The C# foreach loop provides simple syntax to cycle through all the elements in an array or collection unless you explicitly end the loop with the break command.
The structure of a C# foreach loop is as follows:
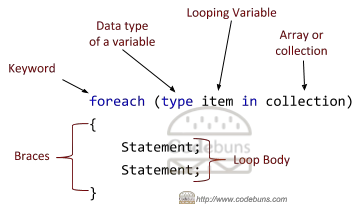
C# foreach loop execution order | C# foreach loop flow diagram |
![]() |
![]() |
The C# foreach loop starts with the foreach keyword followed by parentheses. See the above images.
- The type is used to declare the data-type of the variable. You can use the var keyword, which instructs the compiler to infer the type of the item from the type of the collection.
- The item is the looping variable used inside the loop body into which the next element is automatically acquired from the collection on each iteration. The variable (the iterator) data-type must be the same as the items in the array or list.
- The collection is the array or list representing a number of values over which you want to iterate.
- The statements are the block of code that executes for each iteration of the loop multiple times.
Note: The compiler only allows reading from, not writing to, the collection during the execution of a foreach loop.
C# foreach
Loop with a Single Statement
Curly brackets for the loop are optional, if there is only one statement in the code block.
int[] integerValues = { 1, 2, 3, 4 };
foreach (int value in integerValues)
System.Console.WriteLine(value);
Output
1
2
3
4
C# foreach
Loop with a Block Statements
If you have more than one statement within the loop, you must enclose the statements in the opening and closing braces. You can use braces with the single statement as well.
int[] integerValues = { 1, 2, 3, 4 };
foreach (int value in integerValues)
{
System.Console.WriteLine(value);
}
In the code above, you have an int variable value
that is used for looping. Each time the loop runs, an element in the integerValues array is assigned to the variable value. For example, the first time the loop runs, the number 1 is assigned to value. It then executes the code within the block that makes up the foreach loop body. The line System.Console.Write(value); simply prints out the number 1 on the console. In the second iteration, the number 2 is assigned and printed out. This continues until all the elements in the array have been printed. This means that the code inside the C# foreach loop is called as many times as there are elements in the integerValues array. So, if integerValues contains 4 elements, the code inside the loop block will be executed 4 times.
Output
1
2
3
4
Note: The iterator variable is read-only and can, therefore, not be used to change elements in the array.
int[] integerValues = { 1, 2, 3, 4 };
foreach (int value in integerValues)
{
System.Console.WriteLine($"First Statement : {value}");
System.Console.WriteLine($"Second Statement : {value}");
}
Output
First Statement : 1
Second Statement : 1
First Statement : 2
Second Statement : 2
First Statement : 3
Second Statement : 3
First Statement : 4
Second Statement : 4
More Examples
List Vegetables = new List { "Carrot", "Cucumber", "Tomato", "Onion", "Green Pepper" };
foreach (string veg in Vegetables)
System.Console.WriteLine(veg);
Output
Carrot
Cucumber
Tomato
Onion
Green Pepper
If you use foreach to iterate through a List collection, you cannot use the iteration variable to modify the contents of the collection. Additionally, you cannot call the Remove, Add, or Insert method in a C# foreach loop that iterates through a List collection; any attempt to do so results in an InvalidOperationException exception.
Note: The foreach statement only allows reading from, not writing to, the collection.
public class Planet
{
public int Id { get; set; }
public string Name { get; set; }
}
var planets = new List
{
new Planet() {Id = 1, Name ="Mercury"},
new Planet() {Id = 2, Name = "Venus"},
new Planet() {Id = 3, Name = "Earth"}
};
foreach (var planet in planets)
{
Console.WriteLine($"{planet.Id}, {planet.Name}");
}
Output
1, Mercury
2, Venus
3, Earth
Video: C# foreach
loop
Loop Control Statements
You may need to use jump statements to control the order in which the statements are executed. Read more on …
C# Reference | Microsoft Docs
- C# foreach loop