The objective of this article and YouTube video is to familiarize you with the C# if Statement. How to use different control flow statements like if Statement, if-else Statement, if else-if Statement, if else-if else Statement, and finally if-else shorthand. Another common arrangement is to have multiple if conditions one inside another called nested if condition.
Table of Contents | |
C# if Statement
C# if statement is one of the most commonly used control flow statement. With if statement, you can tell the computer to make a choice by evaluating a Boolean logical expression (true or false) called condition. It allows you to tell the computer whether to run the code inside the block based on the condition or set of conditions. Each Boolean expression can be independent of the others and can include any of the comparison and logical operators.
C# if statement can be extended by any number of else if clauses to test more conditions. Each additional condition will only be tested if all previous conditions are false. Finally, you can use the else statement at the end to execute some code if none of the above conditions are true.
Here’s the complete structure which lets you use multiple conditions:
if else-if else Syntax with a Block of Statements
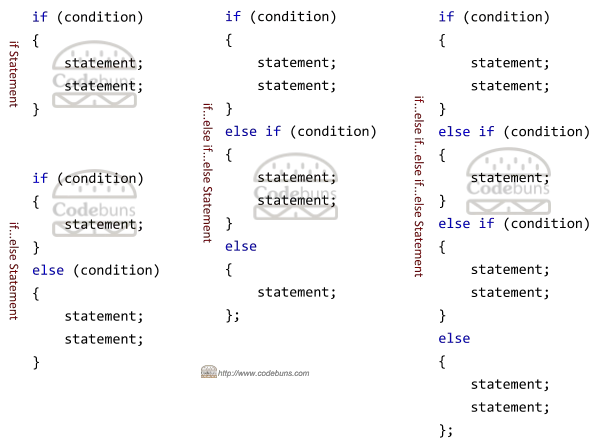
if else-if else flowchart
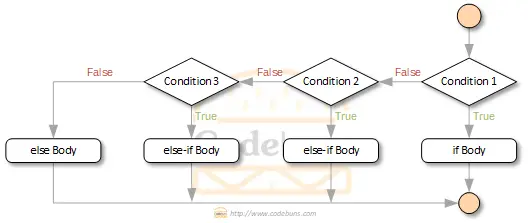
Here, condition is some Boolean expression that evaluates to either true or false, and the statements inside the code block {…} should be executed if a condition is true.
if else-if else Syntax with a Single Statement
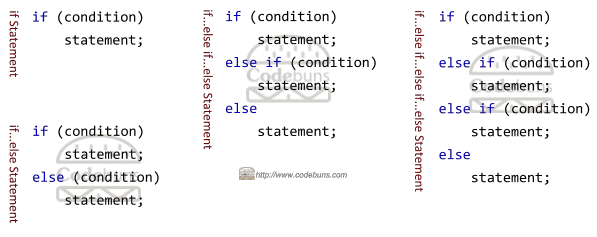
if Condition with a Single Statement
When you only need to execute code for a true condition, use an if statement. It is better to use parentheses for each clause. However, if a clause only contains a single statement, then you can omit the parentheses as in the following example:
if Condition flowchart
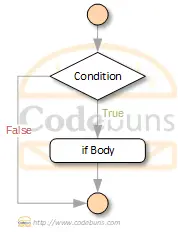
if Condition Example
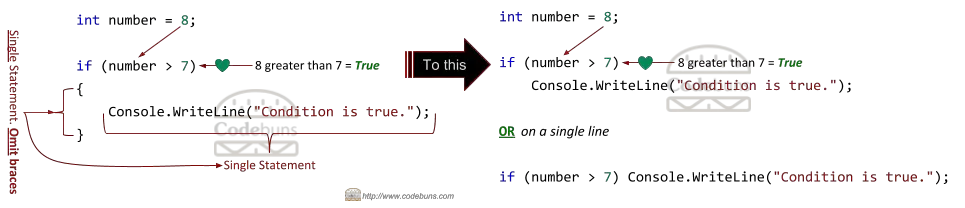
OUTPUT
Condition is true.
Condition is true on a single line.
if Condition with a Block of Statements
Brackets ({ }) would be required for more than one statement. You can code any number of statements between the braces. Coding the braces makes it easy to identify the statements for each clause which makes it easier for you to add more statements later during development.
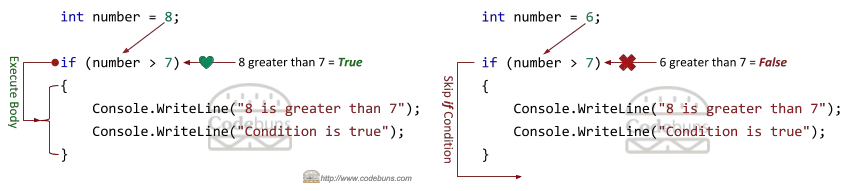
The value of the number variable is 8. So, the expression (number > 7) is evaluated to true because 8 is greater than 7. Hence, the statements inside the body of the if block are executed. If you change the value of the number variable from 8 to 6, then the expression will return false, which will ignore the execution of the if body.
OUTPUT
8 is greater than 7
Condition is true
C# else Statement
Optionally, you can also have an else clause. Whenever the Boolean condition of the if statement is false, the else clause executes as shown in the following code block. Of course, you can use multiple conditions by adding more else if clauses in between if and else.
if-else flowchart
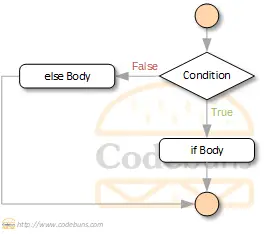
if-else Condition Example
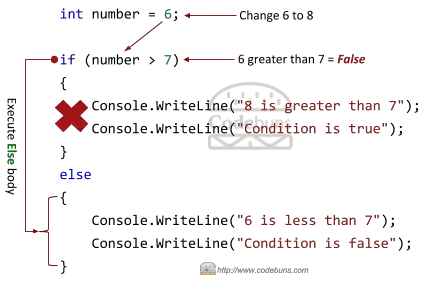
In the example above, the value of the number variable is 6. So the expression (number > 7) is evaluated to false because 6 is less than 7. Hence, the code inside else block is executed. One way of visualizing an if statement is a flow chart. This shows the above if statement in graphical form.
OUTPUT
6 is less than 7
Condition is false
C# else-if Statement
The else-if statement follows directly after the if statement to let you use multiple conditions for testing. Each additional condition will only be evaluated if all previous conditions are false.
if else-if else flowchart
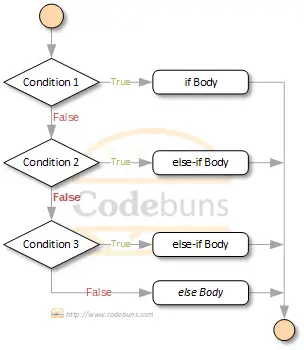
Note: Order of tests is important if you are using an if else-if construct.
if else-if else Condition Example
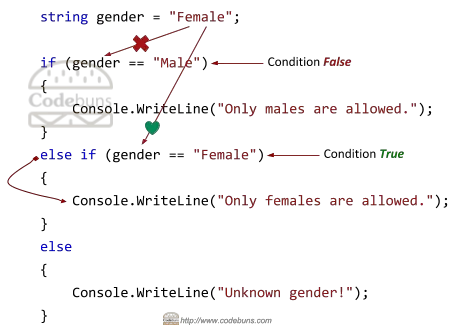
if else-if else Condition Example
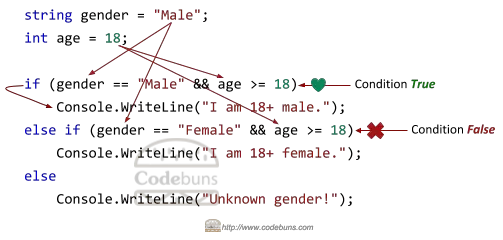
The if statement tests the value of the gender entered. If the gender equals the value Female, everything inside the pair of braces ({ }) is carried out and the rest of if statement will be skipped. On the other hand, if none of the condition is true, then the else block is executed by default.
In the above example, the expression (gender == “Female“) is evaluated to true, which is the condition of the else-if block, and the statement following is executed.
Notice that a test for equality uses the == operator (not the = operator, which is used for assignment).
OUTPUT
Only females are allowed.
Nested if Statement in C#
Another common arrangement is to have multiple if statements one inside another to test multiple conditions. It is called nesting. Nesting helps you create complex programs that rely on chained conditions. There is no limit on the use of nesting that can be achieved with these structures.
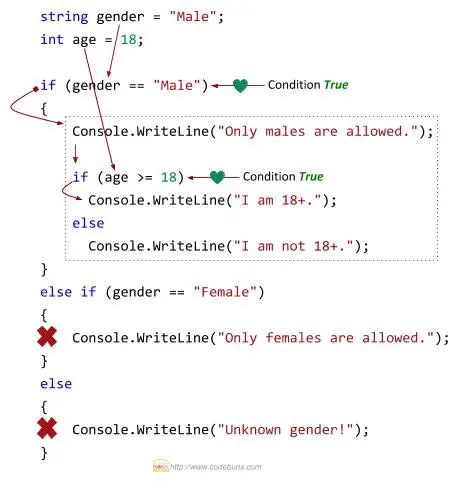
In the above example, the value of the gender variable is equal to Male. So, the expression (gender == “Male“) is evaluated to true, and the program will execute the statement or sequence of statements within the curly braces that follow. Next, you will see that the second if statement is completely contained within the first. This is called nesting. Once the nested if is encountered, the statement (age >= 18) is checked and evaluates to true because 18 is less than or equal to 18. It then prints “I am 18+. “, followed by “Only males are allowed.”
OUTPUT
Only males are allowed.
I am 18+.
if-else shorthand in C#
C# provides a conditional operator “?:”, which is sometimes called C# ternary or question operator. This operator is a shorthand method of writing a simple single line if-else statement.
I have discussed this in detail. Please read C# if else shorthand