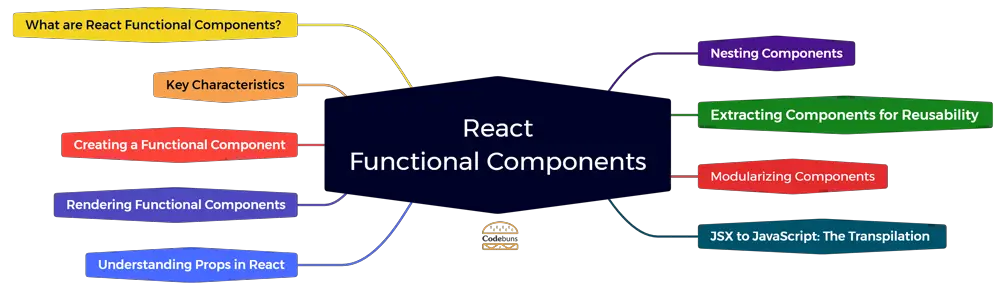
Table of Contents | |
What are React Functional Components?
React functional components are reusable building blocks for creating user interfaces in React applications. They are essentially JavaScript functions that return JSX (JavaScript Syntax Extension) code describing the UI elements to display. These components are lightweight and easy to understand, making them a great starting point for learning React.
Simple Example of a React Functional Component
function Greeting() {
return (
<h1>Hello, ReactJS!</h1>
);
}
Explanation
function Greeting() {...}
: Defines a JavaScript function namedGreeting
.return (...)
: Function must return JSX code, which describes the structure of the UI element this component will render.<h1>Hello, ReactJS!</h1>
: The JSX within thereturn
statement defines anh1
element (a heading) with the static text content “Hello, ReactJS!”. This indicates the component will render a heading with that greeting.
Key Characteristics
React functional components have several key characteristics that make them popular for building UIs. Here are some of the points:
- Declarative: They describe what the UI should look like rather than how to achieve it step-by-step. This makes them easier to understand and reason about.
- Stateless: They don’t hold internal state directly. However, they can access and utilize props (external data) to control their output.
- Pure: Given the same props, a functional component returns the same JSX code, promoting predictability and easier testing.
- Lightweight and Easy to Understand: Compared to class-based components with lifecycle methods, functional components have a simpler syntax and are easier to grasp for beginners learning React.
Example
function Button(props) {
// Button text from props
const text = props.text;
return (
<button>{text}</button>
);
}
Explanation
- This component describes a button (
<button>...</button>
) with its text content coming from thetext
prop. - It doesn’t manage any internal state and relies solely on the provided props to determine its appearance.
- Given the same
text
prop value, it consistently returns the same JSX code.
Creating a Functional Component
Building user interfaces in React applications often involves creating reusable components. React functional components, defined by JavaScript functions, are a great starting point for beginners due to their simplicity and clarity. Let’s explore the steps involved in creating a functional component:
- Define the Function: Start by creating a JavaScript function using the
function
keyword followed by a name (e.g.,function MyComponent() {...}
). This function name will serve as the component’s name. - Optional Props: React functional components can optionally accept arguments called props, which act as external data passed down from parent components to customize the component’s behavior or appearance. You can define props within the function’s parentheses (e.g.,
function MyComponent(props) {...}
). - Return JSX: The core functionality of a React functional component lies in returning JSX (JavaScript Syntax Extension) code. JSX resembles HTML but allows embedding JavaScript expressions for dynamic content. This returned JSX code defines the structure and content of the UI element your component represents.
Example
function Greeting(props) {
// Prop for customization (optional)
const name = props.name || "ReactJS";
return (
<h1>Hello, {name}!</h1>
);
}
Explanation
function Greeting(props) {...}
: Defines a functional component namedGreeting
that can optionally receive props.const name = props.name || "ReactJS";
: Extracts thename
prop value (if provided) or assigns a default value of “ReactJS”.return (...)
: Returns JSX code displaying anh1
element with a greeting message that dynamically includes thename
prop value (or the default value).
Rendering Functional Components
React functional components must be rendered to display their UI elements on the screen. This process involves integrating them into the React application’s hierarchy. Let’s look into the steps involved in rendering React functional components:
- Component Creation: You define your functional components using JavaScript functions that return JSX code.
- Integration into the Application: These functional components are typically called within the JSX code of parent components, effectively nesting them to create the overall UI structure.
- React Takes Over: When React encounters a functional component in the JSX, it executes the function, retrieves the returned JSX code, and transforms it into actual DOM elements in the browser, bringing your UI to life.
Example
//Include Greeting Component from the Previous Example.
function App() {
return (
<div>
<Greeting name="Mark" />
</div>
);
}
Explanation
function App() {...}
: Defines a parent component namedApp
.<Greeting name="Mark" />
: Within theApp
component’s JSX, theGreeting
component is rendered, passing “Mark” as a prop.React
takes the JSX returned byGreeting
and translates it into a corresponding DOM element (anh1
heading) displaying “Hello, Mark!”.
Understanding Props in React
React components rely on props (short for properties) to communicate and share data between them. Props act as a mechanism for passing data downwards from parent components to their child components, a concept often referred to as one-way data flow.
Key Points Regarding Props and One-Way Data Flow
- Data Flow Direction: Data can only be passed from parent to child components using props. This unidirectional flow helps maintain predictable and manageable data flow within your React application.
- Customization: Props allow parent components to customize the behavior and appearance of child components by providing them with specific data or information.
- Read-Only in Child Components: Child components can access and utilize the props they receive but cannot directly modify them. This promotes data consistency and prevents unintended side effects.
Example: Props and One-Way Data Flow
function Greeting(props) {
// Accessing the name prop
const name = props.name;
return (
<h1>Hello, {name}!</h1>
);
}
function App() {
// Data to pass as a prop
const userName = "Bob";
return (
<div>
<Greeting name={userName} />
</div>
);
}
Explanation
function Greeting(props) { ... }
: Defines aGreeting
component that accepts props (including a potentialname
prop).function App() { ... }
: Defines a parent component,App
.const userName = "Bob";
: Stores data to be passed as a prop.<Greeting name={userName} />
: Renders theGreeting
component, passing theuserName
value as thename
prop.- Within the
Greeting
component,const name = props.name;
retrieves the value of thename
prop for use in the JSX.
Nesting Components
React functional components excel at creating reusable building blocks for your UI. But how do you create complex interfaces with multiple components working together? Nesting is a crucial concept that allows you to achieve this.
- Hierarchical Structure: Nesting empowers you to create a hierarchy of components. You can embed functional components within the JSX code of other components. Smaller components forming the building blocks of larger ones.
- Organizing UIs: Nesting promotes organization within your React application. You improve code readability and maintainability by breaking down your UI into smaller, nested components.
Component Nesting Example
function ProfileImage(props) {
return <img src={props.imageUrl} alt="Profile" />;
}
function UserInfo(props) {
return (
<div>
<h2>{props.name}</h2>
<p>{props.bio}</p>
</div>
);
}
function UserProfile(props) {
return (
<div className="user-profile">
<ProfileImage imageUrl={props.imageUrl} /> {/* Nesting ProfileImage */}
<UserInfo name={props.name} bio={props.bio} /> {/* Nesting UserInfo */}
</div>
);
}
Explanation
function ProfileImage(props) {...}
: Defines aProfileImage
component.function UserInfo(props) {...}
: Defines aUserInfo
component.function UserProfile(props) {...}
: Defines aUserProfile
component.<ProfileImage imageUrl={props.imageUrl} />
and<UserInfo name={props.name} bio={props.bio} />
: WithinUserProfile
‘s JSX, bothProfileImage
andUserInfo
components are nested, each receiving appropriate props.
Extracting Components for Reusability
Building UIs often involves repetitive patterns or frequently used elements. React functional components shine when it comes to reusability. Extracting these recurring UI elements into separate functional components allows you to streamline your development process.
- Reduced Code Duplication: Extracting components eliminates the need to write the same UI logic repeatedly, reducing code duplication and making your codebase more concise and easier to maintain.
- Improved Maintainability: Changes to a reusable component propagate throughout your application wherever it’s used.
- Enhanced Readability: Breaking complex UIs into smaller, reusable components improves code readability.
Example
function Button(props) {
return <button>{props.text}</button>;
}
function UserRating(props) {
const rating = props.rating || 0; // Default rating
return (
<div>
User Rating: {rating} stars
<Button text="Leave a Review" /> {/* Reusable Button component */}
</div>
);
}
Explanation
function Button(props) {...}
: Defines a reusableButton
component.function UserRating(props) {...}
: Defines aUserRating
component.<Button text="Leave a Review" />
: TheButton
component is used withinUserRating
, demonstrating reusability.
Modularizing Components
As your React application grows, managing numerous functional components within a single file can become cumbersome. Here’s where separating components into individual files comes into play.
- Improved Organization: You enhance code organization by storing each functional component in its own JavaScript file.
- Enhanced Readability: Each file focuses on a single component, promoting better code readability.
- Easier Collaboration: Modularizing components into separate files facilitates collaboration within development teams.
Button.js
function Button(props) {
return <button>{props.text}</button>;
}
export default Button;
UserRating.js
import Button from './Button'; // Importing the Button component
function UserRating(props) {
const rating = props.rating || 0; // Default rating
return (
<div>
User Rating: {rating} stars
<Button text="Leave a Review" /> {/* Using the imported Button component */}
</div>
);
}
export default UserRating;
Explanation
Button.js
: Defines and exports the reusableButton
component.UserRating.js
: Defines theUserRating
component, importing and using theButton
component from a separate file.
JSX to JavaScript: The Transpilation Process
React components use JSX (JavaScript Syntax Extension) to write UI elements directly within JavaScript code. But browsers don’t understand JSX natively. That’s where JSX transpilation comes in.
Before Transpilation (JSX)
JSX allows you to write code that resembles HTML but with the power of JavaScript expressions embedded within. This syntax improves readability and makes it easier to visualize the UI structure.
Example
function Greeting(props) {
const name = props.name || "ReactJS";
return (
<h1>Hello, {name}!</h1>
);
}
Explanation
- This code defines a
Greeting
component that displays a heading. - The
return
statement uses JSX to define anh1
element with dynamic content ({name}
).
After Transpilation (JavaScript)
Build tools like Babel take your JSX code and transform it into plain JavaScript that browsers can understand. This transpiled code achieves the same functionality as the original JSX, but in a browser-compatible format.
Here’s what the transpiled JavaScript for the above code might look like (exact output may vary slightly depending on the transpiler configuration):
function Greeting(props) {
const name = props.name || "ReactJS";
return React.createElement("h1", null, "Hello, ", name, "!");
}
Explanation
- The transpiled code uses the
React.createElement
function to create JSX elements. - It achieves the same UI structure as the original JSX but uses plain JavaScript function calls.
Conclusion
React functional components are the cornerstone of modern user interface development within the React ecosystem. Their simplicity, emphasis on declarative programming, and ability to embrace reusable logic make them a powerful tool for building scalable and maintainable web applications. As you dive deeper into React, you’ll discover that functional components, coupled with React’s rich features, provide a robust foundation for creating dynamic and interactive user experiences. By mastering React functional components, you’ll enhance your efficiency as a developer to craft exceptional web applications.